Using the igloohome API to Get Devices in Javascript
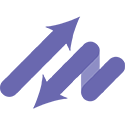
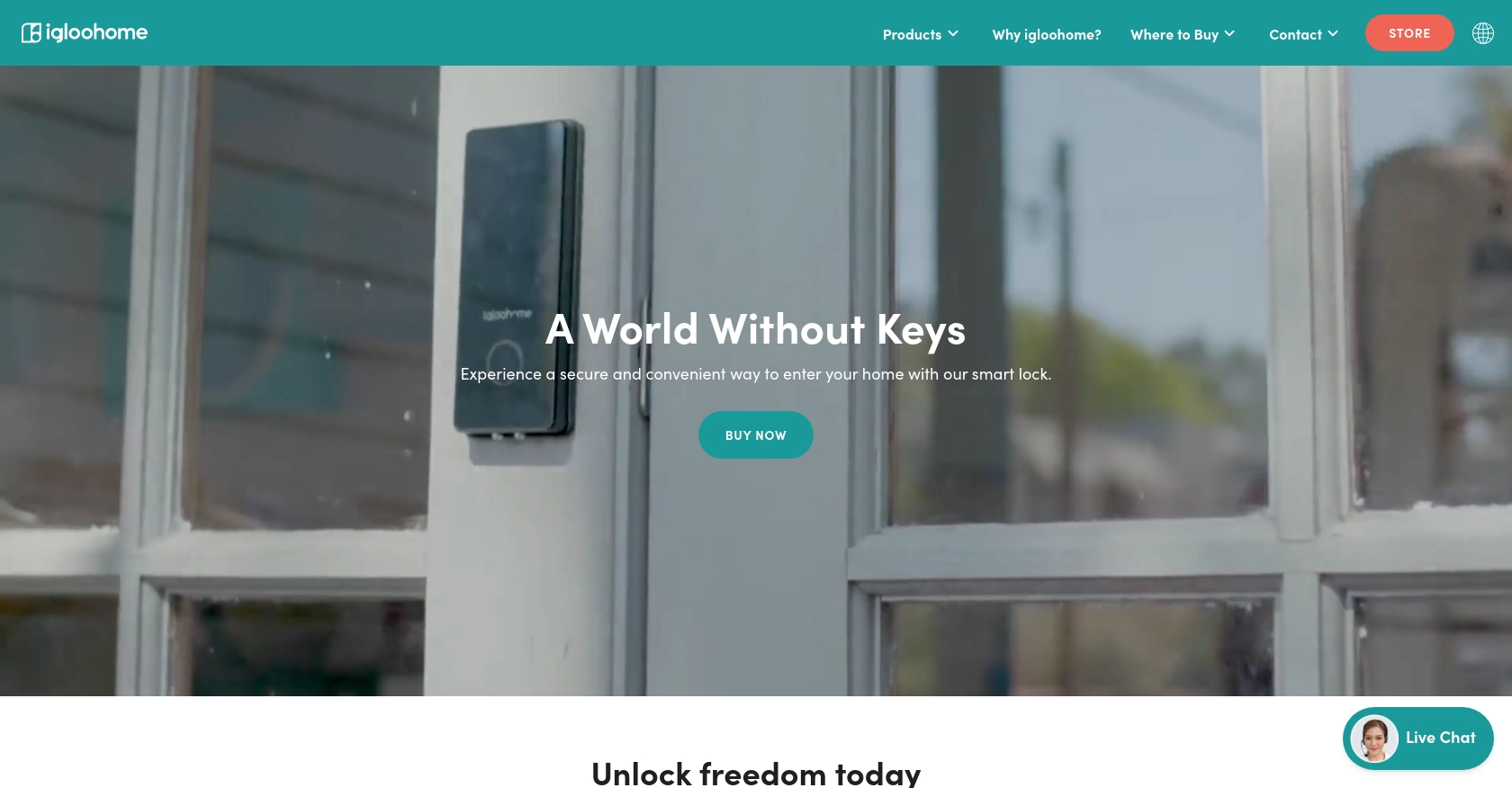
Introduction to igloohome API
igloohome is a leading provider of smart access solutions, offering a range of innovative products designed to enhance security and convenience for both residential and commercial properties. Their solutions include smart locks, keyboxes, and padlocks, all of which can be managed remotely via their comprehensive API.
Developers may want to integrate with the igloohome API to automate and streamline access management processes. For example, by using the API, a developer can retrieve a list of all devices associated with an account, enabling seamless integration with property management systems or custom applications.
This article will guide you through using JavaScript to interact with the igloohome API, specifically focusing on retrieving device information. By following this tutorial, you'll learn how to efficiently access and manage your igloohome devices programmatically.
Setting Up Your igloohome Test/Sandbox Account
Before you can start interacting with the igloohome API, you'll need to set up a test or sandbox account. This will allow you to experiment with the API without affecting any live data. Follow these steps to get started:
Register for an igloohome API Trial
To access the igloohome API, you need to sign up for a 30-day trial. Visit the igloohome registration page and complete the registration process. Once registered, you'll have access to the API and can begin testing.
Create an igloohome App for OAuth Authentication
The igloohome API uses OAuth for authentication. Follow these steps to create an app and obtain the necessary credentials:
- Log in to your igloohome account.
- Navigate to the developer section and select "Create New App."
- Fill in the required details, such as the app name and description.
- Once the app is created, you'll receive a client ID and client secret. Make sure to store these securely as they will be used for authenticating API requests.
Authorize Your App to Access igloohome Devices
With your app created, you need to authorize it to access device information:
- In the app settings, navigate to the "Scopes" section.
- Select the necessary scopes that allow access to device information.
- Save the changes to ensure your app has the required permissions.
With your test account and app set up, you're now ready to start making API calls to retrieve device information using JavaScript. In the next section, we'll cover how to make these calls effectively.
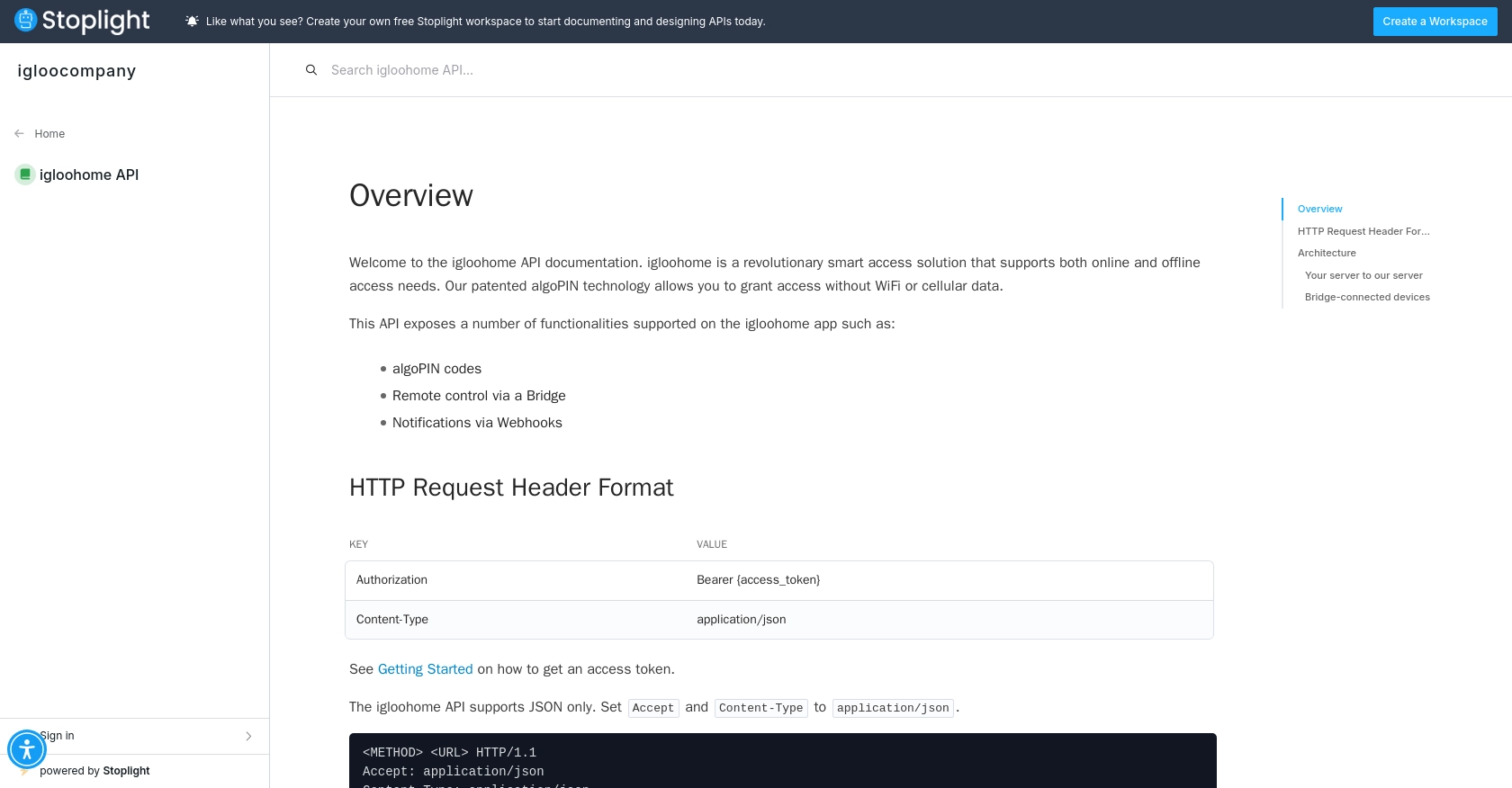
sbb-itb-96038d7
Making API Calls to Retrieve igloohome Devices Using JavaScript
With your igloohome test account and app set up, you can now proceed to make API calls to retrieve device information using JavaScript. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling responses and errors effectively.
Setting Up Your JavaScript Environment for igloohome API Integration
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine. You can download it from the official Node.js website.
- A code editor like Visual Studio Code.
- Install the Axios library for making HTTP requests by running the following command in your terminal:
npm install axios
Writing JavaScript Code to Get Devices from igloohome API
Create a new JavaScript file named getDevices.js
and add the following code:
const axios = require('axios');
// Replace with your client ID and client secret
const clientId = 'YOUR_CLIENT_ID';
const clientSecret = 'YOUR_CLIENT_SECRET';
// Function to get access token
async function getAccessToken() {
try {
const response = await axios.post('https://api.igloohome.co/oauth/token', {
client_id: clientId,
client_secret: clientSecret,
grant_type: 'client_credentials'
});
return response.data.access_token;
} catch (error) {
console.error('Error obtaining access token:', error);
}
}
// Function to get devices
async function getDevices() {
const accessToken = await getAccessToken();
if (!accessToken) return;
try {
const response = await axios.get('https://api.igloohome.co/v1/devices', {
headers: {
Authorization: `Bearer ${accessToken}`
}
});
console.log('Devices:', response.data);
} catch (error) {
console.error('Error fetching devices:', error);
}
}
getDevices();
In this code, we use Axios to handle HTTP requests. The getAccessToken
function retrieves an OAuth access token using your client ID and client secret. The getDevices
function then uses this token to authenticate and fetch the list of devices from the igloohome API.
Running the JavaScript Code and Verifying the Output
To run the code, open your terminal and execute the following command:
node getDevices.js
If successful, you should see a list of devices printed in the console. This output confirms that the API call was successful and that you have retrieved the device information from igloohome.
Handling Errors and igloohome API Response Codes
When making API calls, it's essential to handle potential errors. The igloohome API may return various HTTP status codes indicating the success or failure of a request. Common error codes include:
- 400 Bad Request: The request was invalid. Check your parameters and try again.
- 401 Unauthorized: Authentication failed. Verify your client ID and client secret.
- 404 Not Found: The requested resource does not exist.
- 500 Internal Server Error: An error occurred on the server. Try again later.
By implementing error handling in your code, you can ensure a more robust integration with the igloohome API.
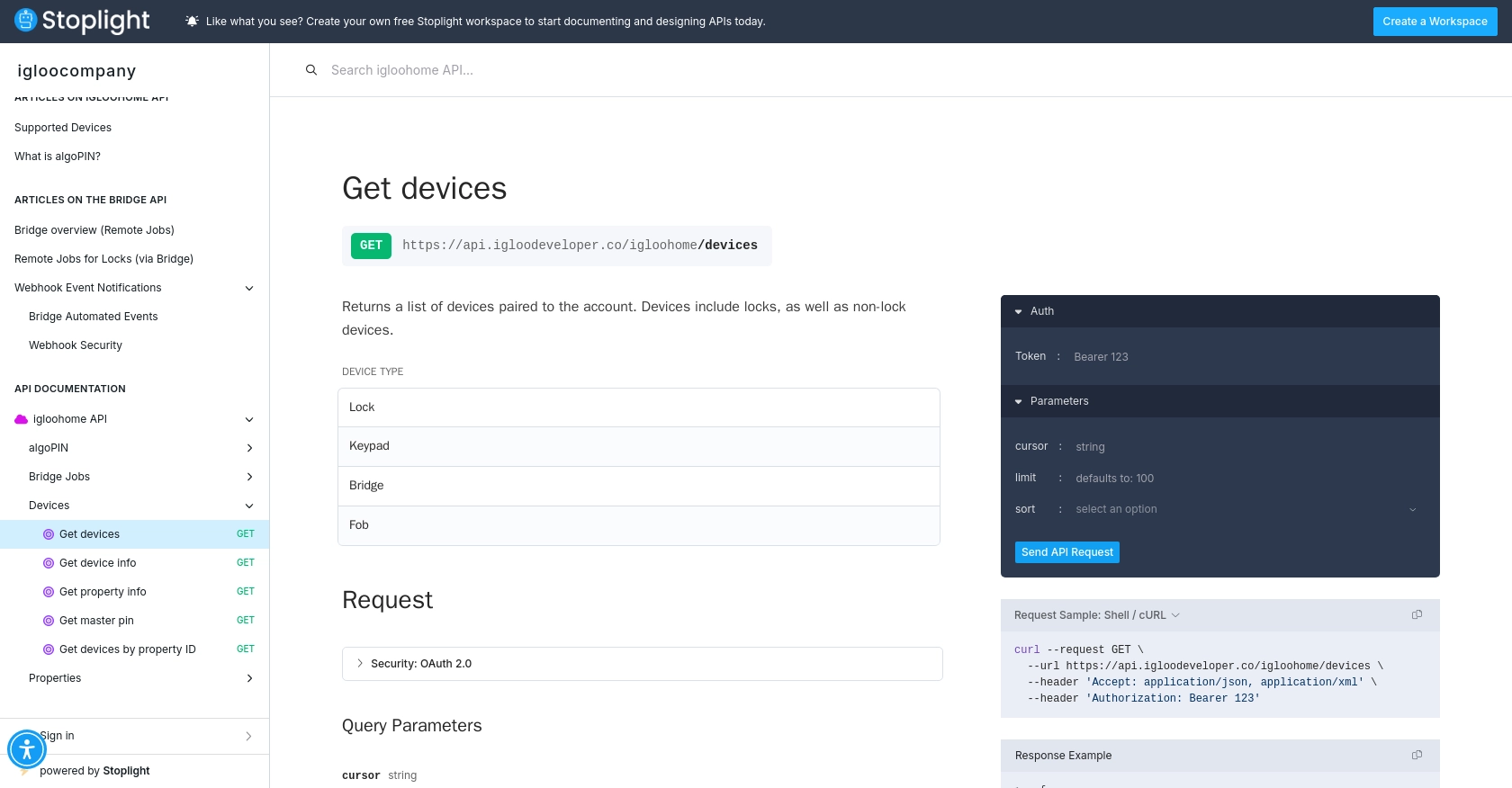
Conclusion and Best Practices for Integrating igloohome API with JavaScript
Integrating with the igloohome API using JavaScript allows developers to efficiently manage smart access devices, enhancing both security and convenience for users. By following the steps outlined in this guide, you can successfully retrieve device information and incorporate it into your applications or systems.
Best Practices for Secure and Efficient igloohome API Integration
- Secure Storage of Credentials: Always store your client ID and client secret securely. Consider using environment variables or a secure vault to prevent unauthorized access.
- Handle Rate Limiting: Be aware of any rate limits imposed by the igloohome API. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that the data retrieved from the API is standardized and transformed as needed to fit your application's requirements.
- Error Handling: Implement comprehensive error handling to manage different HTTP status codes and ensure a robust integration.
Streamline Your Integrations with Endgrate
While integrating with the igloohome API can be straightforward, managing multiple integrations across various platforms can become complex and time-consuming. Endgrate offers a unified API solution that simplifies this process by providing a single endpoint to connect with multiple services, including igloohome.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development while ensuring a seamless integration experience for your customers. Explore how Endgrate can enhance your integration strategy by visiting Endgrate today.
Read More
Ready to get started?