How to Get Balance Transactions with the Stripe API in PHP
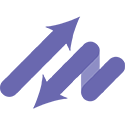
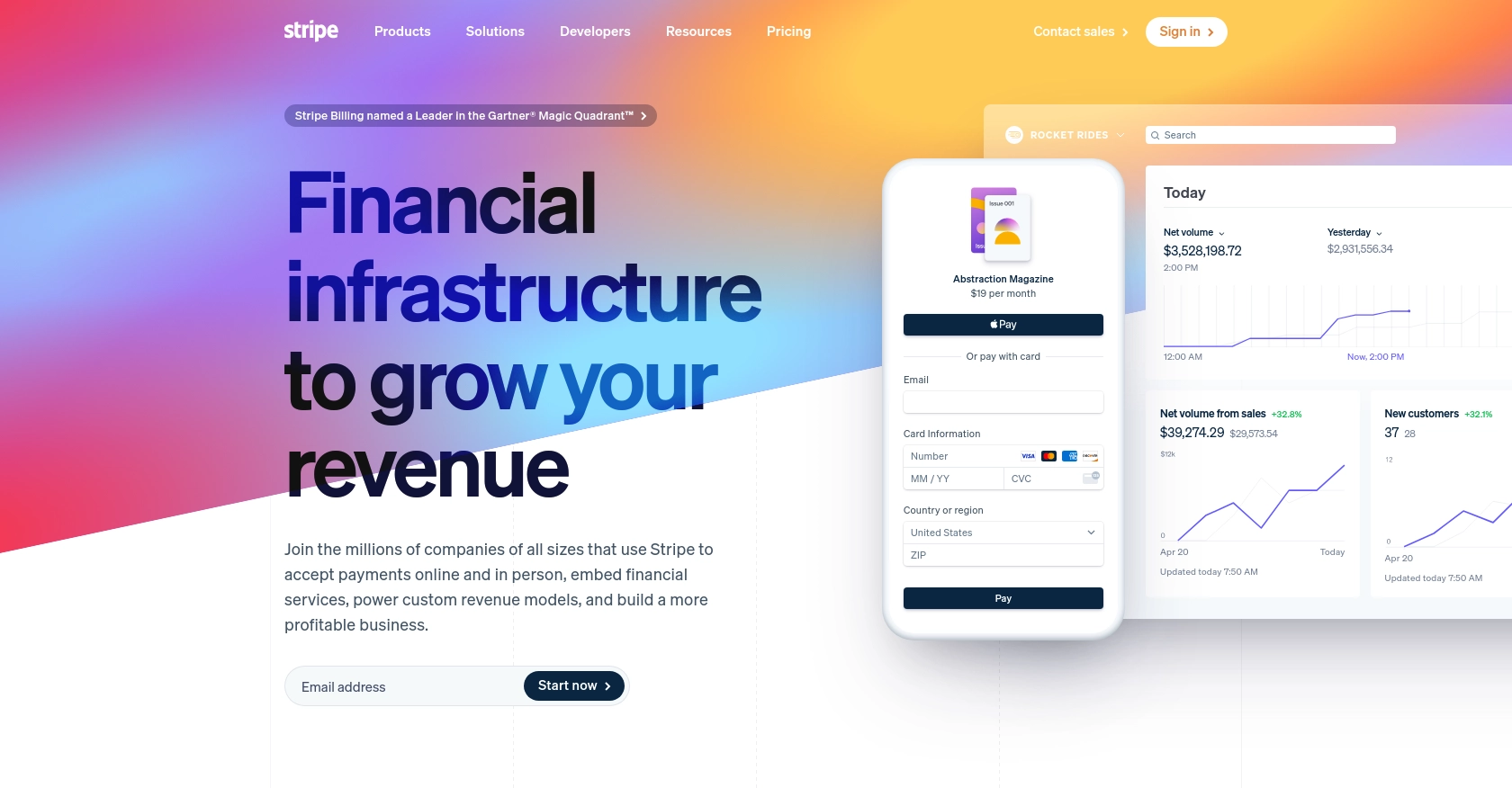
Introduction to Stripe API for Balance Transactions
Stripe is a powerful and flexible payment processing platform that enables businesses to manage their online transactions seamlessly. Known for its robust API and extensive documentation, Stripe is a preferred choice for developers looking to integrate payment solutions into their applications.
By integrating with the Stripe API, developers can access a wide range of financial data, including balance transactions. This capability is crucial for businesses that need to track the flow of funds, manage payouts, and ensure accurate financial reporting. For example, a developer might use the Stripe API to retrieve balance transaction data to generate detailed financial reports or reconcile accounts.
Setting Up Your Stripe Test Account for Balance Transactions
Before you can start interacting with the Stripe API to retrieve balance transactions, you need to set up a test account. Stripe offers a test mode that allows developers to simulate transactions without affecting live data. This is essential for testing and development purposes.
Creating a Stripe Account
If you don't already have a Stripe account, you can sign up for free on the Stripe website. Once your account is created, you'll have access to the Stripe Dashboard, where you can manage your API keys and other settings.
Accessing the Stripe Dashboard
Log in to your Stripe account and navigate to the Dashboard. Here, you can manage your API keys, view test data, and configure other settings necessary for development.
Generating a Stripe API Key for Test Mode
Stripe uses API keys to authenticate requests. To interact with the Stripe API in test mode, you'll need a test API key. Follow these steps to obtain it:
- In the Stripe Dashboard, go to the Developers section.
- Select API keys from the submenu.
- Locate the Secret key under the Test data section. It typically starts with
sk_test_
. - Copy this key and keep it secure, as it will be used to authenticate your API requests.
Configuring API Key in Your PHP Application
Once you have your test API key, you need to configure it in your PHP application. This will allow you to authenticate and make requests to the Stripe API. Here's a basic example of how to set up your API key in PHP:
// Set your secret API key
$stripeApiKey = 'sk_test_your_test_key_here';
// Include the Stripe PHP library
require 'vendor/autoload.php';
// Set the API key for Stripe
\Stripe\Stripe::setApiKey($stripeApiKey);
Replace sk_test_your_test_key_here
with your actual test API key.
Testing Your Stripe Integration
With your test account and API key set up, you can now proceed to test your integration. Use the test mode to simulate balance transactions and verify that your application correctly interacts with the Stripe API. This ensures that your integration is robust and ready for production.
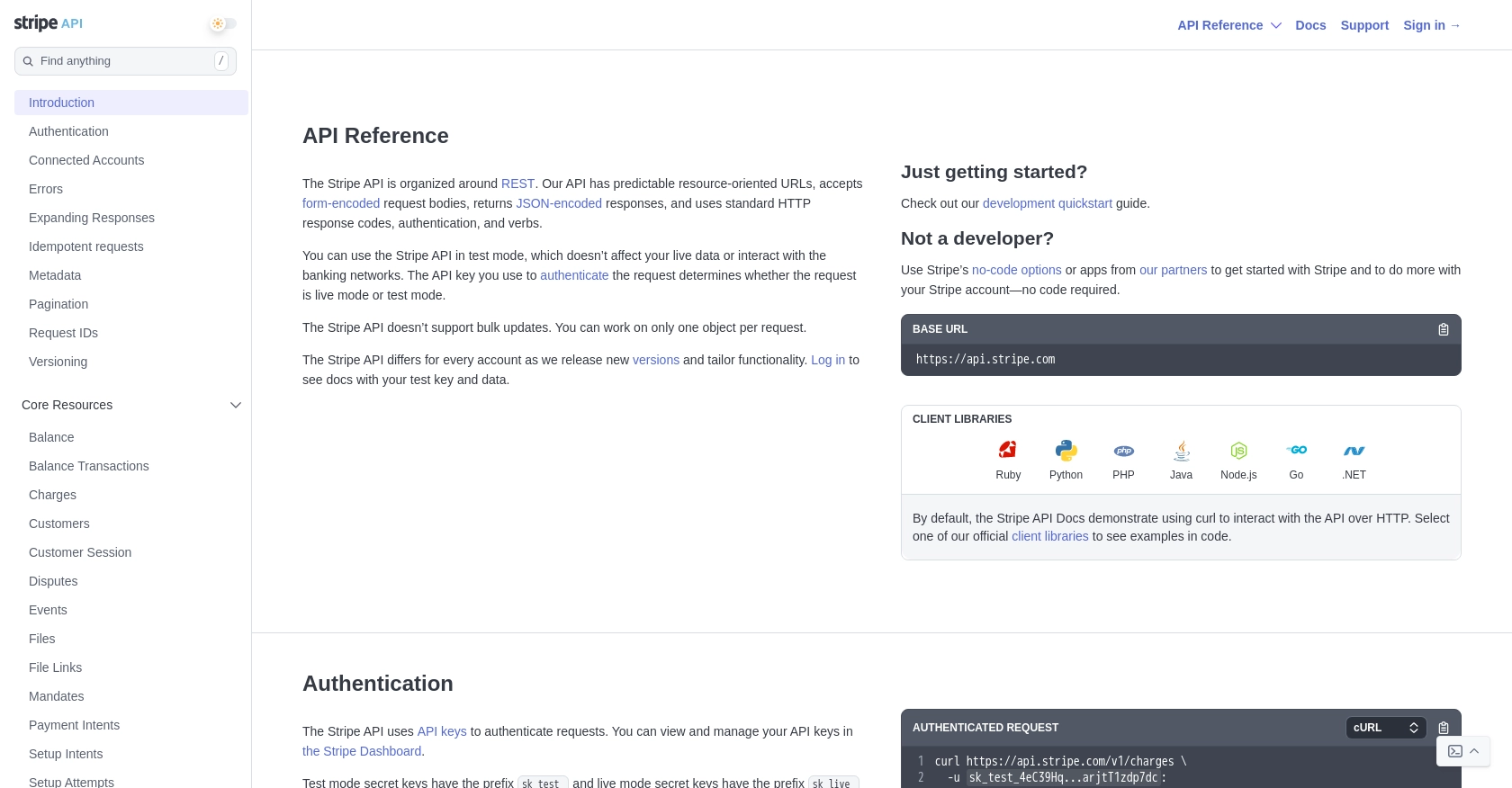
sbb-itb-96038d7
Making API Calls to Retrieve Balance Transactions with Stripe API in PHP
To retrieve balance transactions using the Stripe API in PHP, you'll need to set up your environment with the necessary tools and libraries. This section will guide you through the process of making API calls to access balance transaction data, ensuring your application can effectively interact with Stripe's robust financial services.
Setting Up Your PHP Environment for Stripe API Integration
Before making API calls, ensure your PHP environment is properly configured. You'll need PHP version 7.2 or higher and the Stripe PHP library. You can install the Stripe library using Composer, a dependency manager for PHP:
composer require stripe/stripe-php
This command will add the Stripe library to your project, allowing you to interact with the Stripe API seamlessly.
Writing PHP Code to Retrieve Balance Transactions from Stripe
With your environment set up, you can now write the PHP code to make an API call to Stripe and retrieve balance transactions. Create a new PHP file and add the following code:
10]);
// Display the balance transactions
foreach ($balanceTransactions->data as $transaction) {
echo 'ID: ' . $transaction->id . '<br>';
echo 'Amount: ' . $transaction->amount . '<br>';
echo 'Currency: ' . $transaction->currency . '<br>';
echo 'Description: ' . $transaction->description . '<br><br>';
}
} catch (\Stripe\Exception\ApiErrorException $e) {
// Handle error
echo 'Error: ' . $e->getMessage();
}
?>
Replace sk_test_your_test_key_here
with your actual test API key. This code initializes the Stripe library, sets the API key, and retrieves a list of balance transactions. It then iterates through the transactions and displays their details.
Understanding the Sample Output of Stripe Balance Transactions
When you run the above PHP script, you should see a list of balance transactions displayed in your browser or terminal. Each transaction includes details such as the transaction ID, amount, currency, and description. This output helps you verify that your API call was successful and that you are correctly retrieving data from Stripe.
Handling Errors and Verifying API Call Success
Stripe uses conventional HTTP response codes to indicate the success or failure of an API request. Successful requests return codes in the 2xx range, while errors return codes in the 4xx or 5xx range. The code sample includes error handling to catch and display any exceptions that occur during the API call.
For more detailed information on error codes and handling, refer to the Stripe API documentation.
Conclusion and Best Practices for Using Stripe API in PHP
Integrating with the Stripe API to retrieve balance transactions in PHP offers a powerful way to manage and analyze financial data. By following the steps outlined in this guide, you can efficiently access transaction details, enabling accurate financial reporting and reconciliation.
Best Practices for Secure and Efficient Stripe API Integration
- Secure API Keys: Always keep your API keys secure and never expose them in client-side code or public repositories. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Stripe imposes rate limits on API requests. Implement exponential backoff strategies to handle
429 Too Many Requests
errors gracefully. - Data Standardization: Ensure consistent data formats when processing transactions. This helps in maintaining data integrity across different systems.
- Error Handling: Implement robust error handling to manage different types of API errors. Refer to the Stripe API documentation for detailed error code explanations.
Streamline Your Integration Process with Endgrate
While integrating with Stripe is straightforward, managing multiple integrations can become complex. Endgrate simplifies this process by providing a unified API endpoint for various platforms, including Stripe. This allows you to focus on your core product while Endgrate handles the intricacies of integration.
Visit Endgrate to learn how you can save time and resources by outsourcing your integration needs, ensuring a seamless experience for your customers.
Read More
Ready to get started?