Using the Salesloft API to Get Accounts in Python
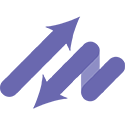
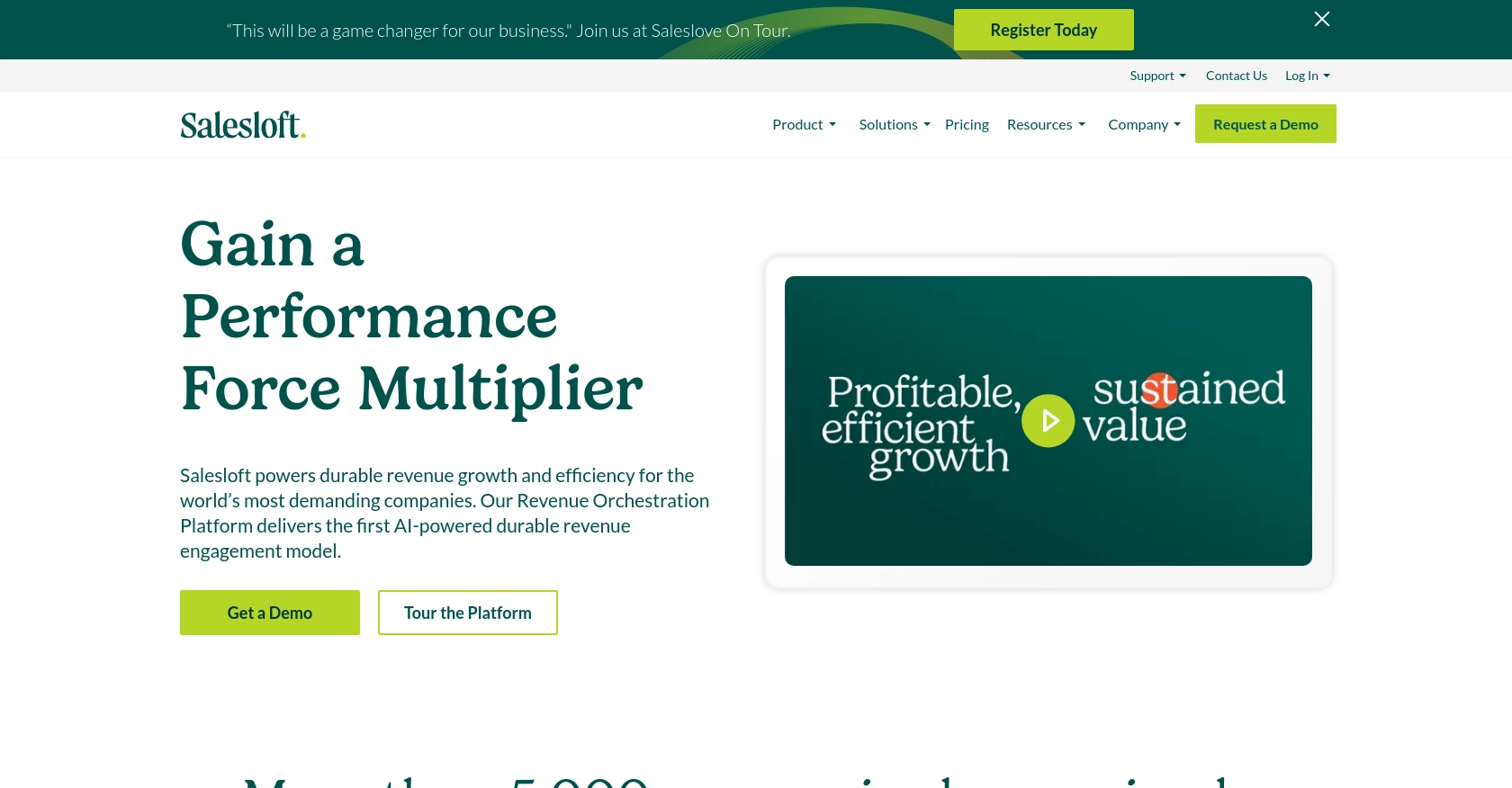
Introduction to Salesloft API Integration
Salesloft is a powerful sales engagement platform that enables sales teams to connect with prospects more effectively. It offers a suite of tools designed to streamline the sales process, enhance productivity, and improve communication with potential clients.
Integrating with the Salesloft API allows developers to access and manage sales data programmatically. This can be particularly useful for automating tasks such as retrieving account information, which can help sales teams maintain up-to-date records and improve their outreach strategies.
For example, a developer might use the Salesloft API to fetch account details and integrate them into a custom CRM system, ensuring that sales representatives have the most current information at their fingertips.
Setting Up Your Salesloft Test Account for API Integration
Before you can start interacting with the Salesloft API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data.
Create a Salesloft Account
If you don't already have a Salesloft account, you can sign up for a free trial on the Salesloft website. This will give you access to the platform's features and allow you to create a sandbox environment for testing.
- Visit the Salesloft website and click on "Sign Up."
- Follow the on-screen instructions to create your account.
- Once your account is set up, log in to access the Salesloft dashboard.
Creating an OAuth App in Salesloft
To interact with the Salesloft API, you'll need to create an OAuth application. This will provide you with the necessary credentials to authenticate your API requests.
- Navigate to Your Applications in your Salesloft account.
- Select OAuth Applications and click on Create New.
- Fill in the required fields and click Save.
- After saving, you'll receive your Application Id (Client Id) and Secret (Client Secret).
Obtaining Authorization Code and Access Tokens
With your OAuth app set up, you can now obtain an authorization code and access tokens to authenticate your API requests.
- Generate a request to the authorization endpoint using your
Client Id
andRedirect URI
: - Authorize the application when prompted.
- Upon approval, your
redirect_uri
will receive a query parametercode
. - Use this code to obtain access and refresh tokens by making a POST request:
- Store the
access_token
andrefresh_token
securely for future use.
https://accounts.salesloft.com/oauth/authorize?client_id=YOUR_CLIENT_ID&redirect_uri=YOUR_REDIRECT_URI&response_type=code
POST https://accounts.salesloft.com/oauth/token
{
"client_id": "YOUR_CLIENT_ID",
"client_secret": "YOUR_CLIENT_SECRET",
"code": "YOUR_AUTHORIZATION_CODE",
"grant_type": "authorization_code",
"redirect_uri": "YOUR_REDIRECT_URI"
}
For more details, refer to the Salesloft OAuth Authentication documentation.
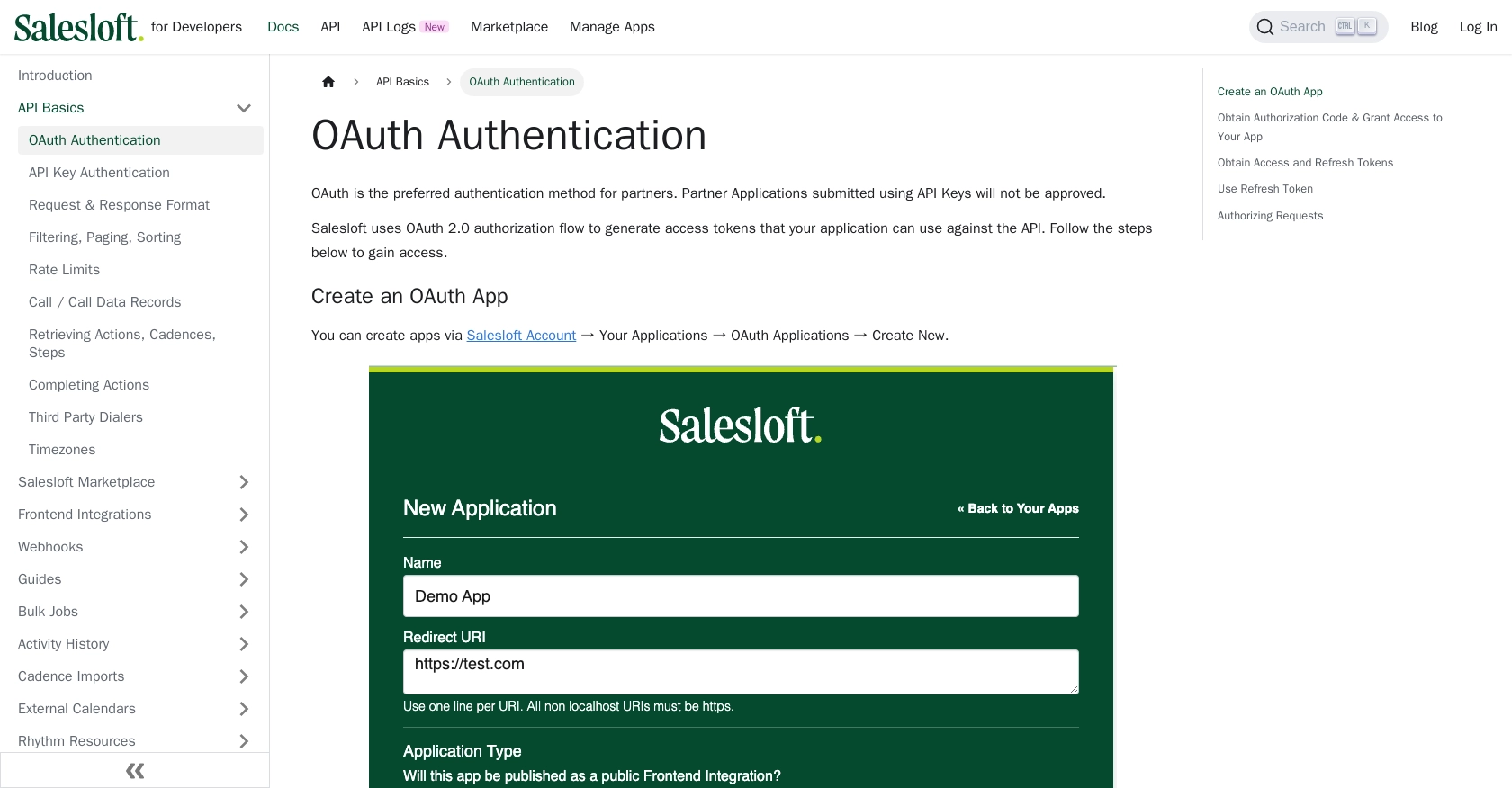
sbb-itb-96038d7
Making API Calls to Retrieve Salesloft Accounts Using Python
To interact with the Salesloft API and retrieve account information, you'll need to use Python. This section will guide you through setting up your environment, making the API call, and handling the response.
Setting Up Your Python Environment for Salesloft API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
- Install Python 3.11.1 from the official Python website if you haven't already.
- Use pip to install the
requests
library by running the following command in your terminal:
pip install requests
Example Code to Fetch Accounts from Salesloft API
Now that your environment is set up, you can write a Python script to fetch account data from Salesloft. Create a file named get_salesloft_accounts.py
and add the following code:
import requests
# Set the API endpoint and headers
endpoint = "https://api.salesloft.com/v2/accounts"
headers = {
"Authorization": "Bearer YOUR_ACCESS_TOKEN",
"Content-Type": "application/json"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
# Parse the JSON data from the response
data = response.json()
# Loop through the accounts and print their information
for account in data['data']:
print(f"Account Name: {account['name']}, Domain: {account['domain']}")
else:
print(f"Failed to retrieve accounts: {response.status_code} - {response.text}")
Replace YOUR_ACCESS_TOKEN
with the access token you obtained during the OAuth setup.
Running the Python Script and Verifying the Output
Execute the script from your terminal using the following command:
python get_salesloft_accounts.py
If successful, you should see a list of account names and domains printed in your terminal. This confirms that the API call was made correctly and the data was retrieved.
Handling Errors and Understanding Salesloft API Response Codes
It's crucial to handle potential errors when making API calls. The Salesloft API may return various status codes indicating the success or failure of your request:
- 200 OK: The request was successful, and the accounts were retrieved.
- 403 Forbidden: You do not have permission to access the resource. Check your access token.
- 404 Not Found: The requested resource could not be found.
- 422 Unprocessable Entity: There was an issue with the request parameters.
For more detailed information on error handling, refer to the Salesloft Request & Response Format documentation.
Verifying API Call Success in Salesloft Sandbox
To ensure the API call was successful, you can log into your Salesloft sandbox account and verify that the account data matches the output from your script. This step helps confirm that your integration is working as expected.
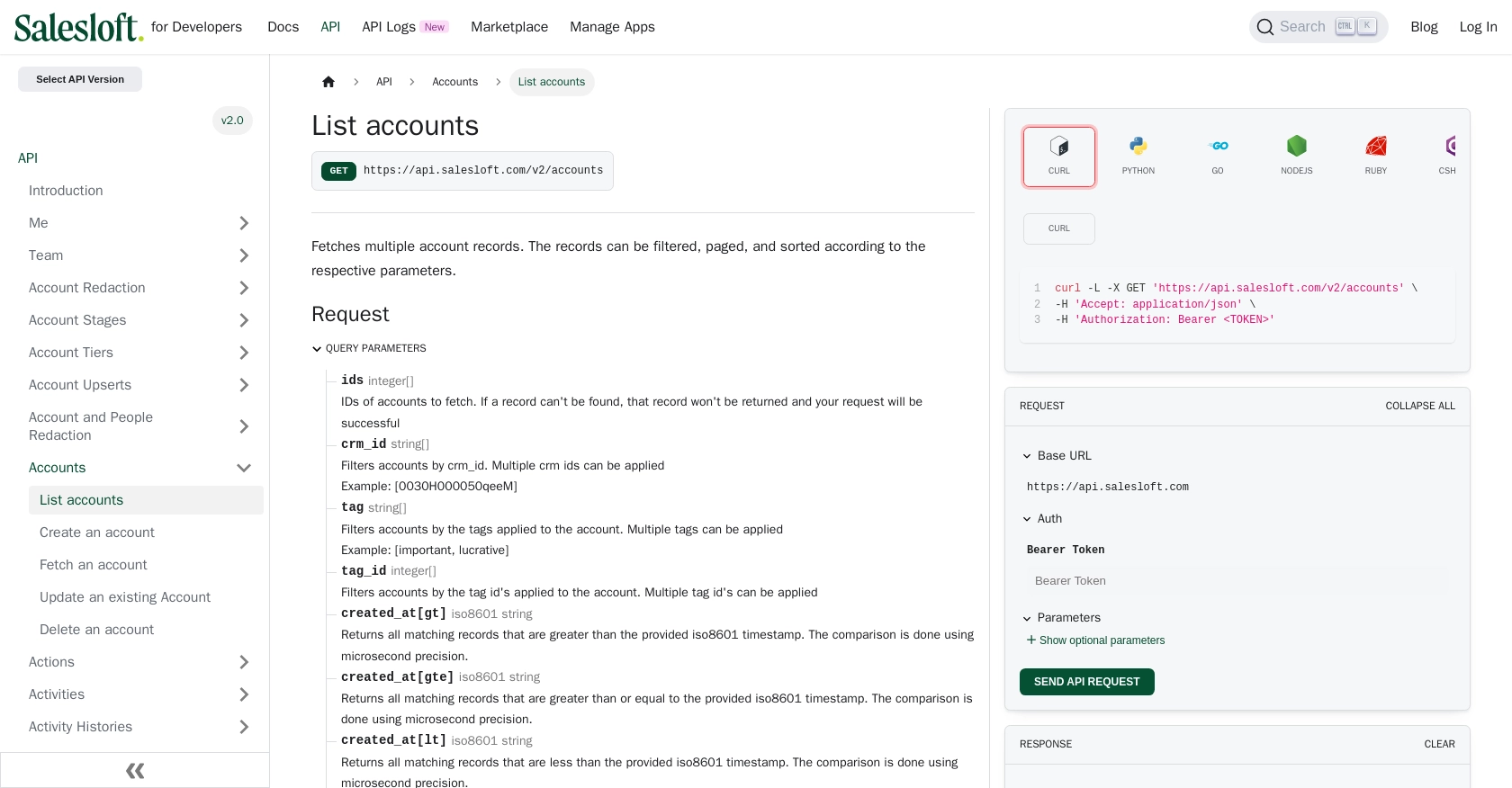
Conclusion and Best Practices for Salesloft API Integration
Integrating with the Salesloft API using Python provides a powerful way to automate and streamline your sales processes. By following the steps outlined in this guide, you can efficiently retrieve account information and incorporate it into your custom applications or CRM systems.
Best Practices for Secure and Efficient Salesloft API Usage
- Securely Store Credentials: Always store your
access_token
andrefresh_token
securely. Consider using environment variables or a secure vault to protect sensitive information. - Handle Rate Limits: Be mindful of Salesloft's rate limits, which are set at 600 cost per minute. Plan your API calls accordingly to avoid hitting these limits. For more details, refer to the Salesloft Rate Limits documentation.
- Implement Error Handling: Ensure your application gracefully handles errors by checking response codes and implementing retry logic where appropriate.
- Optimize Data Handling: Use filtering, paging, and sorting to manage large datasets efficiently. This helps reduce the load on your application and ensures faster response times.
Enhance Your Integration Strategy with Endgrate
While integrating with Salesloft directly can be effective, using a tool like Endgrate can further simplify the process. Endgrate offers a unified API endpoint that connects to multiple platforms, including Salesloft, allowing you to manage integrations more efficiently.
With Endgrate, you can focus on your core product development while outsourcing the complexities of integration management. This approach not only saves time and resources but also provides an intuitive experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website and discover the benefits of a streamlined integration process.
Read More
- https://endgrate.com/provider/salesloft
- https://developers.salesloft.com/docs/platform/api-basics/oauth-authentication/
- https://developers.salesloft.com/docs/platform/api-basics/request-response-format/
- https://developers.salesloft.com/docs/platform/api-basics/filtering-paging-sorting/
- https://developers.salesloft.com/docs/platform/api-basics/rate-limits/
- https://developers.salesloft.com/docs/api/accounts-index/
Ready to get started?