Using the Younium API to Create or Update Products (with PHP examples)
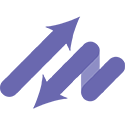
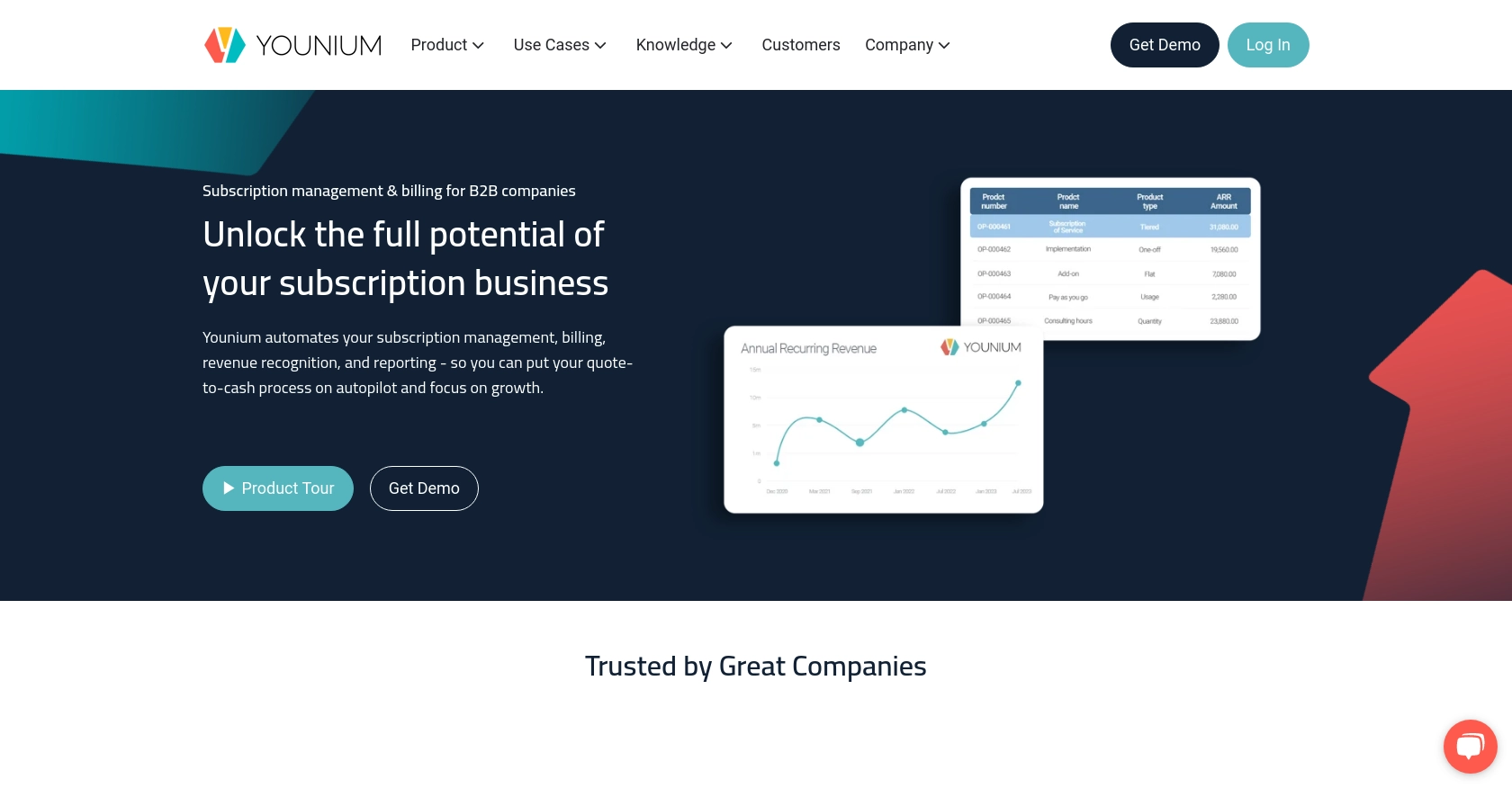
Introduction to Younium API Integration
Younium is a comprehensive subscription management platform designed to streamline billing, invoicing, and financial operations for B2B SaaS companies. With its robust API, Younium enables developers to automate and enhance various business processes, ensuring seamless integration with existing systems.
Integrating with the Younium API allows developers to efficiently manage product data, such as creating or updating product information. For example, a developer might use the Younium API to automate the process of updating product pricing or descriptions in response to market changes, ensuring that all systems reflect the latest information.
Setting Up Your Younium Test/Sandbox Account for API Integration
Before diving into the Younium API integration, it's essential to set up a test or sandbox account. This environment allows developers to experiment with API calls without affecting live data, ensuring a safe and controlled testing space.
Creating a Younium Sandbox Account
To begin, you'll need to create a sandbox account on the Younium platform. Follow these steps:
- Visit the Younium Developer Portal.
- Sign up for a sandbox account if you don't already have one. This typically involves providing your email and setting a password.
- Once registered, log in to your sandbox account to access the developer dashboard.
Generating API Credentials for Younium
With your sandbox account ready, the next step is to generate the necessary API credentials. These credentials will allow you to authenticate your API requests:
- Navigate to your user profile by clicking your name in the top right corner.
- Select “Privacy & Security” from the dropdown menu.
- In the left panel, click on “Personal Tokens” and then “Generate Token.”
- Provide a relevant description for your token and click “Create.”
- Copy the generated Client ID and Secret Key. These will not be visible again, so store them securely.
Acquiring a JWT Access Token
To authenticate API requests, you'll need a JWT access token. Follow these steps to acquire one:
// Set the API endpoint for token generation
$url = "https://api.sandbox.younium.com/auth/token";
// Prepare the request headers and body
$headers = [
"Content-Type: application/json"
];
$body = json_encode([
"clientId" => "Your_Client_ID",
"secret" => "Your_Secret_Key"
]);
// Use cURL to make the POST request
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_POSTFIELDS, $body);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
// Decode the response to get the access token
$data = json_decode($response, true);
$accessToken = $data['accessToken'];
Replace Your_Client_ID
and Your_Secret_Key
with the credentials you generated earlier. The access token is valid for 24 hours, after which you will need to request a new one.
Handling Authentication Errors
If you encounter errors during authentication, refer to the error codes provided by Younium:
- 400 Bad Request: Indicates invalid credentials or malformed request.
- 401 Unauthorized: The access token is expired, missing, or incorrect.
- 403 Forbidden: The request is authorized but the action is forbidden, possibly due to incorrect legal entity information.
For more details, consult the Younium Authentication Documentation.
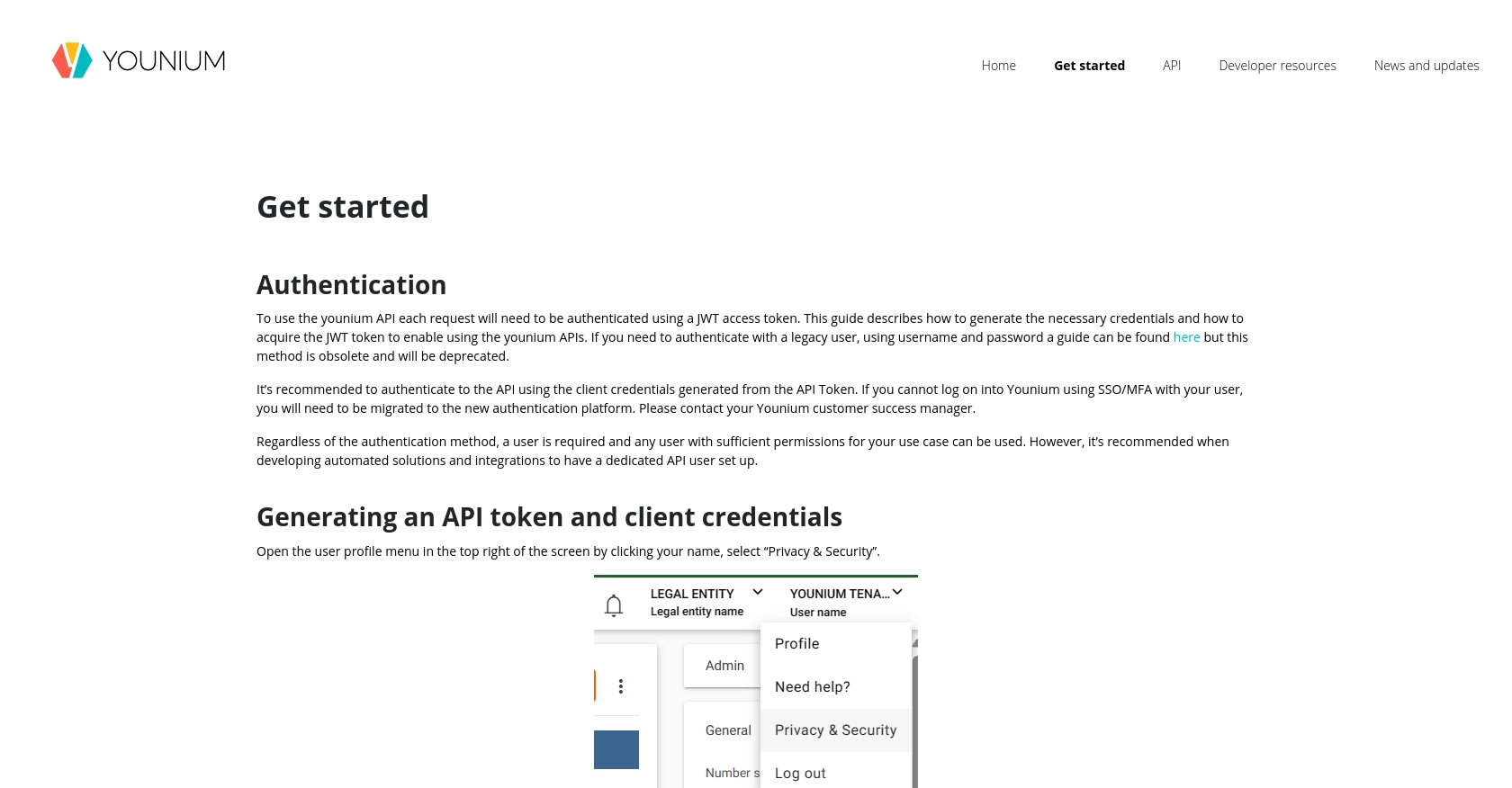
sbb-itb-96038d7
Making API Calls to Create or Update Products in Younium Using PHP
With your JWT access token in hand, you can now make API calls to Younium to create or update product information. This section will guide you through the process using PHP, ensuring you can efficiently manage product data within the Younium platform.
Setting Up Your PHP Environment for Younium API Integration
Before making API calls, ensure your PHP environment is correctly set up. You'll need:
- PHP 7.4 or higher
- cURL extension enabled
These prerequisites will allow you to execute HTTP requests and handle JSON data effectively.
Creating a Product in Younium Using PHP
To create a product, you'll need to make a POST request to the Younium API. Here's a step-by-step guide:
// Define the API endpoint for creating a product
$url = "https://api.sandbox.younium.com/products";
// Set the request headers, including the JWT token
$headers = [
"Authorization: Bearer Your_Access_Token",
"Content-Type: application/json"
];
// Define the product data
$productData = json_encode([
"name" => "New Product",
"description" => "Description of the new product",
"price" => 100.00
]);
// Initialize cURL session
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_POSTFIELDS, $productData);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Execute the request
$response = curl_exec($ch);
curl_close($ch);
// Decode the response
$result = json_decode($response, true);
// Output the result
if (isset($result['id'])) {
echo "Product created successfully with ID: " . $result['id'];
} else {
echo "Failed to create product: " . $result['error'];
}
Replace Your_Access_Token
with the JWT token obtained earlier. This script sends a POST request to create a new product, and if successful, it will return the product ID.
Updating a Product in Younium Using PHP
To update an existing product, you'll need to make a PUT request. Here's how:
// Define the API endpoint for updating a product
$productId = "Existing_Product_ID";
$url = "https://api.sandbox.younium.com/products/" . $productId;
// Set the request headers, including the JWT token
$headers = [
"Authorization: Bearer Your_Access_Token",
"Content-Type: application/json"
];
// Define the updated product data
$updateData = json_encode([
"name" => "Updated Product Name",
"description" => "Updated description",
"price" => 150.00
]);
// Initialize cURL session
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, "PUT");
curl_setopt($ch, CURLOPT_POSTFIELDS, $updateData);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Execute the request
$response = curl_exec($ch);
curl_close($ch);
// Decode the response
$result = json_decode($response, true);
// Output the result
if (isset($result['id'])) {
echo "Product updated successfully with ID: " . $result['id'];
} else {
echo "Failed to update product: " . $result['error'];
}
Replace Your_Access_Token
and Existing_Product_ID
with the appropriate values. This script updates the specified product and returns the updated product ID if successful.
Verifying API Call Success in Younium
After making API calls, verify their success by checking the Younium sandbox account. Ensure that the created or updated product appears as expected. If there are discrepancies, review the API response for error messages and adjust your request accordingly.
Handling Errors and Common Issues with Younium API Calls
When making API calls, you might encounter errors. Here are some common issues and their solutions:
- 400 Bad Request: Check your request body for missing or incorrect fields.
- 401 Unauthorized: Ensure your JWT token is valid and not expired.
- 403 Forbidden: Verify the legal entity information in your request headers.
For more detailed error handling, refer to the Younium API Documentation.
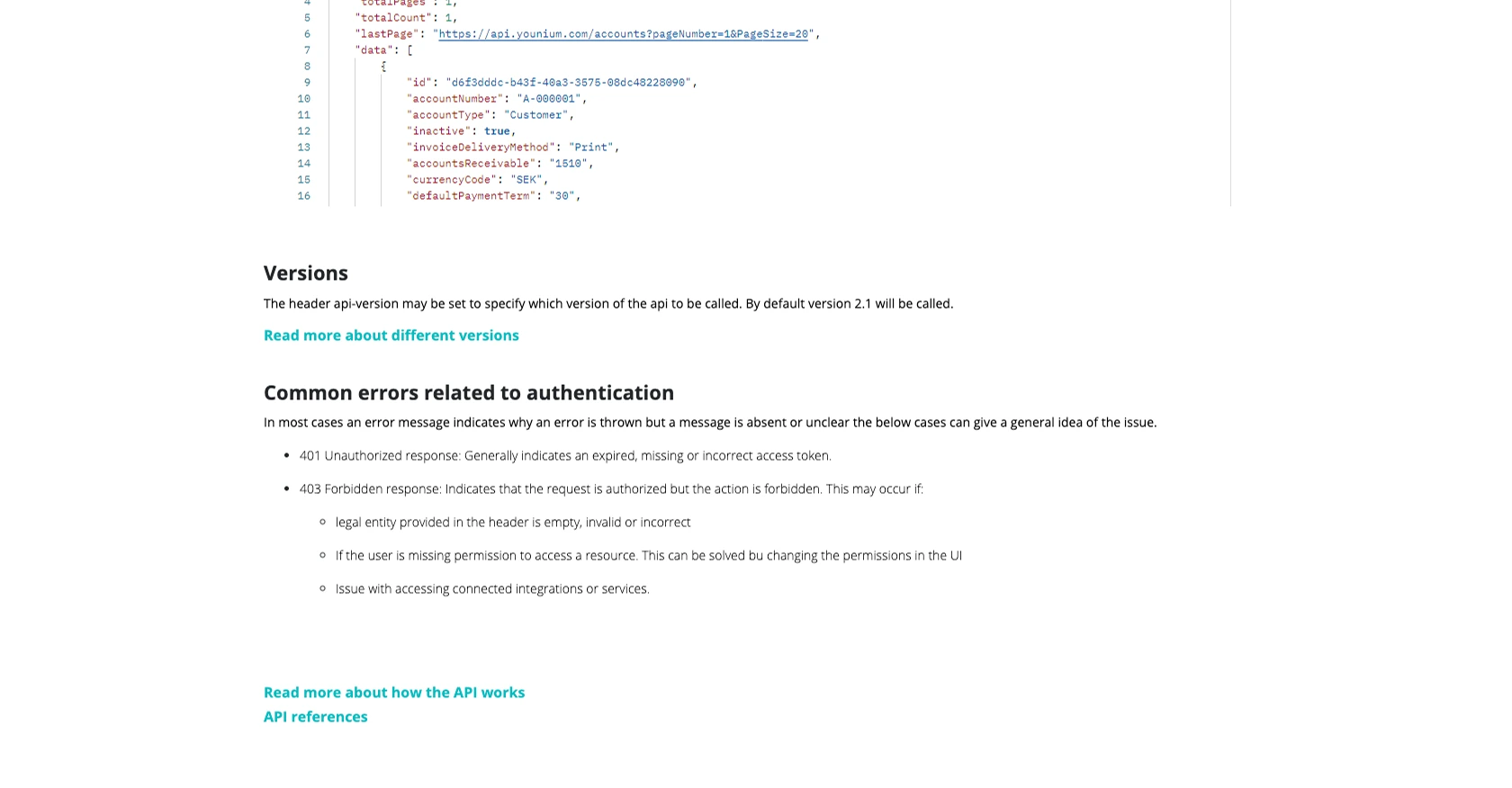
Conclusion and Best Practices for Younium API Integration
Integrating with the Younium API using PHP allows developers to efficiently manage product data, automate updates, and ensure that all systems are synchronized with the latest information. By following the steps outlined in this guide, you can create and update products seamlessly within the Younium platform.
Best Practices for Secure and Efficient Younium API Usage
- Secure Storage of Credentials: Always store your Client ID, Secret Key, and JWT tokens securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of the API's rate limits to avoid throttling. Implement retry logic with exponential backoff for handling rate limit responses gracefully.
- Data Standardization: Ensure that product data is standardized before making API calls. This helps maintain consistency across systems and reduces the likelihood of errors.
- Regular Token Refreshing: Since JWT tokens are valid for 24 hours, implement a mechanism to refresh tokens automatically to maintain uninterrupted API access.
Streamlining Integrations with Endgrate
While integrating with Younium's API can significantly enhance your product management capabilities, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies integration processes across various platforms, including Younium.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development. With a single API endpoint, you can manage multiple integrations effortlessly, providing an intuitive experience for your customers.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website and discover the benefits of a streamlined integration approach.
Read More
Ready to get started?