Using the Sugar Market API to Get Tasks (with Python examples)
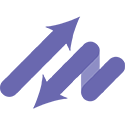
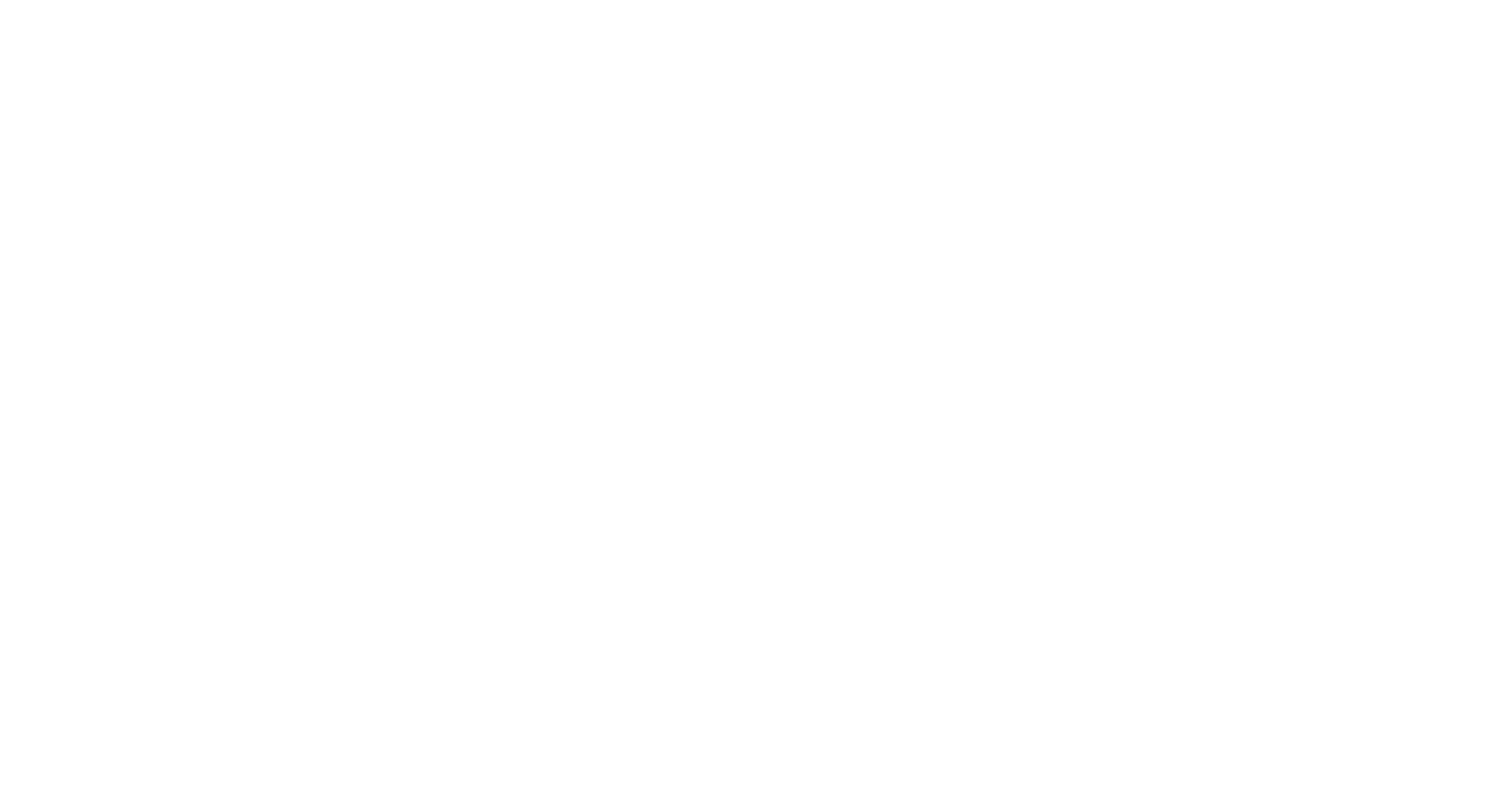
Introduction to Sugar Market API
Sugar Market is a robust marketing automation platform designed to help businesses streamline their marketing efforts and drive growth. It offers a comprehensive suite of tools for email marketing, lead nurturing, and campaign management, making it a popular choice for businesses looking to enhance their marketing strategies.
Integrating with the Sugar Market API allows developers to access and manage various marketing resources programmatically. For example, you might want to retrieve tasks from Sugar Market to analyze campaign performance or automate task management processes. This integration can significantly enhance productivity by reducing manual data handling and enabling seamless data flow between systems.
Setting Up a Sugar Market Test/Sandbox Account for API Integration
Before you can start interacting with the Sugar Market API, you need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data. Follow these steps to get started:
Creating a Sugar Market Account
If you don't already have a Sugar Market account, you'll need to sign up for one. Visit the Sugar Market website and register for a free trial or demo account. This will give you access to the necessary tools and resources to begin your integration.
Generating API Credentials for Sugar Market
Once your account is set up, you'll need to generate API credentials to authenticate your requests. Sugar Market uses a custom authentication method, so follow these steps to obtain your credentials:
- Log in to your Sugar Market account.
- Navigate to the API settings section, typically found under the integrations or developer tools menu.
- Create a new API application. You will be prompted to provide details such as the application name and description.
- After creating the application, you will receive a client ID and client secret. Make sure to store these securely, as they are required for authenticating your API requests.
Configuring OAuth for Sugar Market API Access
Although Sugar Market uses a custom authentication method, it may involve OAuth-like steps for token generation. Here’s a general approach:
- Use your client ID and client secret to request an access token from the Sugar Market authorization server.
- Include the access token in the header of your API requests to authenticate them.
Refer to the Sugar Market API documentation for specific details on the authentication process.
Testing Your Sugar Market API Setup
With your API credentials in hand, you can now test your setup by making a simple API call. This will ensure that your authentication is correctly configured and that you can interact with the Sugar Market API:
import requests
# Set the API endpoint and headers
endpoint = "https://api.sugarmarket.com/v1/tasks"
headers = {
"Authorization": "Bearer Your_Access_Token",
"Content-Type": "application/json"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
print("API setup successful!")
else:
print("Error:", response.status_code)
Replace Your_Access_Token
with the token you obtained earlier. If the request is successful, you should see a confirmation message.
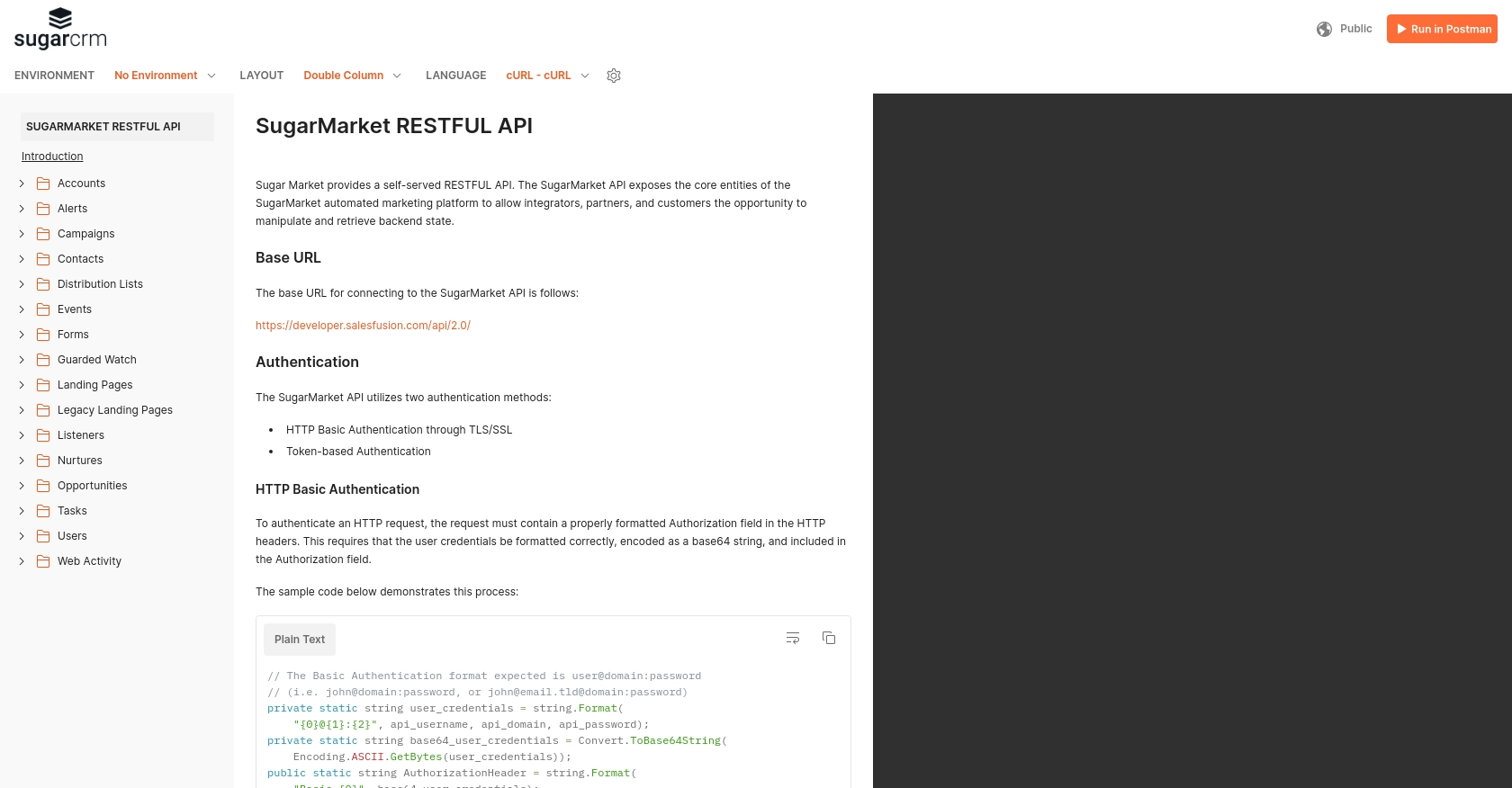
sbb-itb-96038d7
Making API Calls to Retrieve Tasks from Sugar Market Using Python
To interact with the Sugar Market API and retrieve tasks, you'll need to use Python, a versatile programming language known for its simplicity and readability. This section will guide you through the process of making API calls to Sugar Market, ensuring you have the right setup and understanding to execute these calls effectively.
Setting Up Your Python Environment for Sugar Market API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests. Install it using the following command:
pip install requests
With Python and the necessary library installed, you're ready to start coding.
Executing a GET Request to Retrieve Tasks from Sugar Market
To retrieve tasks from Sugar Market, you'll make a GET request to the appropriate API endpoint. Here's a step-by-step guide with example code:
import requests
# Define the API endpoint and headers
endpoint = "https://api.sugarmarket.com/v1/tasks"
headers = {
"Authorization": "Bearer Your_Access_Token",
"Content-Type": "application/json"
}
# Make the GET request to the Sugar Market API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
tasks = response.json()
for task in tasks:
print(task)
else:
print("Error:", response.status_code)
Replace Your_Access_Token
with the access token you obtained during the authentication setup. This script sends a GET request to the Sugar Market API to fetch tasks and prints them if the request is successful.
Verifying API Call Success and Handling Errors
After executing the API call, it's crucial to verify the success of the request. A status code of 200 indicates success, and you should see the tasks printed in your console. If the request fails, the script will print the error code, allowing you to troubleshoot the issue.
Refer to the Sugar Market API documentation for detailed information on error codes and their meanings.
Best Practices for Error Handling and Debugging
- Always check the status code of your API response to ensure successful requests.
- Implement error handling to manage different HTTP error codes gracefully.
- Log errors for further analysis and debugging.
By following these practices, you can efficiently manage API interactions and ensure robust integration with Sugar Market.
Conclusion: Best Practices for Using the Sugar Market API
Integrating with the Sugar Market API can significantly enhance your marketing automation processes by allowing seamless access to tasks and other resources. To ensure a smooth integration experience, consider the following best practices:
Securely Storing Sugar Market API Credentials
- Always store your client ID, client secret, and access tokens securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Regularly rotate your API credentials to maintain security.
Handling Sugar Market API Rate Limiting
Be mindful of any rate limits imposed by the Sugar Market API to avoid disruptions in service. Implement logic to handle rate limit responses gracefully, such as retrying requests after a specified delay.
Standardizing and Transforming Data from Sugar Market API
- Ensure that the data retrieved from the Sugar Market API is standardized and transformed to fit your application's data model.
- Implement data validation checks to maintain data integrity and consistency.
Enhancing Your Integration Strategy with Endgrate
Consider leveraging Endgrate to streamline your integration processes. By using Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product development. Endgrate provides a unified API endpoint that simplifies interactions with multiple platforms, including Sugar Market, offering an intuitive integration experience for your customers.
Visit Endgrate to learn more about how you can optimize your integration strategy and enhance your product's capabilities.
Read More
Ready to get started?