Using the Microsoft Dynamics 365 API to Get Accounts in Javascript
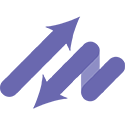
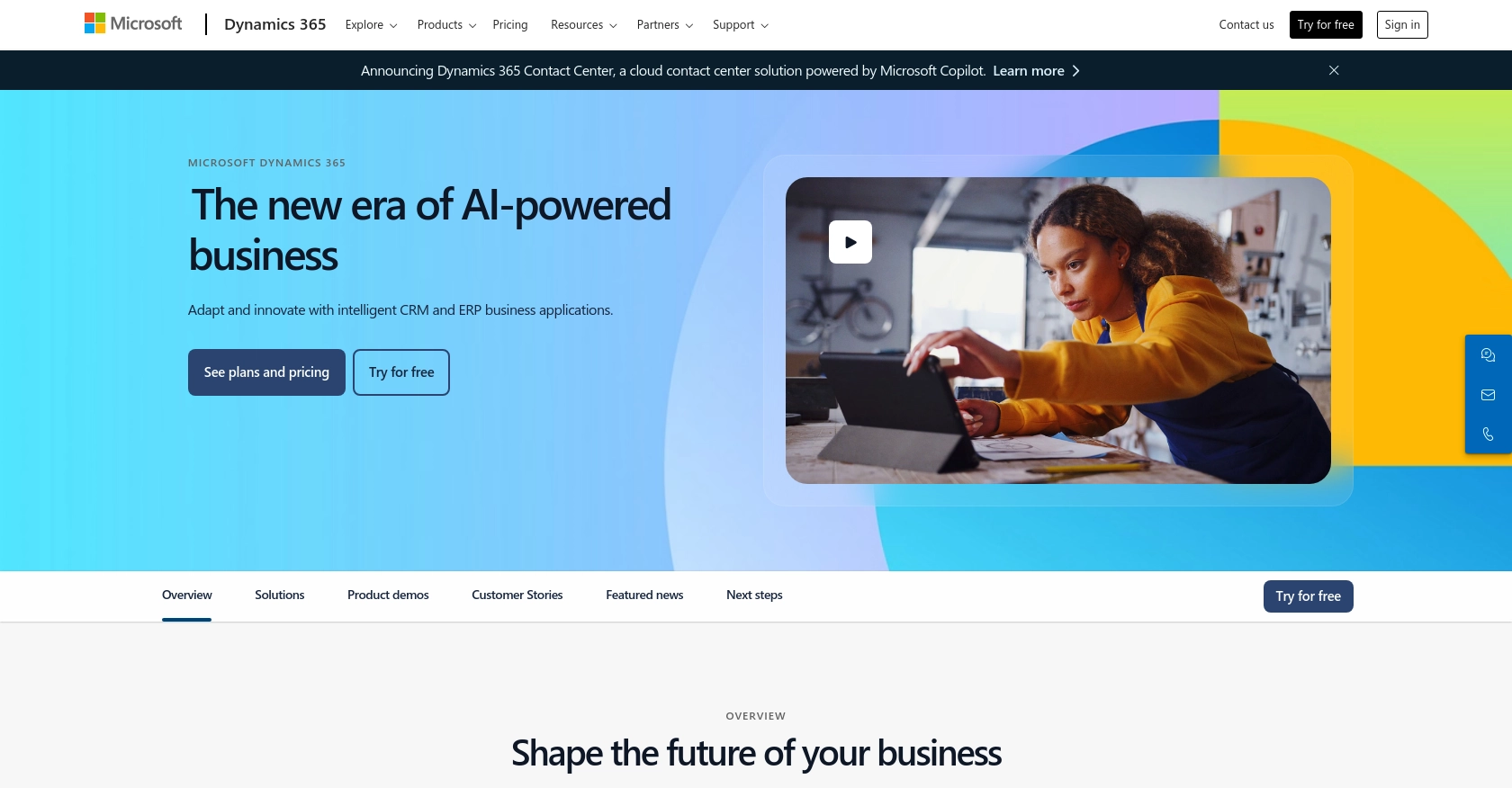
Introduction to Microsoft Dynamics 365 API
Microsoft Dynamics 365 is a powerful suite of business applications that combines CRM and ERP capabilities to streamline business processes and enhance customer engagement. It offers a comprehensive platform for managing sales, customer service, finance, operations, and more.
Developers often integrate with the Microsoft Dynamics 365 API to access and manipulate business data programmatically. For example, retrieving account information using the API can help automate reporting and analytics, enabling businesses to make data-driven decisions efficiently.
In this article, we will explore how to use JavaScript to interact with the Microsoft Dynamics 365 API to fetch account details, providing a practical guide for developers looking to leverage this integration in their applications.
Setting Up a Microsoft Dynamics 365 Test/Sandbox Account
Before you can begin integrating with the Microsoft Dynamics 365 API, it's essential to set up a test or sandbox account. This environment allows developers to safely test API interactions without affecting live data. Microsoft Dynamics 365 offers a trial version that can be used for this purpose.
Step-by-Step Guide to Creating a Microsoft Dynamics 365 Trial Account
- Visit the Microsoft Dynamics 365 Website: Go to the Microsoft Dynamics 365 website and navigate to the free trial section.
- Sign Up for a Free Trial: Click on the "Try for free" button and fill out the necessary information, including your email address and company details.
- Verify Your Email: After signing up, you will receive a verification email. Follow the instructions to verify your account.
- Access the Dynamics 365 Dashboard: Once verified, log in to your Dynamics 365 account to access the dashboard and explore the available features.
Registering an Application for OAuth Authentication
To interact with the Microsoft Dynamics 365 API, you need to register an application in your Microsoft Entra ID tenant. This process involves obtaining the necessary credentials for OAuth authentication.
- Navigate to Azure Portal: Go to the Azure Portal and sign in with your Microsoft account.
- Register a New Application: In the Azure Portal, select "Azure Active Directory" and then "App registrations." Click on "New registration" to create a new application.
-
Fill in Application Details: Provide a name for your application and set the redirect URI. For development purposes, you can use
https://localhost
. - Configure API Permissions: After registration, navigate to "API permissions" and add the necessary permissions for Dynamics 365. Ensure you include the "Access Dynamics 365 as organization users" permission.
- Generate Client Secret: Under "Certificates & secrets," create a new client secret. Note down the secret value as it will be used for authentication.
For more detailed instructions on app registration and OAuth authentication, refer to the official documentation: Use OAuth authentication with Microsoft Dataverse.
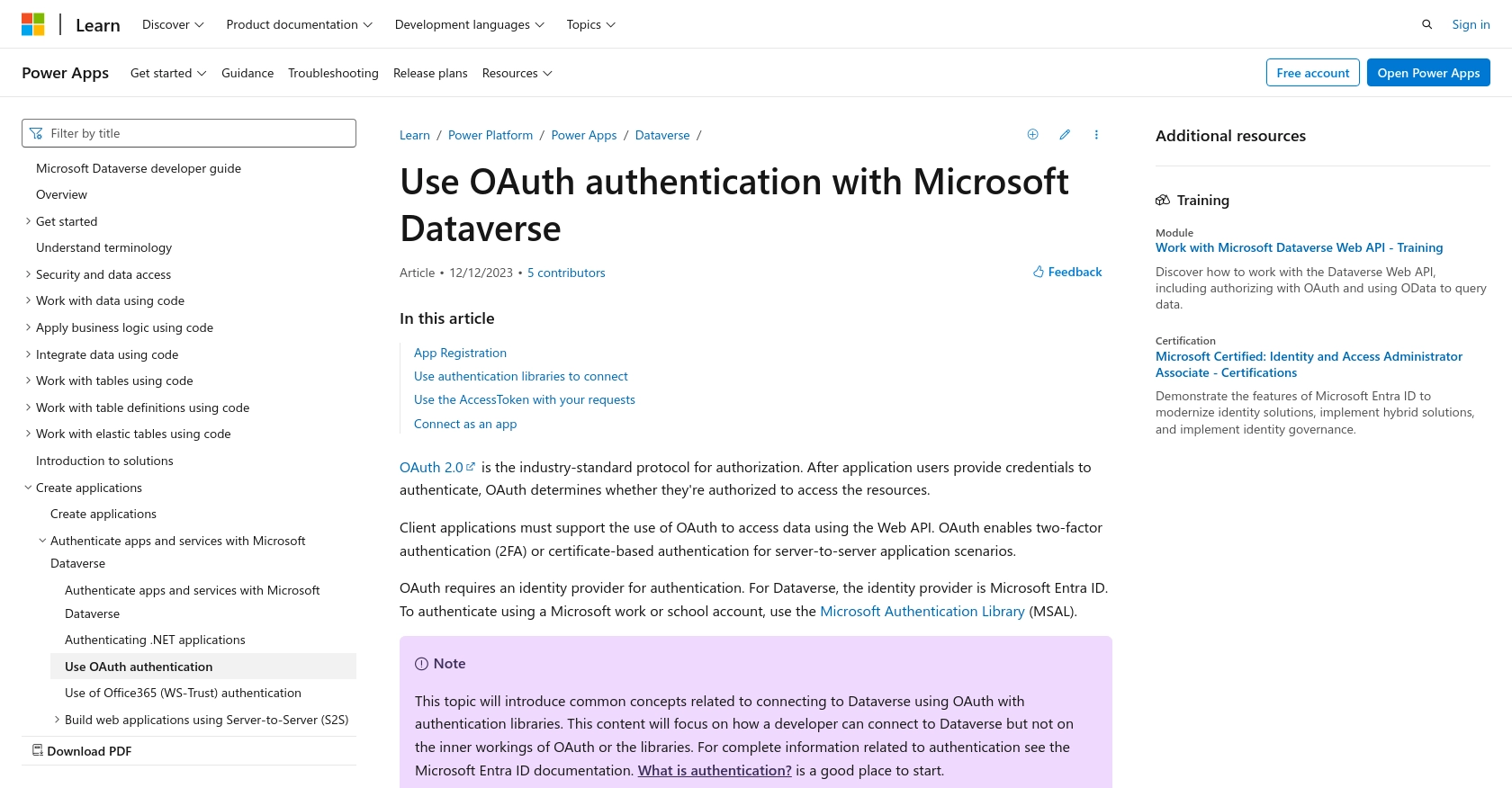
sbb-itb-96038d7
Making API Calls to Microsoft Dynamics 365 Using JavaScript
To interact with Microsoft Dynamics 365 API using JavaScript, you need to set up your development environment and make HTTP requests to the API endpoints. This section will guide you through the process of fetching account details using JavaScript.
Setting Up Your JavaScript Environment
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine.
- A code editor like Visual Studio Code.
- Access to the Microsoft Dynamics 365 sandbox account with OAuth credentials.
Installing Required Dependencies
To make HTTP requests in JavaScript, you can use the popular axios
library. Install it using npm:
npm install axios
Fetching Account Details from Microsoft Dynamics 365
Now, let's write a JavaScript script to fetch account details using the Microsoft Dynamics 365 API.
const axios = require('axios');
// Set up the API endpoint and headers
const endpoint = 'https://yourorg.api.crm.dynamics.com/api/data/v9.2/accounts';
const token = 'Your_Access_Token'; // Replace with your OAuth access token
// Configure headers
const headers = {
'Authorization': `Bearer ${token}`,
'OData-MaxVersion': '4.0',
'OData-Version': '4.0',
'Accept': 'application/json'
};
// Make a GET request to the API
axios.get(endpoint, { headers })
.then(response => {
// Handle successful response
console.log('Accounts:', response.data.value);
})
.catch(error => {
// Handle errors
console.error('Error fetching accounts:', error.response ? error.response.data : error.message);
});
Replace Your_Access_Token
with the token obtained during the OAuth authentication process. This script sets up the API endpoint and headers, makes a GET request to retrieve account details, and handles the response.
Verifying API Call Success
After running the script, you should see the account details logged in the console. Verify the data against your Microsoft Dynamics 365 sandbox account to ensure accuracy.
Handling Errors and Common Error Codes
When making API calls, it's crucial to handle potential errors. Common HTTP status codes you might encounter include:
- 401 Unauthorized: Check if your access token is valid and not expired.
- 403 Forbidden: Ensure your application has the necessary permissions.
- 404 Not Found: Verify the endpoint URL and resource identifiers.
For more detailed error handling, refer to the official documentation: Get accounts - Business Central.
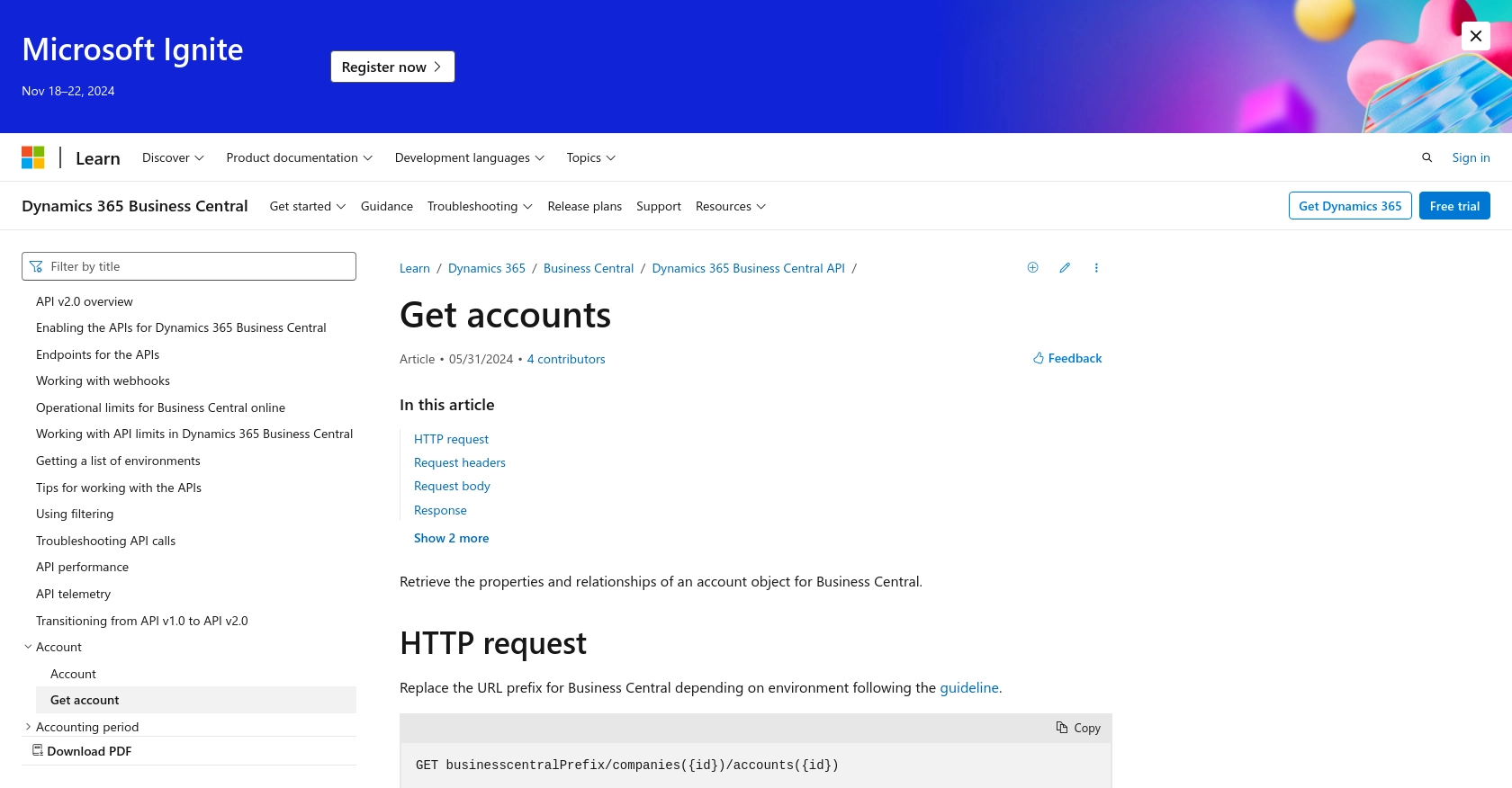
Conclusion and Best Practices for Using Microsoft Dynamics 365 API with JavaScript
Integrating with the Microsoft Dynamics 365 API using JavaScript provides developers with a powerful way to access and manage business data programmatically. By following the steps outlined in this guide, you can efficiently retrieve account details and incorporate them into your applications to enhance functionality and automate processes.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store OAuth credentials securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limiting: Be aware of any rate limits imposed by the API. Implement retry logic with exponential backoff to handle rate limit responses gracefully.
- Standardize Data Fields: Transform and standardize data fields to ensure consistency across different systems and applications.
- Monitor API Usage: Regularly monitor API usage and performance to identify any potential issues or optimizations.
Leverage Endgrate for Simplified Integration Management
While integrating with Microsoft Dynamics 365 API can be rewarding, managing multiple integrations can become complex and time-consuming. Endgrate offers a streamlined solution by providing a unified API endpoint that connects to various platforms, including Microsoft Dynamics 365.
By using Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product development. With Endgrate's intuitive integration experience, you can build once for each use case and easily manage multiple integrations, enhancing your product's capabilities and user experience.
Explore how Endgrate can simplify your integration needs by visiting Endgrate and discover the benefits of a unified API solution.
Read More
- https://endgrate.com/provider/microsoftdynamics365
- https://learn.microsoft.com/en-us/power-apps/developer/data-platform/authenticate-oauth
- https://learn.microsoft.com/en-us/graph/use-the-api
- https://learn.microsoft.com/en-us/dynamics365/business-central/dev-itpro/api-reference/v2.0/api/dynamics_account_get
Ready to get started?