Using the Salesflare API to Create or Update Contacts in PHP
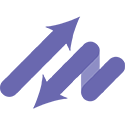
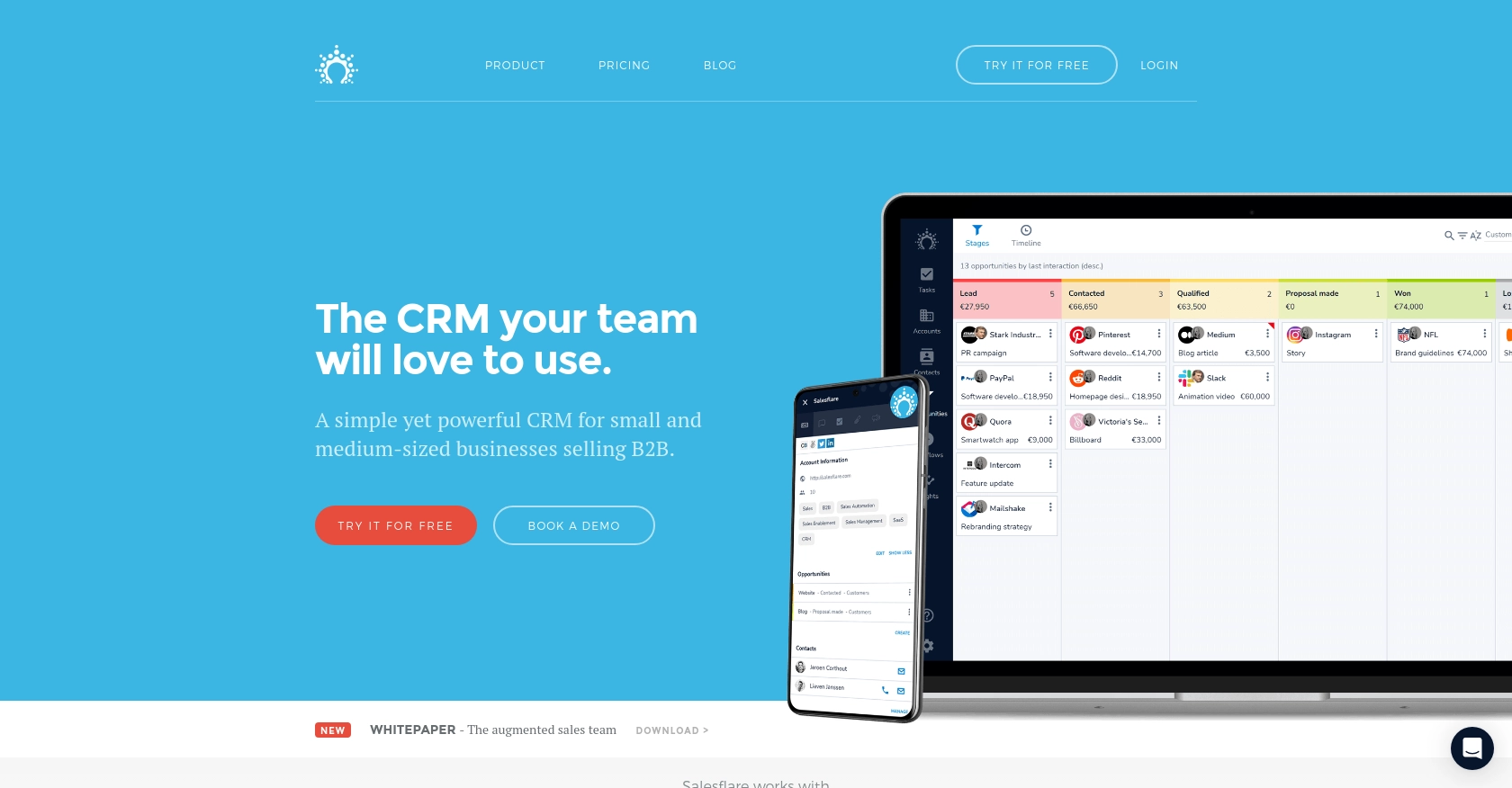
Introduction to Salesflare API
Salesflare is a powerful CRM platform designed to simplify the sales process for small and medium-sized businesses. With its intuitive interface and automation capabilities, Salesflare helps sales teams manage leads, track customer interactions, and close deals more efficiently.
Integrating with the Salesflare API allows developers to automate and enhance CRM functionalities, such as creating or updating contact information. For example, you can streamline your workflow by automatically syncing contact data from external sources into Salesflare, ensuring your sales team always has the most up-to-date information.
Setting Up Your Salesflare Test Account for API Integration
Before diving into the integration process with Salesflare's API, it's essential to set up a test account. This will allow you to safely experiment with API calls without affecting live data. Salesflare offers a straightforward way to create a test account, enabling developers to explore its CRM functionalities.
Creating a Salesflare Test Account
To begin, you'll need to sign up for a Salesflare account. Follow these steps to get started:
- Visit the Salesflare website and click on the "Sign Up" button.
- Fill in the required information, such as your name, email address, and password.
- Once registered, log in to your Salesflare account to access the dashboard.
Generating Your Salesflare API Key
Salesflare uses API key-based authentication to authorize requests. Follow these steps to generate your API key:
- Navigate to the "Settings" section in your Salesflare dashboard.
- Look for the "API & Integrations" tab and click on it.
- Click on "Generate API Key" to create a new key for your application.
- Copy the generated API key and store it securely, as you'll need it to authenticate your API requests.
For more detailed information, refer to the Salesflare API documentation.
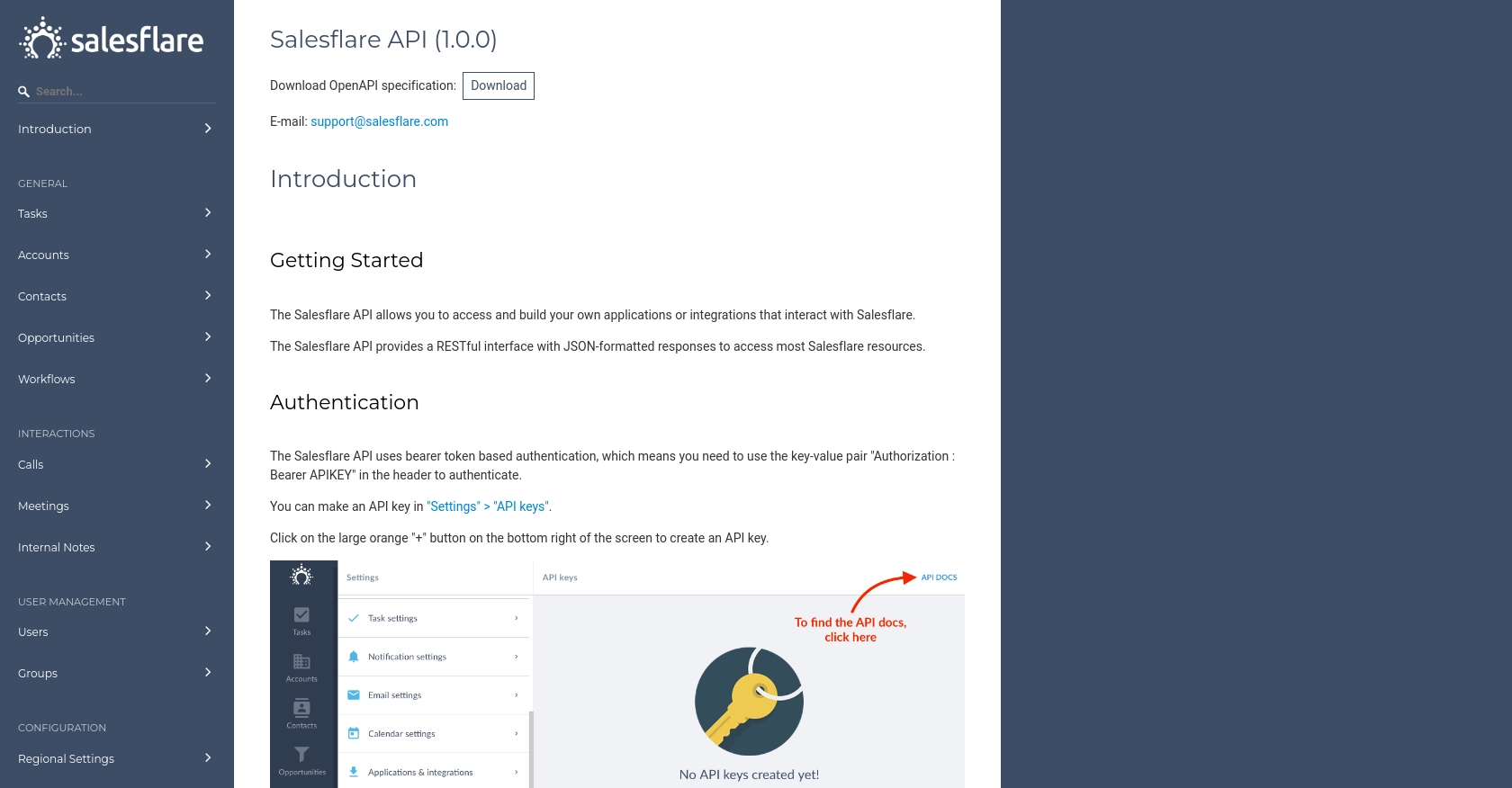
sbb-itb-96038d7
Making API Calls with Salesflare in PHP
To interact with the Salesflare API and manage contacts, you'll need to make HTTP requests using PHP. This section will guide you through setting up your environment, writing the necessary code, and handling responses effectively.
Setting Up Your PHP Environment for Salesflare API Integration
Before making API calls, ensure your PHP environment is ready. You'll need:
- PHP 7.4 or later
- Composer for dependency management
- The
guzzlehttp/guzzle
library for making HTTP requests
Install the Guzzle library using Composer with the following command:
composer require guzzlehttp/guzzle
Creating or Updating Contacts with Salesflare API
Now, let's write a PHP script to create or update contacts in Salesflare. This example demonstrates how to send a POST request to the Salesflare API.
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$apiKey = 'Your_API_Key'; // Replace with your Salesflare API key
// Define the contact data
$contactData = [
'email' => 'example@salesflare.com',
'first_name' => 'John',
'last_name' => 'Doe'
];
try {
$response = $client->request('POST', 'https://api.salesflare.com/v1/contacts', [
'headers' => [
'Authorization' => 'Bearer ' . $apiKey,
'Content-Type' => 'application/json'
],
'json' => $contactData
]);
if ($response->getStatusCode() === 200) {
echo "Contact created or updated successfully.";
} else {
echo "Failed to create or update contact.";
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Replace Your_API_Key
with the API key you generated earlier. This script uses Guzzle to send a POST request to the Salesflare API endpoint for contacts. It includes the necessary headers for authentication and the contact data in JSON format.
Verifying API Call Success in Salesflare
After running the script, verify the success of your API call by checking the Salesflare dashboard. The contact should appear with the details you provided. If the contact already exists, the API will update the existing record.
Handling Errors and Troubleshooting
When making API calls, it's crucial to handle potential errors. The Salesflare API may return various HTTP status codes indicating success or failure. Common error codes include:
- 400 Bad Request: The request was invalid or cannot be served.
- 401 Unauthorized: Authentication failed due to invalid API key.
- 404 Not Found: The requested resource could not be found.
- 500 Internal Server Error: An error occurred on the server.
For more detailed information, refer to the Salesflare API documentation.
Conclusion and Best Practices for Salesflare API Integration
Integrating with the Salesflare API using PHP allows developers to efficiently manage contact data, enhancing CRM capabilities and streamlining sales processes. By automating the creation and updating of contacts, your sales team can focus more on closing deals and less on manual data entry.
Best Practices for Secure and Efficient Salesflare API Usage
- Secure API Key Storage: Always store your API keys securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the Salesflare API to avoid service disruptions. Implement exponential backoff strategies to handle rate limit errors gracefully.
- Data Standardization: Ensure consistent data formats when syncing contact information to avoid discrepancies and maintain data integrity across platforms.
Enhancing Integration Capabilities with Endgrate
While integrating with Salesflare is a powerful step, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Salesflare. By leveraging Endgrate, you can:
- Save time and resources by outsourcing integration management, allowing your team to focus on core product development.
- Build once for each use case, reducing the need for multiple integration efforts across different platforms.
- Offer an intuitive and seamless integration experience for your customers, enhancing user satisfaction and engagement.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?