Using the Microsoft Dynamics 365 API to Create or Update Account in Python
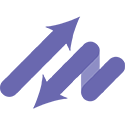
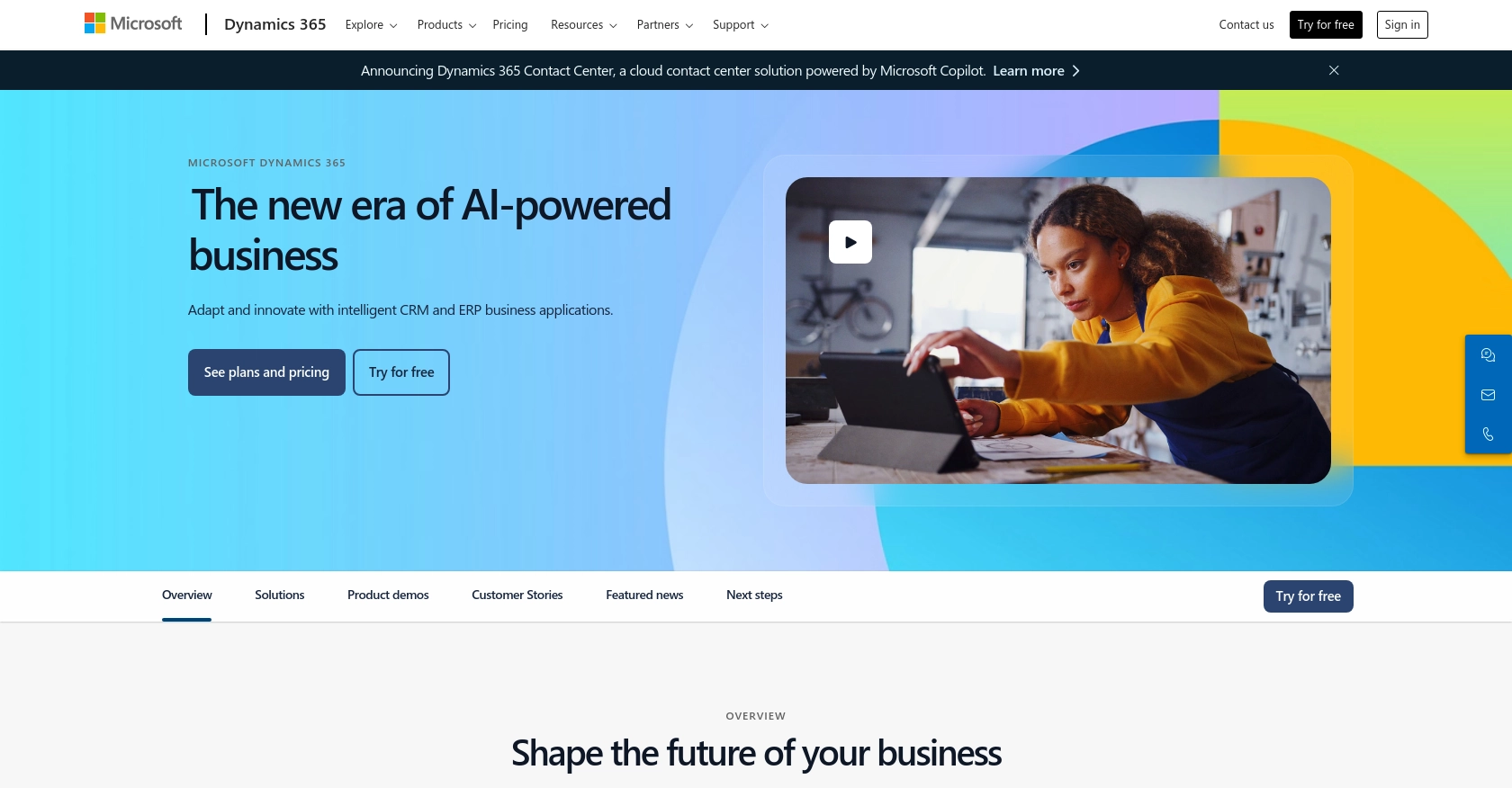
Introduction to Microsoft Dynamics 365 API
Microsoft Dynamics 365 is a powerful suite of business applications that combines CRM and ERP capabilities to streamline business processes and improve customer engagement. It offers a comprehensive platform for managing sales, customer service, finance, operations, and more, making it a popular choice for businesses looking to enhance their operational efficiency.
Integrating with the Microsoft Dynamics 365 API allows developers to automate and optimize various business processes. For example, you can use the API to create or update account information directly from your application, ensuring that your customer data is always up-to-date and accessible. This integration can be particularly useful for businesses that need to manage large volumes of customer data efficiently.
In this article, we will explore how to use Python to interact with the Microsoft Dynamics 365 API, focusing on creating or updating account records. This guide will provide step-by-step instructions to help you seamlessly integrate your application with Microsoft Dynamics 365, leveraging its robust API capabilities.
Setting Up a Microsoft Dynamics 365 Test or Sandbox Account
Before you can start integrating with the Microsoft Dynamics 365 API, it's essential to set up a test or sandbox account. This environment allows developers to experiment and test API interactions without affecting live data. Follow these steps to create and configure your sandbox account:
Step 1: Sign Up for a Microsoft Dynamics 365 Free Trial
If you don't already have a Microsoft Dynamics 365 account, you can sign up for a free trial. Visit the Microsoft Dynamics 365 website and follow the instructions to create an account. This trial will provide you with access to the necessary tools and features to test API integrations.
Step 2: Access the Microsoft Power Platform Admin Center
Once your account is set up, navigate to the Microsoft Power Platform Admin Center. Here, you can manage your environments, including creating a sandbox environment for testing purposes.
Step 3: Create a Sandbox Environment
- In the Admin Center, select Environments from the left-hand menu.
- Click on New to create a new environment.
- Choose Sandbox as the type of environment.
- Fill in the required details, such as the name and region, and click Create.
This sandbox environment will serve as your testing ground for API interactions.
Step 4: Register an Application with Microsoft Entra ID
To authenticate API requests, you need to register an application with Microsoft Entra ID. Follow these steps:
- Go to the Azure Portal and sign in with your Microsoft account.
- Navigate to Azure Active Directory > App registrations.
- Click on New registration and fill in the application details.
- Set the Redirect URI to
https://localhost
for local development. - Click Register to create the application.
Step 5: Configure API Permissions
After registering your application, configure the necessary API permissions:
- In the application registration, go to API permissions.
- Click Add a permission and select Dynamics CRM.
- Choose the permissions required for your application, such as user_impersonation.
- Click Add permissions to save your changes.
Step 6: Generate Client Secret
To authenticate your application, generate a client secret:
- In the application registration, navigate to Certificates & secrets.
- Click on New client secret.
- Provide a description and set an expiration period.
- Click Add and copy the generated secret value. Store it securely as it will be used in your API requests.
With these steps completed, your Microsoft Dynamics 365 sandbox environment and application registration are ready for API integration testing. You can now proceed to authenticate and interact with the API using Python.
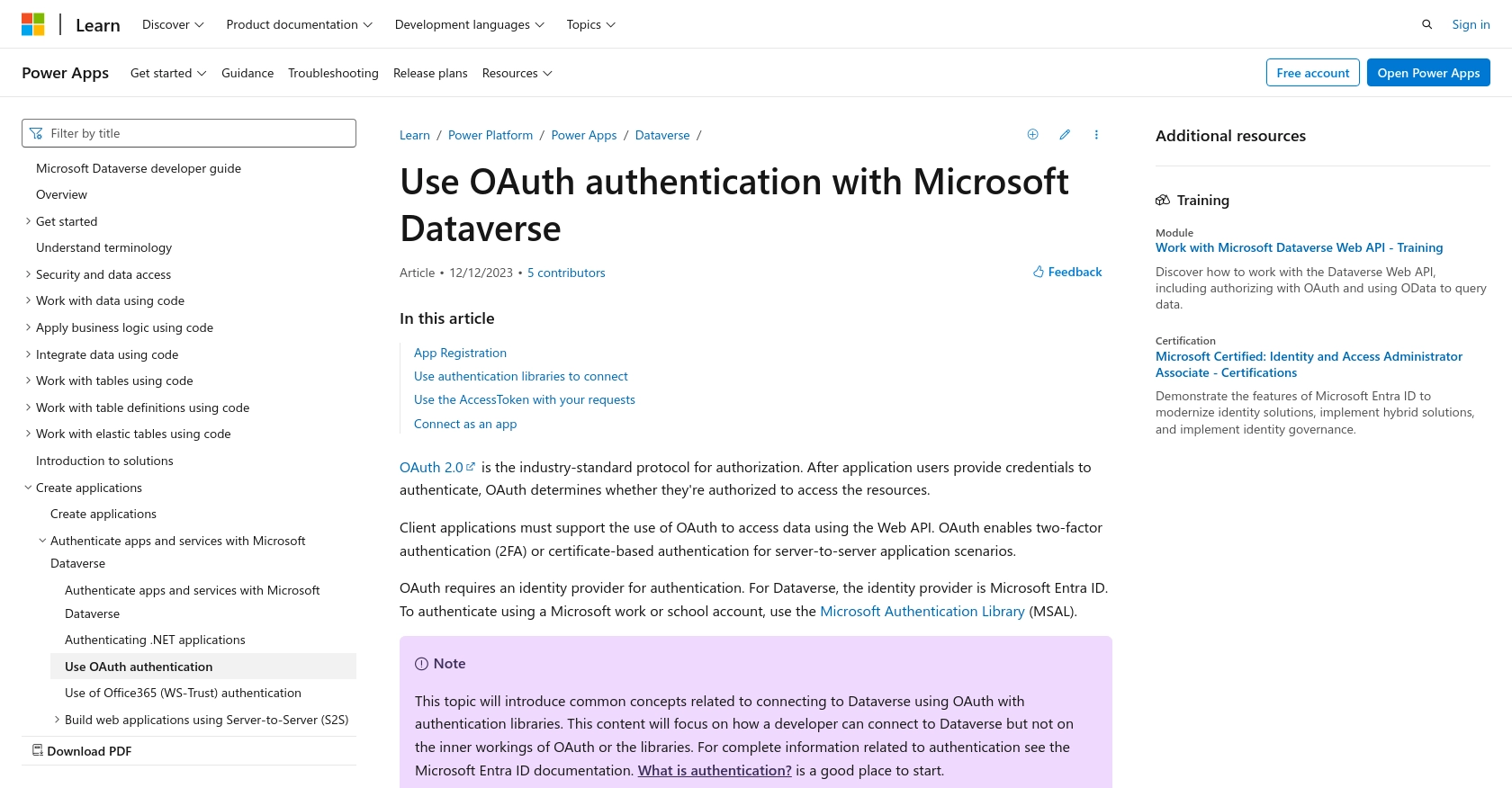
sbb-itb-96038d7
How to Make an API Call to Microsoft Dynamics 365 Using Python
To interact with the Microsoft Dynamics 365 API using Python, you'll need to set up your environment and write the necessary code to create or update account records. This section will guide you through the process, including setting up Python, installing dependencies, and executing API calls.
Setting Up Your Python Environment for Microsoft Dynamics 365 API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1 or later
- The Python package installer, pip
Once you have these installed, open your terminal or command prompt and install the required libraries:
pip install requests msal
Creating or Updating an Account in Microsoft Dynamics 365 Using Python
With your environment set up, you can now write the Python code to interact with the Microsoft Dynamics 365 API. Below is a sample script to create or update an account:
import requests
from msal import ConfidentialClientApplication
# Define your credentials and endpoints
client_id = 'Your_Client_ID'
client_secret = 'Your_Client_Secret'
tenant_id = 'Your_Tenant_ID'
resource = 'https://yourorg.crm.dynamics.com'
authority = f'https://login.microsoftonline.com/{tenant_id}'
endpoint = f'{resource}/api/data/v9.2/accounts'
# Create a confidential client application
app = ConfidentialClientApplication(
client_id,
authority=authority,
client_credential=client_secret
)
# Acquire a token
token_response = app.acquire_token_for_client(scopes=[f'{resource}/.default'])
access_token = token_response.get('access_token')
# Define headers
headers = {
'Authorization': f'Bearer {access_token}',
'Content-Type': 'application/json',
'OData-MaxVersion': '4.0',
'OData-Version': '4.0',
'Accept': 'application/json'
}
# Define the account data
account_data = {
'name': 'New Account Name',
'telephone1': '123-456-7890',
'emailaddress1': 'example@domain.com'
}
# Make the API request
response = requests.post(endpoint, headers=headers, json=account_data)
# Check the response
if response.status_code == 204:
print('Account created or updated successfully.')
else:
print(f'Failed to create or update account: {response.status_code} - {response.text}')
Verifying the API Call Success in Microsoft Dynamics 365
After running the script, verify the success of your API call by checking the Microsoft Dynamics 365 sandbox environment. If the account was created or updated successfully, you should see the changes reflected in the account records.
Handling Errors and Common Error Codes in Microsoft Dynamics 365 API
When interacting with the API, you may encounter errors. Here are some common error codes and their meanings:
- 400 Bad Request: The request was malformed or contains invalid data.
- 401 Unauthorized: Authentication failed. Check your credentials and token.
- 403 Forbidden: You do not have permission to access the resource.
- 404 Not Found: The specified resource could not be found.
- 500 Internal Server Error: An error occurred on the server. Try again later.
For more detailed error handling, refer to the Microsoft Dynamics 365 API documentation.
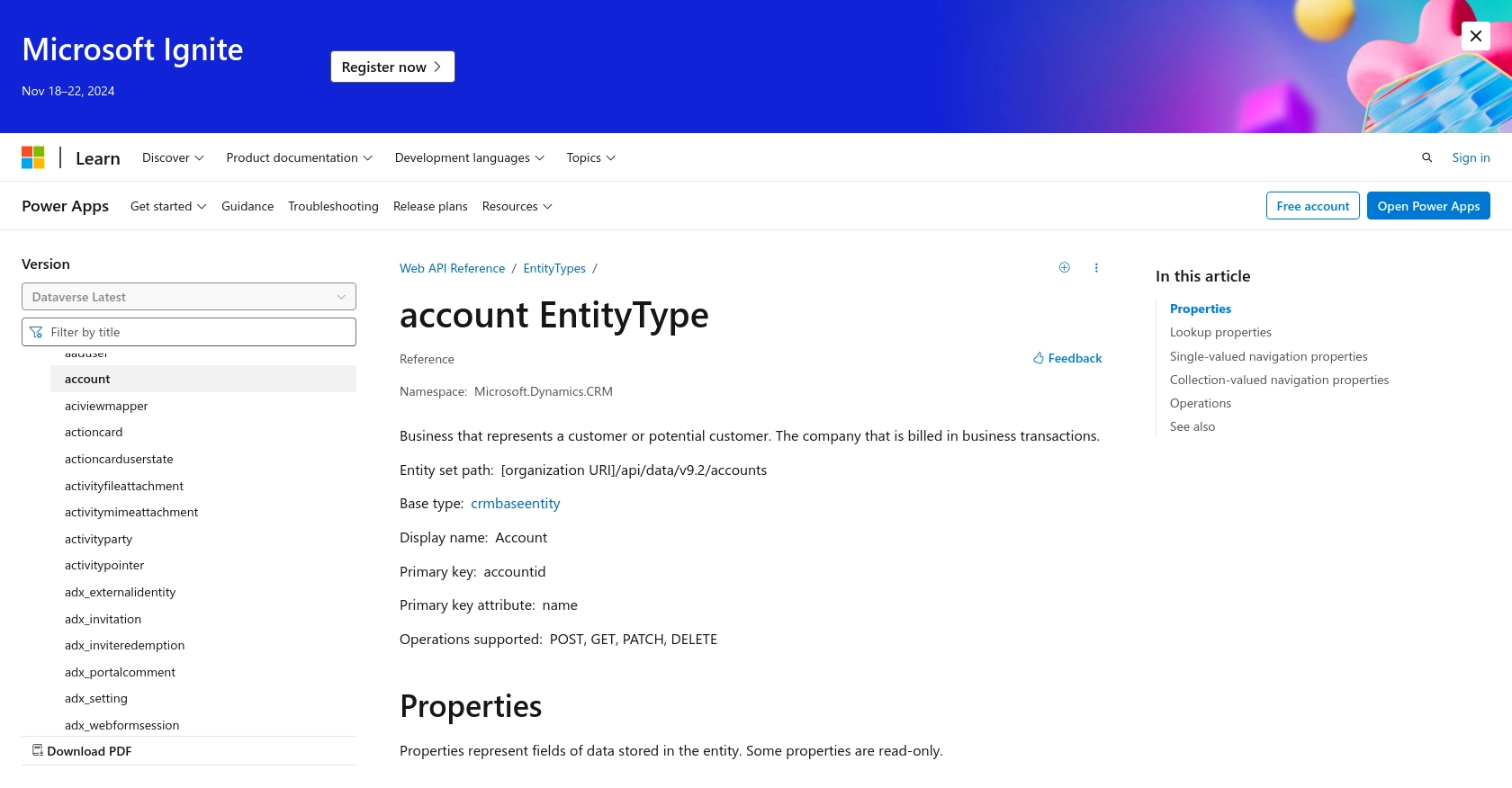
Best Practices for Microsoft Dynamics 365 API Integration
Successfully integrating with the Microsoft Dynamics 365 API requires adherence to best practices to ensure security, efficiency, and maintainability. Here are some recommendations:
- Securely Store Credentials: Always store client secrets and access tokens securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Be aware of API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit responses gracefully.
- Data Standardization: Ensure data consistency by standardizing fields and formats across your application and Microsoft Dynamics 365.
- Monitor API Usage: Regularly monitor API usage and performance to identify potential issues and optimize your integration.
Enhance Your Integration Strategy with Endgrate
Integrating with multiple platforms can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Microsoft Dynamics 365. By leveraging Endgrate, you can:
- Save time and resources by outsourcing integration tasks and focusing on your core product development.
- Build once for each use case, eliminating the need to develop separate integrations for different platforms.
- Offer an intuitive integration experience for your customers, enhancing their satisfaction and engagement.
Explore how Endgrate can streamline your integration efforts and help you achieve seamless connectivity across platforms. Visit Endgrate to learn more and get started today.
Read More
- https://endgrate.com/provider/microsoftdynamics365
- https://learn.microsoft.com/en-us/power-apps/developer/data-platform/authenticate-oauth
- https://learn.microsoft.com/en-us/graph/use-the-api
- https://learn.microsoft.com/en-us/power-apps/developer/data-platform/webapi/reference/account?view=dataverse-latest
Ready to get started?