How to Get Accounts with the Zoho Books API in PHP
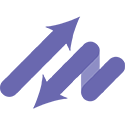
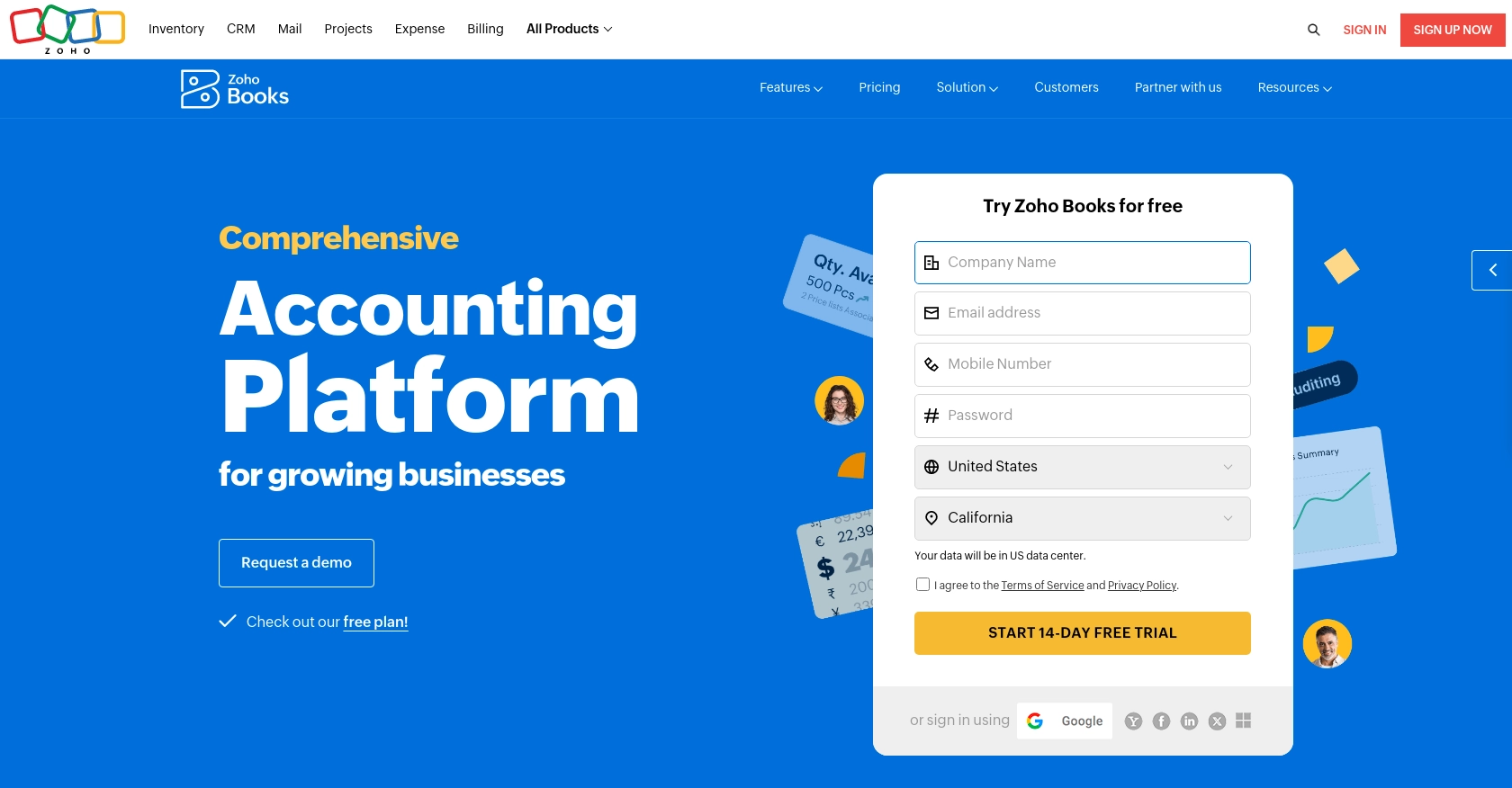
Introduction to Zoho Books API Integration
Zoho Books is a comprehensive accounting software solution designed to simplify financial management for businesses of all sizes. It offers a wide range of features, including invoicing, expense tracking, and financial reporting, making it a popular choice for businesses looking to streamline their accounting processes.
Developers may want to integrate with the Zoho Books API to automate financial tasks and access real-time financial data. For example, using the Zoho Books API, a developer can retrieve account details to generate custom financial reports or synchronize data with other business applications.
This article will guide you through the process of using PHP to interact with the Zoho Books API, specifically focusing on retrieving account information. By following this tutorial, you'll learn how to efficiently access and manage account data within the Zoho Books platform using PHP.
Setting Up Your Zoho Books Test Account for API Integration
Before you begin integrating with the Zoho Books API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data. Zoho Books offers a free trial that you can use to access their API and test your integration.
Creating a Zoho Books Free Trial Account
- Visit the Zoho Books sign-up page.
- Fill in the required details, such as your email address and password, and click on "Sign Up."
- Follow the instructions in the confirmation email to verify your account.
- Once verified, log in to your Zoho Books account to access the dashboard.
Registering a New Client in Zoho Developer Console for OAuth Authentication
Zoho Books uses OAuth 2.0 for authentication. To interact with the API, you need to register your application in the Zoho Developer Console to obtain your Client ID and Client Secret.
- Go to the Zoho Developer Console.
- Click on "Add Client ID" to register your application.
- Provide the necessary details, such as the client name, homepage URL, and authorized redirect URIs.
- After successful registration, note down the Client ID and Client Secret provided. Keep these credentials secure.
Generating OAuth Tokens for Zoho Books API Access
With your Client ID and Client Secret, you can now generate the OAuth tokens needed to authenticate API requests.
- Redirect to the following authorization URL with the required parameters:
- Upon user consent, Zoho will redirect to your specified redirect URI with a code parameter.
- Exchange this code for an access token and refresh token by making a POST request to:
- Store the access token and refresh token securely. The access token is used in API requests, while the refresh token is used to obtain new access tokens when the current one expires.
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoBooks.fullaccess.all&client_id=YOUR_CLIENT_ID&response_type=code&redirect_uri=YOUR_REDIRECT_URI&access_type=offline
https://accounts.zoho.com/oauth/v2/token?code=YOUR_CODE&client_id=YOUR_CLIENT_ID&client_secret=YOUR_CLIENT_SECRET&redirect_uri=YOUR_REDIRECT_URI&grant_type=authorization_code
With your test account and OAuth tokens set up, you're ready to start making API calls to Zoho Books. In the next section, we'll explore how to retrieve account information using PHP.
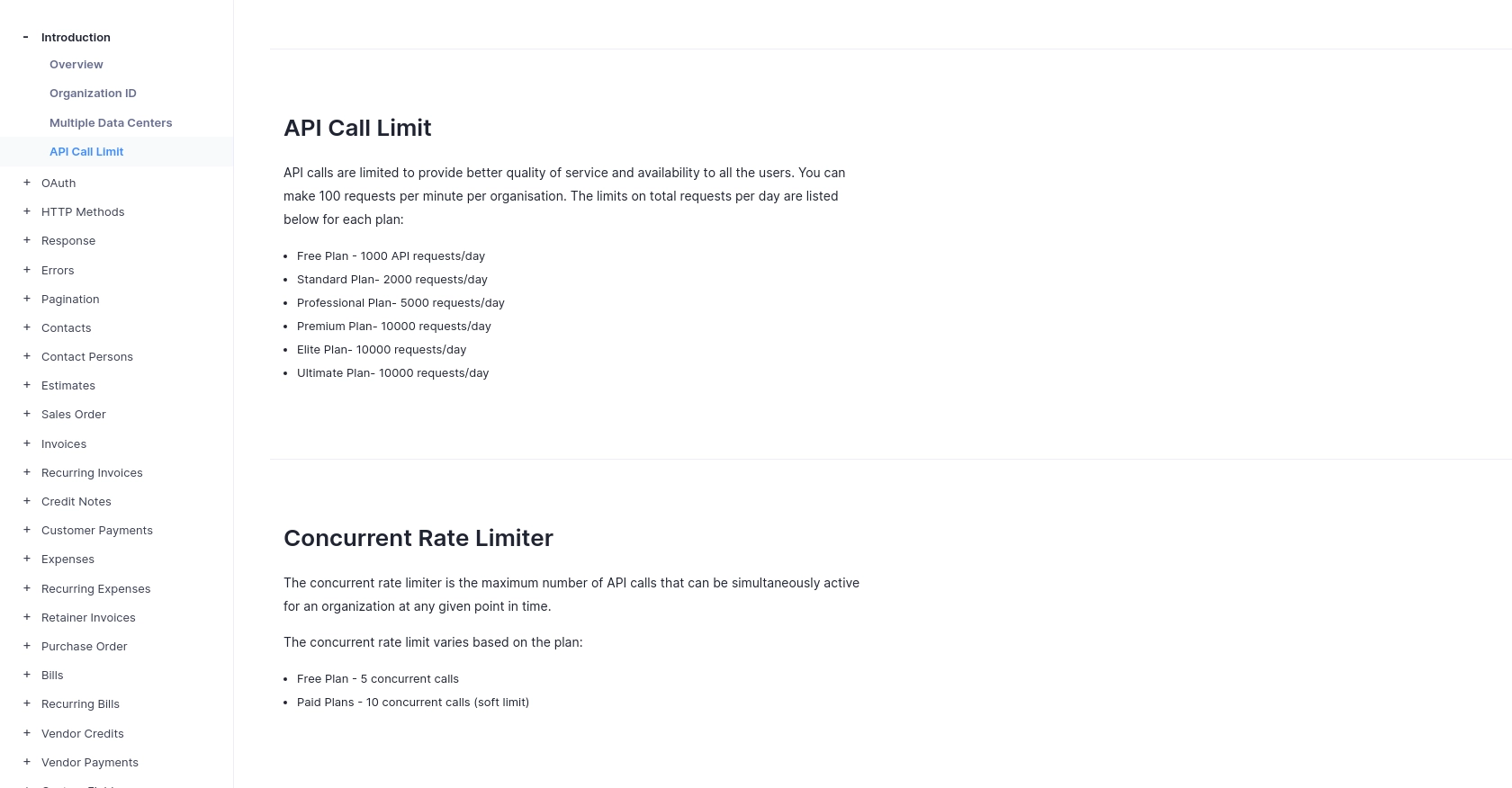
sbb-itb-96038d7
Making API Calls to Retrieve Account Information from Zoho Books Using PHP
With your Zoho Books test account and OAuth tokens ready, you can now proceed to make API calls to retrieve account information. This section will guide you through setting up your PHP environment and executing the necessary API requests.
Setting Up Your PHP Environment for Zoho Books API Integration
Before making API calls, ensure your PHP environment is correctly configured. You'll need PHP 7.4 or later and the cURL extension enabled to handle HTTP requests.
- Verify your PHP version by running the following command in your terminal:
- Ensure the cURL extension is enabled in your
php.ini
file. Look for the lineextension=curl
and remove any semicolon (;) at the beginning. - Restart your web server to apply the changes.
php -v
Installing Required PHP Dependencies for Zoho Books API
To simplify HTTP requests, you can use the Guzzle HTTP client. Install it using Composer:
composer require guzzlehttp/guzzle
Executing the API Call to Retrieve Accounts from Zoho Books
With your environment set up, you can now write a PHP script to retrieve account information from Zoho Books.
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'YOUR_ACCESS_TOKEN';
$organizationId = 'YOUR_ORGANIZATION_ID';
$response = $client->request('GET', 'https://www.zohoapis.com/books/v3/chartofaccounts', [
'headers' => [
'Authorization' => 'Zoho-oauthtoken ' . $accessToken,
],
'query' => [
'organization_id' => $organizationId,
],
]);
$data = json_decode($response->getBody(), true);
foreach ($data['chartofaccounts'] as $account) {
echo 'Account Name: ' . $account['account_name'] . '<br>';
echo 'Account Type: ' . $account['account_type'] . '<br>';
echo 'Currency Code: ' . $account['currency_code'] . '<br><br>';
}
Replace YOUR_ACCESS_TOKEN
and YOUR_ORGANIZATION_ID
with your actual access token and organization ID.
Verifying the API Request and Handling Errors
After running the script, you should see a list of accounts printed on your screen. If the request fails, check the HTTP status code and error message returned by the API. Common error codes include:
- 400 Bad Request: The request was invalid. Check your parameters.
- 401 Unauthorized: The access token is invalid or expired.
- 404 Not Found: The requested resource could not be found.
- 429 Rate Limit Exceeded: You have exceeded the API rate limit.
For more details on error codes, refer to the Zoho Books API error documentation.
By following these steps, you can successfully retrieve account information from Zoho Books using PHP. In the next section, we'll discuss best practices for managing API integrations.
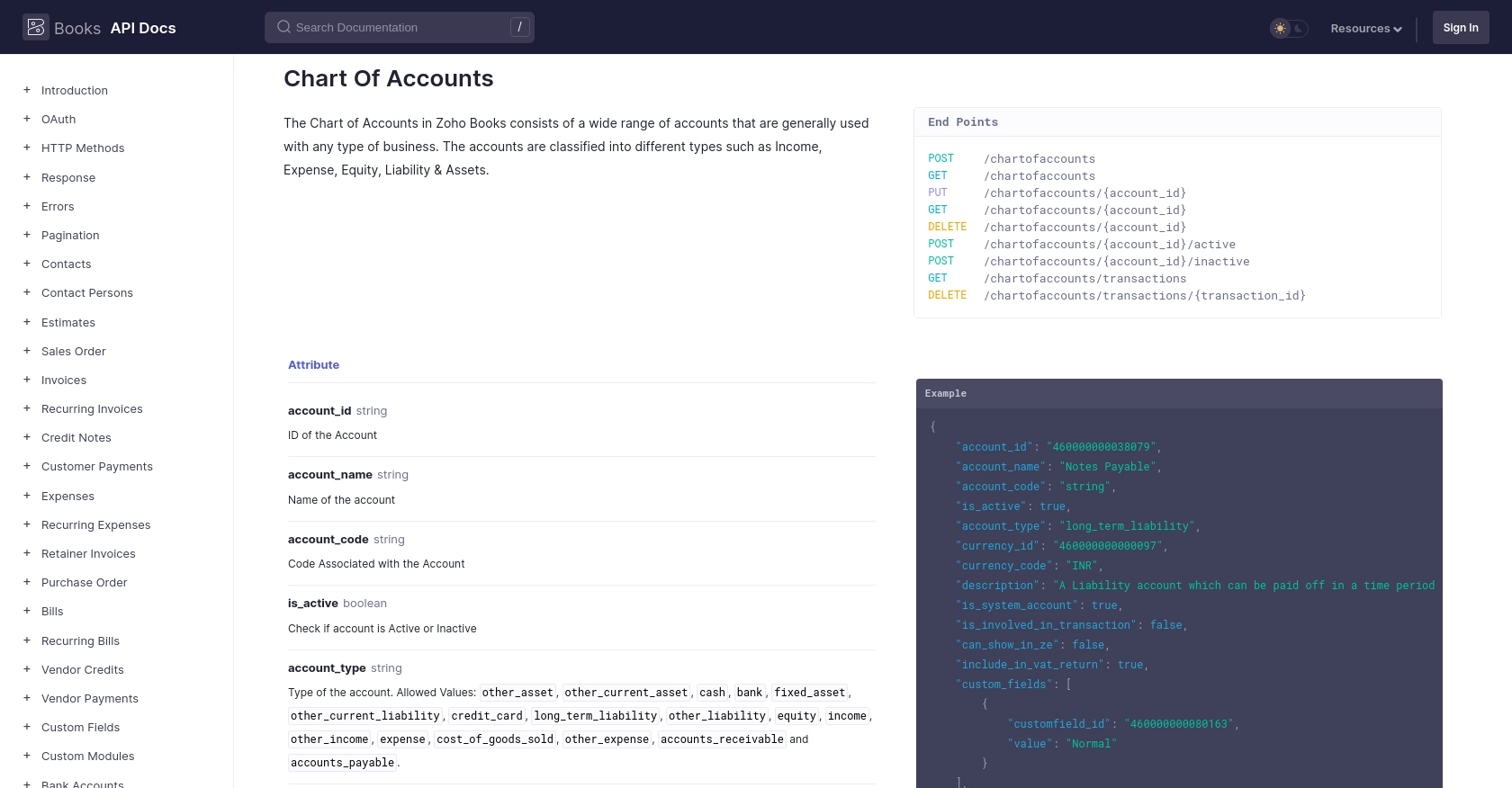
Conclusion: Best Practices for Zoho Books API Integration Using PHP
Integrating with the Zoho Books API using PHP allows developers to automate financial processes and access real-time data efficiently. By following the steps outlined in this guide, you can successfully retrieve account information and enhance your business applications.
Best Practices for Secure and Efficient Zoho Books API Integration
- Securely Store Credentials: Always store your Client ID, Client Secret, access tokens, and refresh tokens securely. Avoid hardcoding them in your scripts.
- Handle Rate Limiting: Zoho Books imposes a rate limit of 100 requests per minute per organization. Implement error handling for HTTP status code 429 to manage rate limits effectively. For more details, refer to the API call limit documentation.
- Refresh Tokens Regularly: Access tokens expire after a specific period. Use the refresh token to obtain new access tokens without user intervention.
- Standardize Data Fields: Ensure that data fields are standardized across your applications to maintain consistency and accuracy.
By adhering to these best practices, you can ensure a robust and secure integration with Zoho Books, allowing your business to leverage its powerful accounting features seamlessly.
Streamline Your Integrations with Endgrate
If managing multiple integrations is becoming a challenge, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers. Visit Endgrate to learn more about how it can simplify your integration needs.
Read More
- https://endgrate.com/provider/zohobooks
- https://www.zoho.com/books/api/v3/introduction/#api-call-limit
- https://www.zoho.com/books/api/v3/oauth/#overview
- https://www.zoho.com/books/api/v3/errors/#overview
- https://www.zoho.com/books/api/v3/pagination/#overview
- https://www.zoho.com/books/api/v3/chart-of-accounts/#overview
Ready to get started?