Using the Stamped API to Create Products in Python
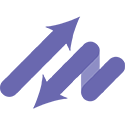
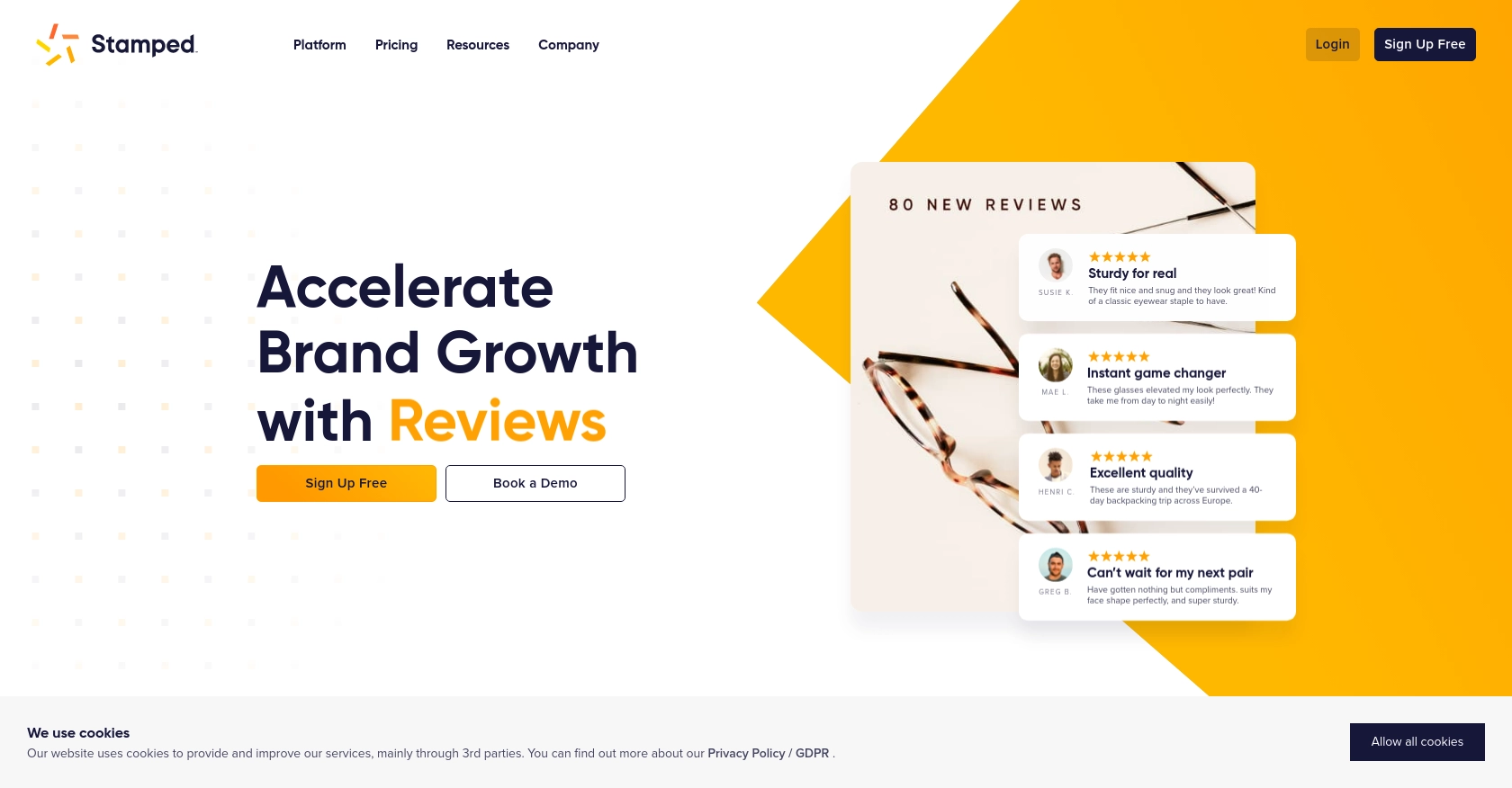
Introduction to Stamped API for Product Creation
Stamped is a powerful eCommerce marketing platform that empowers over 75,000 brands to enhance customer engagement and drive growth through reviews, loyalty programs, and more. With its robust API, developers can seamlessly integrate Stamped's features into their own applications, enabling a more personalized and efficient user experience.
Connecting with the Stamped API allows developers to automate various tasks, such as creating and managing products within their eCommerce platforms. For example, a developer might use the Stamped API to automatically add new products to their store, ensuring that their inventory is always up-to-date and synchronized across multiple platforms.
This article will guide you through the process of using Python to create products via the Stamped API, providing a step-by-step approach to streamline your integration efforts.
Setting Up Your Stamped API Account for Product Creation
Before you can start creating products using the Stamped API, you need to set up your account and obtain the necessary API keys. Stamped requires a Professional or Enterprise plan to access its API features, so ensure your account is appropriately configured.
Creating a Stamped Account
If you don't already have a Stamped account, you can sign up on the Stamped website. Follow the instructions to create your account and select the Professional or Enterprise plan to gain access to the API.
Generating API Keys for Stamped API Access
Once your account is set up, you need to generate API keys to authenticate your requests. Follow these steps to obtain your API keys:
- Log in to your Stamped account and navigate to the Control Panel.
- Go to the API Keys section.
- Here, you will find your public and private API keys. These keys are essential for authenticating your API requests.
Keep these keys secure, as they provide access to your Stamped account's API features.
Understanding Stamped API Authentication
Stamped API uses HTTP Basic Auth for authentication. You will need to include your public API key as the username and your private API key as the password in your requests. This ensures secure communication between your application and the Stamped API.
For more detailed information on authentication, refer to the Stamped REST API documentation.
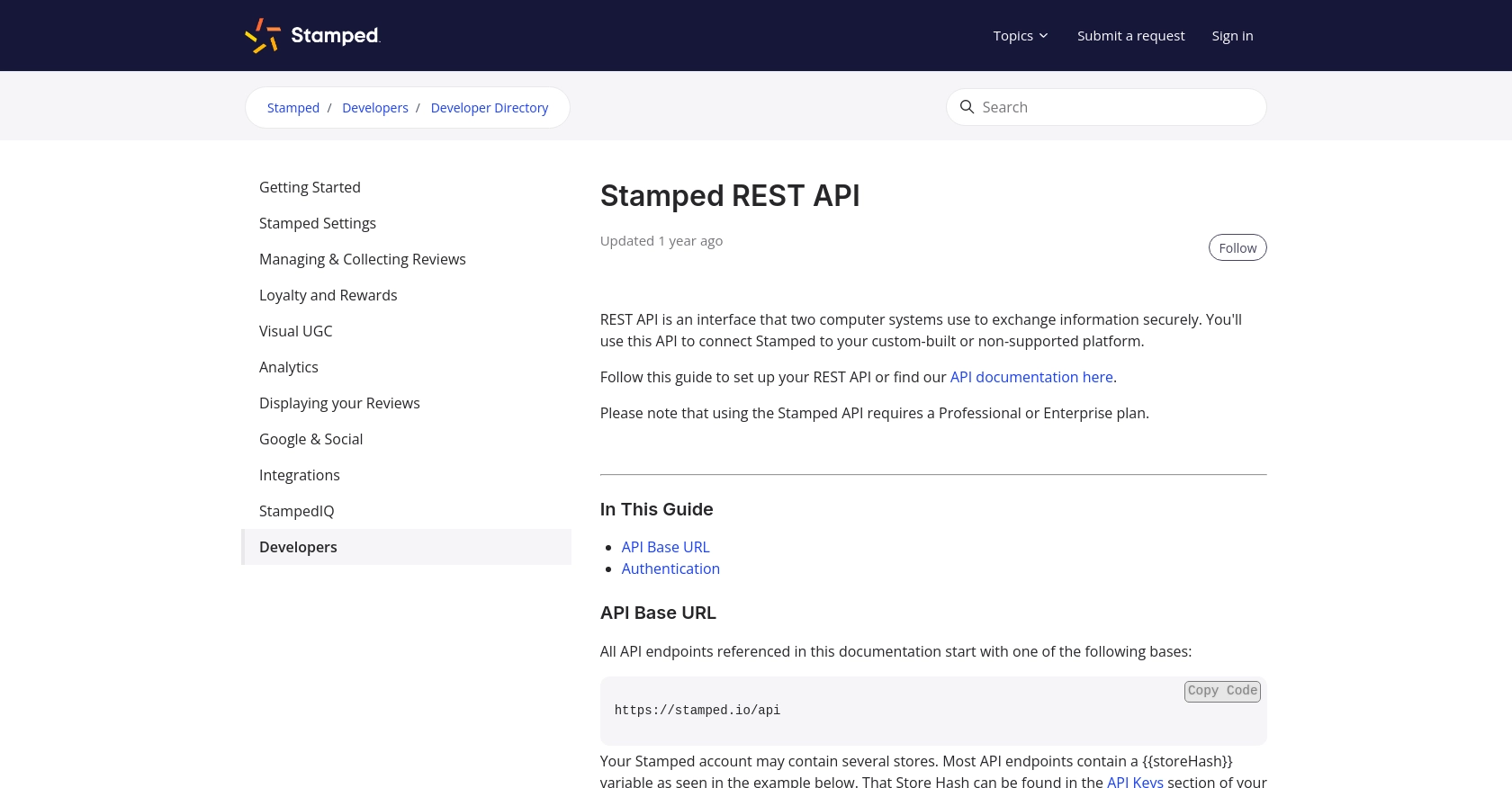
sbb-itb-96038d7
Making API Calls to Create Products with Stamped API in Python
With your Stamped API account set up and API keys in hand, you can now proceed to make API calls to create products. This section will guide you through the process of using Python to interact with the Stamped API, ensuring your eCommerce platform remains synchronized and efficient.
Setting Up Your Python Environment for Stamped API Integration
Before making API calls, ensure your Python environment is ready. You'll need Python 3.x and the requests
library to handle HTTP requests. Install the library using pip:
pip install requests
Creating a Product Using Stamped API with Python
To create a product, you'll need to send a POST request to the Stamped API endpoint. Here's a step-by-step guide:
import requests
# Define the API endpoint and your store hash
store_hash = "your_store_hash"
endpoint = f"https://stamped.io/api/v3/merchant/shops/{store_hash}/products"
# Set your API keys
public_key = "your_public_key"
private_key = "your_private_key"
# Define the product data
product_data = {
"title": "New Product",
"description": "This is a new product description.",
"price": 29.99,
"sku": "NP12345"
}
# Make the POST request
response = requests.post(
endpoint,
json=product_data,
auth=(public_key, private_key)
)
# Check the response
if response.status_code == 201:
print("Product created successfully!")
else:
print(f"Failed to create product: {response.status_code} - {response.text}")
Replace your_store_hash
, your_public_key
, and your_private_key
with your actual Stamped account details. The product_data
dictionary contains the product details you wish to create.
Verifying Product Creation in Stamped Dashboard
After running the script, verify the product creation by checking your Stamped dashboard. If successful, the new product should appear in your product list.
Handling Errors and Troubleshooting Stamped API Requests
When making API calls, it's crucial to handle potential errors. The Stamped API may return various status codes indicating the success or failure of your request:
- 201 Created: The product was successfully created.
- 400 Bad Request: The request was malformed. Check your product data.
- 401 Unauthorized: Authentication failed. Verify your API keys.
- 500 Internal Server Error: An error occurred on the server. Try again later.
For more detailed error handling, refer to the Stamped API documentation.
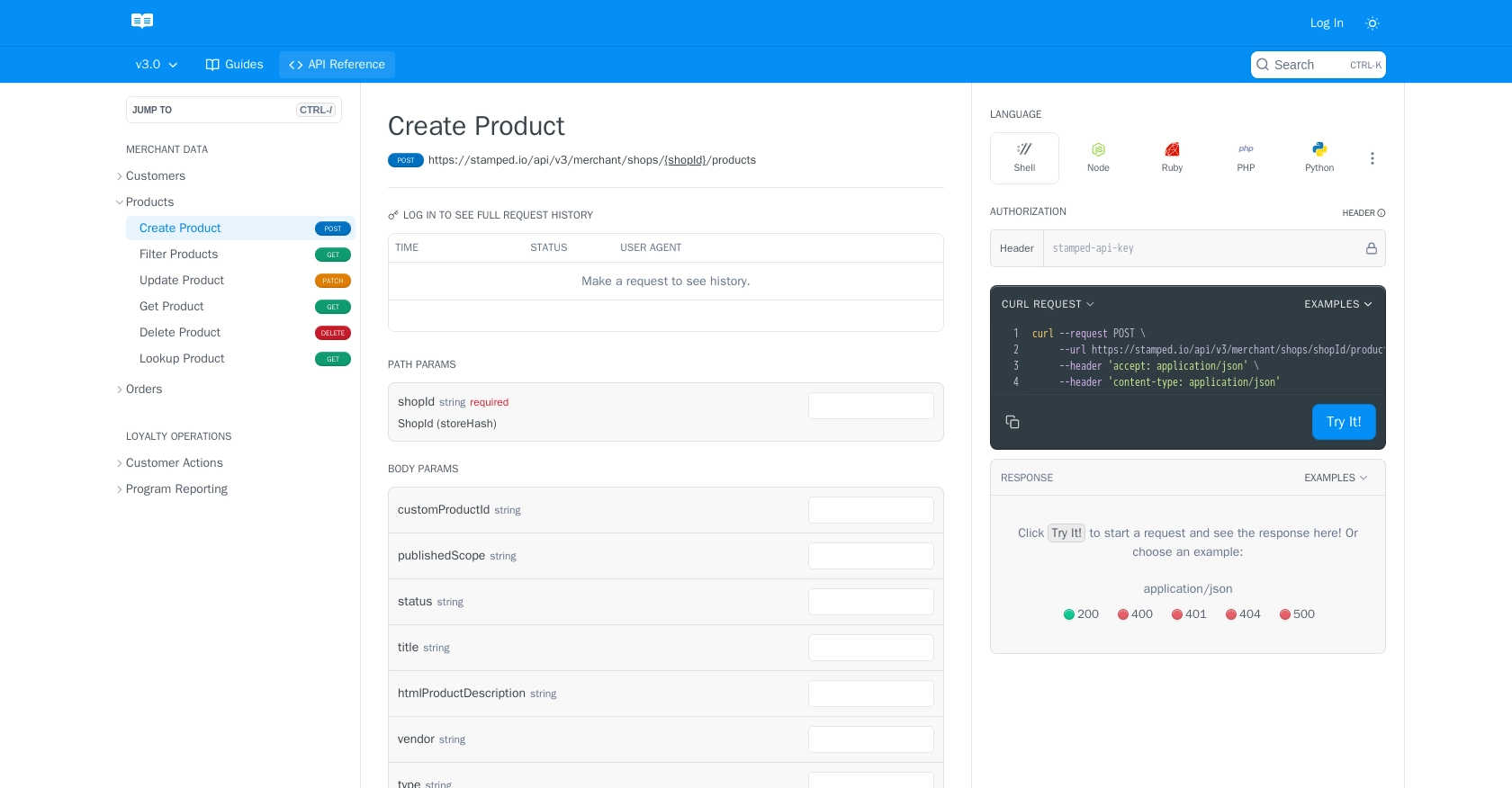
Conclusion and Best Practices for Using Stamped API in Python
Integrating with the Stamped API to create products using Python can significantly enhance your eCommerce platform's efficiency and synchronization. By automating product management, you ensure that your inventory remains up-to-date across various platforms, saving time and reducing manual errors.
Best Practices for Secure and Efficient Stamped API Integration
- Secure API Keys: Always keep your public and private API keys secure. Store them in environment variables or a secure vault to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the Stamped API. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that your product data is standardized before sending it to the API. This includes consistent formatting for fields like price, SKU, and descriptions.
- Error Handling: Implement robust error handling to manage different HTTP status codes. Log errors for further analysis and troubleshooting.
Streamlining Integrations with Endgrate
While integrating with the Stamped API can be straightforward, managing multiple integrations across different platforms can become complex. Endgrate offers a unified API solution that simplifies this process, allowing you to focus on your core product development.
With Endgrate, you can build once for each use case and leverage a single API endpoint to connect with multiple platforms, including Stamped. This not only saves time and resources but also provides an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate and discover the benefits of outsourcing your integration needs.
Read More
Ready to get started?