How to Get Companies with the ZoomInfo API in PHP
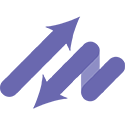
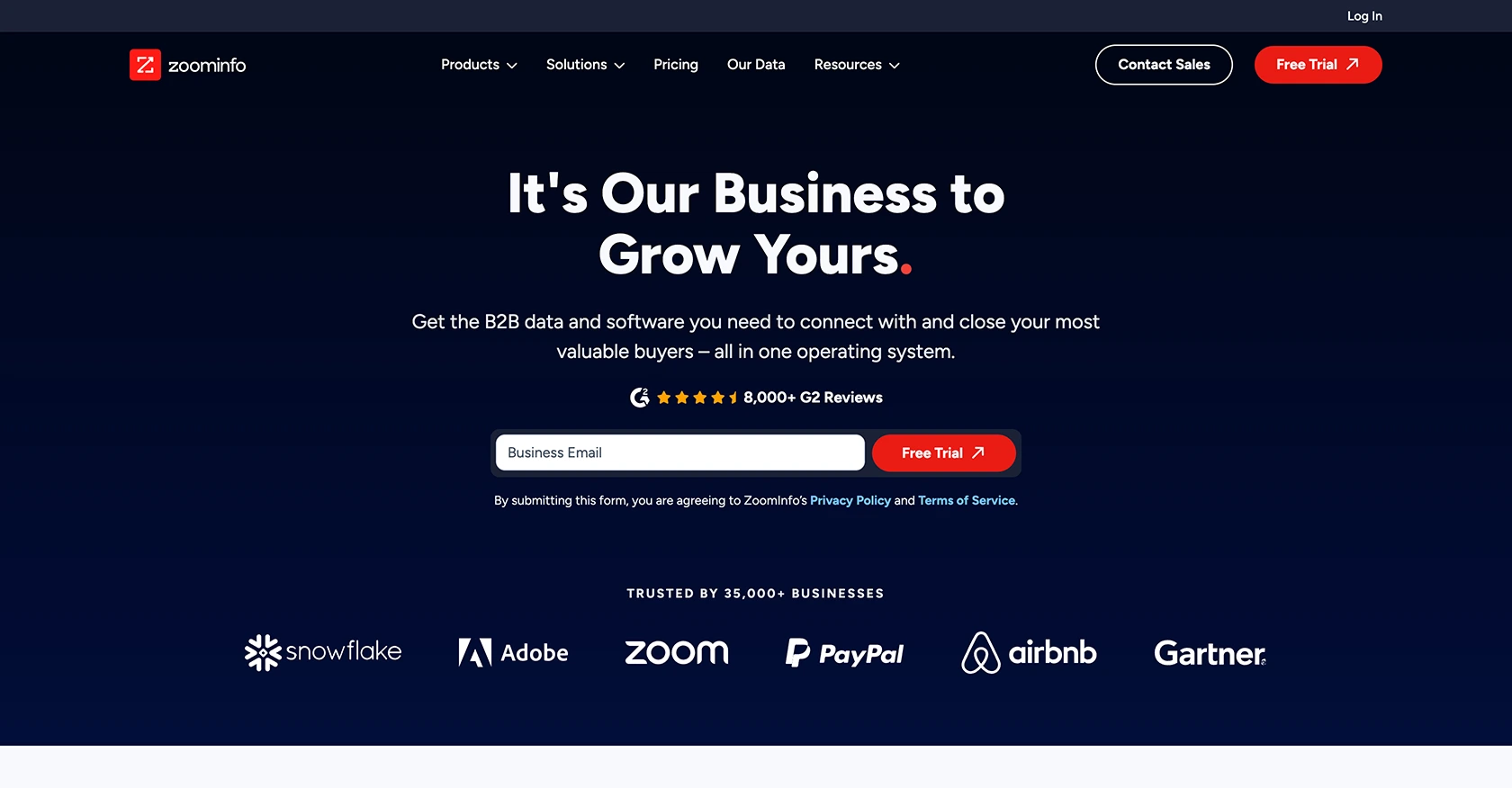
Introduction to ZoomInfo API for Company Data Retrieval
ZoomInfo is a powerful business intelligence platform that provides comprehensive data on companies and professionals. It is widely used by sales and marketing teams to enhance lead generation, market research, and customer relationship management efforts.
For developers, integrating with the ZoomInfo API can unlock a wealth of company data, enabling the creation of applications that leverage detailed business insights. For example, a developer might use the ZoomInfo API to retrieve company information and integrate it into a CRM system, allowing sales teams to access up-to-date company profiles directly within their workflow.
Setting Up Your ZoomInfo Test/Sandbox Account for API Access
Before you can start using the ZoomInfo API to retrieve company data, you need to set up a test or sandbox account. This will allow you to safely experiment with the API without affecting live data. Follow these steps to get started:
Creating a ZoomInfo Developer Account
To access the ZoomInfo API, you first need to create a developer account. Visit the ZoomInfo API documentation page and sign up for a developer account. This will give you access to the necessary credentials and API documentation.
Generating API Credentials for ZoomInfo
Once your developer account is set up, you need to generate API credentials. These credentials are essential for authenticating your API requests. Follow these steps:
- Log in to your ZoomInfo developer account.
- Navigate to the API credentials section.
- Generate a new set of credentials, including a client ID and client secret.
- Store these credentials securely, as you will need them to authenticate your API requests.
Understanding ZoomInfo's Custom Authentication
The ZoomInfo API uses a custom authentication method. To authenticate your requests, you will need to include your client ID and client secret in the request headers. Refer to the ZoomInfo authentication documentation for detailed instructions on how to implement this in your code.
Testing Your ZoomInfo API Setup
After setting up your credentials, it's important to test your configuration to ensure everything is working correctly. You can do this by making a simple API call to retrieve sample data. This will confirm that your authentication is set up properly and that you can interact with the ZoomInfo API.
With your ZoomInfo test account and API credentials ready, you're all set to start integrating ZoomInfo's powerful company data into your applications.
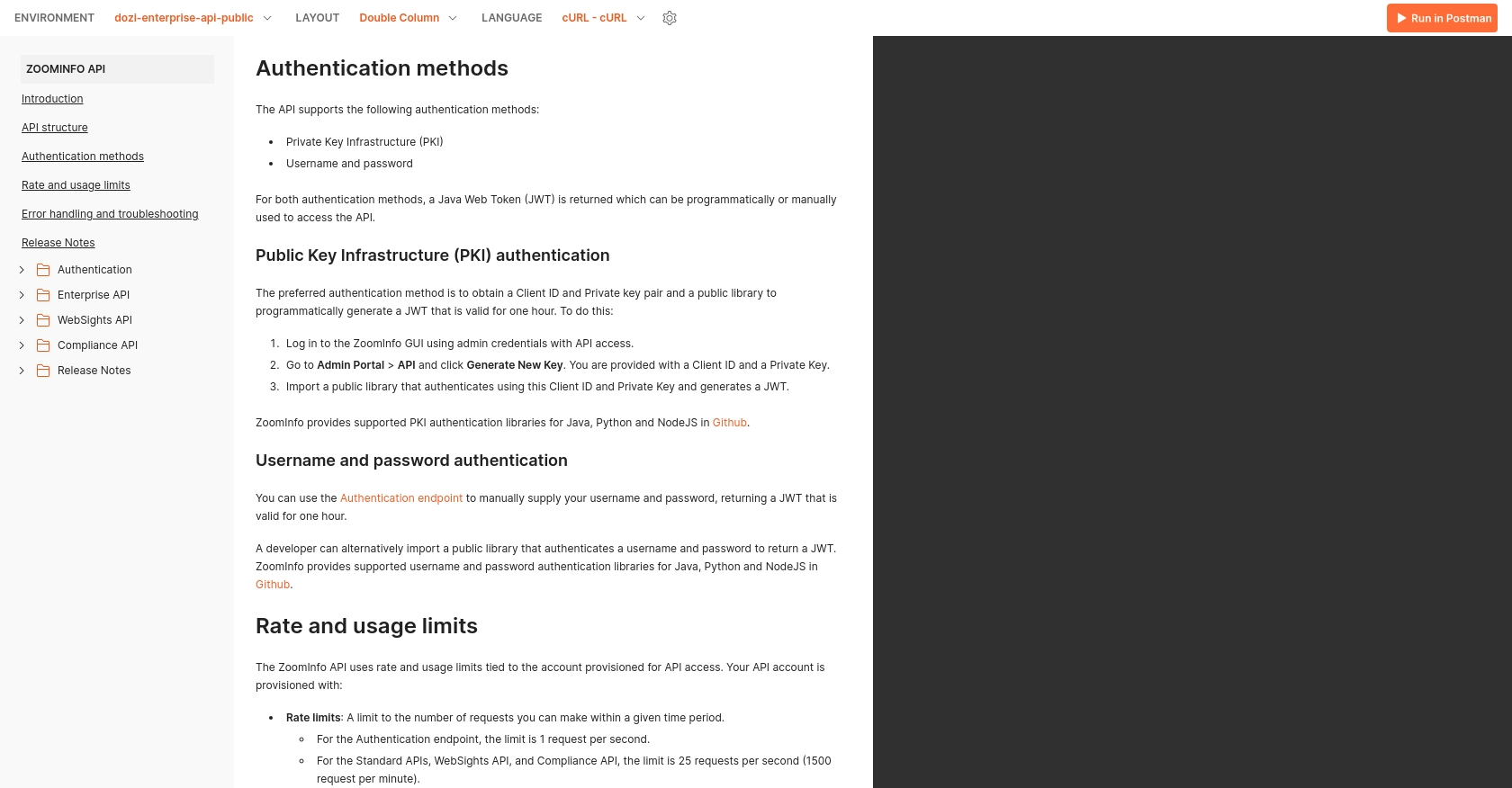
sbb-itb-96038d7
Making API Calls to Retrieve Company Data with ZoomInfo in PHP
To interact with the ZoomInfo API using PHP, you'll need to set up your environment and write the necessary code to make API calls. This section will guide you through the process, ensuring you can efficiently retrieve company data from ZoomInfo.
Setting Up Your PHP Environment for ZoomInfo API Integration
Before making API calls, ensure your PHP environment is ready. You'll need PHP version 7.4 or later and the cURL
extension enabled, as it is essential for making HTTP requests.
- Verify your PHP version by running
php -v
in your terminal. - Ensure the
cURL
extension is enabled by checking yourphp.ini
file or runningphp -m
to list installed modules.
Writing PHP Code to Make a ZoomInfo API Call
With your environment set up, you can now write the PHP code to make an API call to ZoomInfo. The following example demonstrates how to retrieve company data using your API credentials.
<?php
// Set the API endpoint
$endpoint = "https://api.zoominfo.com/company/search";
// Set your API credentials
$clientId = "Your_Client_ID";
$clientSecret = "Your_Client_Secret";
// Initialize cURL session
$ch = curl_init($endpoint);
// Set cURL options
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
"Content-Type: application/json",
"Client-ID: $clientId",
"Client-Secret: $clientSecret"
]);
// Execute the request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Request Error:' . curl_error($ch);
} else {
// Parse and display the response
$data = json_decode($response, true);
print_r($data);
}
// Close cURL session
curl_close($ch);
?>
Replace Your_Client_ID
and Your_Client_Secret
with the credentials you generated earlier. This script initializes a cURL session, sets the necessary headers, and executes a GET request to the ZoomInfo API endpoint.
Verifying API Call Success and Handling Errors
After executing the API call, it's crucial to verify the success of your request. Check the response for any errors and ensure the data returned matches the expected company information.
- If the request is successful, the response will contain the company data in JSON format.
- If an error occurs, the response will include an error message. Handle errors gracefully by checking the response code and displaying appropriate messages.
By following these steps, you can effectively retrieve company data from ZoomInfo using PHP, enabling you to integrate valuable business insights into your applications.
Conclusion: Best Practices for ZoomInfo API Integration in PHP
Integrating with the ZoomInfo API in PHP can significantly enhance your application's capabilities by providing access to rich company data. To ensure a seamless integration, consider the following best practices:
Securely Storing ZoomInfo API Credentials
Always store your API credentials securely. Avoid hardcoding them directly into your scripts. Instead, use environment variables or secure vaults to manage sensitive information, ensuring that your client ID and client secret remain protected.
Handling ZoomInfo API Rate Limiting
Be mindful of ZoomInfo's API rate limits to prevent service disruptions. Implement logic to handle rate limit responses gracefully, such as retrying requests after a specified delay. Check the ZoomInfo API documentation for specific rate limit details.
Standardizing and Transforming ZoomInfo Data
When retrieving data from ZoomInfo, ensure that you standardize and transform the data to fit your application's requirements. This may involve mapping fields, converting data types, or aggregating information to provide meaningful insights to your users.
Leverage Endgrate for Efficient Integration Management
If managing multiple integrations becomes overwhelming, consider using Endgrate to streamline the process. Endgrate allows you to build once for each use case, reducing the complexity of maintaining various integrations. Focus on your core product while Endgrate handles the integration details, providing an intuitive experience for your customers.
By following these best practices, you can effectively integrate ZoomInfo's powerful company data into your PHP applications, enhancing your business intelligence capabilities and driving better decision-making.
Read More
Ready to get started?