How to Get Contacts with the Cloze API in PHP
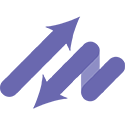
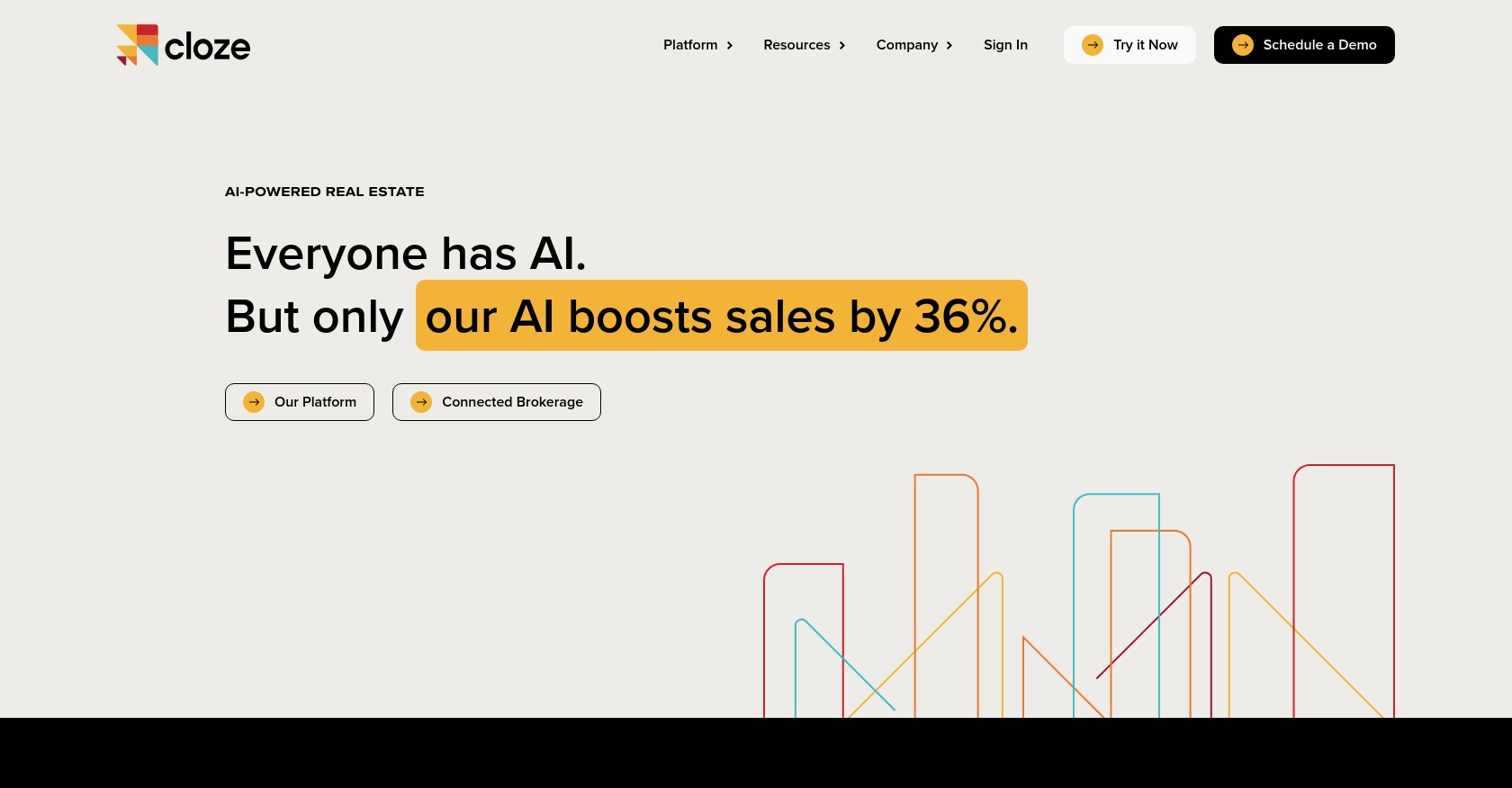
Introduction to Cloze API for Contact Management
Cloze is a comprehensive relationship management platform that helps businesses manage their contacts, projects, and communications effectively. It offers a suite of tools designed to streamline workflows and enhance productivity by integrating various aspects of customer relationship management.
Developers may want to integrate with the Cloze API to access and manage contact data efficiently. By leveraging the Cloze API, developers can automate tasks such as retrieving contact information, updating contact details, and managing communication records. For example, a developer could use the Cloze API to fetch contact lists and synchronize them with other CRM systems, ensuring data consistency across platforms.
Setting Up Your Cloze API Test Account
Before you can start using the Cloze API to manage contacts, you need to set up a test account. This will allow you to safely experiment with API calls without affecting live data. Follow the steps below to create a test account and obtain the necessary credentials for authentication.
Create a Cloze Account
- Visit the Cloze website and sign up for a free trial or demo account. This will give you access to the platform's features and allow you to test API integrations.
- Follow the on-screen instructions to complete the registration process. Once your account is created, you will be logged in to the Cloze dashboard.
Generate an API Key for Cloze API Access
To authenticate API requests, you will need an API key. Here's how to generate one:
- Navigate to the account settings in the Cloze dashboard.
- Locate the API section and follow the instructions to create a new API key.
- Make sure to copy and securely store your API key, as it will be used in your API requests.
Configure API Key Authentication
Once you have your API key, you can configure your PHP application to authenticate with the Cloze API:
// Set your Cloze account email and API key
$userEmail = 'your-email@example.com';
$apiKey = 'your-api-key';
// Example of setting up authentication parameters
$authParams = [
'user' => $userEmail,
'api_key' => $apiKey
];
Replace your-email@example.com
and your-api-key
with your actual Cloze account email and API key.
With your test account and API key set up, you're ready to start making API calls to manage contacts using the Cloze API. For more information on authentication, refer to the Cloze API documentation.
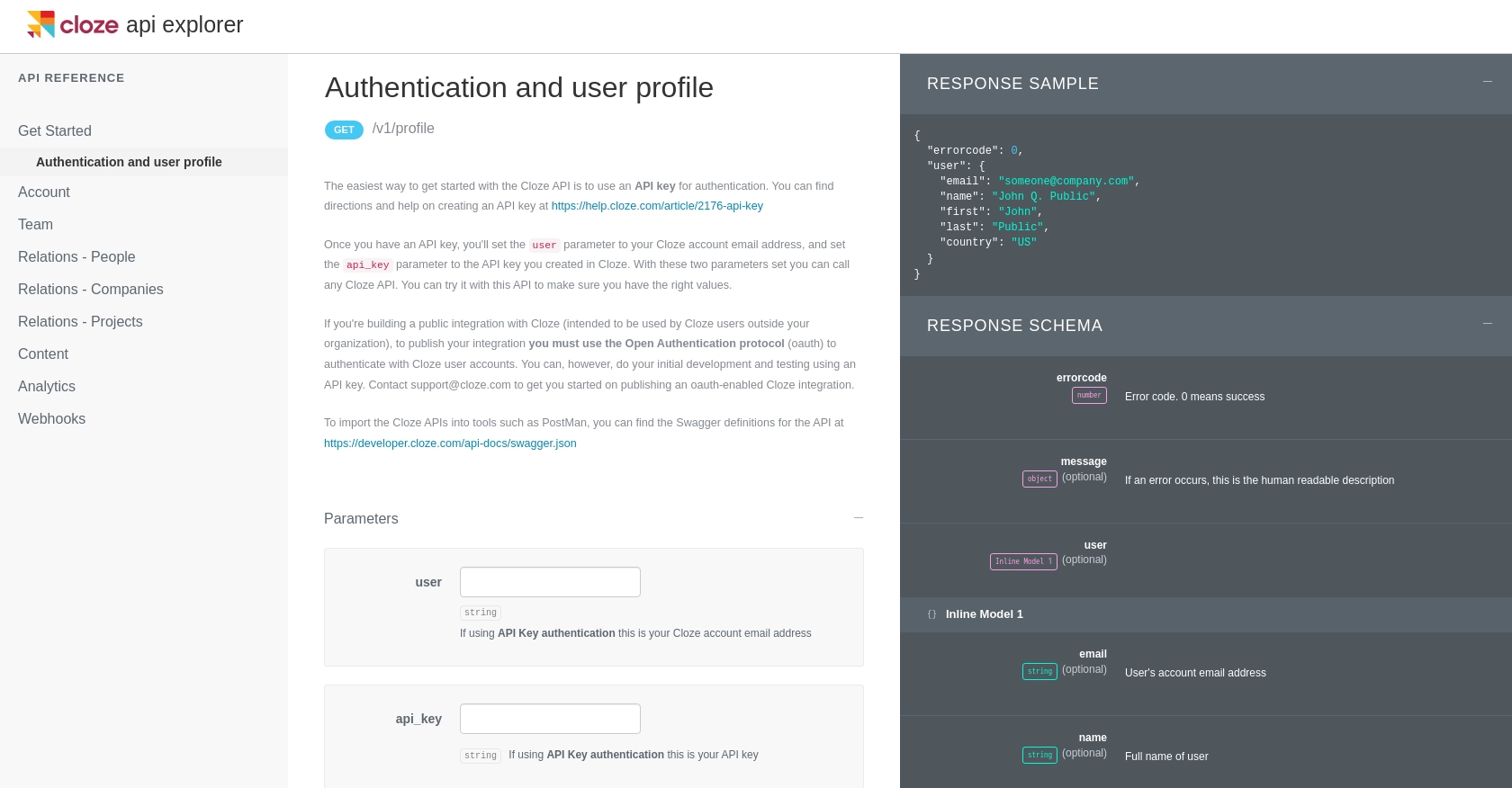
sbb-itb-96038d7
Making API Calls to Retrieve Contacts with Cloze API in PHP
To interact with the Cloze API and retrieve contact information, you'll need to set up your PHP environment and write code to make the necessary API calls. This section will guide you through the process of configuring your PHP application, making API requests, and handling responses.
Setting Up Your PHP Environment for Cloze API Integration
Before you begin, ensure that your PHP environment is properly configured. You'll need PHP version 7.4 or higher and the cURL
extension enabled to make HTTP requests.
- Verify your PHP version by running
php -v
in your terminal. - Ensure the
cURL
extension is enabled by checking yourphp.ini
file or runningphp -m
to list installed modules.
Installing Required PHP Dependencies
To handle HTTP requests, you'll use the cURL
library, which is typically included with PHP. If not, you can install it using your package manager. For example, on Ubuntu, you can run:
sudo apt-get install php-curl
Writing PHP Code to Fetch Contacts from Cloze API
With your environment set up, you can now write the PHP code to make an API call to Cloze and retrieve contacts. Below is a sample script to get you started:
<?php
// Set your Cloze account email and API key
$userEmail = 'your-email@example.com';
$apiKey = 'your-api-key';
// Cloze API endpoint for retrieving contacts
$endpoint = 'https://api.cloze.com/v1/people/get';
// Initialize cURL session
$ch = curl_init();
// Set cURL options
curl_setopt($ch, CURLOPT_URL, $endpoint);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Content-Type: application/json',
'user: ' . $userEmail,
'api_key: ' . $apiKey
]);
// Execute cURL request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'cURL error: ' . curl_error($ch);
} else {
// Parse and display the response
$data = json_decode($response, true);
if ($data['errorcode'] === 0) {
foreach ($data['people'] as $person) {
echo 'Name: ' . $person['name'] . '<br>';
echo 'Email: ' . $person['emails'][0]['value'] . '<br><br>';
}
} else {
echo 'Error: ' . $data['message'];
}
}
// Close cURL session
curl_close($ch);
?>
Replace your-email@example.com
and your-api-key
with your actual Cloze account email and API key. This script initializes a cURL session, sets the necessary headers, and makes a GET request to the Cloze API to retrieve contacts.
Verifying API Call Success and Handling Errors
After executing the API call, it's important to verify the success of the request. The response will include an errorcode
field, where 0
indicates success. If an error occurs, the message
field will provide a human-readable description of the issue.
For more detailed information on error codes and handling, refer to the Cloze API documentation.
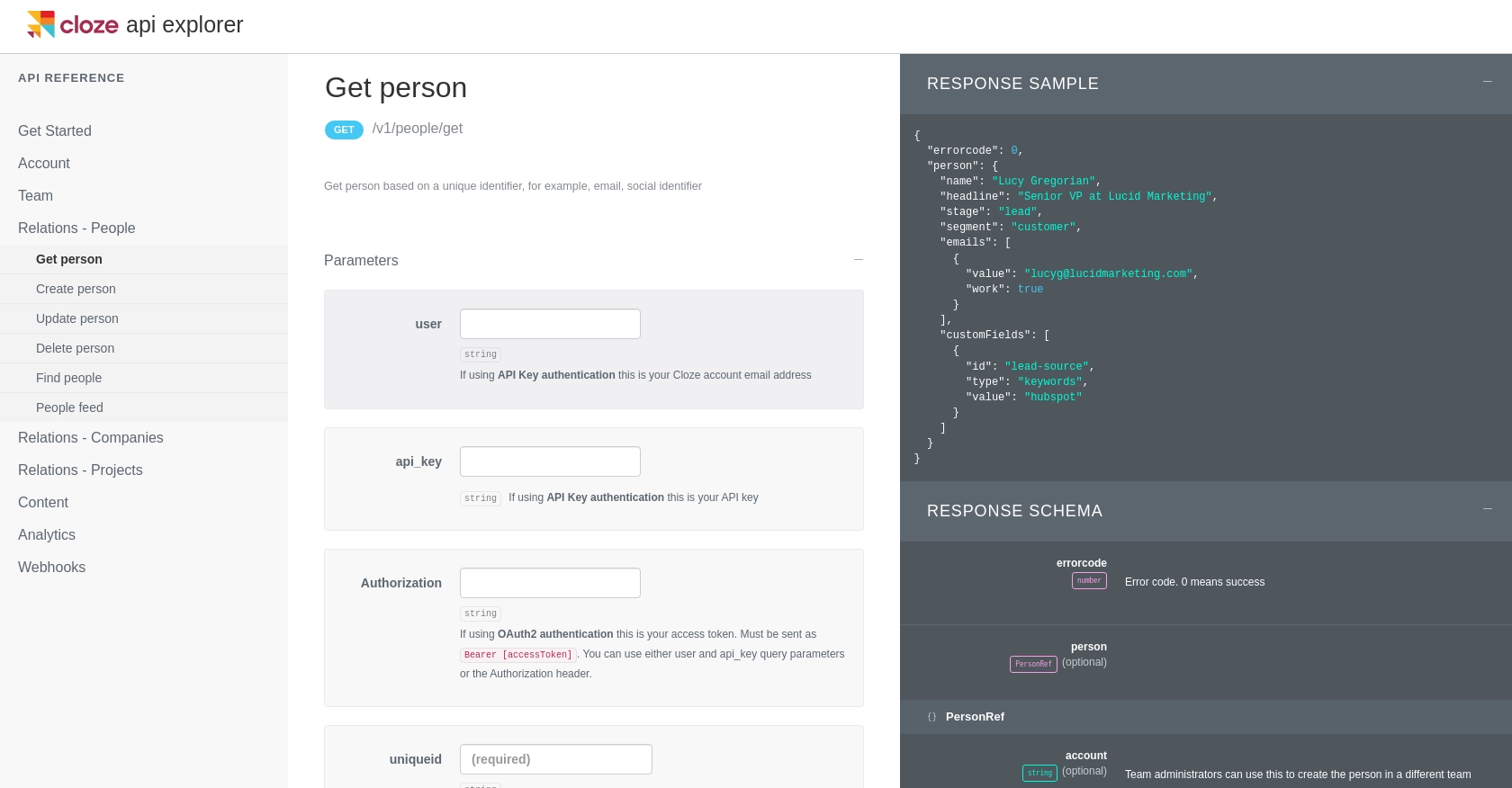
Conclusion and Best Practices for Using Cloze API in PHP
Integrating the Cloze API into your PHP applications can significantly enhance your ability to manage contacts and streamline CRM processes. By automating tasks such as retrieving and updating contact information, you can ensure data consistency and improve productivity across your organization.
Best Practices for Secure and Efficient API Integration
- Secure API Keys: Always store your API keys securely. Avoid hardcoding them into your source code. Instead, use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be aware of any rate limits imposed by the Cloze API to prevent your application from being throttled. Implement exponential backoff strategies to handle retries gracefully.
- Data Transformation: Consider transforming and standardizing data fields to ensure compatibility with other systems you may be integrating with.
- Error Handling: Implement robust error handling to manage API failures and provide meaningful feedback to users. Refer to the Cloze API documentation for detailed error code information.
Streamlining Integrations with Endgrate
While integrating with the Cloze API can be straightforward, managing multiple integrations across different platforms can become complex. Endgrate offers a unified API solution that simplifies this process by providing a single endpoint to connect with various platforms, including Cloze.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development. Build once for each use case and enjoy an intuitive integration experience for your customers. Visit Endgrate to learn more about how you can streamline your integration efforts.
Read More
Ready to get started?