Using the Google Sheets API to Create or Update Records (with PHP examples)
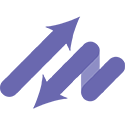
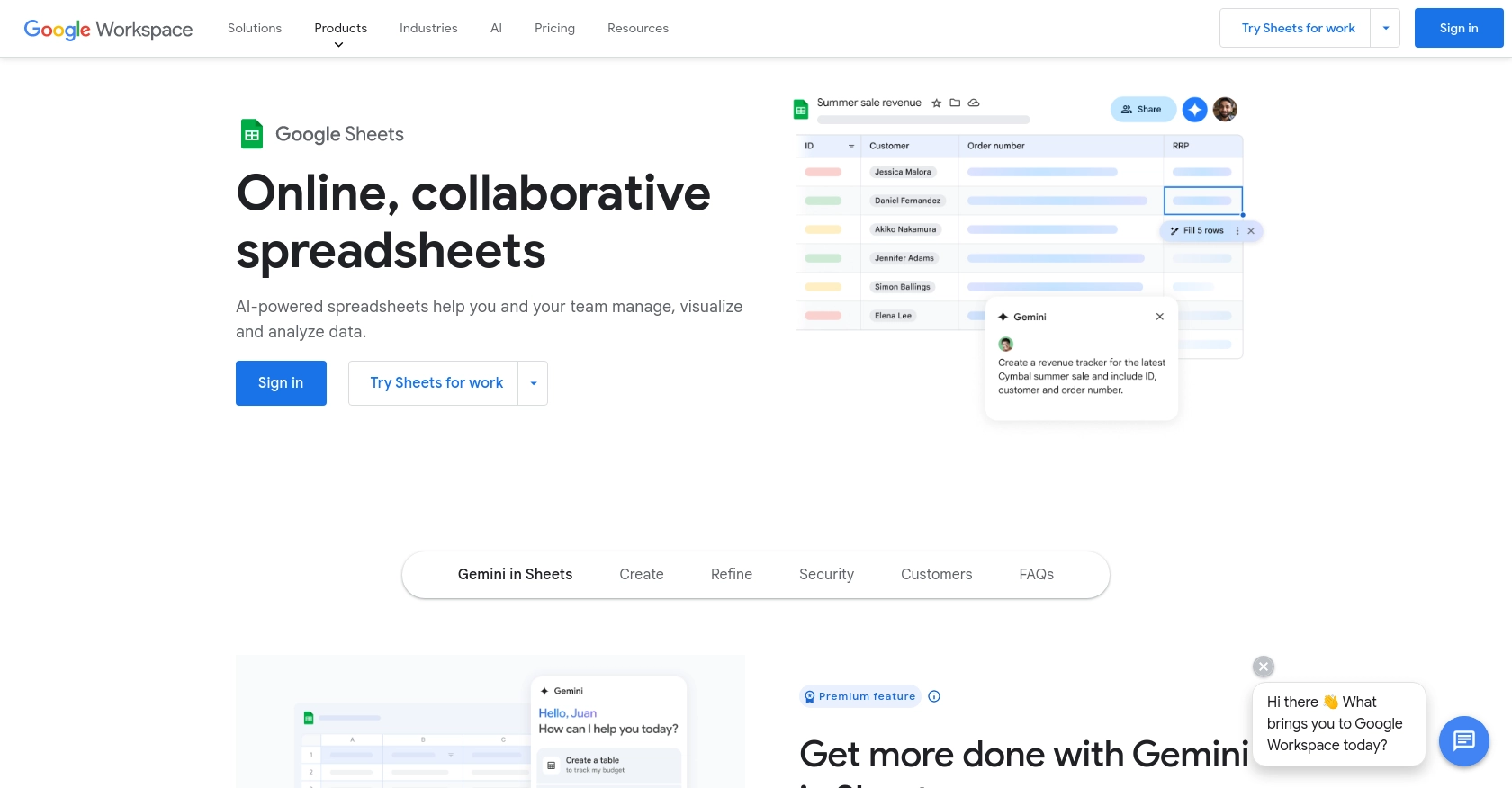
Introduction to Google Sheets API for PHP Developers
Google Sheets is a versatile and widely-used spreadsheet application that allows users to create, edit, and collaborate on spreadsheets online. It is part of the Google Workspace suite and offers powerful features for data management and analysis.
For developers, integrating with the Google Sheets API can significantly enhance the functionality of their applications by enabling automated data manipulation and retrieval. This API provides a RESTful interface to interact with Google Sheets, allowing you to create, read, update, and delete spreadsheet data programmatically.
One common use case for integrating with the Google Sheets API is to automate the process of updating records. For example, a developer might want to update a sales report in Google Sheets with data from a PHP application, ensuring that the spreadsheet always reflects the latest information.
Setting Up Your Google Sheets API Test Environment
Before you can start using the Google Sheets API with PHP, you need to set up a Google Cloud project and configure OAuth 2.0 authentication. This setup allows your application to securely access Google Sheets on behalf of users.
Create a Google Cloud Project for Google Sheets API Access
- Go to the Google Cloud Console and sign in with your Google account.
- Click on the Menu icon, navigate to IAM & Admin, and select Create a Project.
- Enter a descriptive name for your project and click Create.
Enable Google Sheets API in Your Project
- In the Google Cloud Console, go to APIs & Services and select Library.
- Search for Google Sheets API and click on it.
- Click Enable to activate the API for your project.
Configure OAuth Consent Screen for Google Sheets API
- In the Google Cloud Console, navigate to APIs & Services and select OAuth consent screen.
- Select the user type for your app and click Create.
- Fill out the required fields, such as App name and User support email, and click Save and Continue.
Create OAuth 2.0 Credentials for Google Sheets API
- Go to APIs & Services and select Credentials.
- Click Create Credentials and choose OAuth client ID.
- Select Web application as the application type.
- Enter a name for your client ID and configure the Authorized redirect URIs as needed.
- Click Create to generate your Client ID and Client Secret.
Make sure to securely store your Client ID and Client Secret, as you will need them to authenticate your application with the Google Sheets API.
For more detailed information, refer to the official Google Sheets API documentation: Google Sheets API Concepts, Create a Google Cloud Project, and Configure OAuth Consent.
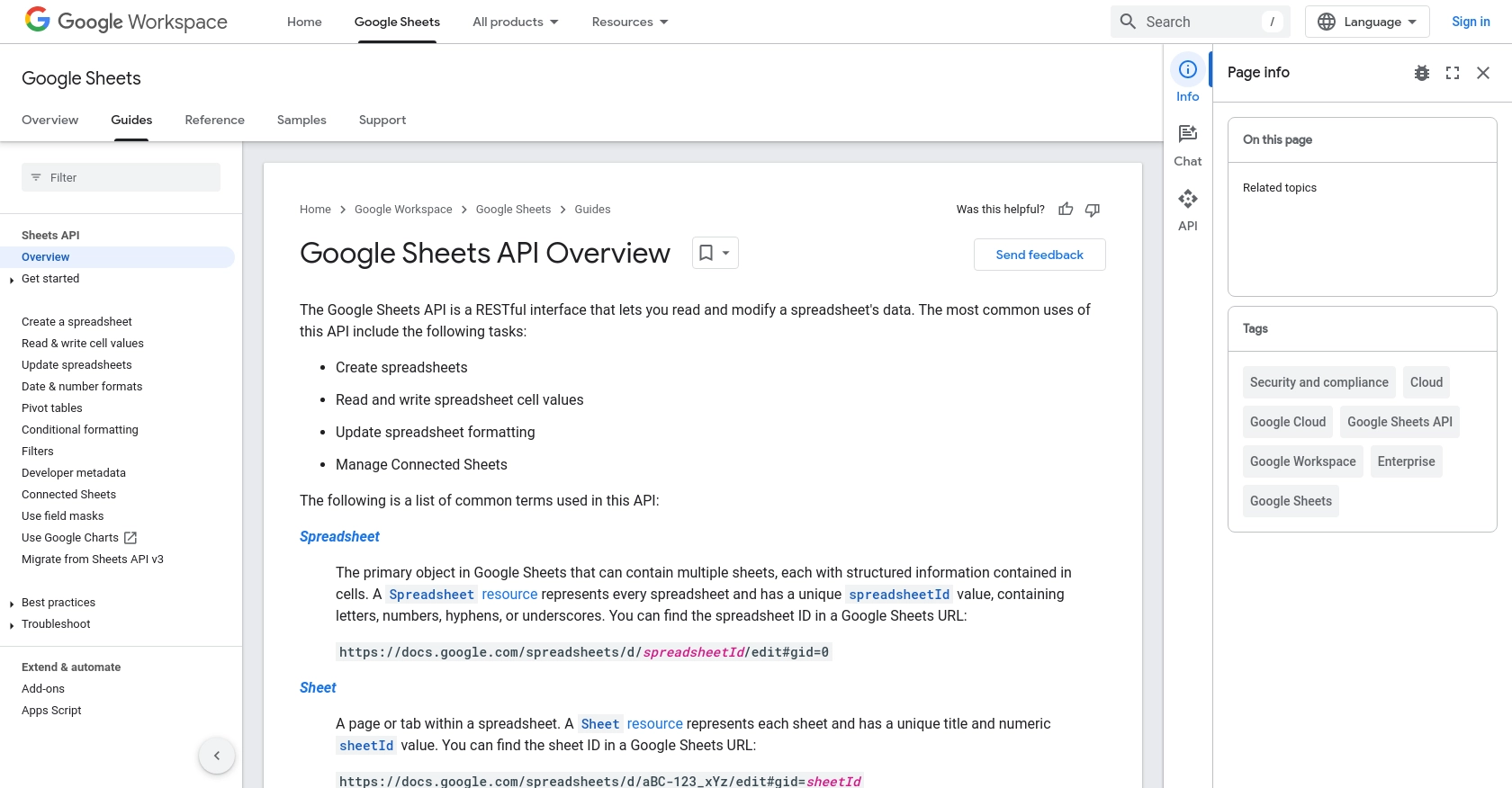
sbb-itb-96038d7
Making API Calls to Google Sheets Using PHP
To interact with Google Sheets using PHP, you need to make HTTP requests to the Google Sheets API. This section will guide you through the process of setting up your PHP environment, installing necessary dependencies, and executing API calls to create or update records in Google Sheets.
Setting Up Your PHP Environment for Google Sheets API
Before making API calls, ensure your PHP environment is properly configured. You will need PHP 7.4 or later and the Composer package manager to manage dependencies.
- Install Composer by following the instructions on the Composer website.
- In your project directory, run the following command to install the Google Client Library for PHP:
composer require google/apiclient:^2.0
Authenticating with Google Sheets API Using OAuth 2.0
To authenticate your application, use the OAuth 2.0 credentials you created earlier. This involves setting up a client and obtaining an access token.
require 'vendor/autoload.php';
use Google\Client;
use Google\Service\Sheets;
$client = new Client();
$client->setApplicationName('Google Sheets API PHP Quickstart');
$client->setScopes(Sheets::SPREADSHEETS);
$client->setAuthConfig('credentials.json');
$client->setAccessType('offline');
$client->setPrompt('select_account consent');
// Load previously authorized token from a file, if it exists.
$tokenPath = 'token.json';
if (file_exists($tokenPath)) {
$accessToken = json_decode(file_get_contents($tokenPath), true);
$client->setAccessToken($accessToken);
}
// If there is no previous token or it's expired, get a new one.
if ($client->isAccessTokenExpired()) {
if ($client->getRefreshToken()) {
$client->fetchAccessTokenWithRefreshToken($client->getRefreshToken());
} else {
$authUrl = $client->createAuthUrl();
printf("Open the following link in your browser:\n%s\n", $authUrl);
print 'Enter verification code: ';
$authCode = trim(fgets(STDIN));
$accessToken = $client->fetchAccessTokenWithAuthCode($authCode);
$client->setAccessToken($accessToken);
if (!file_exists(dirname($tokenPath))) {
mkdir(dirname($tokenPath), 0700, true);
}
file_put_contents($tokenPath, json_encode($client->getAccessToken()));
}
}
Creating or Updating Records in Google Sheets with PHP
Once authenticated, you can perform operations on Google Sheets. Here’s how to create or update records:
$service = new Sheets($client);
$spreadsheetId = 'your_spreadsheet_id';
$range = 'Sheet1!A1:D5';
$values = [
["Name", "Age", "Email", "Location"],
["John Doe", "30", "john.doe@example.com", "New York"],
["Jane Smith", "25", "jane.smith@example.com", "Los Angeles"]
];
$body = new Sheets\ValueRange([
'values' => $values
]);
$params = [
'valueInputOption' => 'RAW'
];
$result = $service->spreadsheets_values->update($spreadsheetId, $range, $body, $params);
printf("%d cells updated.", $result->getUpdatedCells());
In this example, we specify the spreadsheet ID and the range of cells to update. The data is provided in a two-dimensional array, and the update
method is used to write the data to the specified range.
Handling Errors and Verifying API Requests
To ensure your API requests are successful, handle potential errors and verify the results:
- Check the HTTP status code returned by the API. A status code of 200 indicates success.
- Use try-catch blocks to handle exceptions and log error messages for debugging.
- Verify the changes in your Google Sheets by checking the updated data directly in the spreadsheet.
For more detailed information on error handling, refer to the Google Sheets API Reference.
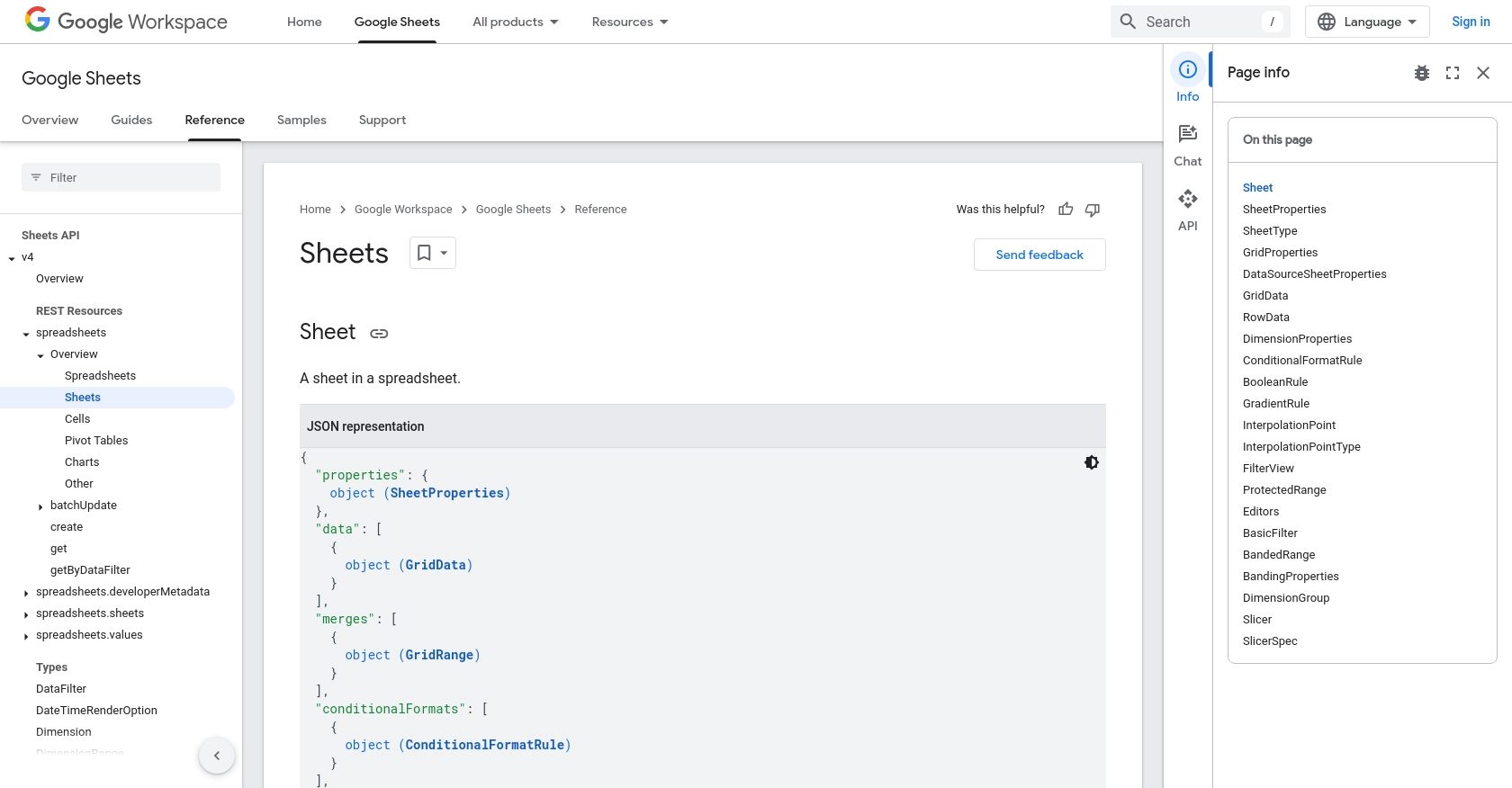
Conclusion and Best Practices for Using Google Sheets API with PHP
Integrating the Google Sheets API with PHP offers a powerful way to automate and manage spreadsheet data programmatically. By following the steps outlined in this guide, you can efficiently create or update records in Google Sheets, enhancing your application's functionality.
Best Practices for Secure and Efficient Google Sheets API Integration
- Securely Store Credentials: Always store your OAuth 2.0 credentials and tokens securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limiting: Be mindful of the API's rate limits to prevent exceeding the allowed number of requests. Implement exponential backoff strategies to handle retries gracefully.
- Optimize Data Handling: When dealing with large datasets, consider batching your requests to minimize the number of API calls and improve performance.
- Validate User Input: Ensure that any data being written to Google Sheets is validated and sanitized to prevent errors and maintain data integrity.
Enhancing Your Integration with Endgrate
While integrating with the Google Sheets API can be straightforward, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process across various platforms, including Google Sheets.
By leveraging Endgrate, you can focus on your core product development while outsourcing the integration complexities. This approach allows you to build once for each use case, providing an intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate.
Read More
- https://endgrate.com/provider/googlesheets
- https://developers.google.com/sheets/api/guides/concepts
- https://developers.google.com/sheets/api/reference/rest
- https://developers.google.com/workspace/guides/create-project
- https://developers.google.com/workspace/guides/enable-apis
- https://developers.google.com/workspace/guides/configure-oauth-consent
- https://developers.google.com/workspace/guides/create-credentials
- https://developers.google.com/sheets/api/reference/rest/v4/spreadsheets/sheets
- https://developers.google.com/sheets/api/reference/rest/v4/spreadsheets/cells
Ready to get started?