Using the Teamleader API to Create Or Update Company (with Python examples)
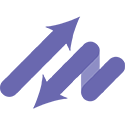
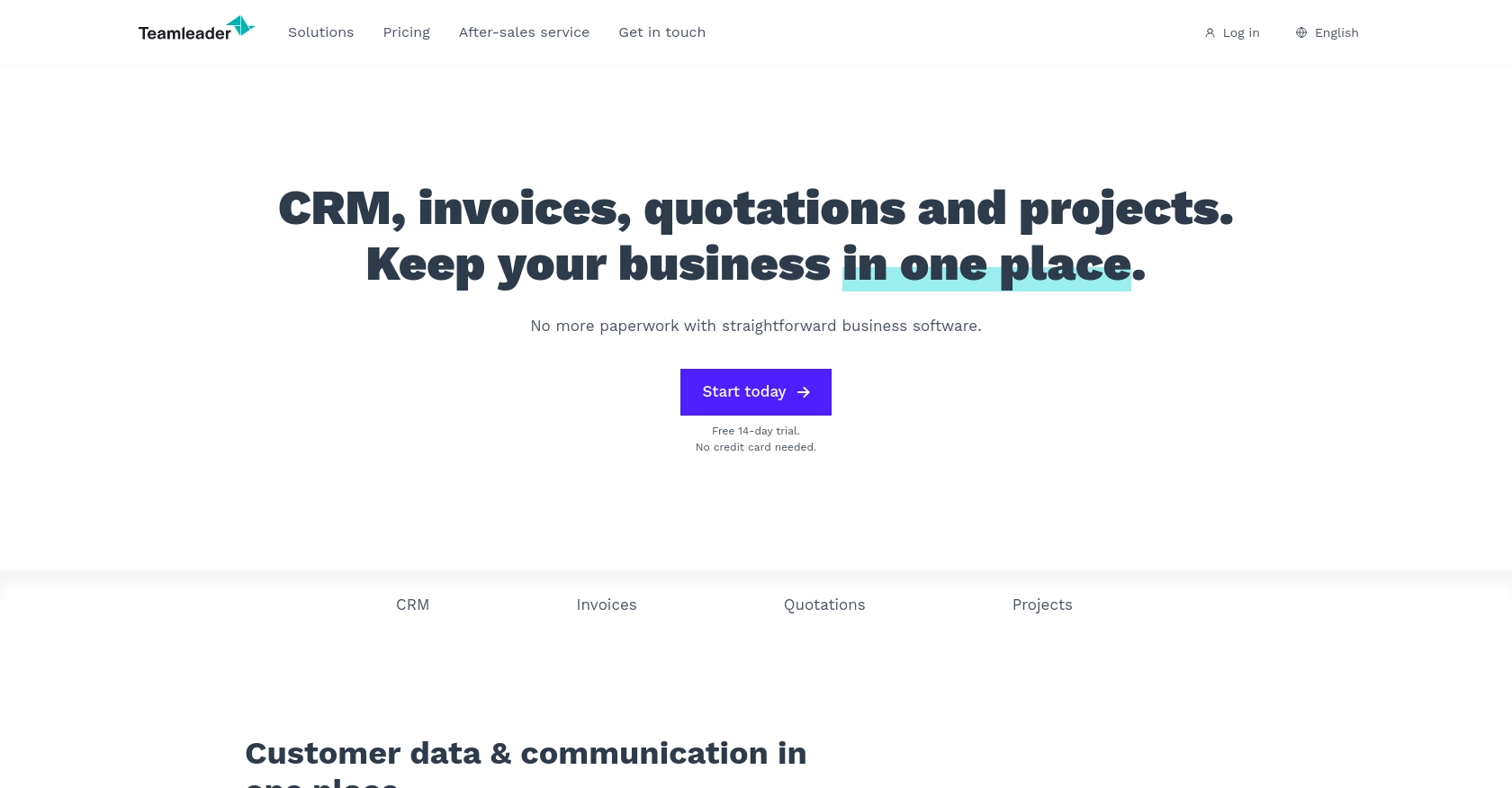
Introduction to Teamleader API
Teamleader is a versatile business management software designed to help companies streamline their operations by integrating CRM, project management, and invoicing functionalities. It offers a comprehensive platform that enables businesses to manage their customer relationships, track project progress, and handle billing efficiently.
Developers might want to integrate with the Teamleader API to automate and enhance business processes. For example, using the Teamleader API, a developer can create or update company information directly from an external application, ensuring that the CRM data remains accurate and up-to-date without manual intervention.
This article will guide you through using Python to interact with the Teamleader API for creating or updating company records, providing a step-by-step approach to streamline your integration efforts.
Setting Up a Teamleader Test or Sandbox Account for API Integration
Before you can start integrating with the Teamleader API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data. Teamleader provides a sandbox environment that mimics the production environment, enabling developers to test their integrations thoroughly.
Creating a Teamleader Sandbox Account
To begin, you'll need to create a Teamleader account if you haven't already. Follow these steps to set up your sandbox environment:
- Visit the Teamleader Developer Portal and sign up for a developer account.
- Once registered, log in to your account and navigate to the sandbox section.
- Follow the instructions to create a new sandbox environment. This will provide you with a separate space to test your API interactions.
Configuring OAuth Authentication for Teamleader API
The Teamleader API uses OAuth 2.0 for authentication. This ensures secure access to your data. To set up OAuth authentication, follow these steps:
- In your sandbox account, navigate to the "Apps" section and create a new app.
- Provide the necessary details for your app, such as the app name and redirect URI.
- Once your app is created, you will receive a client ID and client secret. Keep these credentials secure as they are essential for authenticating API requests.
- Use the client ID and client secret to obtain an access token by following the OAuth 2.0 flow. This token will be used to authorize API requests.
With your sandbox account and OAuth authentication set up, you're ready to start making API calls to Teamleader. In the next section, we'll explore how to use Python to create or update company records using the Teamleader API.
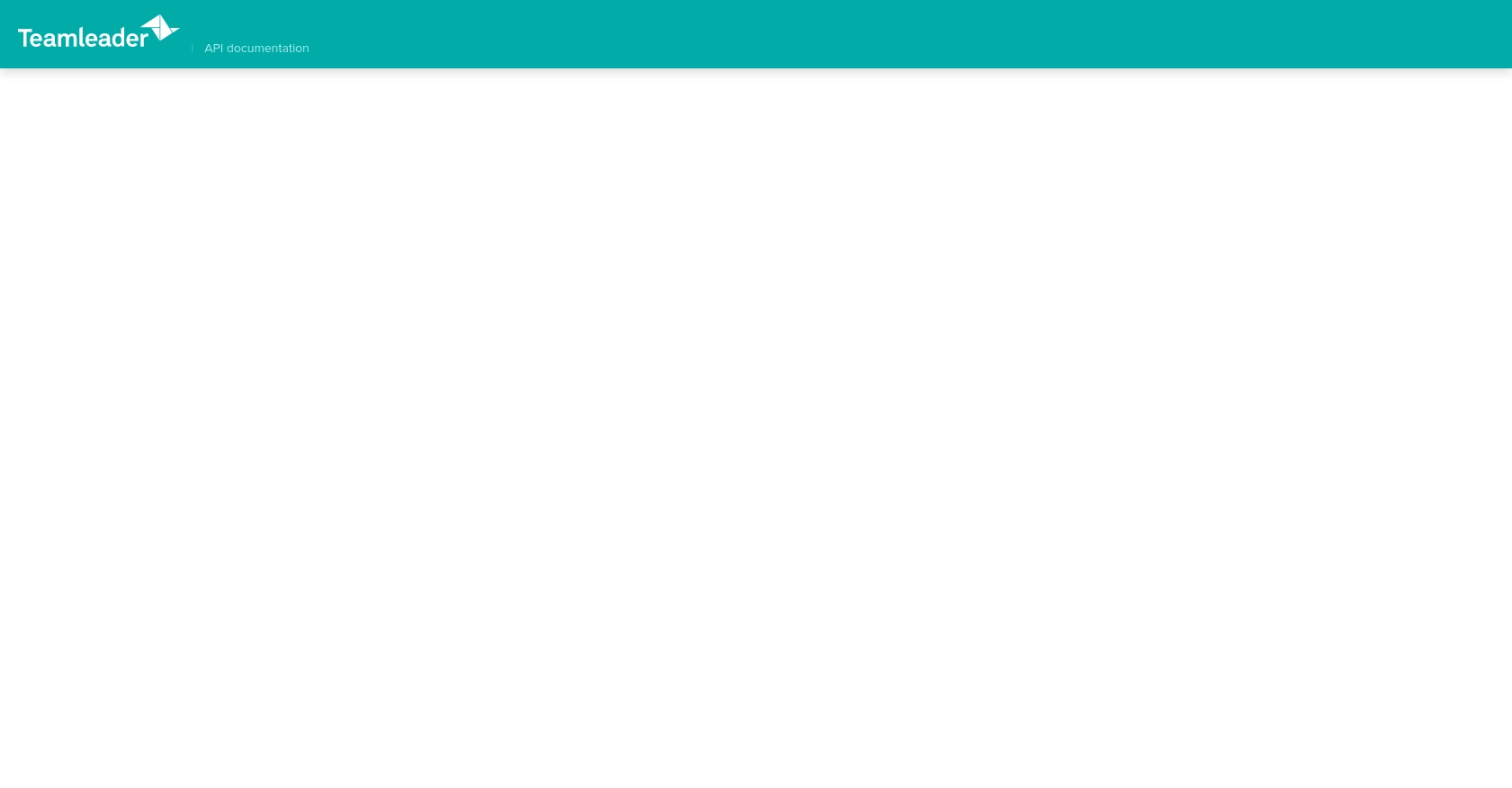
sbb-itb-96038d7
How to Make API Calls to Teamleader for Creating or Updating Company Records Using Python
To interact with the Teamleader API for creating or updating company records, you'll need to use Python. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling responses.
Setting Up Your Python Environment for Teamleader API Integration
Before you begin coding, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1
- The Python package installer, pip
Next, install the requests
library, which is essential for making HTTP requests:
pip install requests
Writing Python Code to Create or Update Company Records in Teamleader
With your environment set up, you can now write the Python code to interact with the Teamleader API. Below is an example of how to create or update a company record:
import requests
# Define the API endpoint for creating or updating a company
url = "https://api.teamleader.eu/companies.createOrUpdate"
# Set the request headers, including the authorization token
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_Access_Token"
}
# Define the company data to be created or updated
company_data = {
"name": "Example Company",
"business_type": "customer",
"emails": [{"type": "primary", "email": "contact@example.com"}],
"telephones": [{"type": "phone", "number": "+123456789"}]
}
# Make the POST request to the Teamleader API
response = requests.post(url, json=company_data, headers=headers)
# Check the response status and handle accordingly
if response.status_code == 200:
print("Company created or updated successfully.")
else:
print(f"Failed to create or update company. Error: {response.status_code} - {response.text}")
Replace Your_Access_Token
with the access token obtained during the OAuth authentication setup.
Verifying API Call Success in Teamleader Sandbox
After running the code, verify the success of your API call by checking the company records in your Teamleader sandbox account. If the operation was successful, the new or updated company should appear in the list of companies.
Handling Errors and Understanding Teamleader API Error Codes
When making API calls, it's crucial to handle potential errors. The Teamleader API may return various error codes, indicating issues such as authentication failures or invalid data. Always check the response status code and handle errors gracefully in your application.
For more detailed information on error codes, refer to the Teamleader API Documentation.
Conclusion and Best Practices for Using Teamleader API with Python
Integrating with the Teamleader API using Python can significantly enhance your business operations by automating the creation and updating of company records. By following the steps outlined in this article, you can streamline your integration process and ensure your CRM data remains accurate and up-to-date.
Best Practices for Secure and Efficient API Integration with Teamleader
- Secure Storage of Credentials: Always store your OAuth credentials, such as client ID, client secret, and access tokens, securely. Consider using environment variables or a secure vault service to keep these sensitive details safe.
- Handling Rate Limits: Be mindful of the API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully. Refer to the Teamleader API Documentation for specific rate limit details.
- Data Standardization: Ensure that the data you send to the API is standardized and validated to prevent errors. Consistent data formats will help maintain data integrity across your systems.
- Error Handling: Implement robust error handling to manage API response errors effectively. Log errors for troubleshooting and provide user-friendly messages to inform users of any issues.
Streamlining Your Integration Process with Endgrate
While integrating with the Teamleader API can be straightforward, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process across various platforms, including Teamleader.
By using Endgrate, you can save time and resources, allowing you to focus on your core product development. With a single API endpoint, you can manage multiple integrations efficiently, providing a seamless experience for your customers.
Explore how Endgrate can transform your integration strategy by visiting Endgrate and discover the benefits of a streamlined integration process.
Read More
Ready to get started?