Using the Zoho CRM API to Get Notes in Javascript
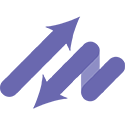
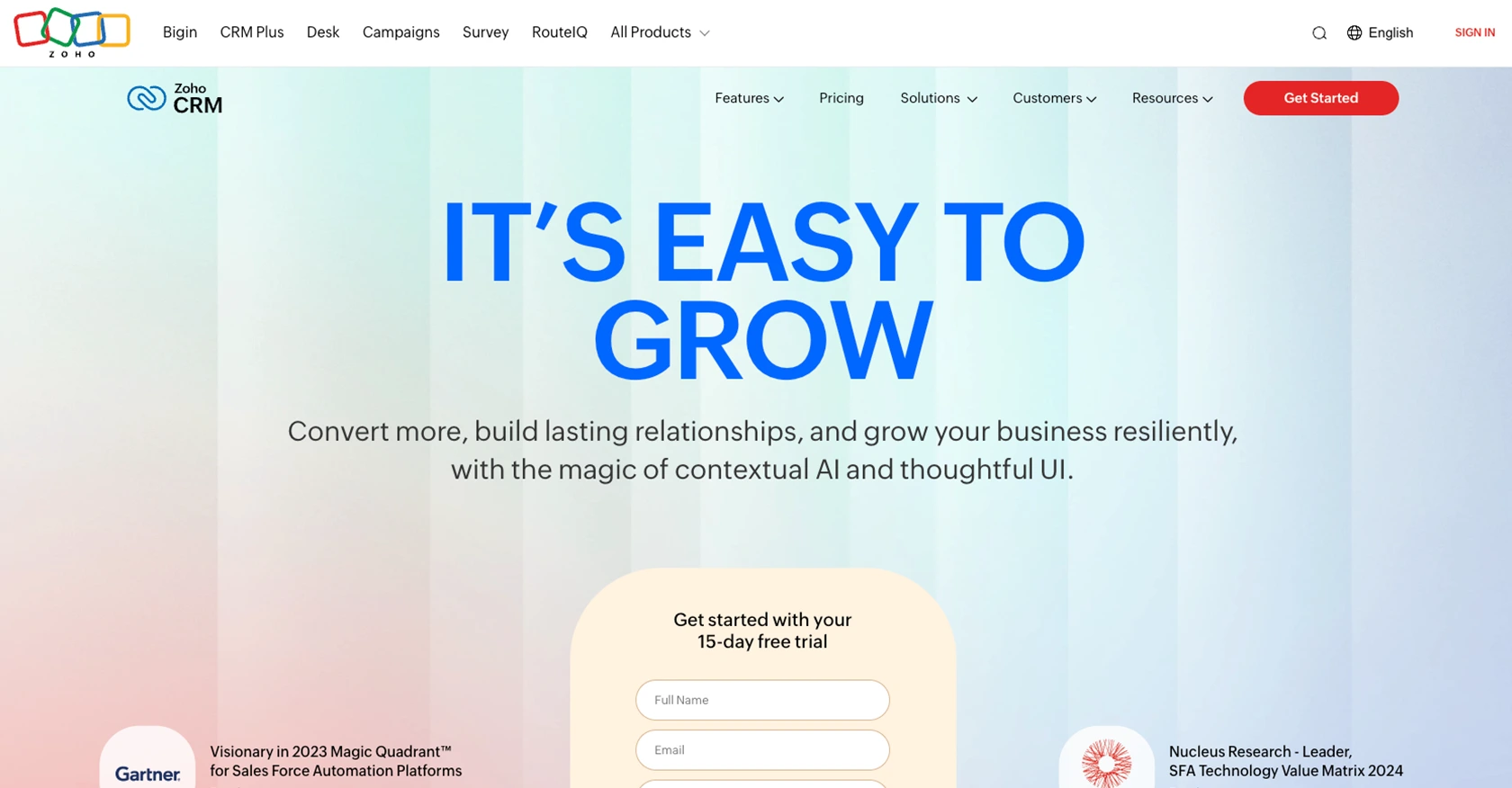
Introduction to Zoho CRM API Integration
Zoho CRM is a comprehensive customer relationship management platform that empowers businesses to manage their sales, marketing, and support in a unified manner. Known for its flexibility and extensive features, Zoho CRM is a popular choice for businesses aiming to enhance their customer interactions and streamline operations.
Integrating with Zoho CRM's API allows developers to automate and enhance various business processes. For example, accessing notes through the Zoho CRM API can help developers keep track of customer interactions and insights, ensuring that all team members have up-to-date information. This can be particularly useful for sales teams looking to personalize their communication strategies based on historical data.
This article will guide you through the process of using JavaScript to interact with the Zoho CRM API, specifically focusing on retrieving notes. By following this tutorial, developers can efficiently access and manage notes within the Zoho CRM platform, enhancing their application's functionality and user experience.
Setting Up Your Zoho CRM Test/Sandbox Account for API Integration
Before you can start interacting with the Zoho CRM API using JavaScript, you need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting your live data.
Create a Zoho CRM Account
If you don't already have a Zoho CRM account, you can sign up for a free trial on the Zoho CRM website. Follow the instructions to create your account. Once your account is set up, you can log in to access the CRM dashboard.
Register Your Application in Zoho Developer Console
To use the Zoho CRM API, you need to register your application to obtain the necessary credentials for OAuth authentication. Follow these steps:
- Go to the Zoho Developer Console.
- Select JavaScript as the client type since you'll be using JavaScript for API calls.
- Fill in the required details:
- Client Name: The name of your application.
- Homepage URL: The URL of your application's homepage.
- Authorized Redirect URIs: A valid URL where Zoho will redirect after successful authentication.
- Click Create to register your application.
Upon successful registration, you will receive a Client ID and Client Secret. Keep these credentials secure as they are essential for authenticating API requests.
Configure OAuth Scopes for Zoho CRM API
Next, you need to configure the OAuth scopes to specify the type of access your application requires. For accessing notes, use the following scope:
scope=ZohoCRM.modules.notes.READ
Ensure that you include this scope when making the authorization request to gain the necessary permissions.
Generate Access and Refresh Tokens
With your application registered and scopes configured, you can now generate access and refresh tokens:
- Direct the user to Zoho's authorization URL with your client ID and requested scopes.
- Upon user consent, Zoho will redirect to your specified URI with an authorization code.
- Exchange this authorization code for access and refresh tokens by making a POST request to Zoho's token endpoint.
Refer to the Zoho CRM OAuth Overview for detailed instructions on generating tokens.
With your test account set up and tokens generated, you're ready to start making API calls to retrieve notes from Zoho CRM using JavaScript.
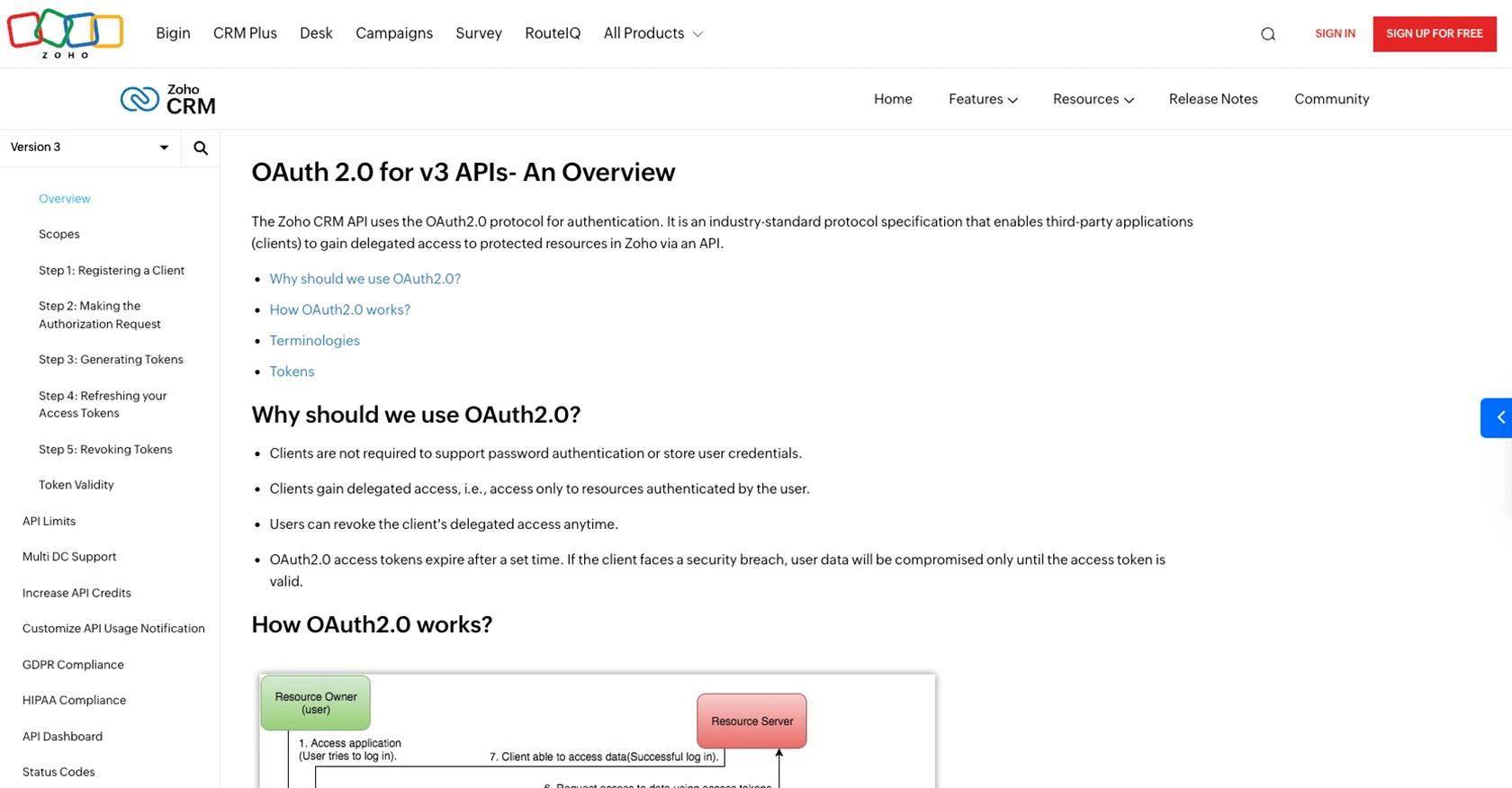
sbb-itb-96038d7
Making API Calls to Retrieve Notes from Zoho CRM Using JavaScript
To interact with the Zoho CRM API and retrieve notes using JavaScript, you'll need to set up your development environment and write the necessary code to make API requests. This section will guide you through the process, including setting up JavaScript, installing dependencies, and writing the code to fetch notes from Zoho CRM.
Setting Up Your JavaScript Environment for Zoho CRM API Integration
Before you start coding, ensure you have the following prerequisites installed on your machine:
- Node.js (version 14 or higher)
- NPM (Node Package Manager)
Once you have Node.js and NPM installed, create a new project directory and initialize it:
mkdir zoho-crm-integration
cd zoho-crm-integration
npm init -y
Installing Required Dependencies for Zoho CRM API Calls
You'll need the axios
library to make HTTP requests to the Zoho CRM API. Install it using the following command:
npm install axios
Writing JavaScript Code to Fetch Notes from Zoho CRM
With your environment set up, you can now write the JavaScript code to retrieve notes from Zoho CRM. Create a new file named getNotes.js
and add the following code:
const axios = require('axios');
// Replace with your access token
const accessToken = 'YOUR_ACCESS_TOKEN';
// Zoho CRM API endpoint for fetching notes
const endpoint = 'https://www.zohoapis.com/crm/v3/Notes';
// Function to get notes from Zoho CRM
async function getNotes() {
try {
const response = await axios.get(endpoint, {
headers: {
Authorization: `Zoho-oauthtoken ${accessToken}`
}
});
// Check if the request was successful
if (response.status === 200) {
const notes = response.data.data;
console.log('Retrieved Notes:', notes);
} else {
console.error('Failed to retrieve notes:', response.status, response.statusText);
}
} catch (error) {
console.error('Error fetching notes:', error.response ? error.response.data : error.message);
}
}
// Execute the function
getNotes();
Replace YOUR_ACCESS_TOKEN
with the access token you generated during the OAuth setup. This script uses the axios
library to send a GET request to the Zoho CRM API endpoint for notes. It handles the response by checking the status code and printing the retrieved notes to the console.
Running Your JavaScript Script to Fetch Zoho CRM Notes
To execute the script and fetch notes from Zoho CRM, run the following command in your terminal:
node getNotes.js
If successful, you should see the notes data printed in the console. This confirms that your API call was successful and the notes were retrieved from Zoho CRM.
Handling Errors and Verifying API Call Success
It's important to handle potential errors when making API calls. The code above includes error handling to catch and log any issues that arise during the request. Additionally, verify the success of your API call by checking the response status and reviewing the notes data in your Zoho CRM sandbox account.
For more details on error codes, refer to the Zoho CRM Status Codes documentation.
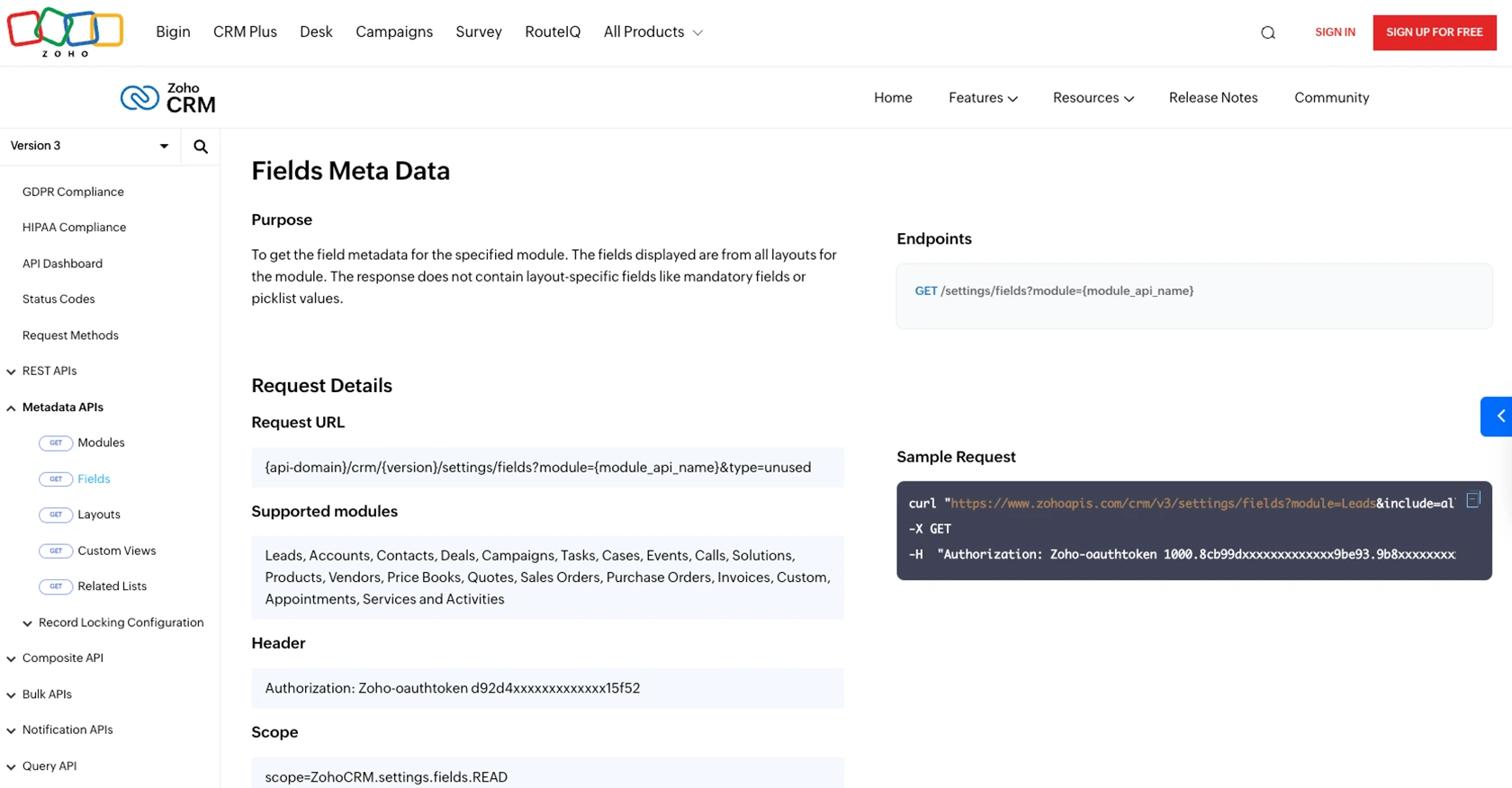
Conclusion and Best Practices for Zoho CRM API Integration
Integrating with the Zoho CRM API using JavaScript provides developers with powerful tools to enhance their applications by accessing and managing customer data efficiently. By following the steps outlined in this guide, you can successfully retrieve notes from Zoho CRM, enabling your team to maintain up-to-date and personalized customer interactions.
Best Practices for Zoho CRM API Integration
- Securely Store Credentials: Ensure that your OAuth tokens and client credentials are stored securely. Avoid exposing them in public repositories or client-side code.
- Handle Rate Limiting: Zoho CRM imposes API rate limits. Monitor your API usage and implement retry logic to handle rate limit errors gracefully. For more details, refer to the Zoho CRM API Limits documentation.
- Standardize Data Fields: When integrating with multiple APIs, consider standardizing data fields to ensure consistency across different platforms.
- Implement Error Handling: Robust error handling is crucial for identifying and resolving issues quickly. Log errors and provide meaningful feedback to users when API calls fail.
Enhance Your Integration Strategy with Endgrate
For developers looking to streamline their integration processes, Endgrate offers a unified API solution that simplifies connecting to multiple platforms, including Zoho CRM. By using Endgrate, you can save time and resources, allowing your team to focus on core product development while providing a seamless integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/zohocrm
- https://www.zoho.com/crm/developer/docs/api/v3/oauth-overview.html
- https://www.zoho.com/crm/developer/docs/api/v3/scopes.html
- https://www.zoho.com/crm/developer/docs/api/v3/register-client.html
- https://www.zoho.com/crm/developer/docs/api/v3/api-limits.html
- https://www.zoho.com/crm/developer/docs/api/v3/status-codes.html
- https://www.zoho.com/crm/developer/docs/api/v3/field-meta.html
- https://www.zoho.com/crm/developer/docs/api/v3/get-records.html
Ready to get started?