Using the Mailchimp API to Create Members in Javascript
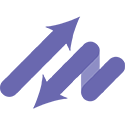
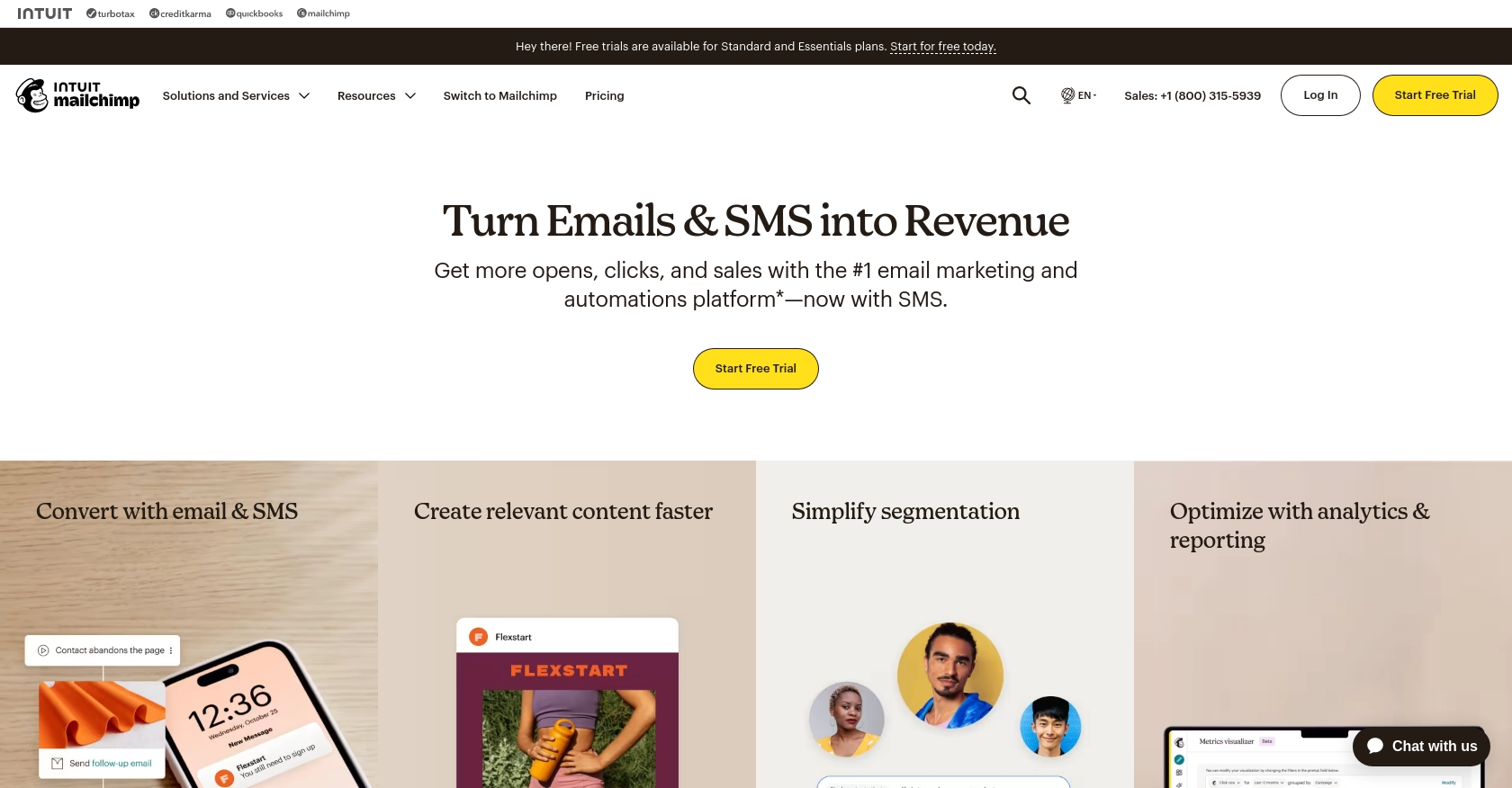
Introduction to Mailchimp API Integration
Mailchimp is a powerful marketing automation platform that helps businesses manage their email marketing campaigns, audience data, and analytics. With its robust suite of tools, Mailchimp enables companies to create personalized marketing strategies and engage with their audience effectively.
Developers often seek to integrate with Mailchimp's API to automate and enhance their marketing workflows. By connecting to the Mailchimp API, developers can programmatically manage audience lists, create and update members, and synchronize data across platforms. For example, a developer might use the Mailchimp API to automatically add new subscribers from a website signup form to a Mailchimp audience, ensuring seamless data integration and efficient audience management.
Setting Up Your Mailchimp Test Account for API Integration
Before diving into the Mailchimp API integration, you need to set up a Mailchimp account. This will allow you to test API calls and manage your audience data effectively. Follow these steps to create a test account and obtain the necessary credentials for API access.
Create a Mailchimp Account
If you don't have a Mailchimp account, start by signing up for a free account on the Mailchimp website. This account will serve as your sandbox environment for testing API interactions.
- Visit the Mailchimp website and click on the "Sign Up Free" button.
- Fill in the required information, including your email, username, and password.
- Follow the on-screen instructions to complete the account setup process.
Generate Your Mailchimp API Key
To authenticate your API requests, you'll need an API key. Here's how to generate one:
- Log in to your Mailchimp account.
- Navigate to the "Account" section by clicking on your profile name in the bottom left corner.
- Select "Extras" and then "API keys" from the dropdown menu.
- Click on "Create A Key" to generate a new API key.
- Copy the generated API key and store it securely, as you won't be able to view it again.
For more details, refer to the Mailchimp API Quick Start Guide.
Understanding Mailchimp OAuth Authentication
If your integration requires access to Mailchimp on behalf of other users, consider setting up OAuth 2 authentication. This method is more secure for multi-user applications.
For detailed instructions on implementing OAuth 2, visit the Mailchimp OAuth Guide.
Configure Your Mailchimp Audience
Before making API calls, ensure you have an audience set up in Mailchimp. This is where you'll manage your contacts and test API interactions.
- Navigate to the "Audience" tab in your Mailchimp dashboard.
- Create a new audience if you don't have one by clicking "Create Audience."
- Fill in the necessary details, such as audience name and default email settings.
With your Mailchimp account and API key ready, you're all set to start integrating with the Mailchimp API using JavaScript. In the next section, we'll explore how to make API calls to create members in your Mailchimp audience.
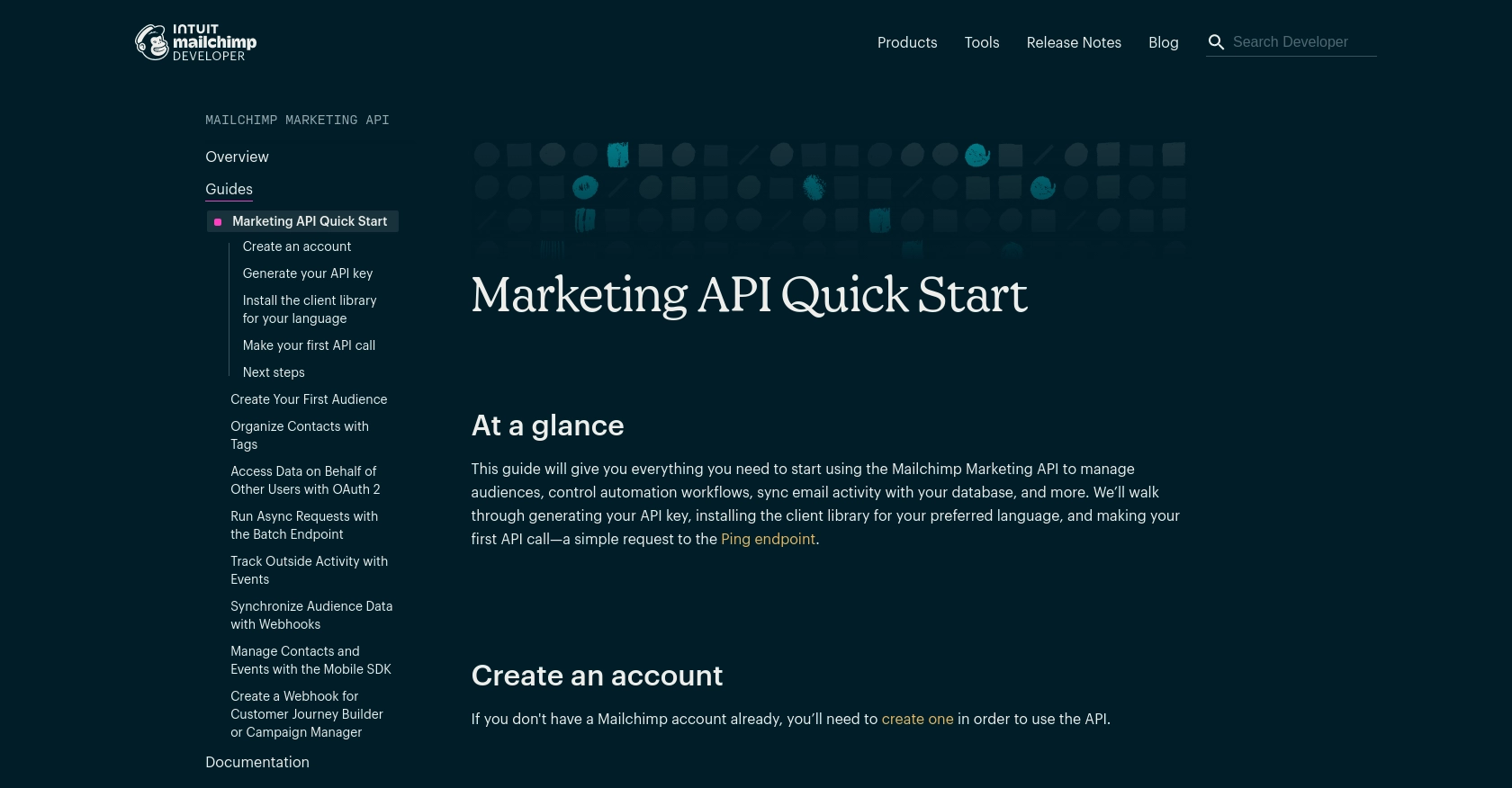
sbb-itb-96038d7
Making API Calls to Add Members to Mailchimp Audience Using JavaScript
In this section, we'll explore how to use JavaScript to interact with the Mailchimp API and add members to your audience. This process involves setting up your environment, writing the necessary code, and handling potential errors effectively.
Setting Up Your JavaScript Environment for Mailchimp API Integration
Before making API calls, ensure you have Node.js installed on your machine. Node.js allows you to run JavaScript code outside of a browser, making it ideal for server-side applications.
- Download and install Node.js from the official website.
- Verify the installation by running
node -v
andnpm -v
in your terminal.
Next, you'll need to install the axios
library, which simplifies making HTTP requests in JavaScript.
npm install axios
Writing JavaScript Code to Add Members to Mailchimp Audience
Now that your environment is set up, you can write the JavaScript code to add members to your Mailchimp audience. Create a new file named addMember.js
and add the following code:
const axios = require('axios');
// Replace with your Mailchimp API key and server prefix
const apiKey = 'YOUR_API_KEY';
const serverPrefix = 'YOUR_SERVER_PREFIX'; // e.g., us19
// Replace with your Mailchimp audience ID
const listId = 'YOUR_LIST_ID';
// Member data to be added
const memberData = {
email_address: 'example@example.com',
status: 'subscribed',
merge_fields: {
FNAME: 'John',
LNAME: 'Doe'
}
};
// Mailchimp API endpoint
const url = `https://${serverPrefix}.api.mailchimp.com/3.0/lists/${listId}/members`;
// Make the API call
axios.post(url, memberData, {
auth: {
username: 'anystring',
password: apiKey
}
})
.then(response => {
console.log('Member added successfully:', response.data);
})
.catch(error => {
console.error('Error adding member:', error.response.data);
});
Replace YOUR_API_KEY
, YOUR_SERVER_PREFIX
, and YOUR_LIST_ID
with your actual Mailchimp API key, server prefix, and audience ID.
Running the JavaScript Code and Verifying the API Call
To execute the code, run the following command in your terminal:
node addMember.js
If successful, you should see a confirmation message in the console. You can verify the new member in your Mailchimp dashboard under the "Audience" section.
Handling Errors and Understanding Mailchimp API Response Codes
When making API calls, it's crucial to handle errors gracefully. The Mailchimp API may return various status codes indicating success or failure:
- 200: Request was successful.
- 400: Bad request, often due to invalid input.
- 401: Unauthorized, check your API key and permissions.
- 403: Forbidden, you don't have permission to perform this action.
- 429: Too many requests, you've hit the rate limit.
For more details on error handling, refer to the Mailchimp API documentation.
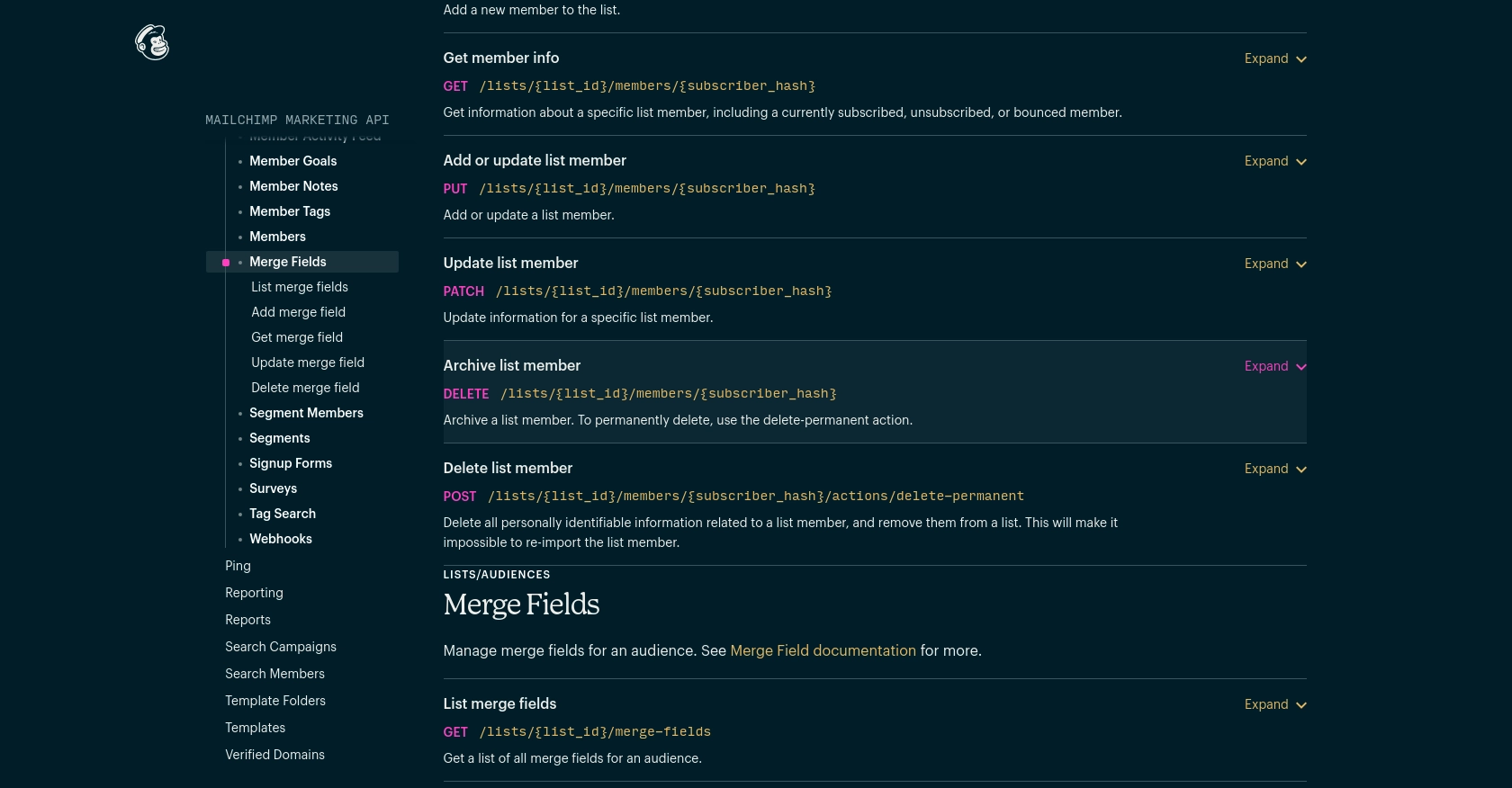
Conclusion and Best Practices for Mailchimp API Integration
Integrating with the Mailchimp API using JavaScript offers a powerful way to automate and enhance your marketing workflows. By following the steps outlined in this guide, you can efficiently add members to your Mailchimp audience and ensure seamless data synchronization across platforms.
Best Practices for Secure and Efficient Mailchimp API Usage
- Secure API Keys: Always store your Mailchimp API keys securely, similar to passwords. Avoid exposing them in client-side code to prevent unauthorized access.
- Handle Rate Limits: Be mindful of Mailchimp's API rate limits, which allow up to 10 simultaneous connections. Implement caching strategies for frequently accessed data to minimize API calls. For more details, refer to the Mailchimp API limits documentation.
- Data Standardization: Ensure consistent data formats when adding or updating members to maintain data integrity across your systems.
- Error Handling: Implement robust error handling to manage API response codes effectively, ensuring your application can gracefully handle failures.
Enhance Your Integration Strategy with Endgrate
While integrating with Mailchimp is a powerful step, managing multiple integrations can be complex and time-consuming. Endgrate offers a streamlined solution by providing a unified API endpoint that connects to various platforms, including Mailchimp. This allows you to build once for each use case, saving time and resources while delivering an intuitive integration experience for your customers.
Explore how Endgrate can simplify your integration strategy and let you focus on your core product by visiting Endgrate.
Read More
Ready to get started?