How to Create or Update Accounts with the Outreach API in Python
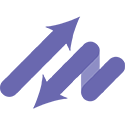
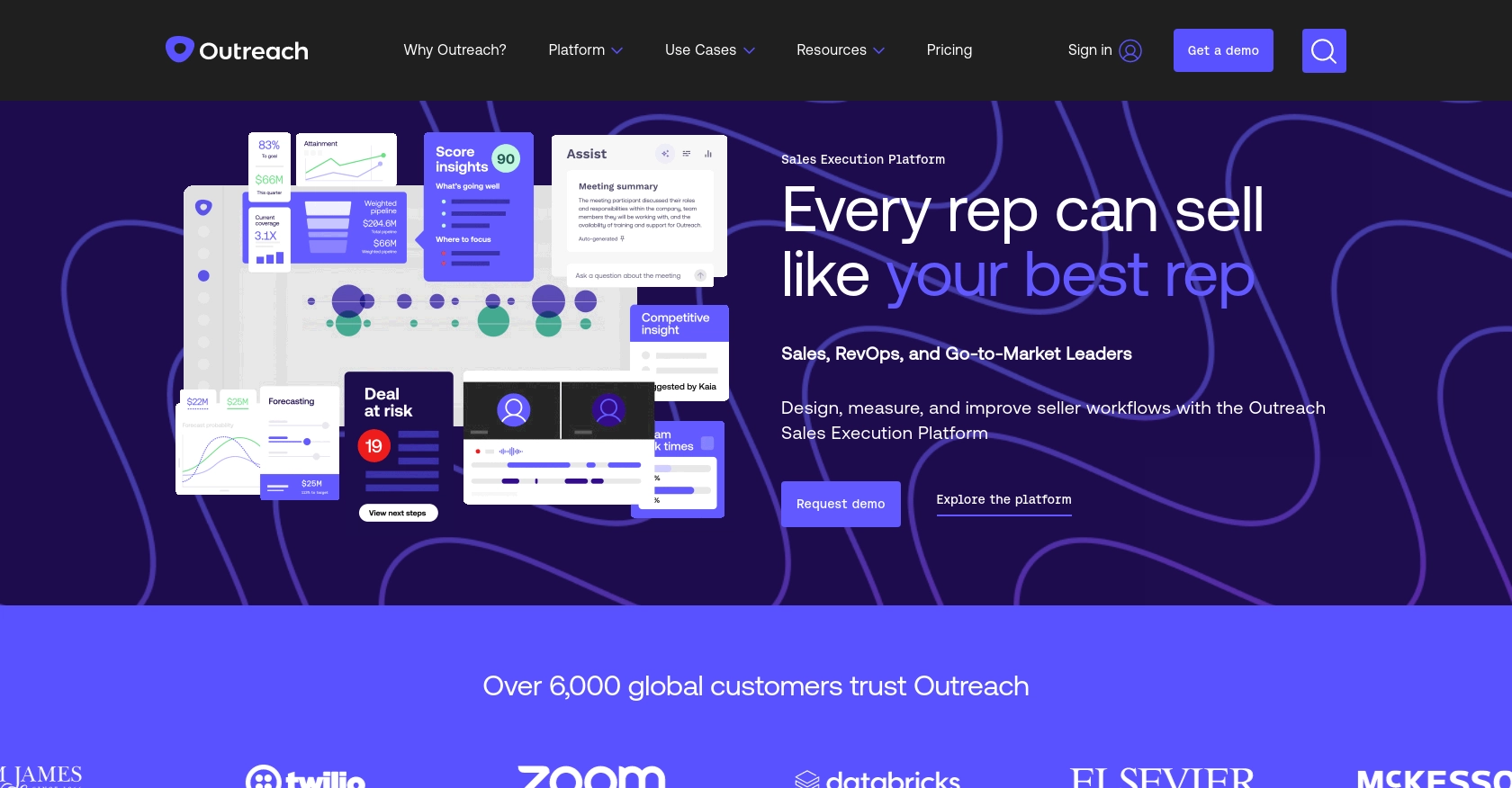
Introduction to Outreach API for Account Management
Outreach is a powerful sales engagement platform that helps businesses streamline their sales processes and improve productivity. With its robust set of tools, Outreach enables sales teams to manage customer interactions, automate workflows, and track performance metrics.
Integrating with the Outreach API allows developers to create or update account information programmatically, enhancing the efficiency of sales operations. For example, a developer might use the Outreach API to automatically update account details based on changes in an external CRM system, ensuring that sales teams always have the most current information at their fingertips.
Setting Up Your Outreach Test/Sandbox Account for API Integration
Before you can start integrating with the Outreach API, you'll need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting live data. Outreach provides a sandbox environment that mimics the production environment, making it ideal for development and testing purposes.
Creating an Outreach Sandbox Account
If you don't already have an Outreach account, you can sign up for a free trial or request access to a sandbox account through the Outreach website. Follow these steps to get started:
- Visit the Outreach website and navigate to the sign-up page.
- Fill out the required information to create your account.
- Once your account is created, log in to the Outreach dashboard.
Setting Up OAuth for Outreach API Access
The Outreach API uses OAuth 2.0 for authentication. You'll need to create an Outreach app to obtain the necessary credentials. Follow these steps to set up OAuth:
- Log in to your Outreach account and navigate to the "Settings" section.
- Under "Integrations," select "API Access" and click on "Create New App."
- Fill in the required details for your app, including the app name and description.
- Specify one or more redirect URIs where Outreach will send the authorization code after user consent.
- Select the OAuth scopes that your application will require. Ensure you choose the appropriate scopes for account management.
- Save your app settings to generate the client ID and client secret. Note that the client secret will only be displayed once, so make sure to store it securely.
Obtaining OAuth Tokens for API Authentication
Once your app is set up, you'll need to obtain an access token to authenticate API requests. Here's how to do it:
- Redirect users to the following URL to request an authorization code:
- After user consent, Outreach will redirect to your specified URI with an authorization code.
- Exchange the authorization code for an access token by making a POST request:
- Store the access token securely. It will be used in the Authorization header for API requests.
https://api.outreach.io/oauth/authorize?client_id=<Your_Client_ID>&redirect_uri=<Your_Redirect_URI>&response_type=code&scope=<Your_Scopes>
curl https://api.outreach.io/oauth/token \
-X POST \
-d client_id=<Your_Client_ID> \
-d client_secret=<Your_Client_Secret> \
-d redirect_uri=<Your_Redirect_URI> \
-d grant_type=authorization_code \
-d code=<Authorization_Code>
For more detailed information, refer to the Outreach OAuth documentation: Outreach OAuth Documentation.
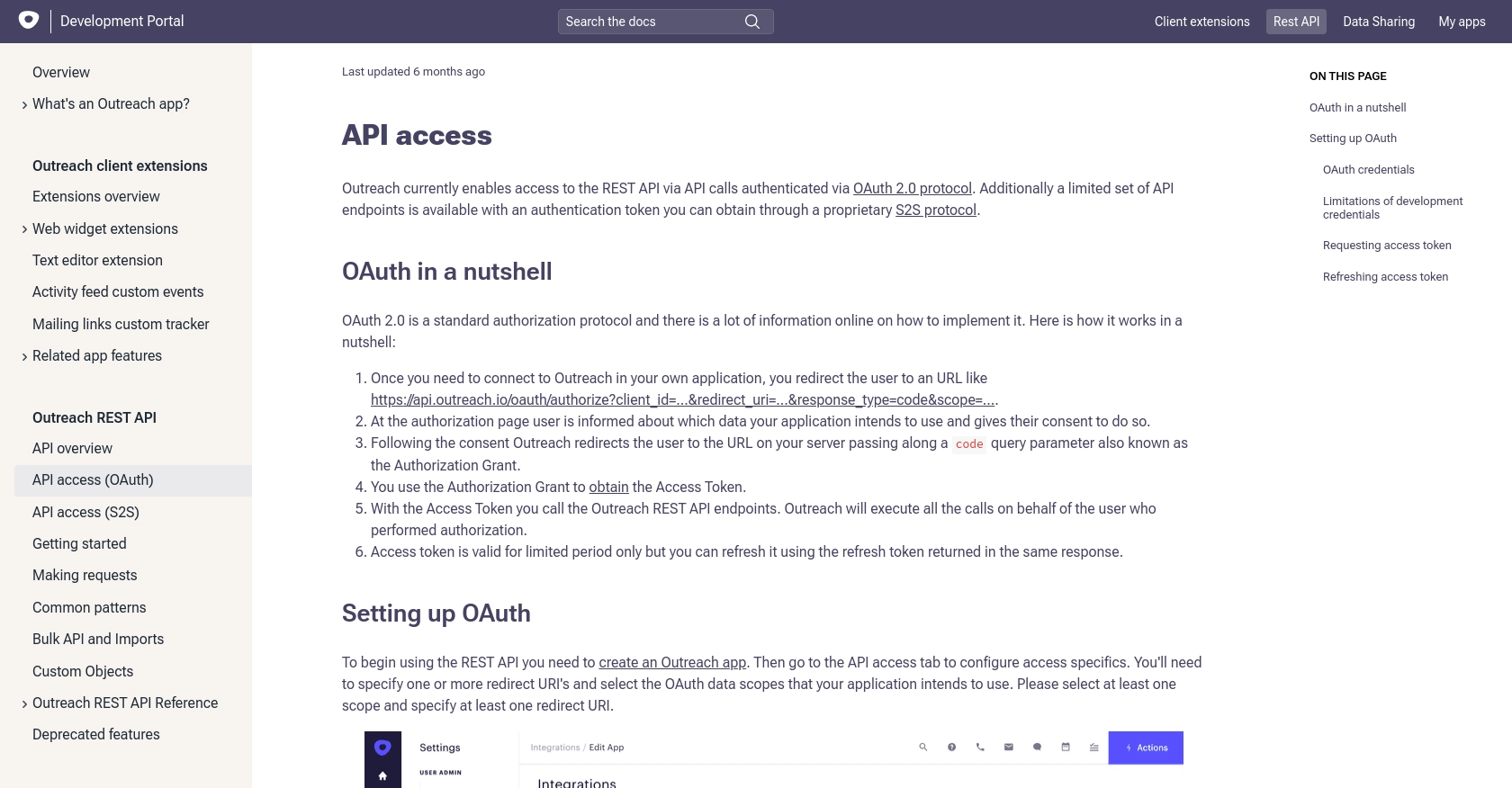
sbb-itb-96038d7
Making API Calls to Create or Update Accounts with Outreach API in Python
To interact with the Outreach API for creating or updating accounts, you'll need to use Python, a versatile programming language widely used for web development and automation. Ensure you have Python 3.x installed on your machine, along with the necessary dependencies.
Setting Up Your Python Environment for Outreach API Integration
Before making API calls, set up your Python environment by installing the required libraries. You'll need the requests
library to handle HTTP requests. Install it using pip:
pip install requests
Creating an Account with Outreach API Using Python
To create a new account in Outreach, you'll need to make a POST request to the Outreach API endpoint. Here's a step-by-step guide:
- Create a new Python file named
create_outreach_account.py
. - Add the following code to the file:
import requests
# Set the API endpoint
url = "https://api.outreach.io/api/v2/accounts"
# Set the request headers
headers = {
"Authorization": "Bearer Your_Access_Token",
"Content-Type": "application/vnd.api+json"
}
# Define the account data
account_data = {
"data": {
"type": "account",
"attributes": {
"name": "Acme Corporation",
"domain": "acme.com",
"industry": "Manufacturing"
}
}
}
# Make the POST request to create the account
response = requests.post(url, json=account_data, headers=headers)
# Check if the request was successful
if response.status_code == 201:
print("Account created successfully!")
else:
print("Failed to create account:", response.json())
Replace Your_Access_Token
with the access token obtained from the OAuth process. Run the script using the command:
python create_outreach_account.py
Upon successful execution, the account should be created in your Outreach sandbox environment.
Updating an Existing Account with Outreach API Using Python
To update an existing account, you'll need to make a PATCH request. Follow these steps:
- Create a new Python file named
update_outreach_account.py
. - Add the following code to the file:
import requests
# Set the API endpoint with the account ID
account_id = "123456"
url = f"https://api.outreach.io/api/v2/accounts/{account_id}"
# Set the request headers
headers = {
"Authorization": "Bearer Your_Access_Token",
"Content-Type": "application/vnd.api+json"
}
# Define the updated account data
updated_data = {
"data": {
"type": "account",
"id": account_id,
"attributes": {
"industry": "Technology"
}
}
}
# Make the PATCH request to update the account
response = requests.patch(url, json=updated_data, headers=headers)
# Check if the request was successful
if response.status_code == 200:
print("Account updated successfully!")
else:
print("Failed to update account:", response.json())
Replace Your_Access_Token
and account_id
with the appropriate values. Run the script using the command:
python update_outreach_account.py
The account should be updated in your Outreach sandbox environment.
Handling Errors and Verifying API Requests
When making API calls, it's crucial to handle potential errors. The Outreach API may return various HTTP status codes indicating success or failure. For example, a 201 status code indicates successful creation, while a 422 status code indicates validation errors. Always check the response and handle errors appropriately.
To verify that your requests have succeeded, log in to your Outreach sandbox account and check the accounts section to see the created or updated accounts.
For more detailed information on error codes, refer to the Outreach API documentation: Outreach API Reference.
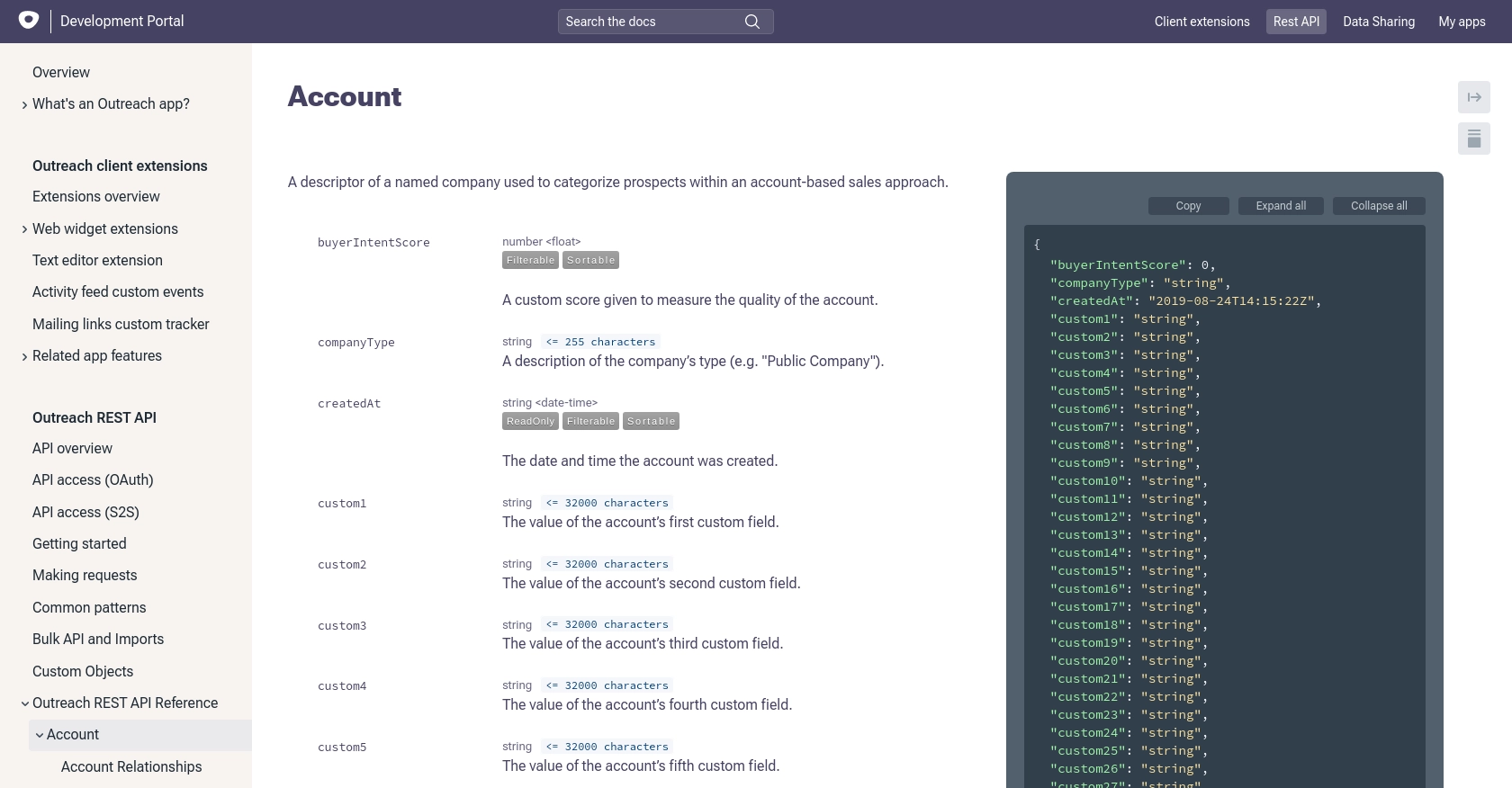
Best Practices for Using Outreach API for Account Management
When working with the Outreach API to manage accounts, it's essential to follow best practices to ensure security, efficiency, and reliability. Here are some key recommendations:
- Securely Store Credentials: Always store your OAuth tokens securely. Avoid hardcoding them in your scripts and consider using environment variables or secure vaults.
- Handle Rate Limiting: The Outreach API has a rate limit of 10,000 requests per hour. Monitor your API usage and implement retry logic with exponential backoff to handle 429 status codes gracefully. For more details, refer to the Outreach API Rate Limiting Documentation.
- Use the Latest Refresh Token: Refresh tokens are short-lived. Always use the most recent refresh token to obtain new access tokens and avoid unnecessary token requests.
- Validate API Responses: Always check the response status codes and handle errors appropriately. Implement logging to track API interactions and troubleshoot issues effectively.
- Optimize Data Handling: When creating or updating accounts, ensure that the data is correctly formatted and validated to prevent errors and maintain data integrity.
Enhancing Integration Efficiency with Endgrate
Building and maintaining integrations can be time-consuming and complex. Endgrate offers a streamlined solution for managing multiple integrations, including Outreach, through a single API endpoint. By leveraging Endgrate, you can:
- Save Time and Resources: Focus on your core product development while outsourcing integration complexities to Endgrate.
- Build Once, Use Everywhere: Develop integration logic once and apply it across various platforms, reducing redundant efforts.
- Improve Customer Experience: Provide your users with a seamless and intuitive integration experience, enhancing satisfaction and engagement.
Explore how Endgrate can simplify your integration processes by visiting Endgrate's website and discover the benefits of a unified integration approach.
Read More
Ready to get started?