Using the CSV API to Export Data as CSV (with PHP examples)
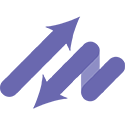
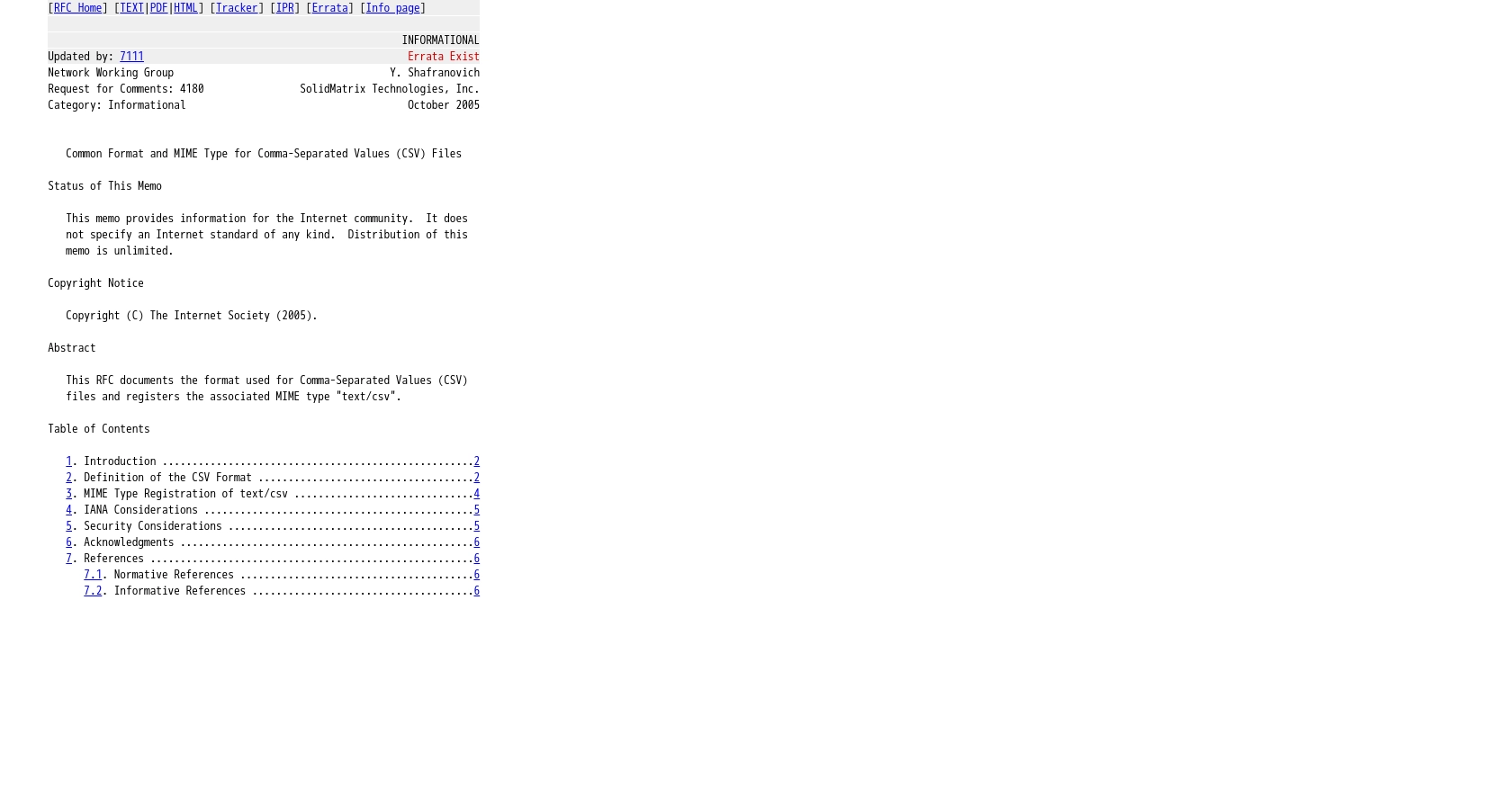
Introduction to CSV Data Integration
CSV (Comma-Separated Values) is a widely-used format for data exchange, allowing for easy import and export of data between different systems. It is especially popular in business environments where data needs to be shared across various platforms and applications.
For developers working with B2B SaaS products, integrating with CSV data can streamline processes such as data migration, reporting, and analytics. By exporting data as CSV, developers can facilitate seamless data transfer, enabling businesses to leverage their data more effectively.
In this article, we'll explore how to use the CSV API to export data as CSV files, with practical examples in PHP. This integration can be particularly useful for automating data exports, ensuring that data is readily available for analysis or sharing with stakeholders.
Setting Up Your CSV Test/Sandbox Account for Data Export
Before diving into exporting data as CSV using the CSV API, it's essential to set up a test or sandbox account. This environment allows developers to experiment with API calls without affecting live data, ensuring a smooth integration process.
Creating a CSV Test Account
To begin, you'll need access to a CSV integration platform that supports sandbox environments. If your integration provider offers a free trial or sandbox account, sign up to gain access to the necessary tools and features.
- Visit the integration provider's website and navigate to the sign-up page.
- Register for a free trial or sandbox account by providing the required information.
- Once registered, log in to your account to access the sandbox environment.
Generating API Credentials for CSV Authentication
The CSV integration uses a custom authentication method, which involves uploading and downloading CSV files in chunks. Follow these steps to set up your authentication:
- Navigate to the API settings section within your sandbox account.
- Locate the option to generate API credentials for CSV access.
- Follow the prompts to create your API key or token, ensuring you store it securely for future use.
Note: The CSV integration requires a custom frontend for handling CSV uploads and downloads. Ensure you have the necessary libraries and tools to facilitate this process.
Preparing Your Environment for CSV API Calls
With your sandbox account and API credentials ready, you can now prepare your development environment. This involves setting up your PHP environment to interact with the CSV API:
- Ensure you have PHP installed on your machine. The recommended version is PHP 7.4 or later.
- Install any necessary PHP libraries for handling CSV files, such as
fgetcsv
andfputcsv
. - Set up a local or cloud-based server to test your API calls in a controlled environment.
With these preparations complete, you're ready to start making API calls to export data as CSV files using PHP. In the next section, we'll explore how to perform these actions with practical code examples.
Making CSV API Calls to Export Data Using PHP
With your environment set up, it's time to dive into making API calls to export data as CSV files using PHP. This section will guide you through the process of interacting with the CSV API, ensuring you can efficiently export data for your B2B SaaS applications.
Setting Up PHP for CSV API Integration
Before making API calls, ensure your PHP environment is configured correctly. You'll need to have the necessary libraries and tools to handle CSV data:
- Verify that PHP 7.4 or later is installed on your system.
- Ensure the
fgetcsv
andfputcsv
functions are available for reading and writing CSV files. - Set up a local server or use a cloud-based solution to test your API interactions.
Example Code for Exporting Data as CSV
Below is a PHP example demonstrating how to make an API call to export data as a CSV file. This example assumes you have already obtained the necessary API credentials and set up your environment:
<?php
// Define the API endpoint and credentials
$apiUrl = "https://api.yourprovider.com/export";
$apiKey = "your_api_key_here";
// Initialize a cURL session
$ch = curl_init($apiUrl);
// Set cURL options
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
"Authorization: Bearer $apiKey",
"Content-Type: application/json"
]);
// Execute the API call
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo "cURL error: " . curl_error($ch);
} else {
// Save the CSV data to a file
$csvFile = fopen("exported_data.csv", "w");
fwrite($csvFile, $response);
fclose($csvFile);
echo "Data exported successfully!";
}
// Close the cURL session
curl_close($ch);
?>
In this code, we initiate a cURL session to make a GET request to the CSV API endpoint. The response, which contains the CSV data, is saved to a file named exported_data.csv
. Ensure you replace your_api_key_here
with your actual API key.
Verifying Successful CSV Data Export
After executing the API call, verify the success of the operation by checking the contents of the exported_data.csv
file. The file should contain the expected data, formatted as CSV. If the file is empty or contains errors, review the API response and error messages for troubleshooting.
Handling Errors and Common Issues
When working with API calls, it's crucial to handle potential errors gracefully. Here are some common issues and how to address them:
- Authentication Errors: Ensure your API key is correct and has the necessary permissions.
- Network Issues: Check your internet connection and the API endpoint URL.
- Data Format Errors: Verify that the CSV data is correctly formatted and that your PHP code handles it appropriately.
By following these guidelines, you can effectively manage errors and ensure a smooth integration process.
Conclusion and Best Practices for CSV Data Export Integration
Exporting data as CSV using the CSV API with PHP can significantly enhance your B2B SaaS application's data handling capabilities. By following the steps outlined in this article, you can automate data exports, ensuring that your data is readily available for analysis and sharing.
Best Practices for Secure and Efficient CSV Data Handling
- Secure Storage of API Credentials: Always store your API keys and tokens securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handling Rate Limits: Be mindful of any rate limits imposed by the CSV API. Implement retry logic and exponential backoff strategies to handle rate limit errors gracefully.
- Data Validation and Transformation: Before exporting data, ensure it is validated and transformed to meet the required CSV format. This helps prevent errors and ensures data consistency.
Enhancing Integration Capabilities with Endgrate
While integrating CSV data export is a powerful feature, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint for various platforms and services, including CSV integrations.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development. Build once for each use case and enjoy an intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration processes by visiting Endgrate today.
Read More
Ready to get started?