How to Get Users with the Pipedrive API in Javascript
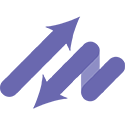
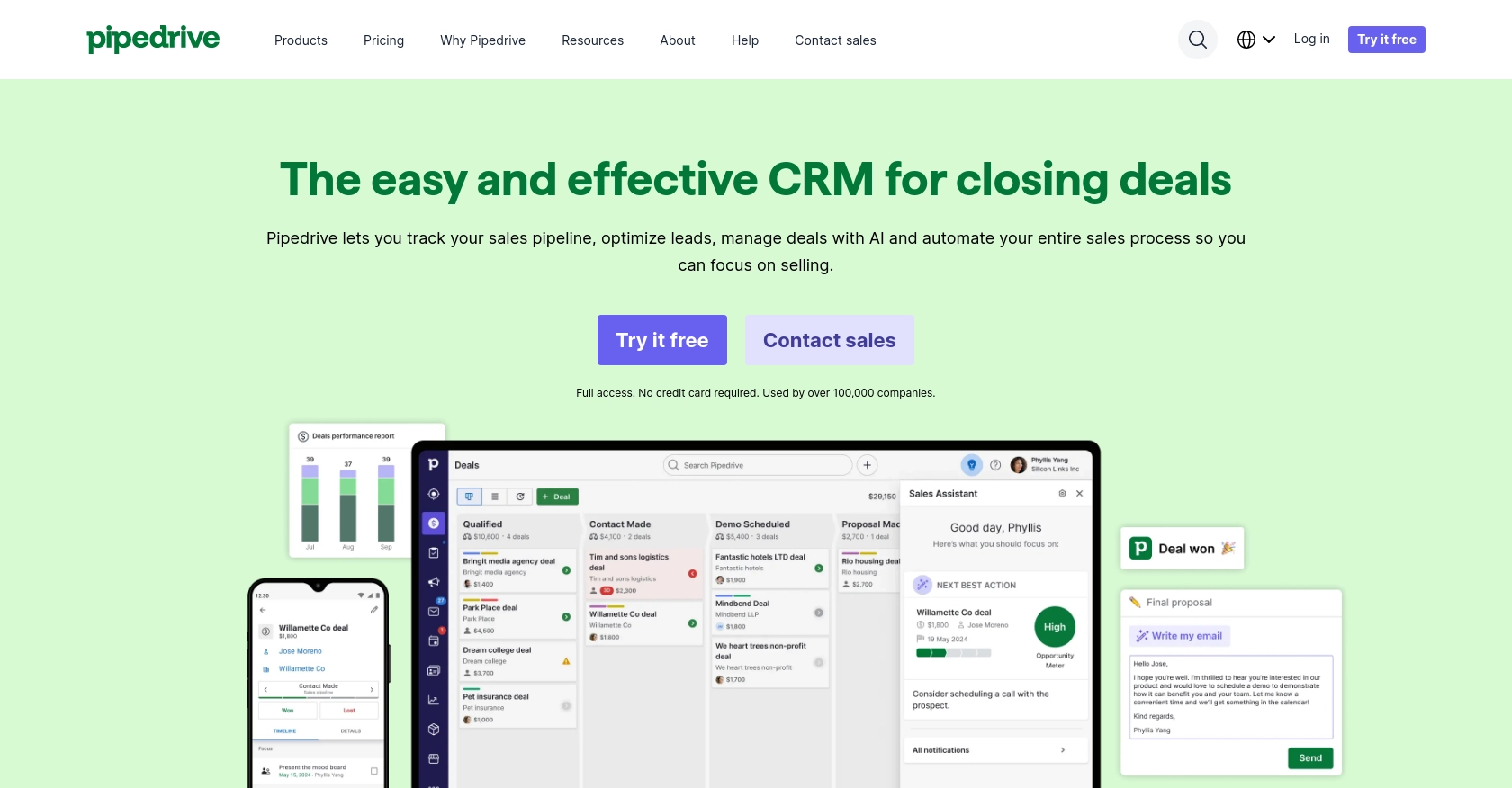
Introduction to Pipedrive API Integration
Pipedrive is a powerful sales CRM platform designed to help businesses manage their sales processes more effectively. With its intuitive interface and robust features, Pipedrive enables sales teams to track leads, manage deals, and automate workflows, making it a popular choice for companies aiming to enhance their sales efficiency.
Integrating with Pipedrive's API allows developers to access and manipulate data within the CRM, facilitating seamless integration with other business tools. For example, a developer might use the Pipedrive API to retrieve user data, enabling the synchronization of user information across multiple platforms, thereby ensuring consistency and accuracy in user management.
Setting Up Your Pipedrive Developer Sandbox Account
Before you can start integrating with the Pipedrive API, you need to set up a developer sandbox account. This account provides a risk-free environment for testing and development, allowing you to experiment with the Pipedrive API without affecting live data.
Creating a Pipedrive Developer Sandbox Account
To create a sandbox account, follow these steps:
- Visit the Pipedrive Developer Sandbox Account page.
- Fill out the form to request access to a sandbox account. This account is similar to a regular Pipedrive company account but is limited to five seats by default.
- Once your account is set up, you can import sample data to familiarize yourself with the Pipedrive interface. To do this, navigate to “...” (More) > Import data > From a spreadsheet in the Pipedrive web app.
Creating a Pipedrive App for OAuth Authentication
Since the Pipedrive API uses OAuth 2.0 for authentication, you'll need to create an app to obtain the necessary credentials:
- Log in to your Pipedrive sandbox account and navigate to the Developer Hub.
- Register your app by providing the required details, such as the app name and description.
- Once registered, you will receive a client ID and client secret. These credentials are essential for implementing the OAuth flow.
- Ensure your app has the proper scopes and permissions to access user data. You can manage these settings in the Developer Hub.
With your sandbox account and app set up, you're ready to start making API calls to Pipedrive. In the next section, we'll explore how to retrieve user data using JavaScript.
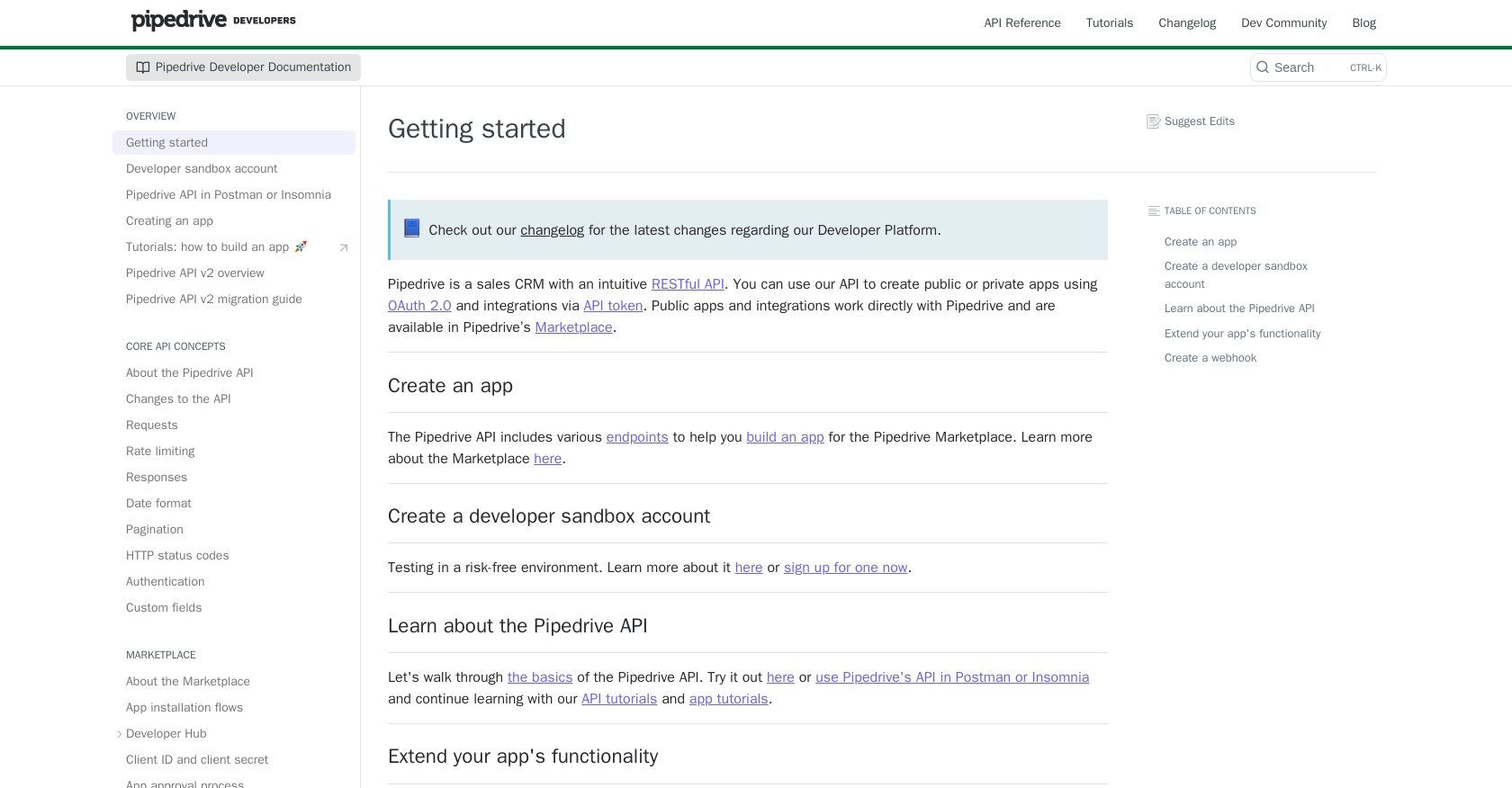
sbb-itb-96038d7
Making API Calls to Retrieve Users with Pipedrive API in JavaScript
To interact with the Pipedrive API using JavaScript, you'll need to set up your environment and write code that can authenticate and make requests to the API. This section will guide you through the process of retrieving user data from Pipedrive using JavaScript.
Setting Up Your JavaScript Environment for Pipedrive API
Before making API calls, ensure you have Node.js installed on your machine. You will also need the axios
library to handle HTTP requests. Install it using the following command:
npm install axios
Writing JavaScript Code to Fetch Users from Pipedrive
Once your environment is ready, you can write the JavaScript code to retrieve users from Pipedrive. Here's a step-by-step guide:
const axios = require('axios');
// Define the API endpoint and headers
const endpoint = 'https://api.pipedrive.com/v1/users';
const headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN'
};
// Function to get users
async function getUsers() {
try {
const response = await axios.get(endpoint, { headers });
const users = response.data.data;
// Display user information
users.forEach(user => {
console.log(`ID: ${user.id}, Name: ${user.name}, Email: ${user.email}`);
});
} catch (error) {
console.error('Error fetching users:', error.response ? error.response.data : error.message);
}
}
// Call the function
getUsers();
Replace YOUR_ACCESS_TOKEN
with the access token obtained from your OAuth setup. This code uses the axios
library to send a GET request to the Pipedrive API endpoint for users. It then logs the user ID, name, and email to the console.
Verifying API Call Success in Pipedrive Sandbox
After running the script, verify the successful retrieval of user data by checking the console output. The data should match the users in your Pipedrive sandbox account. If the request fails, the error message will provide insights into what went wrong.
Handling Errors and Understanding Pipedrive API Response Codes
When making API calls, it's crucial to handle potential errors. The Pipedrive API may return various HTTP status codes, such as:
- 200 OK: Request was successful.
- 401 Unauthorized: Invalid or missing access token.
- 429 Too Many Requests: Rate limit exceeded.
For more detailed information on error codes, refer to the Pipedrive API documentation.
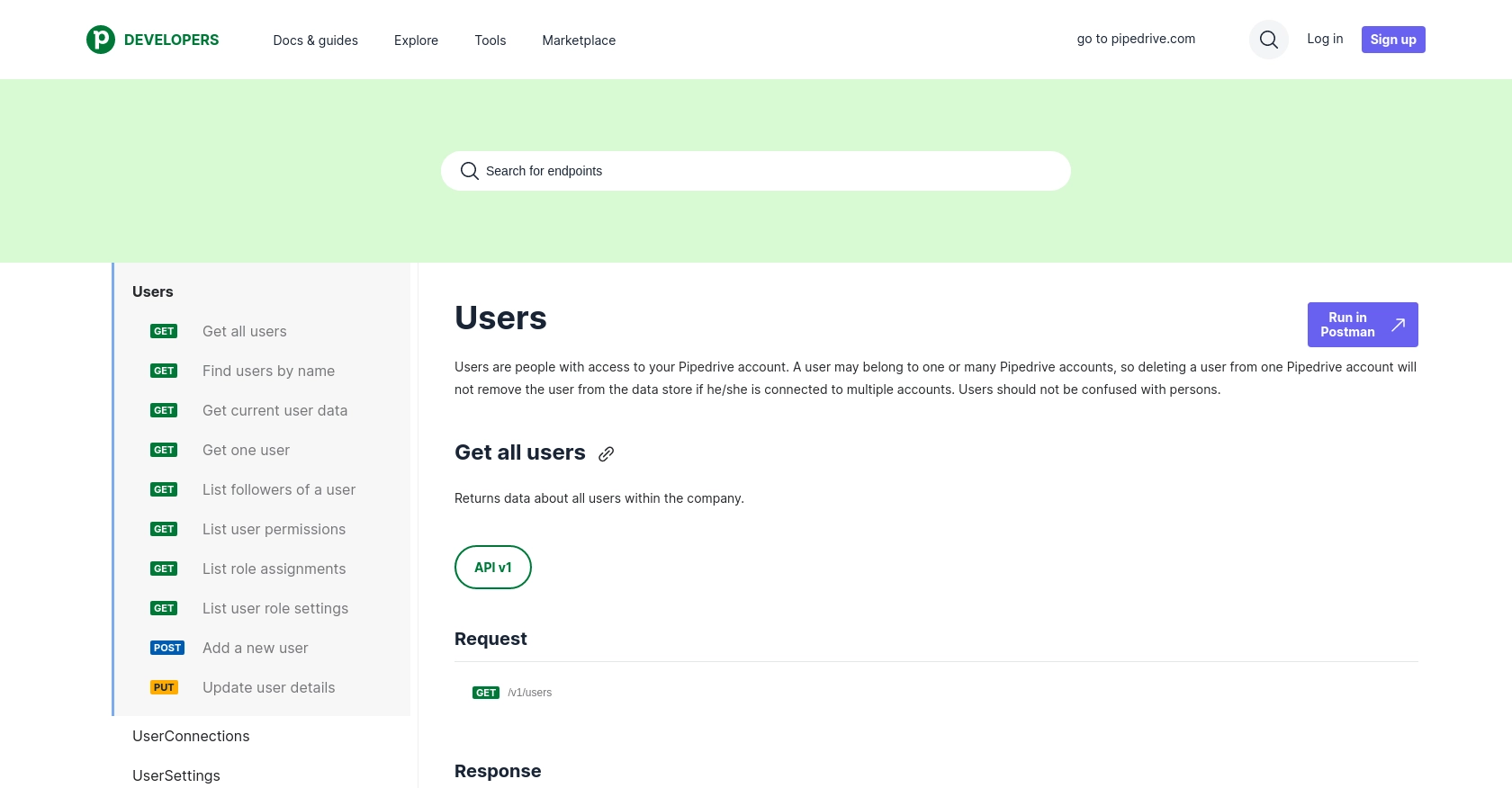
Conclusion and Best Practices for Using Pipedrive API in JavaScript
Integrating with the Pipedrive API using JavaScript provides a powerful way to manage user data and enhance your CRM capabilities. By following the steps outlined in this guide, you can efficiently retrieve user information and ensure seamless synchronization across platforms.
Best Practices for Storing User Credentials
- Always store sensitive information like access tokens securely, using environment variables or secure vaults.
- Avoid hardcoding credentials directly in your codebase to prevent unauthorized access.
Handling Pipedrive API Rate Limits
Pipedrive enforces rate limits to ensure fair usage of its API. For OAuth apps, the rate limit is up to 480 requests per 2 seconds per access token, depending on your plan. To avoid hitting these limits:
- Implement efficient data fetching strategies, such as using webhooks to receive updates instead of polling.
- Consider upgrading your plan if you frequently reach the rate limits.
For more details, refer to the Pipedrive API rate limiting documentation.
Data Transformation and Standardization
When integrating with multiple platforms, ensure that data fields are transformed and standardized to maintain consistency. This practice helps in reducing errors and improving data integrity across systems.
Call to Action: Simplify Integrations with Endgrate
Building and maintaining integrations can be complex and time-consuming. With Endgrate, you can streamline this process by leveraging a unified API endpoint that connects to multiple platforms, including Pipedrive. Focus on your core product while Endgrate handles the intricacies of integration, saving you time and resources. Explore how Endgrate can enhance your integration strategy by visiting Endgrate today.
Read More
- https://endgrate.com/provider/pipedrive
- https://pipedrive.readme.io/docs/getting-started
- https://pipedrive.readme.io/docs/developer-sandbox-account
- https://pipedrive.readme.io/docs/marketplace-creating-a-proper-app
- https://pipedrive.readme.io/docs/core-api-concepts-rate-limiting
- https://pipedrive.readme.io/docs/core-api-concepts-pagination
- https://pipedrive.readme.io/docs/core-api-concepts-http-status-codes
- https://pipedrive.readme.io/docs/core-api-concepts-custom-fields
- https://developers.pipedrive.com/docs/api/v1/Users
Ready to get started?