Using the Endear API to Create Customers in PHP
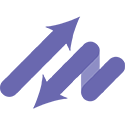
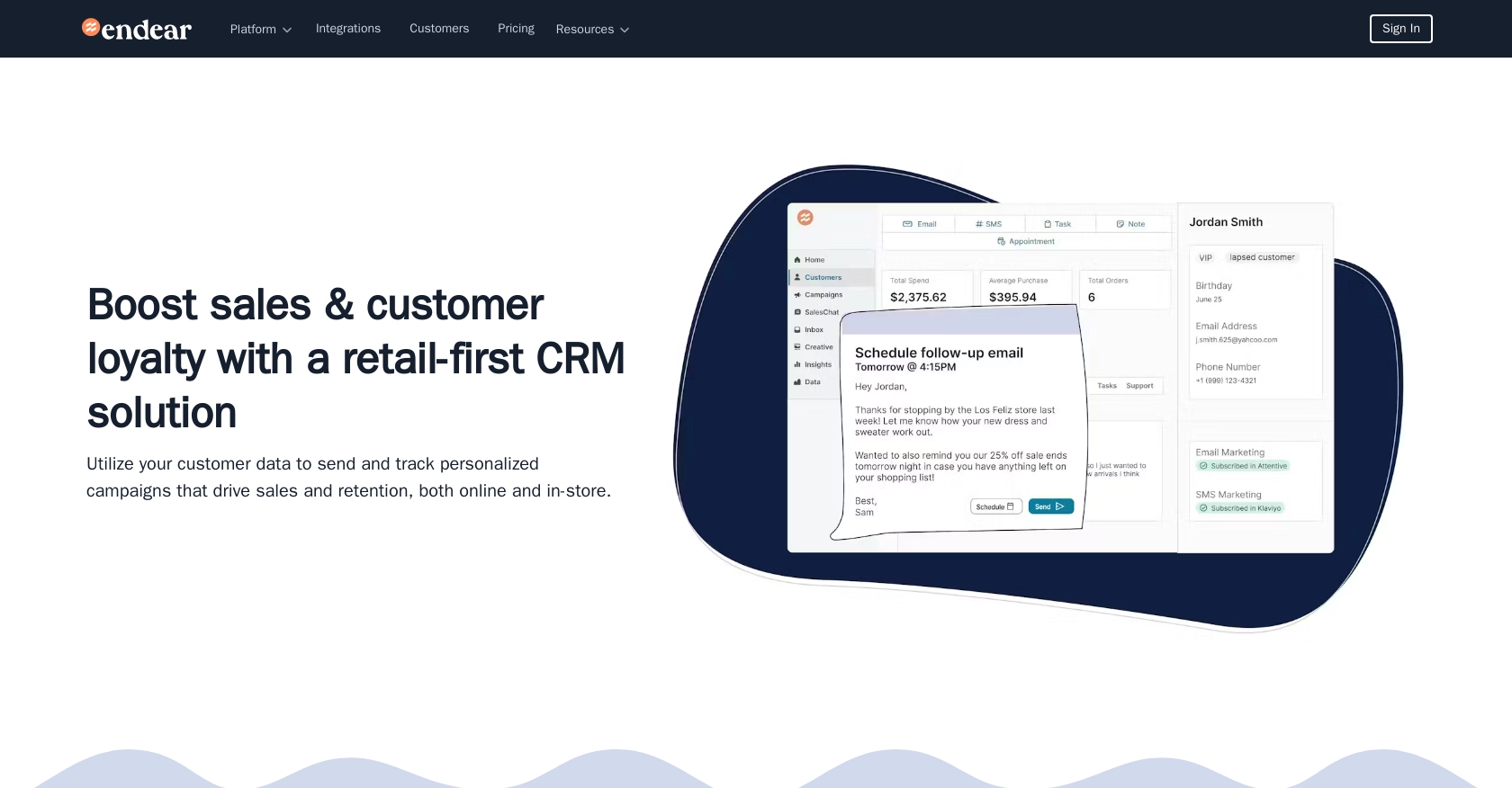
Introduction to Endear API Integration
Endear is a powerful CRM platform designed to enhance customer relationship management for businesses by providing real-time data processing and integration capabilities. Built on Google Cloud Platform, Endear offers a scalable solution for managing customer data across various sources.
Developers may want to integrate with Endear's API to streamline customer data management and improve marketing strategies. For example, by using the Endear API, developers can automate the creation of customer profiles, ensuring that all customer interactions are efficiently recorded and accessible for personalized marketing campaigns.
Setting Up Your Endear Test Account for API Integration
Before you can start integrating with the Endear API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data. Follow these steps to create your test account and obtain the necessary API key for authentication.
Creating an Endear Test Account
If you don't already have an Endear account, you can sign up for a free trial on the Endear website. This will give you access to the platform's features and allow you to explore its capabilities.
- Visit the Endear website and click on the "Sign Up" button.
- Fill out the registration form with your details and submit it to create your account.
- Once your account is created, log in to access the Endear dashboard.
Generating an API Key for Endear Integration
To interact with the Endear API, you'll need to generate an API key. This key will be used to authenticate your requests.
- Navigate to the "Settings" section in your Endear dashboard.
- Click on "Integrations" and then select "Add Integration."
- Choose "API" from the options provided.
- Fill in the required details and submit to generate your API key.
- Make sure to copy and securely store your API key, as you'll need it for authentication.
For more detailed information, you can refer to the Endear Authentication Documentation.
Authenticating API Requests with Your API Key
Once you have your API key, you can authenticate your API requests by including it in the request headers. Here's how you can do it using PHP:
// Set the API endpoint and headers
$endpoint = "https://api.endearhq.com/graphql";
$headers = [
"Content-Type: application/json",
"X-Endear-Api-Key: YOUR_API_KEY"
];
// Initialize a cURL session
$ch = curl_init($endpoint);
// Set cURL options
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Execute the request
$response = curl_exec($ch);
// Close the cURL session
curl_close($ch);
// Output the response
echo $response;
Replace YOUR_API_KEY
with the API key you generated earlier. This example demonstrates how to set up a basic POST request to the Endear API using PHP.
For further details on API authentication, visit the Endear Authentication Documentation.
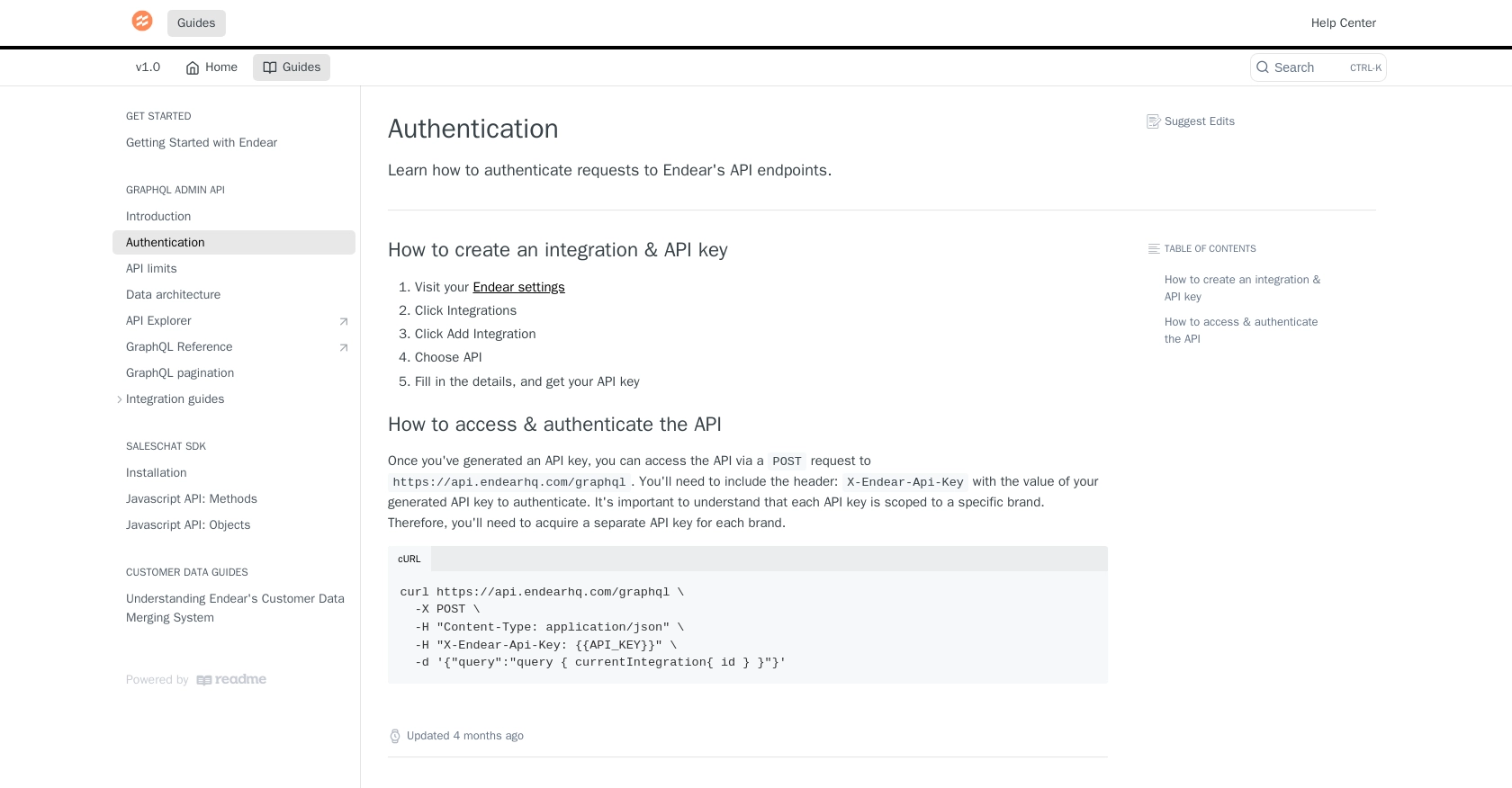
sbb-itb-96038d7
Making API Calls to Endear for Customer Creation Using PHP
To effectively interact with the Endear API and create customer profiles, you'll need to set up your PHP environment and understand the necessary steps for making API calls. This section will guide you through the process of configuring your PHP setup, writing the code to create customers, and handling potential errors.
Setting Up Your PHP Environment for Endear API Integration
Before making API calls, ensure your PHP environment is properly configured. You'll need PHP version 7.4 or higher and the cURL extension enabled. If you haven't already, you can install cURL using the following command:
sudo apt-get install php-curl
Once cURL is installed, you can proceed with writing the PHP code to interact with the Endear API.
Writing PHP Code to Create Customers in Endear
To create a customer in Endear, you'll need to make a POST request to the API endpoint with the appropriate headers and payload. Below is a sample PHP script to achieve this:
// Set the API endpoint and headers
$endpoint = "https://api.endearhq.com/graphql";
$headers = [
"Content-Type: application/json",
"X-Endear-Api-Key: YOUR_API_KEY"
];
// Define the customer data
$customerData = [
"query" => "mutation {
createCustomer(input: {
email: \"example@domain.com\",
firstName: \"John\",
lastName: \"Doe\"
}) {
customer {
id
email
}
}
}"
];
// Initialize a cURL session
$ch = curl_init($endpoint);
// Set cURL options
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($customerData));
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Execute the request and capture the response
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Request Error:' . curl_error($ch);
} else {
// Decode the response
$responseData = json_decode($response, true);
if (isset($responseData['data']['createCustomer']['customer'])) {
echo "Customer Created Successfully: " . $responseData['data']['createCustomer']['customer']['email'];
} else {
echo "Failed to Create Customer";
}
}
// Close the cURL session
curl_close($ch);
Replace YOUR_API_KEY
with the API key you generated earlier. This script demonstrates how to send a GraphQL mutation to create a customer in Endear.
Verifying Successful API Requests and Handling Errors
After executing the API call, it's crucial to verify the success of the request. You can check the response data to confirm that the customer was created successfully. If the request fails, handle errors gracefully by checking for error messages in the response or using curl_errno()
to identify issues with the request.
Endear's API has a rate limit of 120 requests per minute. If you exceed this limit, you'll receive an HTTP 429 error. To avoid this, implement rate limiting strategies in your application. For more information, refer to the Endear Rate Limits Documentation.
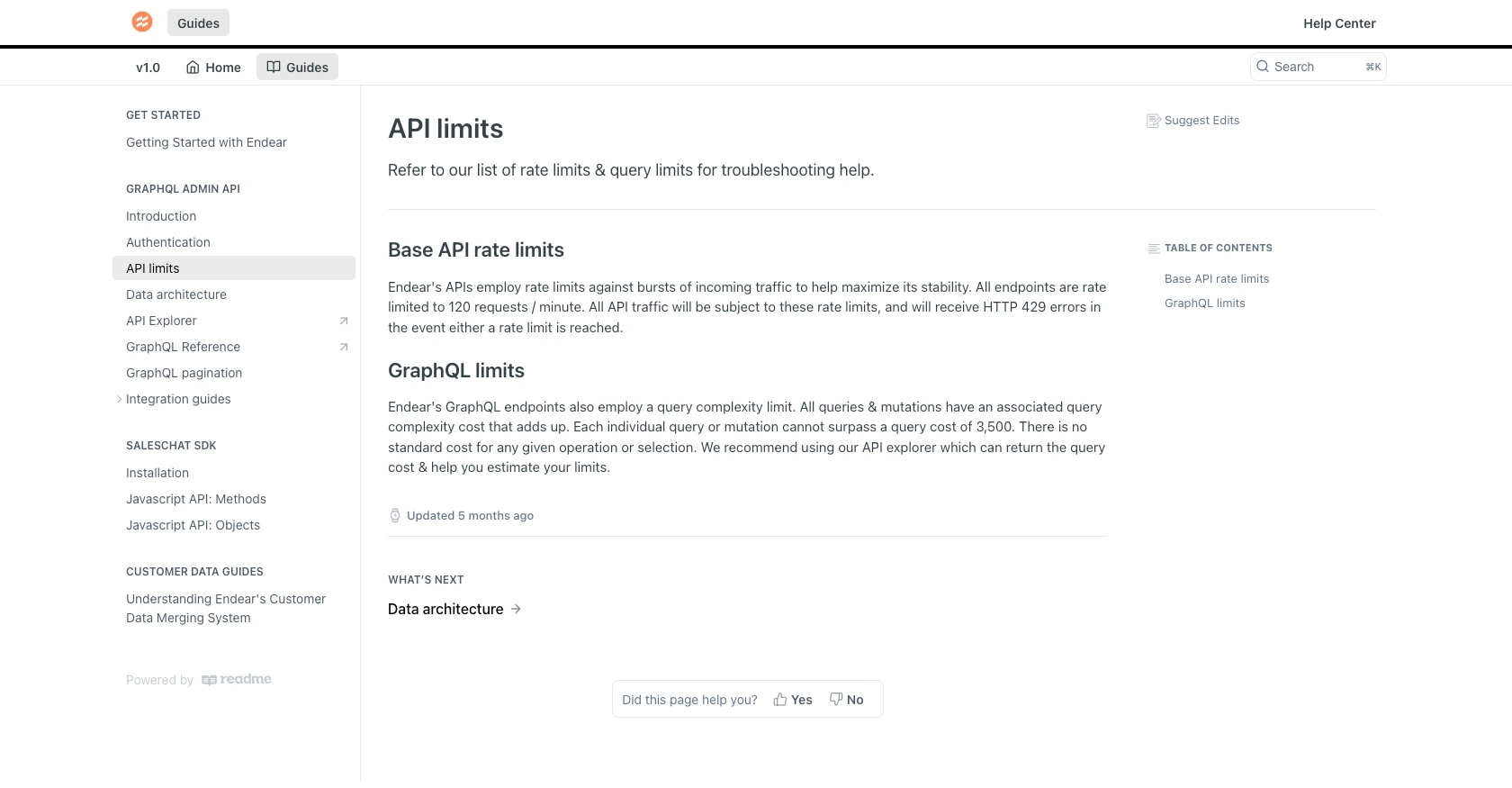
Conclusion and Best Practices for Using Endear API in PHP
Integrating with the Endear API using PHP offers a streamlined approach to managing customer data efficiently. By following the steps outlined in this guide, developers can automate customer profile creation and enhance their marketing strategies with personalized data insights.
Best Practices for Secure and Efficient Endear API Integration
- Secure API Key Storage: Always store your API keys securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limits: Be mindful of Endear's rate limit of 120 requests per minute. Implement strategies such as request queuing or exponential backoff to manage API calls effectively.
- Data Standardization: Ensure consistent data formats when sending customer information to avoid errors and maintain data integrity across integrations.
- Error Handling: Implement robust error handling to capture and log API errors, allowing for quick troubleshooting and minimal disruption.
Enhance Your Integration Experience with Endgrate
While integrating with the Endear API can significantly improve your customer data management, using a tool like Endgrate can further simplify the process. Endgrate allows you to manage multiple integrations through a single API endpoint, saving time and resources. Focus on your core product development while Endgrate handles the complexities of integration.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate today. Build once for each use case and provide an intuitive integration experience for your customers.
Read More
- https://endgrate.com/provider/endear
- https://docs.endearhq.com/docs/authentication
- https://docs.endearhq.com/docs/rate-limits-status-codes-and-errors
- https://docs.endearhq.com/docs/disclaimers
- https://developers.endearhq.com/docs/graphql/objects/Customer
- https://docs.endearhq.com/docs/send-your-first-record
Ready to get started?