Using the Confluence API to Create Space Pages (with PHP examples)
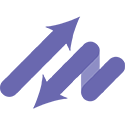
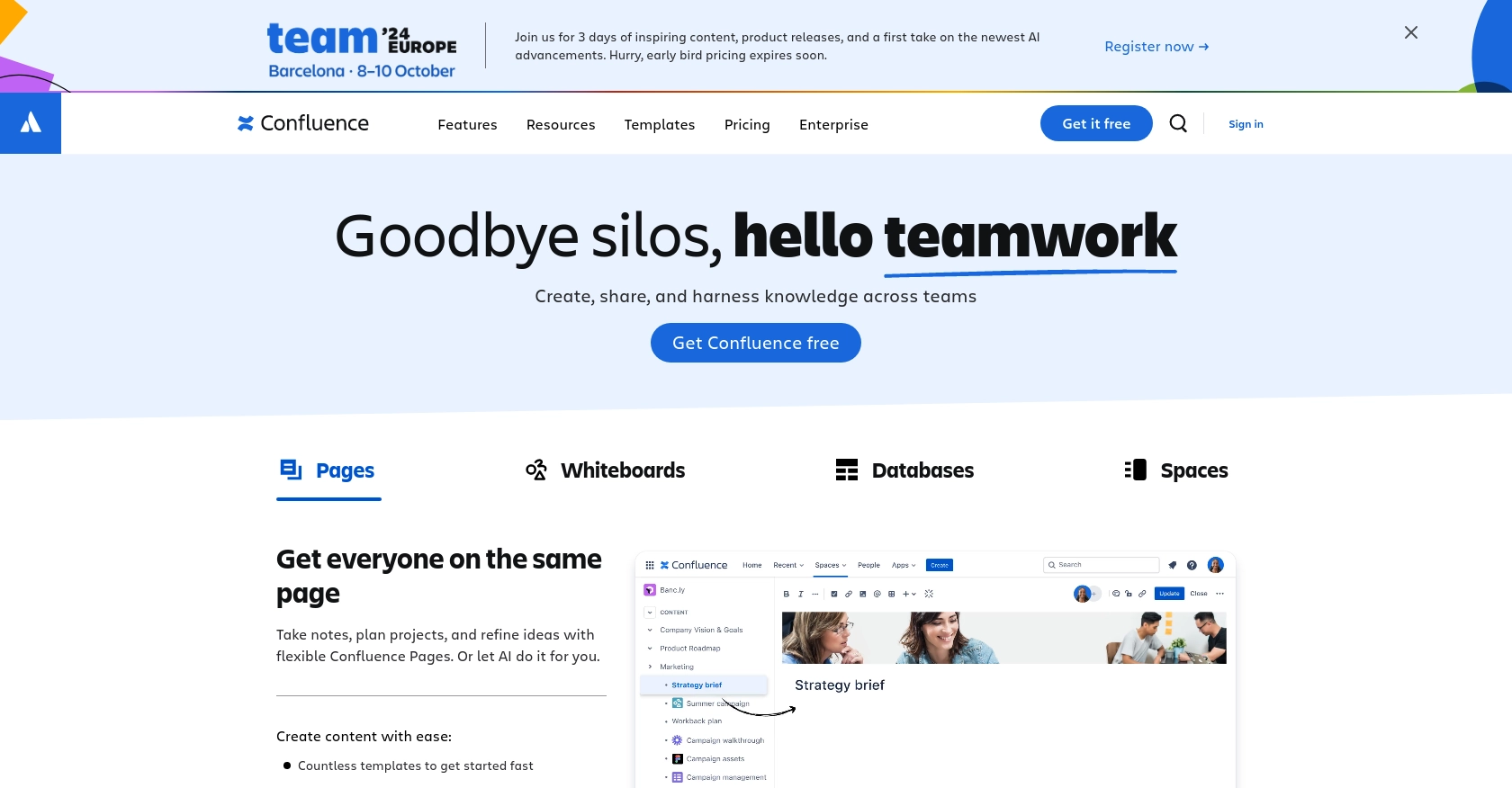
Introduction to Confluence API Integration
Confluence, developed by Atlassian, is a powerful collaboration tool used by teams to create, share, and manage content seamlessly. It offers a centralized platform for documentation, project management, and knowledge sharing, making it an essential tool for businesses aiming to enhance productivity and communication.
Integrating with the Confluence API allows developers to automate content management tasks, such as creating and updating space pages programmatically. For example, a developer might want to automate the creation of project documentation pages in Confluence directly from a project management tool, ensuring that all team members have access to the latest information without manual updates.
Setting Up Your Confluence Test or Sandbox Account
Before you can start creating space pages using the Confluence API, you need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting your live data.
Creating a Confluence Account
If you don't already have a Confluence account, you can sign up for a free trial on the Atlassian website. This trial will give you access to Confluence Cloud, where you can test API integrations.
- Visit the Confluence sign-up page.
- Follow the instructions to create your account.
- Once your account is set up, log in to access your Confluence dashboard.
Setting Up OAuth 2.0 for Confluence API Authentication
Confluence API uses OAuth 2.0 for authentication, which requires setting up an app to obtain the necessary credentials.
- Navigate to the Atlassian Developer Console.
- Create a new app by selecting Create App.
- Fill in the required details, such as app name and description.
- Under the Authorization section, configure OAuth 2.0 settings:
- Set the callback URL to your application's URL.
- Define the necessary scopes, such as
write:page:confluence
for creating pages. - Save your app settings to generate the Client ID and Client Secret.
Ensure you store the Client ID and Client Secret securely, as they will be used to authenticate API requests.
Generating Access Tokens for API Requests
With your app set up, you can now generate access tokens to authenticate your API requests.
- Use the Client ID and Client Secret to request an access token from the OAuth 2.0 token endpoint.
- Include the access token in the Authorization header of your API requests:
$headers = [
'Authorization: Bearer YOUR_ACCESS_TOKEN',
'Content-Type: application/json'
];
With these steps completed, you are ready to interact with the Confluence API and start creating space pages programmatically.
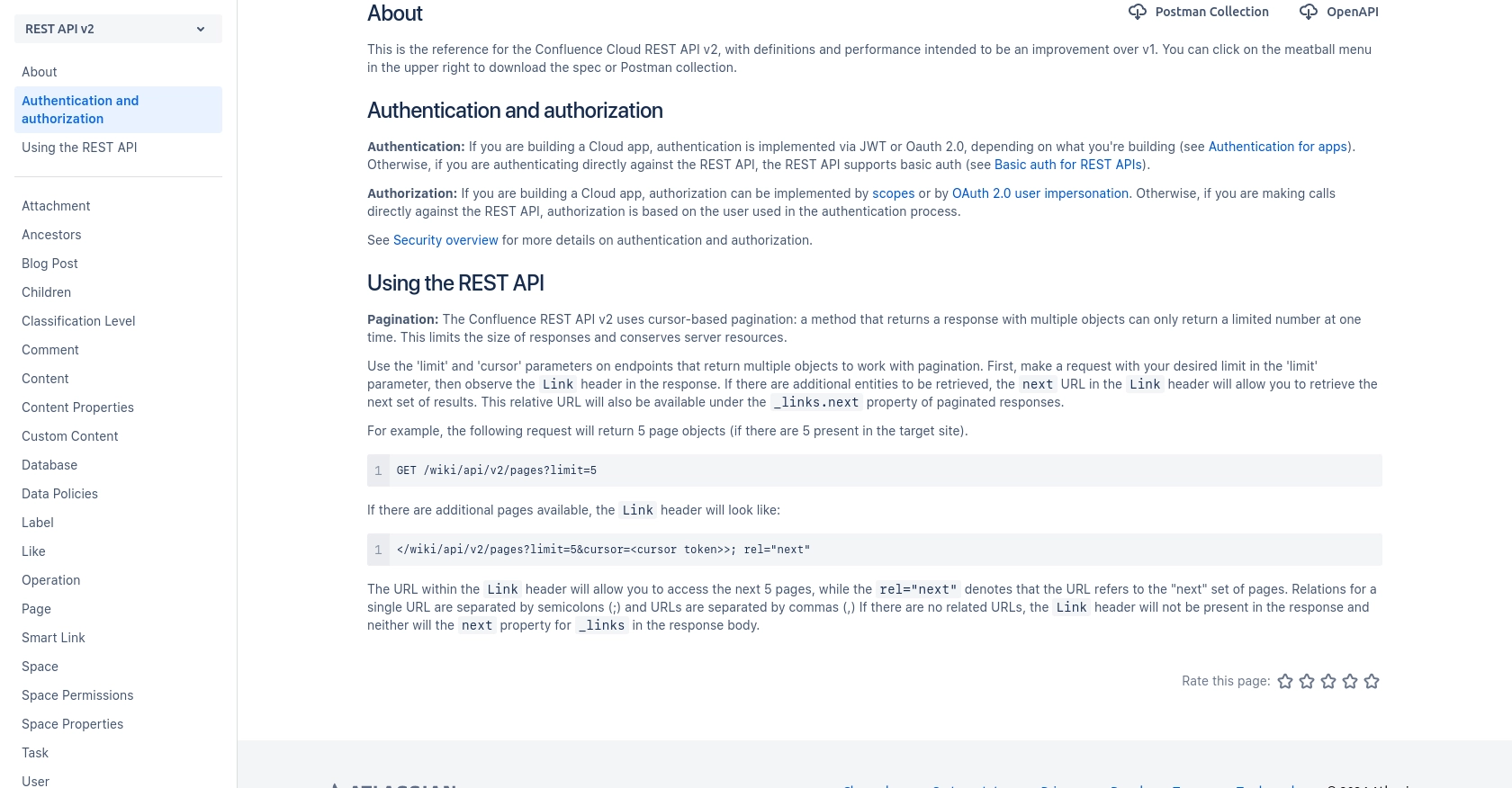
sbb-itb-96038d7
Making API Calls to Create Confluence Space Pages Using PHP
Once you have your Confluence account and OAuth 2.0 setup, you can start making API calls to create space pages. This section will guide you through the process using PHP, ensuring you have the right environment and code to interact with the Confluence API effectively.
Setting Up Your PHP Environment for Confluence API Integration
Before making API calls, ensure your PHP environment is ready. You'll need PHP 7.4 or later and the cURL
extension enabled to handle HTTP requests.
- Verify your PHP version by running
php -v
in your terminal. - Ensure the
cURL
extension is enabled by checking yourphp.ini
file or runningphp -m | grep curl
.
Creating a Confluence Space Page with PHP
To create a space page in Confluence, you'll need to make a POST request to the Confluence API endpoint. Below is a step-by-step guide with example code.
// Set the API endpoint
$url = "https://your-domain.atlassian.net/wiki/api/v2/pages";
// Prepare the request headers
$headers = [
'Authorization: Bearer YOUR_ACCESS_TOKEN',
'Content-Type: application/json'
];
// Define the page data
$data = [
'spaceId' => 'YOUR_SPACE_ID',
'status' => 'current',
'title' => 'Your Page Title',
'body' => [
'representation' => 'storage',
'value' => 'Your page content goes here
'
]
];
// Initialize cURL session
$ch = curl_init($url);
// Set cURL options
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Execute the request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Request Error:' . curl_error($ch);
} else {
echo 'Response:' . $response;
}
// Close cURL session
curl_close($ch);
Replace YOUR_ACCESS_TOKEN
and YOUR_SPACE_ID
with your actual access token and space ID. This code initializes a cURL session, sets the necessary headers and data, and executes the POST request to create a new page in the specified Confluence space.
Verifying the Confluence API Request Success
After executing the API call, check the response to ensure the page was created successfully. A successful response will include the page details in JSON format. You can also verify the page creation by logging into your Confluence account and navigating to the specified space.
Handling Errors and Understanding Confluence API Error Codes
It's crucial to handle potential errors when making API calls. Common error codes include:
- 400 Bad Request: The request was malformed. Check your data and headers.
- 401 Unauthorized: Authentication failed. Verify your access token.
- 404 Not Found: The specified resource could not be found. Check your endpoint URL and parameters.
For more detailed information on error handling, refer to the Confluence API documentation.
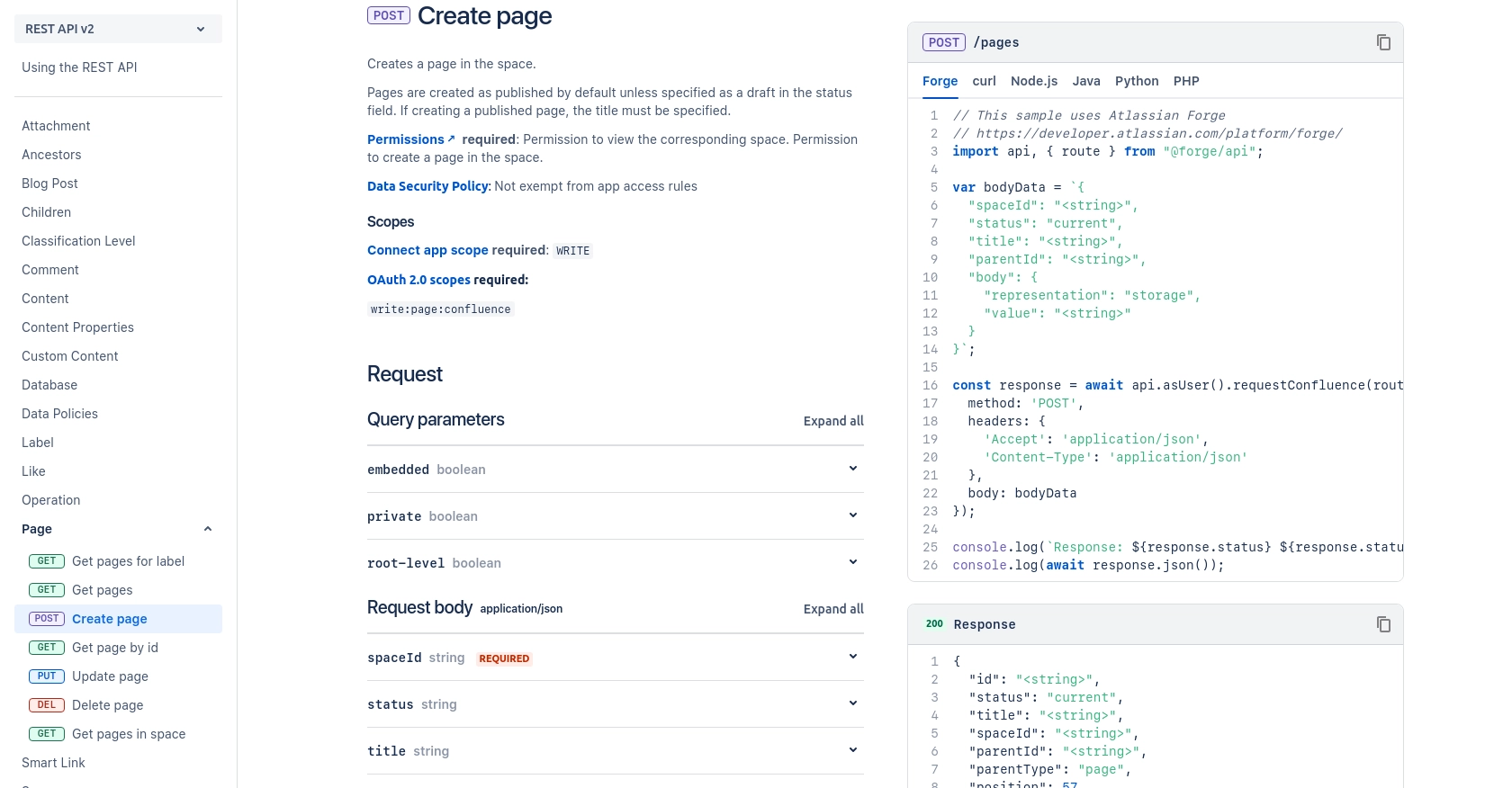
Best Practices for Confluence API Integration and Error Handling
When integrating with the Confluence API, it's essential to follow best practices to ensure a smooth and secure implementation. Here are some recommendations:
- Secure Storage of Credentials: Always store your Client ID, Client Secret, and access tokens securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Be mindful of Confluence's rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit responses gracefully.
- Data Standardization: Ensure that data fields are standardized and consistent across your application to avoid discrepancies and errors during API interactions.
- Comprehensive Error Handling: Implement robust error handling to manage different HTTP status codes and provide meaningful feedback to users or logs for debugging.
Enhancing Integration Efficiency with Endgrate
Building and maintaining multiple integrations can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Confluence. By using Endgrate, you can:
- Save time and resources by outsourcing integrations, allowing you to focus on your core product development.
- Build once for each use case instead of multiple times for different integrations, streamlining your development process.
- Offer an intuitive integration experience for your customers, enhancing user satisfaction and engagement.
Explore how Endgrate can transform your integration strategy by visiting Endgrate and discover the benefits of a seamless integration experience.
Read More
Ready to get started?