How to Create Recipients with the Mailshake API in Javascript
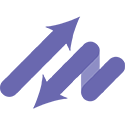
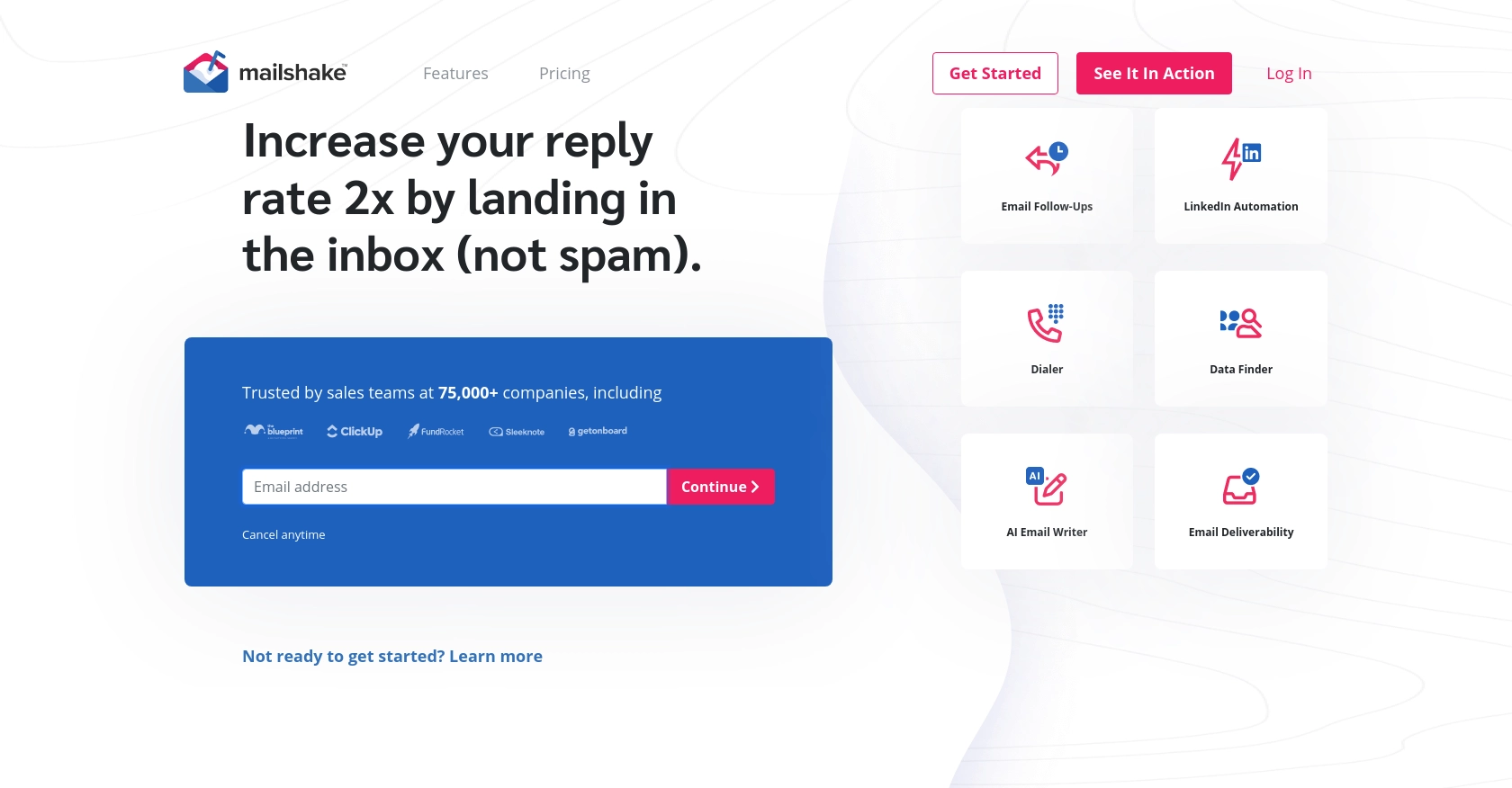
Introduction to Mailshake API Integration
Mailshake is a powerful sales engagement platform designed to streamline email outreach and follow-up processes. It offers a suite of tools that enable sales teams to automate their email campaigns, track engagement, and manage leads effectively.
Integrating with the Mailshake API allows developers to enhance their email marketing strategies by programmatically managing campaigns and recipients. For example, you can automate the addition of new recipients to a campaign, ensuring your outreach efforts are always up-to-date and targeted.
This article will guide you through the process of creating recipients using the Mailshake API with JavaScript, providing a step-by-step approach to streamline your email marketing workflows.
Setting Up Your Mailshake API Account for Testing
Before you can start integrating with the Mailshake API, you'll need to set up your account to access the necessary API keys. Mailshake provides a straightforward process for obtaining an API key, which is essential for authenticating your requests.
Creating a Mailshake Account
If you don't already have a Mailshake account, you can sign up for a free trial or a paid plan on the Mailshake website. This will give you access to the platform's features and allow you to generate an API key.
Generating Your Mailshake API Key
Once your account is set up, follow these steps to generate your API key:
- Log in to your Mailshake account.
- Navigate to the Extensions section in the dashboard.
- Select API from the menu.
- Click on Create API Key to generate a new key.
- Copy the API key and store it securely, as you'll need it to authenticate your API requests.
For more details on obtaining your API key, refer to the Mailshake API documentation.
Understanding Mailshake API Authentication
The Mailshake API uses simple API key-based authentication. You can include your API key in your requests in one of the following ways:
- As a query string parameter:
?apiKey=your-api-key
- In the request body as JSON:
{"apiKey": "your-api-key"}
- Via an HTTP Authorization header:
Authorization: Basic [base-64 encoded version of your api key]
Ensure you replace your-api-key
with the actual key you generated. This key will allow your application to interact with the Mailshake API securely.
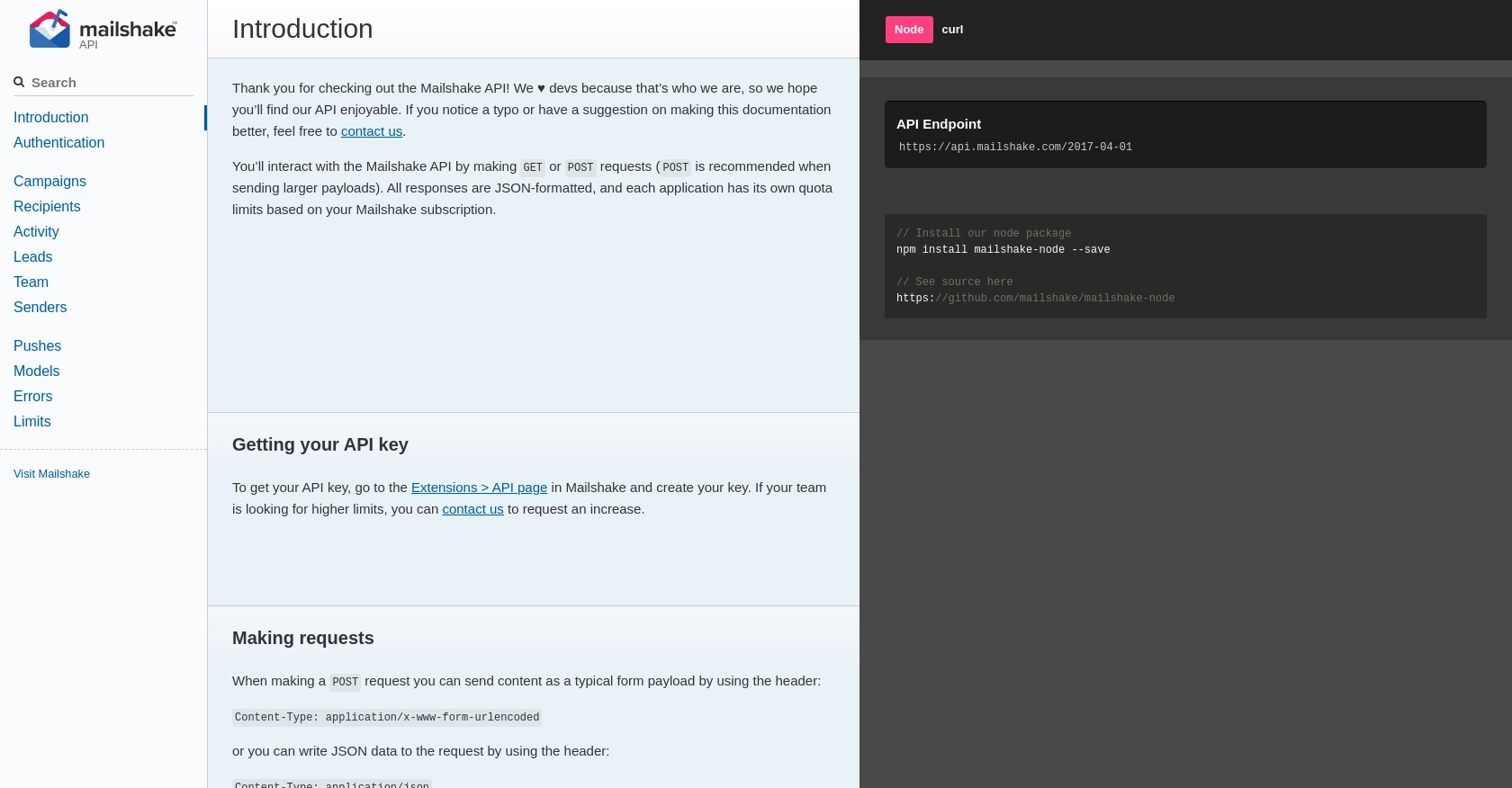
sbb-itb-96038d7
Making API Calls to Add Recipients with Mailshake API in JavaScript
To effectively interact with the Mailshake API using JavaScript, you need to ensure you have the right environment set up. This section will guide you through the necessary steps to make API calls for adding recipients to your Mailshake campaigns.
Setting Up Your JavaScript Environment
Before making API calls, ensure you have Node.js installed on your machine. Node.js allows you to run JavaScript code outside of a browser, which is essential for server-side operations like API calls.
To check if Node.js is installed, run the following command in your terminal:
node -v
If Node.js is not installed, download and install it from the official Node.js website.
Installing Required Dependencies
For making HTTP requests in JavaScript, you can use the popular axios
library. Install it using npm (Node Package Manager) with the following command:
npm install axios
Creating Recipients with Mailshake API
With your environment set up, you can now proceed to create recipients using the Mailshake API. Below is an example of how to make a POST request to add recipients to a campaign.
const axios = require('axios');
// Define the API endpoint and your API key
const endpoint = 'https://api.mailshake.com/2017-04-01/recipients/add';
const apiKey = 'your-api-key';
// Set the request headers
const headers = {
'Content-Type': 'application/json',
'Authorization': `Basic ${Buffer.from(apiKey).toString('base64')}`
};
// Define the recipient data
const recipientData = {
campaignID: 1,
addAsNewList: true,
listOfEmails: '"John Doe" <john@doe.com>, "Jane Doe" <jane@doe.com>'
};
// Make the POST request
axios.post(endpoint, recipientData, { headers })
.then(response => {
console.log('Recipients added successfully:', response.data);
})
.catch(error => {
console.error('Error adding recipients:', error.response.data);
});
Replace your-api-key
with the API key you generated earlier. This code snippet demonstrates how to add recipients to a specific campaign using the Mailshake API. The listOfEmails
parameter contains the email addresses of the recipients you want to add.
Verifying API Call Success
After executing the API call, you can verify the success by checking the response data. A successful addition will return a confirmation message. Additionally, you can log into your Mailshake account and navigate to the campaign to see the newly added recipients.
Handling Errors and Error Codes
When making API calls, it's crucial to handle potential errors. The Mailshake API provides specific error codes that can help you diagnose issues. For example, an invalid_api_key
error indicates a problem with your API key. Refer to the Mailshake API documentation for a comprehensive list of error codes and their meanings.
Conclusion and Best Practices for Using Mailshake API in JavaScript
Integrating with the Mailshake API using JavaScript offers a powerful way to automate and enhance your email marketing campaigns. By following the steps outlined in this guide, you can efficiently add recipients to your Mailshake campaigns, ensuring your outreach efforts are both targeted and effective.
Best Practices for Secure and Efficient Mailshake API Integration
- Secure API Key Storage: Always store your API keys securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limits: Be mindful of Mailshake's rate limits to avoid interruptions in service. Implement logic to handle the
limit_reached
error and retry requests after the specified wait time. - Data Standardization: Ensure that recipient data is standardized before adding it to campaigns. This includes validating email addresses and formatting names correctly.
- Error Handling: Implement robust error handling to manage API response errors effectively. Refer to the Mailshake API documentation for detailed error codes and solutions.
Streamlining Integrations with Endgrate
While integrating with individual APIs like Mailshake can be beneficial, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process across various platforms, including Mailshake.
By using Endgrate, you can save time and resources, allowing your team to focus on core product development. With a single API endpoint, you can manage multiple integrations seamlessly, providing an intuitive experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate and discover the benefits of a streamlined, efficient integration process.
Read More
Ready to get started?