Using the Zoho Books API to Create or Update Accounts in Javascript
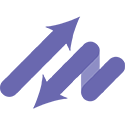
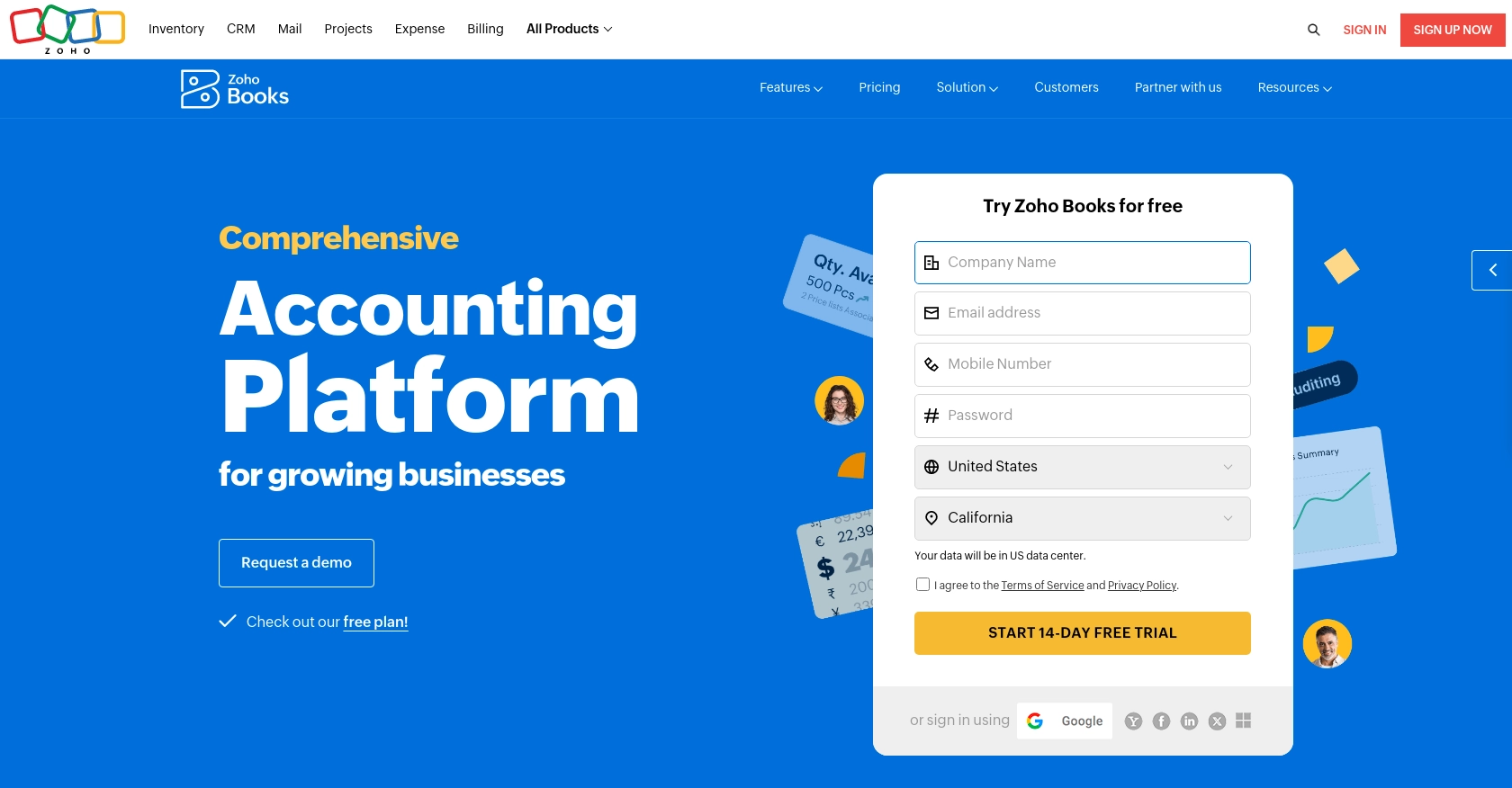
Introduction to Zoho Books API for Account Management
Zoho Books is a comprehensive online accounting software designed to manage your finances, automate business workflows, and help you work collectively across departments. It offers a wide range of features including invoicing, expense tracking, and inventory management, making it a popular choice for businesses looking to streamline their accounting processes.
Integrating with the Zoho Books API allows developers to automate and enhance financial operations by programmatically interacting with the platform. For example, you can create or update accounts directly from your application, ensuring that your financial data is always up-to-date and accurate. This can be particularly useful for businesses that need to manage a large number of accounts or frequently update account information.
In this article, we will explore how to use JavaScript to interact with the Zoho Books API, specifically focusing on creating or updating accounts. This integration can help developers save time and reduce errors by automating repetitive tasks, allowing them to focus on more strategic activities.
Setting Up Your Zoho Books Test/Sandbox Account for API Integration
Before diving into the integration process, it's essential to set up a Zoho Books account to test and interact with the API. Zoho Books offers a free trial, allowing developers to explore its features without any initial investment.
Creating a Zoho Books Account
To get started, follow these steps to create your Zoho Books account:
- Visit the Zoho Books signup page and fill out the registration form with your details.
- Once registered, log in to your account and navigate to the dashboard.
- Familiarize yourself with the platform by exploring its various features and settings.
Setting Up OAuth for Zoho Books API Access
Zoho Books uses OAuth 2.0 for secure API authentication. Follow these steps to set up OAuth and obtain the necessary credentials:
- Go to the Zoho Developer Console and click on "Add Client ID" to register your application.
- Fill in the required details, including the redirect URI, and submit the form to receive your Client ID and Client Secret.
- Keep these credentials secure, as they are essential for authenticating your API requests.
Generating an OAuth Access Token
With your Client ID and Client Secret, you can now generate an OAuth access token:
- Redirect users to the following URL to obtain a grant token:
- Once the user consents, Zoho will redirect to your specified URI with a code parameter.
- Exchange this code for an access token by making a POST request:
- Store the access token securely, as it will be used in API requests.
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoBooks.fullaccess.all&client_id=YOUR_CLIENT_ID&response_type=code&redirect_uri=YOUR_REDIRECT_URI&access_type=offline
https://accounts.zoho.com/oauth/v2/token?code=YOUR_CODE&client_id=YOUR_CLIENT_ID&client_secret=YOUR_CLIENT_SECRET&redirect_uri=YOUR_REDIRECT_URI&grant_type=authorization_code
For more detailed information on OAuth setup, refer to the Zoho Books OAuth documentation.
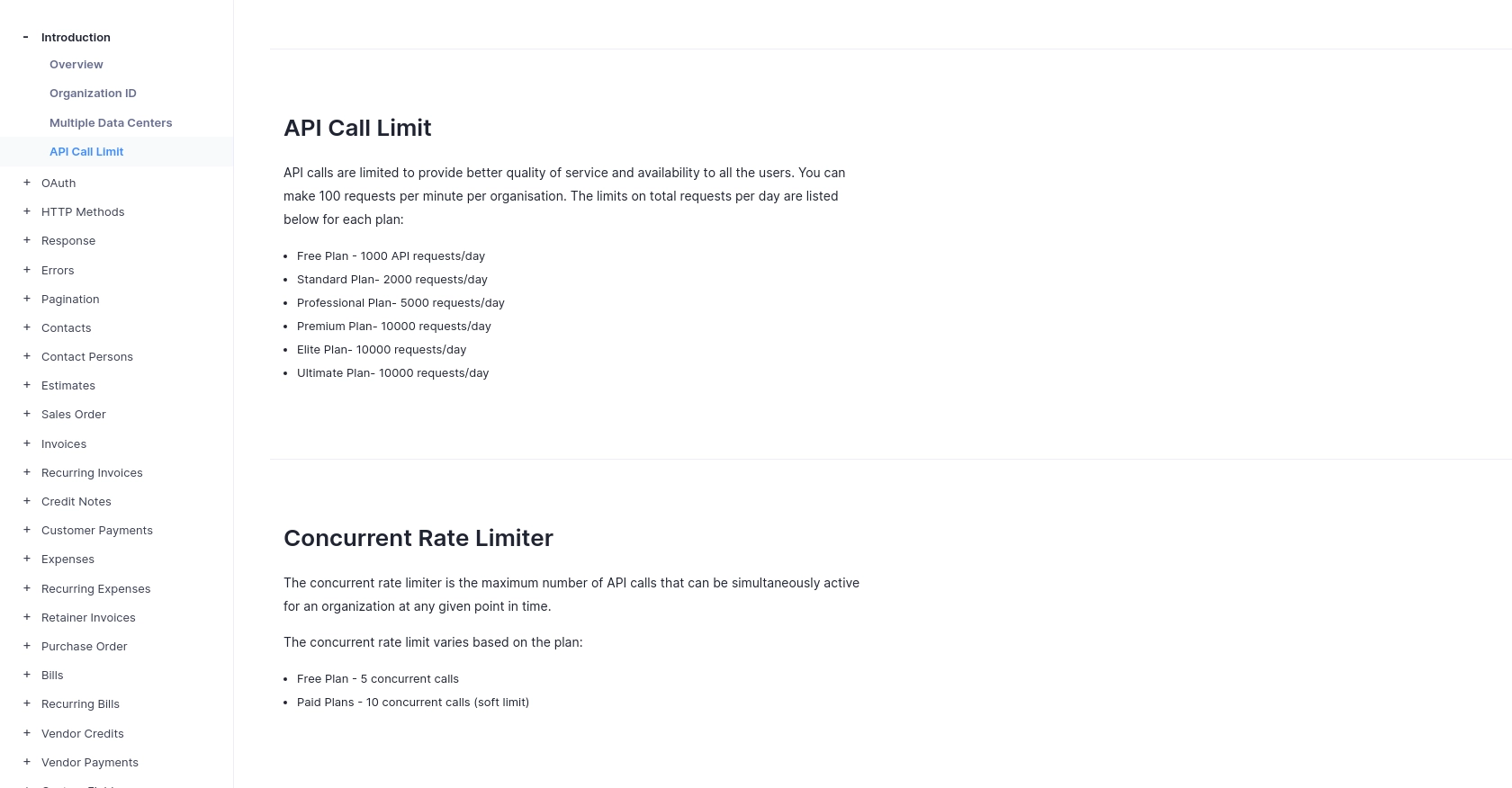
sbb-itb-96038d7
Making API Calls to Zoho Books for Account Creation and Updates Using JavaScript
To interact with the Zoho Books API for creating or updating accounts, you need to make HTTP requests using JavaScript. This section will guide you through the process, including setting up your environment, writing the code, and handling responses.
Setting Up Your JavaScript Environment for Zoho Books API Integration
Before making API calls, ensure your JavaScript environment is ready. You will need:
- A modern JavaScript runtime, such as Node.js.
- Access to the
fetch
API or a similar HTTP client like Axios for making HTTP requests.
Creating an Account in Zoho Books Using JavaScript
To create an account in Zoho Books, you will use a POST request. Here’s how you can do it:
const options = {
method: 'POST',
headers: {
'Authorization': 'Zoho-oauthtoken YOUR_ACCESS_TOKEN',
'Content-Type': 'application/json'
},
body: JSON.stringify({
account_name: 'New Account',
account_code: '12345',
account_type: 'income',
currency_id: '460000000000097',
description: 'This is a new income account.'
})
};
fetch('https://www.zohoapis.com/books/v3/chartofaccounts?organization_id=YOUR_ORG_ID', options)
.then(response => response.json())
.then(data => console.log('Account Created:', data))
.catch(error => console.error('Error:', error));
Replace YOUR_ACCESS_TOKEN
and YOUR_ORG_ID
with your actual access token and organization ID. This code sends a request to create a new account with specified details.
Updating an Existing Account in Zoho Books Using JavaScript
To update an existing account, you will use a PUT request. Here’s an example:
const updateOptions = {
method: 'PUT',
headers: {
'Authorization': 'Zoho-oauthtoken YOUR_ACCESS_TOKEN',
'Content-Type': 'application/json'
},
body: JSON.stringify({
account_name: 'Updated Account Name',
description: 'Updated description for the account.'
})
};
fetch('https://www.zohoapis.com/books/v3/chartofaccounts/YOUR_ACCOUNT_ID?organization_id=YOUR_ORG_ID', updateOptions)
.then(response => response.json())
.then(data => console.log('Account Updated:', data))
.catch(error => console.error('Error:', error));
Replace YOUR_ACCOUNT_ID
, YOUR_ACCESS_TOKEN
, and YOUR_ORG_ID
with the relevant account ID, access token, and organization ID. This code updates the account details as specified in the request body.
Handling API Responses and Errors for Zoho Books Integration
After making API calls, it’s crucial to handle responses and potential errors. Zoho Books uses standard HTTP status codes:
- 200 OK: The request was successful.
- 201 Created: The account was successfully created.
- 400 Bad Request: The request was invalid.
- 401 Unauthorized: Authentication failed. Check your access token.
- 404 Not Found: The specified account ID does not exist.
- 429 Rate Limit Exceeded: You have exceeded the API call limit.
For more details on error handling, refer to the Zoho Books Errors documentation.
By following these steps, you can efficiently create and update accounts in Zoho Books using JavaScript, streamlining your financial operations and reducing manual effort.
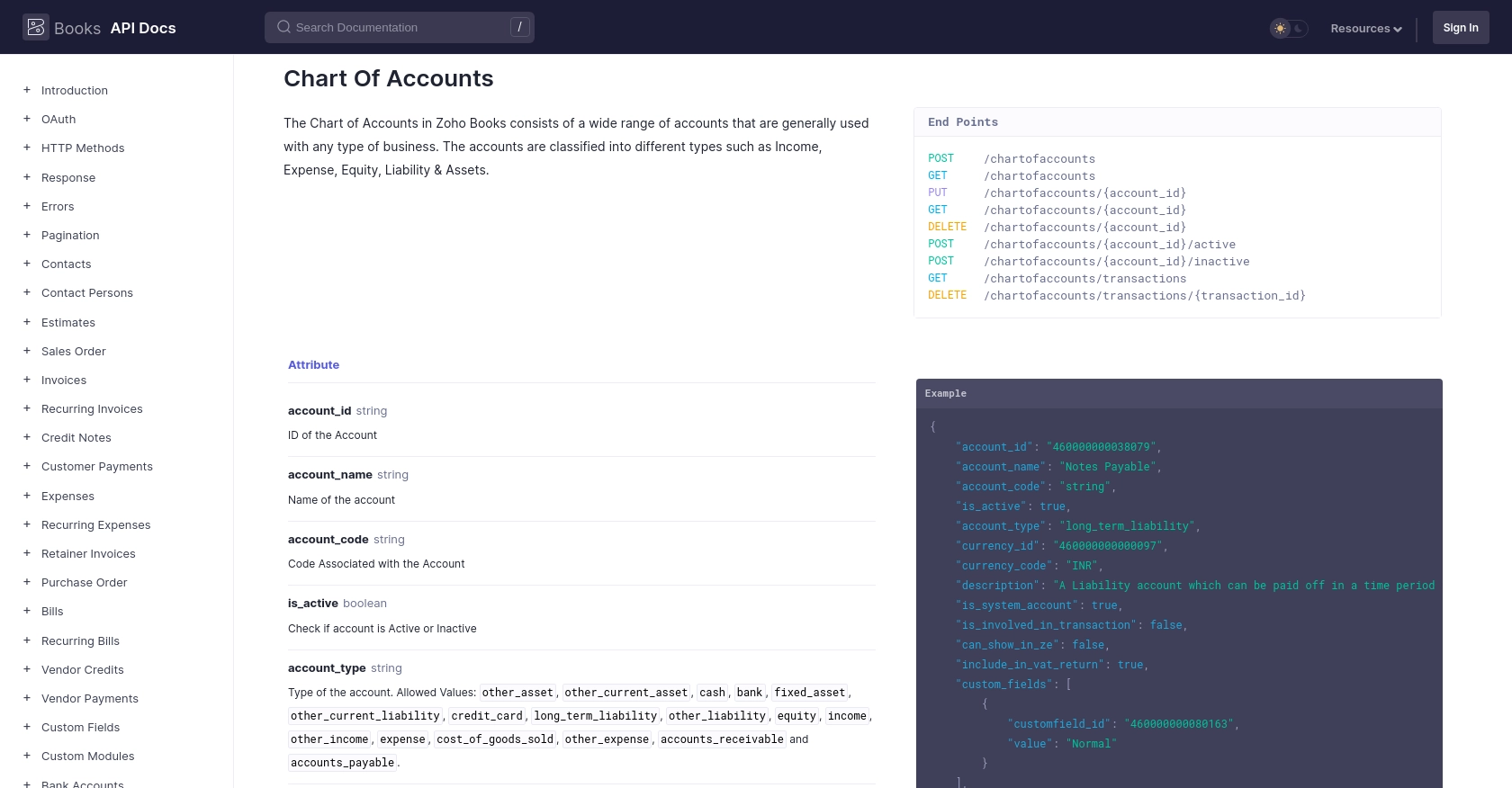
Conclusion and Best Practices for Zoho Books API Integration
Integrating with the Zoho Books API using JavaScript offers a powerful way to automate financial operations and ensure data accuracy across your business systems. By following the steps outlined in this guide, you can efficiently create and update accounts, reducing manual effort and minimizing errors.
Best Practices for Secure and Efficient Zoho Books API Usage
- Securely Store Credentials: Always keep your OAuth credentials, such as the Client ID, Client Secret, and access tokens, secure. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limiting: Zoho Books imposes rate limits on API calls. Ensure your application handles these limits gracefully by implementing retry logic or backoff strategies. For more details, refer to the Zoho Books API Call Limit documentation.
- Standardize Data Fields: When creating or updating accounts, ensure that data fields are standardized to maintain consistency across your financial records.
- Monitor API Usage: Regularly monitor your API usage to stay within the allowed limits and optimize your application's performance.
Streamline Your Integrations with Endgrate
While integrating with Zoho Books directly can be beneficial, managing multiple integrations can become complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Zoho Books.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development. With Endgrate, you build once for each use case, rather than multiple times for different integrations, offering an intuitive experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate today.
Read More
- https://endgrate.com/provider/zohobooks
- https://www.zoho.com/books/api/v3/introduction/#api-call-limit
- https://www.zoho.com/books/api/v3/oauth/#overview
- https://www.zoho.com/books/api/v3/errors/#overview
- https://www.zoho.com/books/api/v3/pagination/#overview
- https://www.zoho.com/books/api/v3/chart-of-accounts/#overview
Ready to get started?