Using the Zoho Books API to Create or Update Sales Orders in Javascript
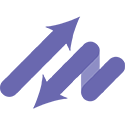
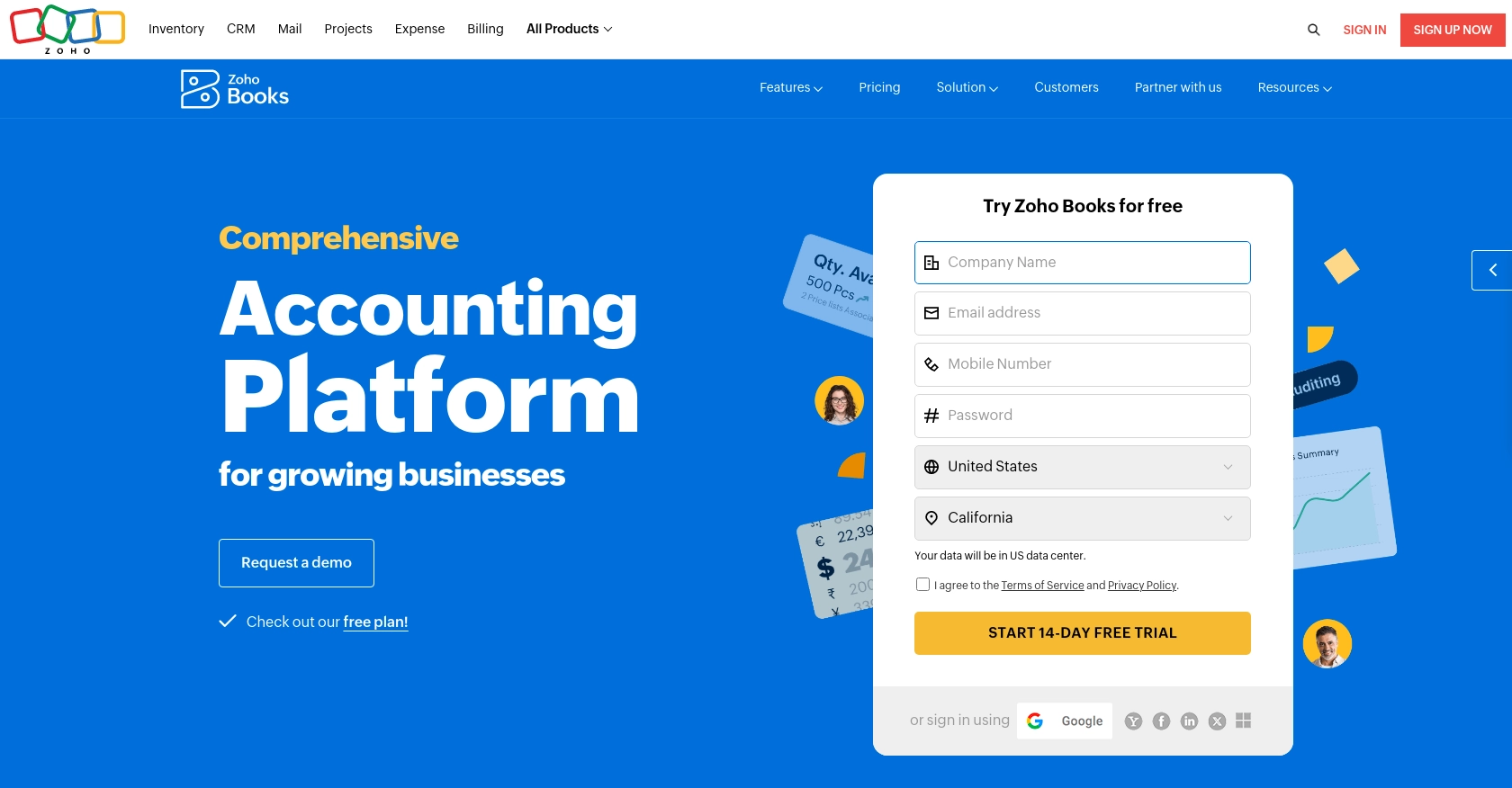
Introduction to Zoho Books API for Sales Order Management
Zoho Books is a comprehensive online accounting software designed to manage your finances, automate business workflows, and help you work collectively across departments. It offers a robust API that allows developers to integrate and automate various accounting tasks, including creating and updating sales orders.
Integrating with the Zoho Books API can significantly enhance the efficiency of managing sales orders. For example, developers can automate the creation and updating of sales orders directly from their e-commerce platforms, ensuring real-time synchronization and reducing manual entry errors.
This article will guide you through using JavaScript to interact with the Zoho Books API, focusing on creating and updating sales orders. By the end of this tutorial, you'll be able to seamlessly integrate Zoho Books into your existing systems, streamlining your sales order management process.
Setting Up Your Zoho Books Test/Sandbox Account for API Integration
Before you can start integrating with the Zoho Books API, you'll need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting your live data. Zoho Books provides a sandbox environment that mimics the production environment, making it ideal for testing your integration.
Creating a Zoho Books Sandbox Account
- Visit the Zoho Books website and sign up for a free trial if you don't already have an account.
- Once logged in, navigate to the Developer Console by clicking on your profile picture and selecting Developer Space.
- In the Developer Console, click on Sandbox to create a new sandbox environment. Follow the prompts to set up your sandbox account.
Configuring OAuth for Zoho Books API Access
The Zoho Books API uses OAuth 2.0 for authentication. Follow these steps to configure OAuth:
- In the Developer Console, click on Add Client ID to register a new application.
- Fill in the required details, including the Redirect URI, which is the URL where users will be redirected after authentication.
- After registration, you'll receive a Client ID and Client Secret. Keep these credentials secure.
Generating OAuth Tokens for Zoho Books API
With your Client ID and Client Secret, you can generate OAuth tokens to authenticate API requests:
- Redirect users to the following URL to obtain an authorization code:
- After user consent, you'll receive an authorization code at your redirect URI.
- Exchange the authorization code for access and refresh tokens by making a POST request:
- Store the access token securely, as it will be used to authenticate your API requests.
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoBooks.salesorders.CREATE,ZohoBooks.salesorders.UPDATE&client_id=YOUR_CLIENT_ID&response_type=code&redirect_uri=YOUR_REDIRECT_URI
fetch('https://accounts.zoho.com/oauth/v2/token', {
method: 'POST',
headers: {
'Content-Type': 'application/x-www-form-urlencoded'
},
body: new URLSearchParams({
code: 'YOUR_AUTH_CODE',
client_id: 'YOUR_CLIENT_ID',
client_secret: 'YOUR_CLIENT_SECRET',
redirect_uri: 'YOUR_REDIRECT_URI',
grant_type: 'authorization_code'
})
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
With your sandbox account and OAuth tokens set up, you're ready to start making API calls to Zoho Books. This setup ensures that you can test your integration thoroughly before deploying it to a live environment.
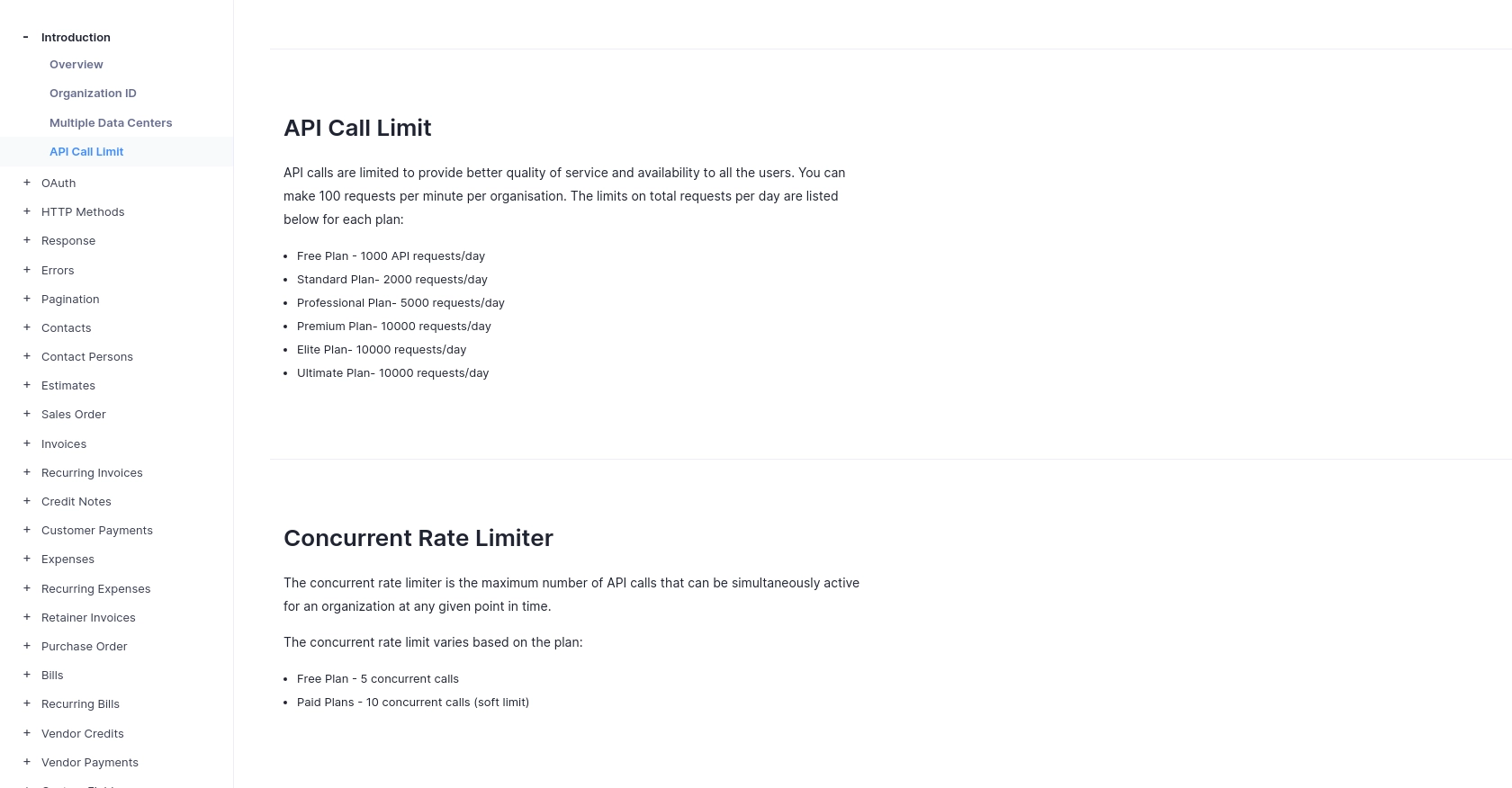
sbb-itb-96038d7
Making API Calls to Zoho Books for Sales Order Management Using JavaScript
With your Zoho Books sandbox account and OAuth tokens ready, you can now proceed to make API calls to create or update sales orders. This section will guide you through the process of setting up your JavaScript environment and executing API requests to Zoho Books.
Setting Up Your JavaScript Environment for Zoho Books API Integration
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine.
- A code editor like Visual Studio Code.
To manage HTTP requests, you can use the node-fetch
library. Install it using the following command:
npm install node-fetch
Creating a Sales Order in Zoho Books Using JavaScript
To create a sales order, you need to make a POST request to the Zoho Books API. Here's a step-by-step guide:
- Create a new JavaScript file,
createSalesOrder.js
, and add the following code: - Replace
YOUR_ORG_ID
andYOUR_ACCESS_TOKEN
with your actual organization ID and access token. - Run the script using the command:
const fetch = require('node-fetch');
const createSalesOrder = async () => {
const url = 'https://www.zohoapis.com/books/v3/salesorders?organization_id=YOUR_ORG_ID';
const token = 'YOUR_ACCESS_TOKEN';
const salesOrderData = {
customer_id: '460000000017138',
line_items: [
{
item_id: '460000000017088',
quantity: 10,
rate: 100
}
]
};
try {
const response = await fetch(url, {
method: 'POST',
headers: {
'Authorization': `Zoho-oauthtoken ${token}`,
'Content-Type': 'application/json'
},
body: JSON.stringify(salesOrderData)
});
const data = await response.json();
console.log('Sales Order Created:', data);
} catch (error) {
console.error('Error creating sales order:', error);
}
};
createSalesOrder();
node createSalesOrder.js
Updating a Sales Order in Zoho Books Using JavaScript
To update an existing sales order, make a PUT request. Follow these steps:
- Create a new JavaScript file,
updateSalesOrder.js
, and add the following code: - Replace
YOUR_ORG_ID
,YOUR_ACCESS_TOKEN
, andsalesOrderId
with your actual organization ID, access token, and the sales order ID you wish to update. - Run the script using the command:
const fetch = require('node-fetch');
const updateSalesOrder = async () => {
const salesOrderId = '460000000039129';
const url = `https://www.zohoapis.com/books/v3/salesorders/${salesOrderId}?organization_id=YOUR_ORG_ID`;
const token = 'YOUR_ACCESS_TOKEN';
const updatedData = {
line_items: [
{
line_item_id: '460000000039131',
quantity: 20
}
]
};
try {
const response = await fetch(url, {
method: 'PUT',
headers: {
'Authorization': `Zoho-oauthtoken ${token}`,
'Content-Type': 'application/json'
},
body: JSON.stringify(updatedData)
});
const data = await response.json();
console.log('Sales Order Updated:', data);
} catch (error) {
console.error('Error updating sales order:', error);
}
};
updateSalesOrder();
node updateSalesOrder.js
Handling API Responses and Errors
After executing the API calls, verify the responses to ensure successful operations. The Zoho Books API returns a JSON response with a code
and message
indicating the status of the request. Common HTTP status codes include:
- 200: OK
- 201: Created
- 400: Bad Request
- 401: Unauthorized
- 404: Not Found
- 429: Rate Limit Exceeded
For more details on error codes, refer to the Zoho Books API documentation.
By following these steps, you can effectively manage sales orders in Zoho Books using JavaScript, enhancing your business's operational efficiency.
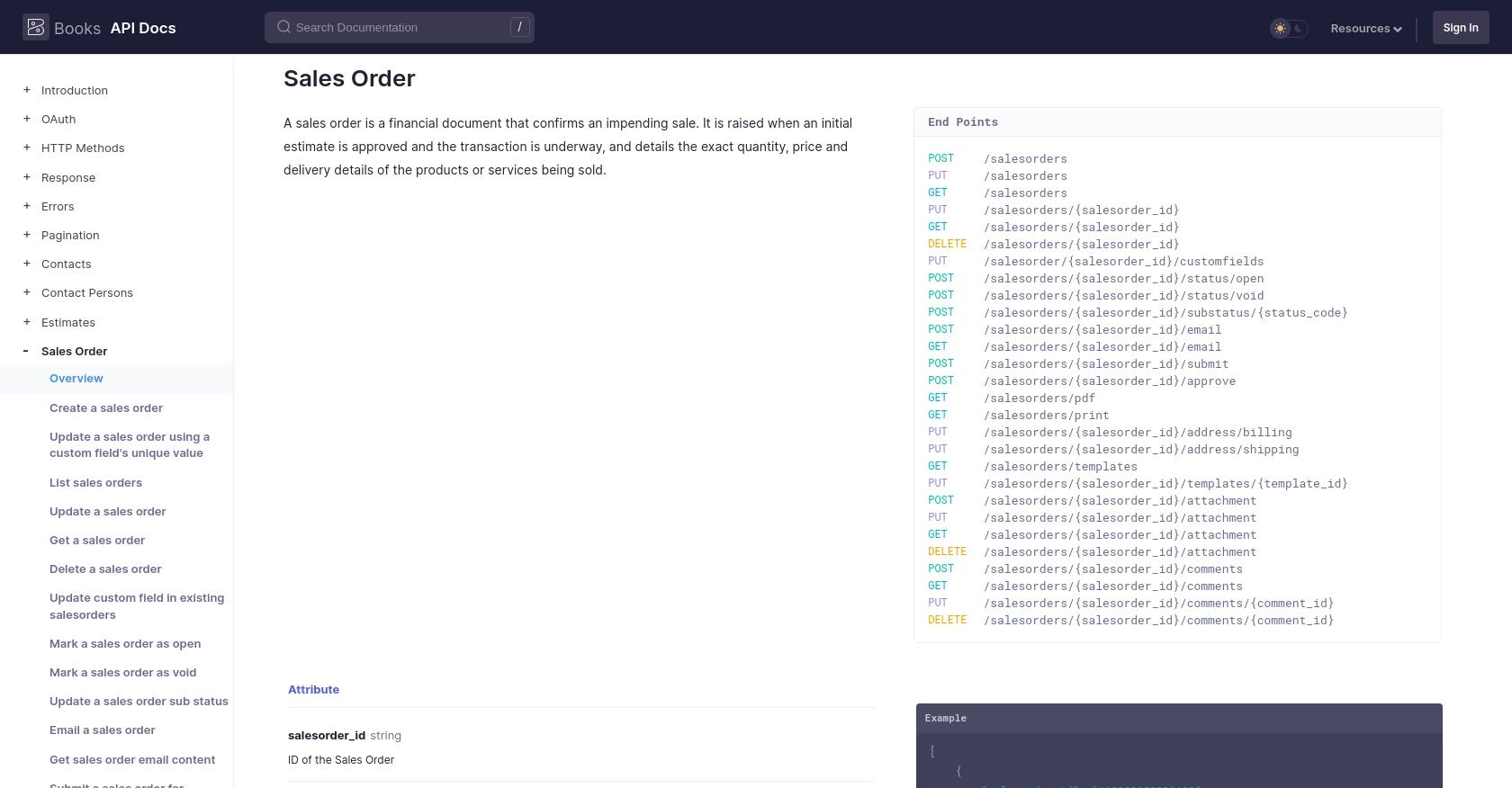
Conclusion: Best Practices for Zoho Books API Integration
Integrating with the Zoho Books API using JavaScript can significantly streamline your sales order management process. By automating the creation and updating of sales orders, you can reduce manual errors and improve efficiency. However, to ensure a smooth integration, it's essential to follow best practices.
Securely Storing Zoho Books API Credentials
Always store your OAuth tokens securely. Avoid hardcoding them in your scripts. Use environment variables or secure vaults to manage sensitive information. This practice helps prevent unauthorized access and protects your data.
Handling Zoho Books API Rate Limits
Zoho Books imposes rate limits to ensure fair usage. The API allows 100 requests per minute per organization. Exceeding this limit will result in a 429 Rate Limit Exceeded error. Implement retry logic with exponential backoff to handle rate limiting gracefully.
Transforming and Standardizing Data for Zoho Books API
Ensure that the data you send to Zoho Books is correctly formatted and standardized. This includes validating data types and ensuring required fields are populated. Proper data handling reduces the risk of errors and improves the reliability of your integration.
Enhancing Integration with Endgrate
For developers looking to simplify their integration processes, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Endgrate provides a unified API endpoint that connects to multiple platforms, including Zoho Books, offering an intuitive integration experience for your customers.
By following these best practices and leveraging tools like Endgrate, you can optimize your integration with Zoho Books, ensuring a seamless and efficient sales order management process.
Read More
- https://endgrate.com/provider/zohobooks
- https://www.zoho.com/books/api/v3/introduction/#api-call-limit
- https://www.zoho.com/books/api/v3/oauth/#overview
- https://www.zoho.com/books/api/v3/errors/#overview
- https://www.zoho.com/books/api/v3/pagination/#overview
- https://www.zoho.com/books/api/v3/sales-order/#overview
Ready to get started?