How to Create or Update Leads with the Copper API in PHP
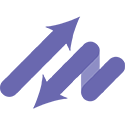
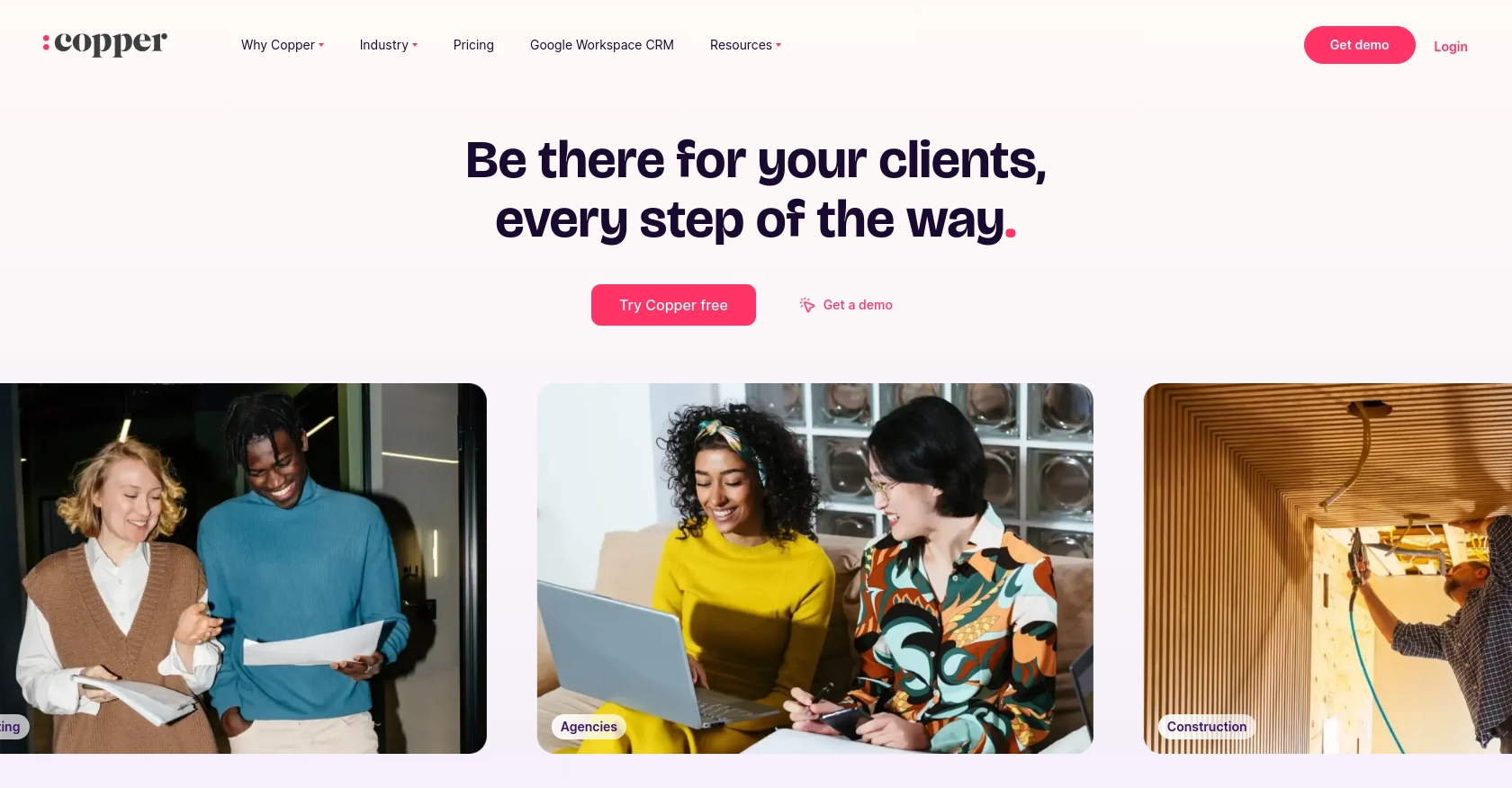
Introduction to Copper CRM API
Copper is a powerful CRM platform designed to seamlessly integrate with Google Workspace, providing businesses with an efficient way to manage customer relationships and sales processes. Its user-friendly interface and robust features make it a popular choice for companies looking to enhance their CRM capabilities.
Developers may wish to integrate with Copper's API to automate and streamline various CRM tasks, such as creating or updating leads. For example, you could use the Copper API to automatically update lead information from an external source, ensuring your sales team always has the most current data.
Setting Up Your Copper CRM Test or Sandbox Account
Before you can start integrating with the Copper API, you need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting your live data. Follow these steps to get started:
Create a Copper CRM Account
- Visit the Copper website and sign up for a free trial or demo account.
- Follow the on-screen instructions to complete the registration process.
- Once your account is created, log in to access the Copper dashboard.
Generate Copper API Key and Secret
To authenticate your API requests, you'll need an API key and secret. Here's how to generate them:
- Navigate to the "Settings" section in your Copper dashboard.
- Under "Integrations," select "API Keys."
- Click on "Create API Key" and provide a name for your key.
- Copy the generated API key and secret. Store them securely as you'll need them for authentication.
Configure OAuth for Copper API Access
Copper uses a custom authentication method, which requires setting up OAuth. Follow these steps:
- In the Copper dashboard, go to "Settings" and select "Integrations."
- Choose "OAuth Apps" and click "Create OAuth App."
- Fill in the required fields, including the redirect URL for your application.
- Save the app and note the client ID and client secret provided.
Test Your Copper API Connection
With your API key, secret, client ID, and client secret ready, you can now test your connection to the Copper API:
// Example PHP code to test Copper API connection
$apiKey = 'your_api_key';
$apiSecret = 'your_api_secret';
$clientId = 'your_client_id';
$clientSecret = 'your_client_secret';
// Set up the API endpoint and headers
$endpoint = 'https://api.copper.com/v1/leads';
$headers = [
'Authorization: Bearer ' . $apiKey,
'Content-Type: application/json'
];
// Initialize cURL session
$ch = curl_init($endpoint);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Execute the request
$response = curl_exec($ch);
curl_close($ch);
// Output the response
echo $response;
Replace the placeholders with your actual API credentials. This code snippet demonstrates how to make a basic API call to retrieve leads from Copper.
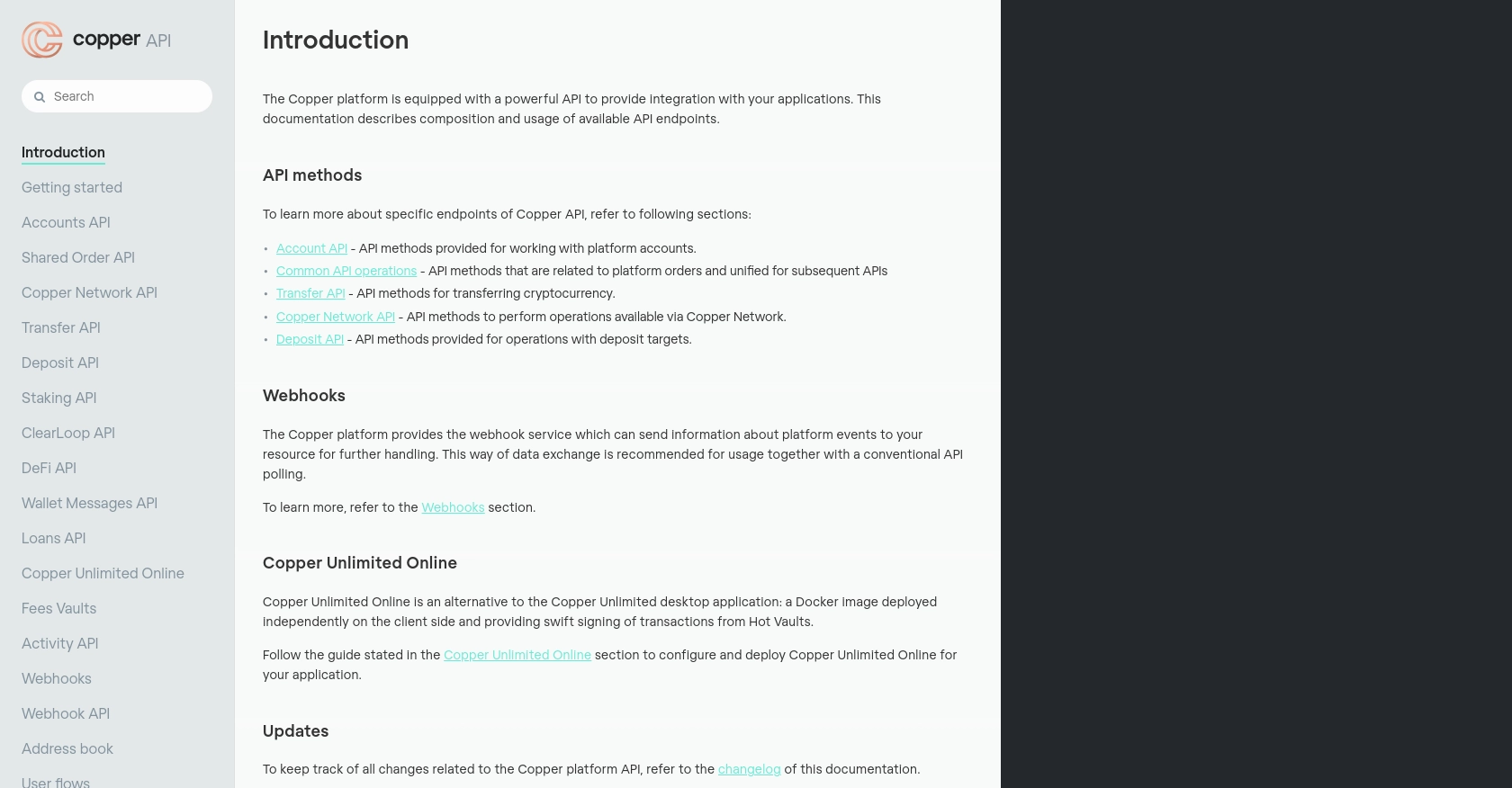
sbb-itb-96038d7
Making API Calls to Create or Update Leads with Copper API in PHP
Understanding PHP Requirements for Copper API Integration
To interact with the Copper API using PHP, ensure you have PHP 7.4 or later installed on your system. Additionally, you need the cURL extension enabled to make HTTP requests. You can verify this by running the following command:
php -m | grep curl
If cURL is not enabled, you can enable it by modifying your php.ini
file or installing it via your package manager.
Installing Necessary PHP Dependencies for Copper API
To streamline API requests, consider using a library like Guzzle, a popular PHP HTTP client. Install it via Composer:
composer require guzzlehttp/guzzle
Creating or Updating Leads with Copper API Using PHP
With your environment set up, you can now create or update leads in Copper. Here's a sample PHP script to demonstrate how to make an API call:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$apiKey = 'your_api_key';
$apiSecret = 'your_api_secret';
$clientId = 'your_client_id';
$clientSecret = 'your_client_secret';
// Set the API endpoint and headers
$endpoint = 'https://api.copper.com/v1/leads';
$headers = [
'Authorization' => 'Bearer ' . $apiKey,
'Content-Type' => 'application/json'
];
// Define lead data
$leadData = [
'name' => 'John Doe',
'email' => 'john.doe@example.com',
'company_name' => 'Example Corp'
];
// Make a POST request to create a new lead
$response = $client->post($endpoint, [
'headers' => $headers,
'json' => $leadData
]);
// Output the response
echo $response->getBody();
Replace the placeholders with your actual API credentials and lead information. This script demonstrates how to create a new lead in Copper using a POST request.
Handling Copper API Responses and Errors in PHP
After making an API call, it's crucial to handle the response and any potential errors. Here's how you can manage this in PHP:
// Check the response status code
$statusCode = $response->getStatusCode();
if ($statusCode == 201) {
echo "Lead created successfully!";
} else {
echo "Failed to create lead. Status code: " . $statusCode;
}
// Handle exceptions
try {
// Your API call here
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
By checking the status code and catching exceptions, you can ensure your application handles errors gracefully, providing useful feedback for debugging.
Verifying API Call Success in Copper Dashboard
To confirm that your API call succeeded, log into your Copper dashboard and navigate to the Leads section. You should see the newly created or updated lead listed there. This verification step ensures that your integration is functioning correctly.
Best Practices for Copper API Integration in PHP
When integrating with the Copper API, it's essential to follow best practices to ensure a secure and efficient implementation. Here are some key recommendations:
- Secure API Credentials: Store your API key, secret, client ID, and client secret securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Copper API has rate limits to prevent abuse. Implement logic to handle HTTP 429 errors by retrying requests after a delay. This ensures your application remains compliant with Copper's usage policies.
- Data Standardization: Ensure that data fields are consistent and standardized before sending them to the Copper API. This minimizes errors and ensures data integrity across your CRM system.
- Monitor API Usage: Regularly monitor your API usage and logs to identify any anomalies or issues. This proactive approach helps in maintaining a robust integration.
Enhancing Your CRM Integration with Endgrate
Integrating multiple platforms can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Copper. By leveraging Endgrate, you can:
- Save Time and Resources: Focus on your core product while Endgrate handles the intricacies of integration.
- Build Once, Deploy Everywhere: Create a single integration for each use case, reducing the need for multiple implementations.
- Enhance User Experience: Offer your customers a seamless and intuitive integration experience.
Visit Endgrate to learn more about how you can streamline your integration processes and enhance your CRM capabilities.
Read More
Ready to get started?