Using the Younium API to Get Payments in Javascript
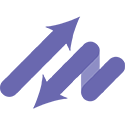
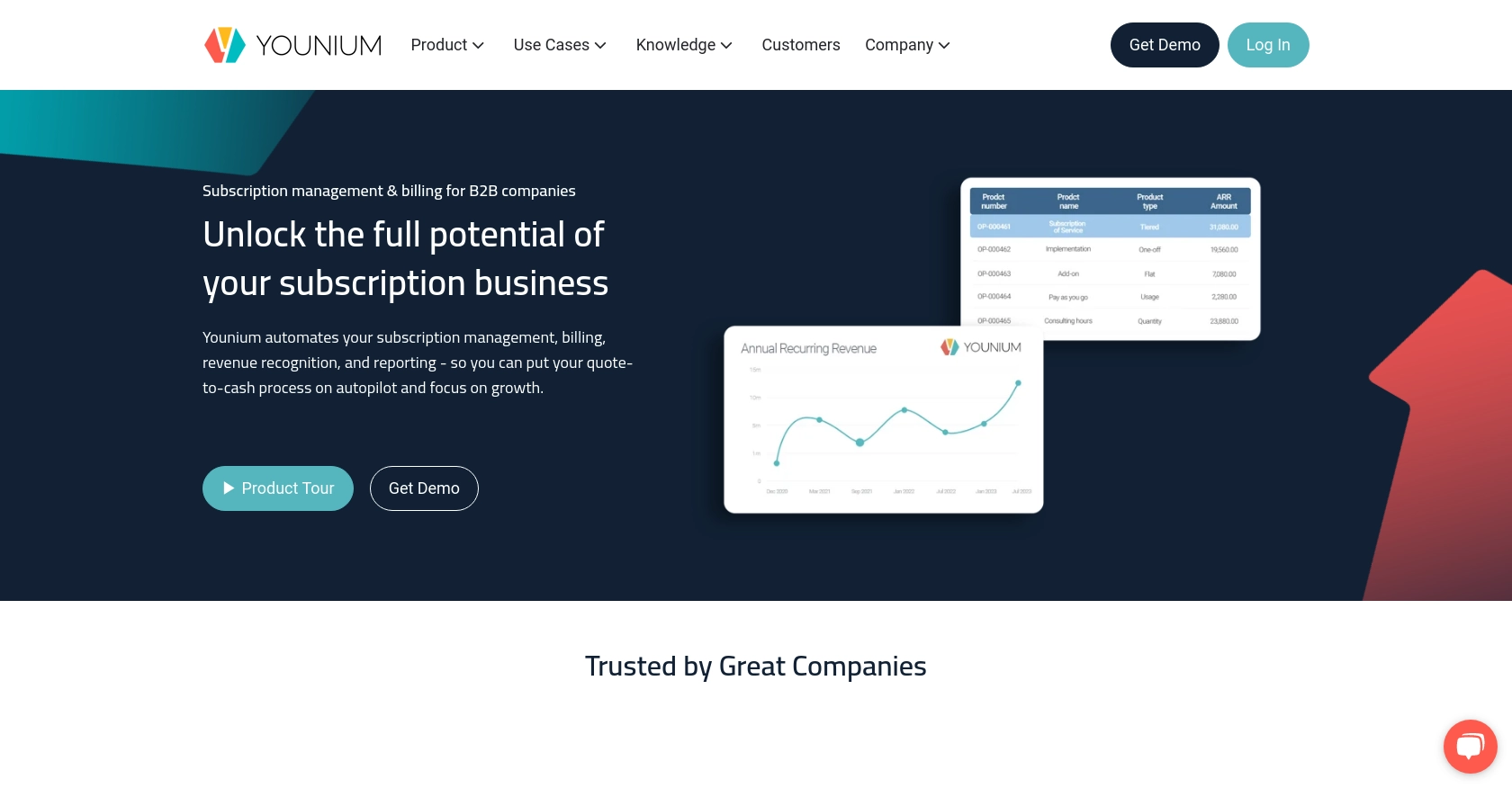
Introduction to Younium API Integration
Younium is a comprehensive subscription management platform designed to streamline billing and revenue operations for B2B SaaS companies. It offers a robust API that allows developers to integrate and automate various financial processes, enhancing efficiency and accuracy in managing subscriptions, invoices, and payments.
Integrating with the Younium API can be particularly beneficial for developers looking to automate payment retrieval processes. For example, by using the Younium API, developers can programmatically access payment data, enabling seamless financial reporting and analysis within their applications.
Setting Up Your Younium Sandbox Account for API Integration
Before diving into the Younium API, it's essential to set up a sandbox account. This environment allows developers to test API interactions without affecting live data, ensuring a smooth integration process.
Creating a Younium Sandbox Account
To begin, you need to create a sandbox account on Younium. This account will provide a safe space to experiment with API calls and understand how the platform operates.
- Visit the Younium Developer Portal.
- Sign up for a sandbox account by following the on-screen instructions.
- Once registered, log in to your sandbox account to access the dashboard.
Generating API Tokens and Client Credentials
Authentication with the Younium API requires a JWT access token. Follow these steps to generate the necessary credentials:
- Navigate to your user profile by clicking your name in the top right corner.
- Select “Privacy & Security” from the dropdown menu.
- Click on “Personal Tokens” in the left panel and then “Generate Token.”
- Provide a relevant description for the token and click “Create.”
- Copy the generated Client ID and Secret Key immediately, as they will not be visible again.
Acquiring a JWT Access Token
With your client credentials ready, you can now obtain a JWT access token:
// Example POST request to acquire JWT token
const fetch = require('node-fetch');
const getToken = async () => {
const response = await fetch('https://api.sandbox.younium.com/auth/token', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({
clientId: 'Your_Client_ID',
secret: 'Your_Secret_Key'
})
});
const data = await response.json();
console.log('Access Token:', data.accessToken);
};
getToken();
Replace Your_Client_ID
and Your_Secret_Key
with the credentials you generated earlier. This script will return an access token, valid for 24 hours, which you will use to authenticate API requests.
For more details on authentication, refer to the Younium Authentication Guide.
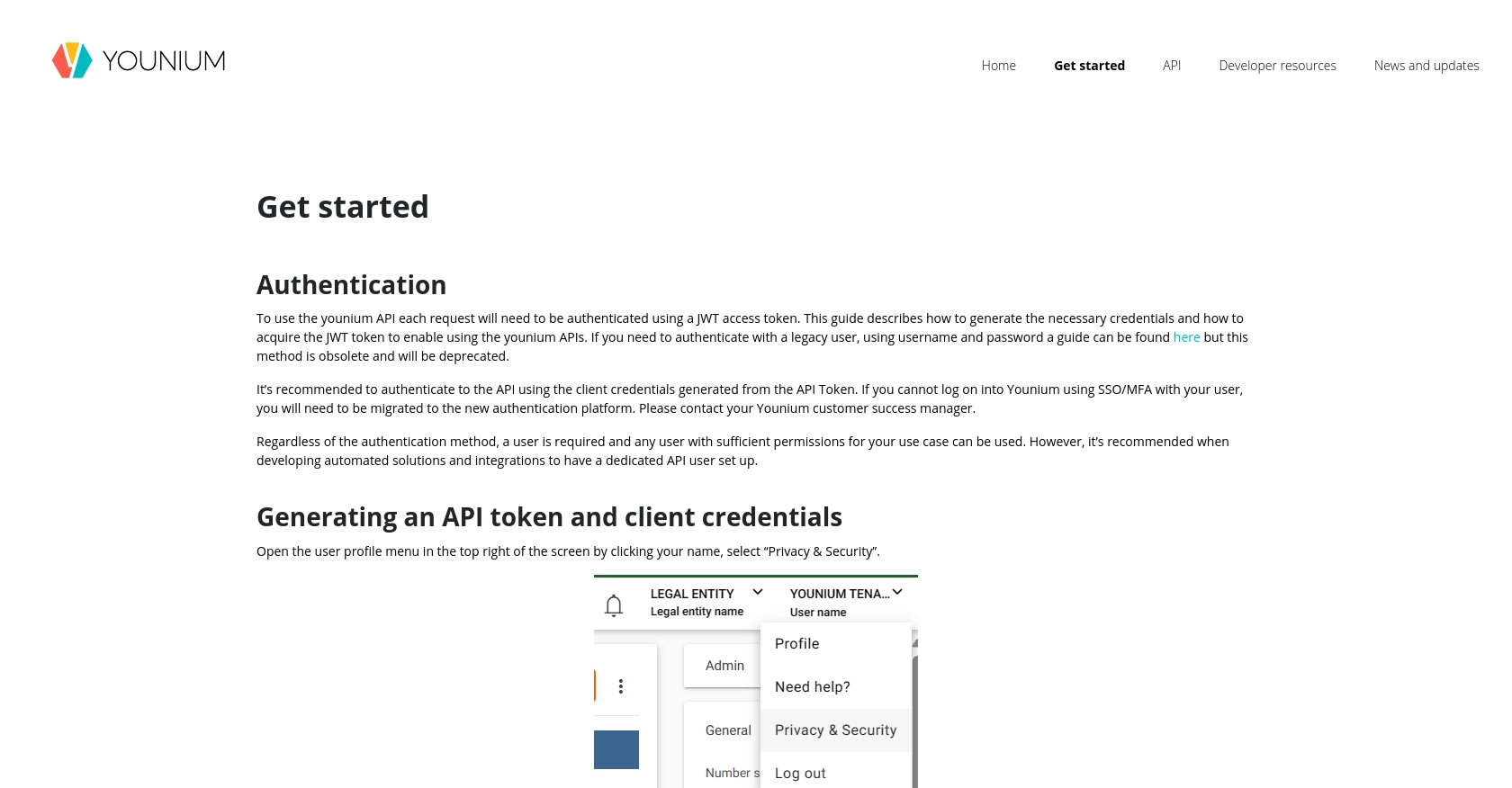
sbb-itb-96038d7
Making API Calls to Retrieve Payments Using Younium API in JavaScript
To interact with the Younium API and retrieve payment data, you'll need to make authenticated API calls using JavaScript. This section will guide you through the process of setting up your environment, writing the necessary code, and handling potential errors.
Setting Up Your JavaScript Environment for Younium API Integration
Before making API calls, ensure you have the following prerequisites installed:
- Node.js (version 14.x or higher)
- npm (Node Package Manager)
Once you have Node.js and npm installed, you can use the node-fetch
library to make HTTP requests. Install it by running the following command in your terminal:
npm install node-fetch
Writing JavaScript Code to Fetch Payments from Younium API
With your environment set up, you can now write the JavaScript code to fetch payment data from the Younium API. Create a file named getPayments.js
and add the following code:
const fetch = require('node-fetch');
const getPayments = async (accessToken) => {
const response = await fetch('https://api.sandbox.younium.com/payments', {
method: 'GET',
headers: {
'Authorization': `Bearer ${accessToken}`,
'Content-Type': 'application/json',
'api-version': '2.1'
}
});
if (response.ok) {
const data = await response.json();
console.log('Payments:', data);
} else {
console.error('Error fetching payments:', response.status, response.statusText);
}
};
// Replace 'Your_Access_Token' with the JWT token obtained earlier
getPayments('Your_Access_Token');
Replace Your_Access_Token
with the JWT token you obtained in the previous section. This script sends a GET request to the Younium API to retrieve payment data and logs the response to the console.
Verifying Successful API Requests and Handling Errors
After running the script, you should see the payment data output in your console. If the request is successful, the data will match the payments available in your Younium sandbox account.
In case of errors, the script will log the HTTP status code and status text. Common error codes include:
- 401 Unauthorized: Indicates an expired or invalid access token. Ensure your token is correct and not expired.
- 403 Forbidden: Occurs if the legal entity specified in the headers is incorrect or if the user lacks necessary permissions.
For more details on error handling, refer to the Younium API Documentation.
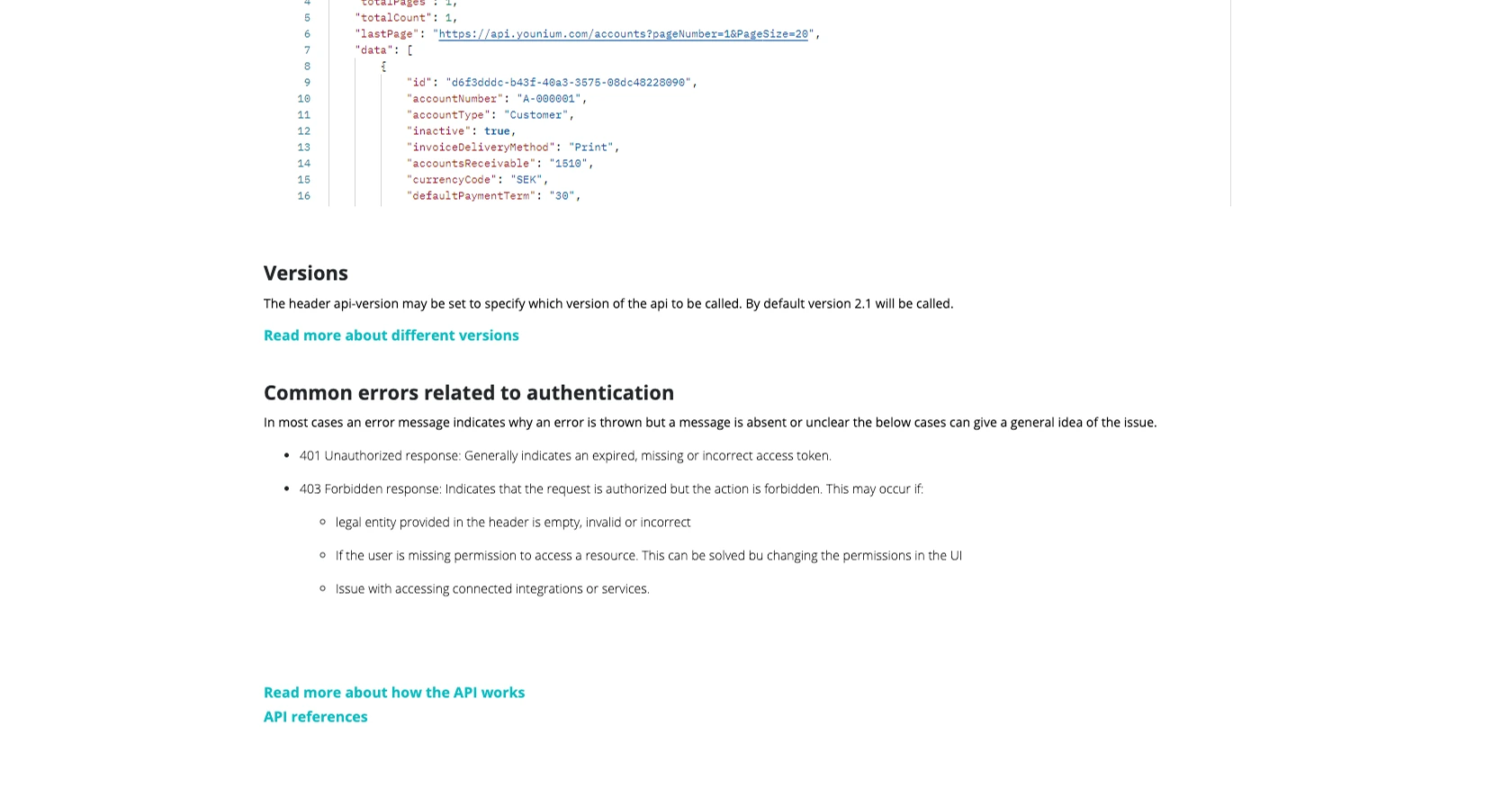
Conclusion and Best Practices for Younium API Integration in JavaScript
Integrating with the Younium API to retrieve payment data using JavaScript can significantly enhance your application's financial management capabilities. By automating the retrieval of payment information, you can streamline reporting and analysis processes, ultimately improving operational efficiency.
Best Practices for Secure and Efficient Younium API Usage
- Secure Storage of Credentials: Always store your Client ID, Secret Key, and JWT access tokens securely. Consider using environment variables or a secure vault to prevent unauthorized access.
- Handling Rate Limits: Be mindful of the API rate limits to avoid throttling. Implement exponential backoff strategies for retrying requests when encountering rate limit errors.
- Data Transformation and Standardization: Ensure that the data retrieved from the Younium API is transformed and standardized to fit your application's data model. This will facilitate seamless integration and data consistency.
- Regular Token Refresh: Since the JWT access token is valid for 24 hours, implement a mechanism to refresh the token automatically to maintain uninterrupted access to the API.
Streamlining Integrations with Endgrate
While integrating with the Younium API can be straightforward, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process across various platforms, including Younium.
By leveraging Endgrate, you can focus on your core product development while outsourcing the intricacies of integration management. This approach not only saves time and resources but also provides an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website and discover the benefits of a streamlined, efficient integration process.
Read More
Ready to get started?