Using the Applied Epic API to Get People in PHP
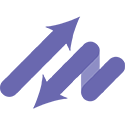
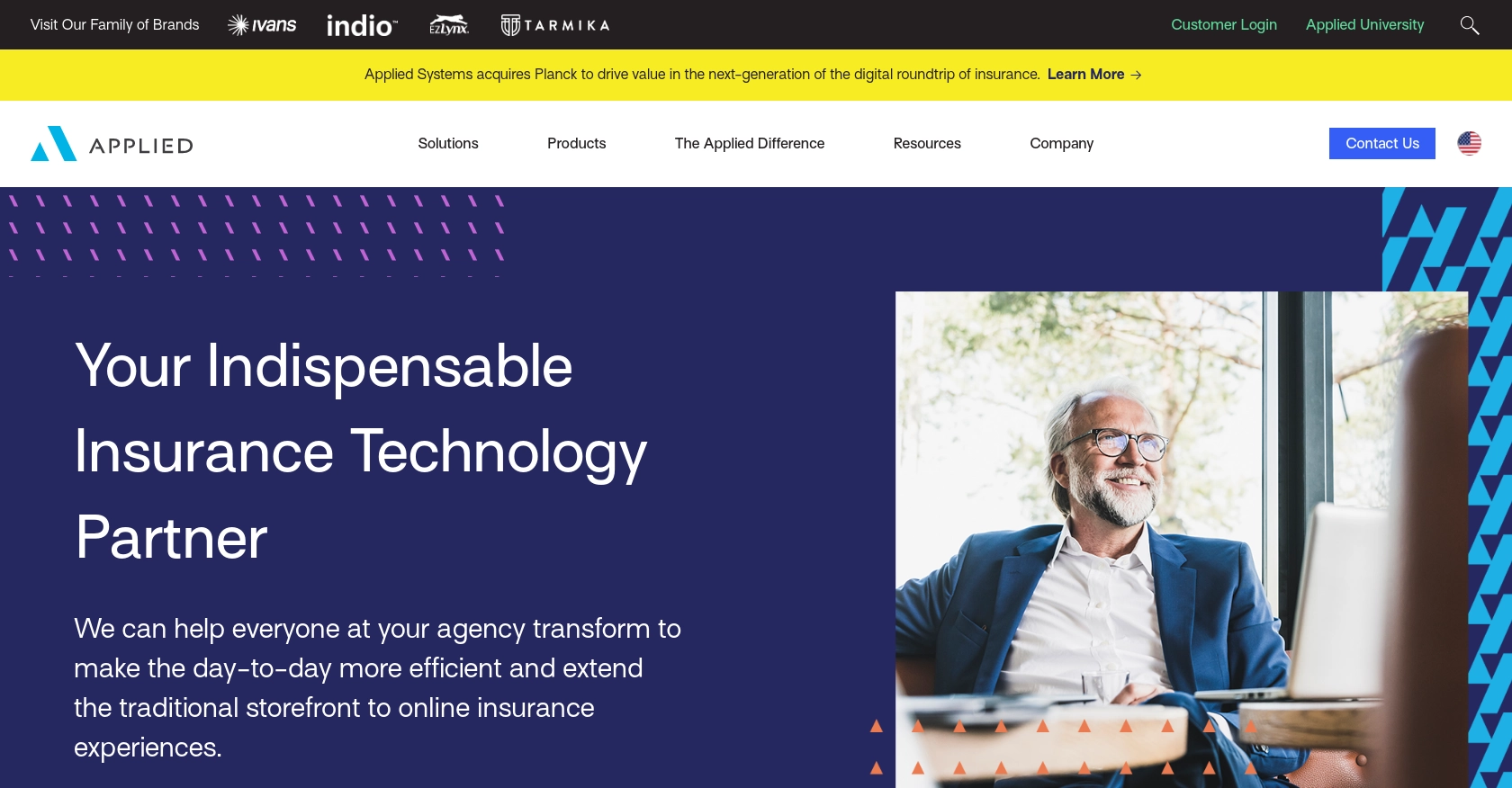
Introduction to Applied Epic API
Applied Epic is a comprehensive insurance management platform that streamlines operations for insurance agencies and brokerages. It offers a unified solution for managing client data, policies, and claims, enhancing efficiency and client service.
Integrating with the Applied Epic API allows developers to access and manage client-related data programmatically. For example, you might want to retrieve a list of people associated with an account to automate client communications or update records in your internal systems.
Setting Up Your Applied Epic Test/Sandbox Account
Before diving into the Applied Epic API, you need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data.
Creating an Applied Epic Developer Account
To begin, you'll need to sign up for an Applied Epic developer account. Follow these steps:
- Visit the Applied Epic Developer Portal.
- Complete the registration form with your details.
- Verify your email address to activate your account.
Generating API Credentials
Once your developer account is set up, you need to generate API credentials to authenticate your requests:
- Log in to the Applied Epic Developer Portal.
- Navigate to the "API Credentials" section.
- Create a new application to obtain your client ID and client secret.
Understanding Custom Authentication
The Applied Epic API uses a custom authentication method. Ensure you have your client ID and client secret ready for the next steps. These credentials will be used to obtain an access token for API requests.
Setting Up OAuth for Applied Epic API
To authenticate using OAuth, follow these steps:
- Use your client ID and client secret to request an access token from the OAuth endpoint.
- Include the access token in the Authorization header of your API requests.
For detailed instructions, refer to the Applied Epic API Documentation.
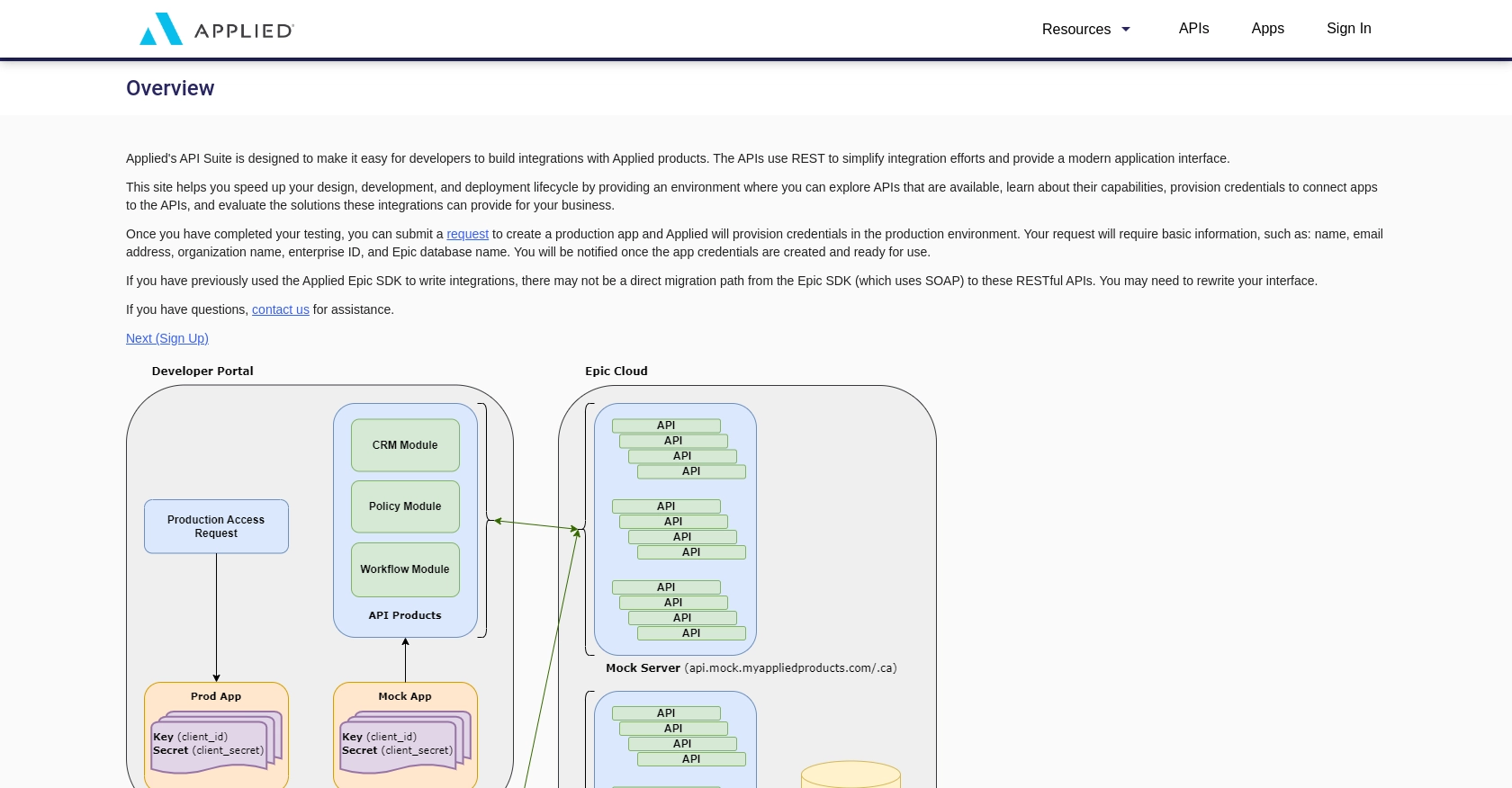
sbb-itb-96038d7
Making API Calls to Retrieve People Data from Applied Epic Using PHP
To interact with the Applied Epic API and retrieve a list of people, you'll need to set up your PHP environment and make HTTP requests. This section will guide you through the necessary steps, including setting up PHP, installing dependencies, and executing the API call.
Setting Up Your PHP Environment
Before making API calls, ensure your development environment is ready:
- Install PHP 7.4 or later on your machine.
- Ensure you have Composer installed for managing dependencies.
Installing Required PHP Libraries
To make HTTP requests, you'll need the Guzzle HTTP client. Install it using Composer:
composer require guzzlehttp/guzzle
Writing PHP Code to Call the Applied Epic API
Create a PHP script to retrieve people data from the Applied Epic API. Follow the example below:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'Your_Access_Token'; // Replace with your actual access token
try {
$response = $client->request('GET', 'https://api.myappliedproducts.com/crm/v1/people', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Accept' => 'application/hal+json',
],
'query' => [
'limit' => 5,
'offset' => 0,
],
]);
$data = json_decode($response->getBody(), true);
foreach ($data['_embedded']['people'] as $person) {
echo 'ID: ' . $person['id'] . "\n";
echo 'Name: ' . $person['firstName'] . ' ' . $person['lastName'] . "\n";
echo 'Date of Birth: ' . $person['dateOfBirth'] . "\n\n";
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Understanding the API Response
The API returns a JSON response containing a list of people. The example above parses this response and prints each person's ID, name, and date of birth. Ensure your access token is valid and included in the request headers.
Handling Errors and Verifying API Requests
Check the response status code to verify the success of your API call. A status code of 200 indicates success, while codes like 400, 401, and 403 indicate errors:
- 400 Bad Request: Check your request parameters for errors.
- 401 Unauthorized: Verify your access token.
- 403 Forbidden: Ensure you have the necessary permissions.
For more details, refer to the Applied Epic API Documentation.
Conclusion and Best Practices for Using Applied Epic API in PHP
Integrating with the Applied Epic API can significantly enhance your ability to manage client data efficiently within your insurance management systems. By following the steps outlined in this guide, you can successfully retrieve and handle people data using PHP, streamlining your operations and improving client interactions.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the API. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that data retrieved from the API is standardized and transformed as needed to fit your internal systems. This helps maintain consistency and accuracy across platforms.
Streamlining Integrations with Endgrate
If managing multiple integrations is becoming a challenge, consider using Endgrate to simplify the process. Endgrate provides a unified API endpoint that connects to various platforms, including Applied Epic, allowing you to focus on your core product while outsourcing integration complexities.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Visit Endgrate to learn more about how it can help you save time and resources.
Read More
Ready to get started?