How to Get Page Views with the Pendo API in Python
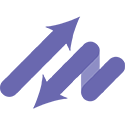
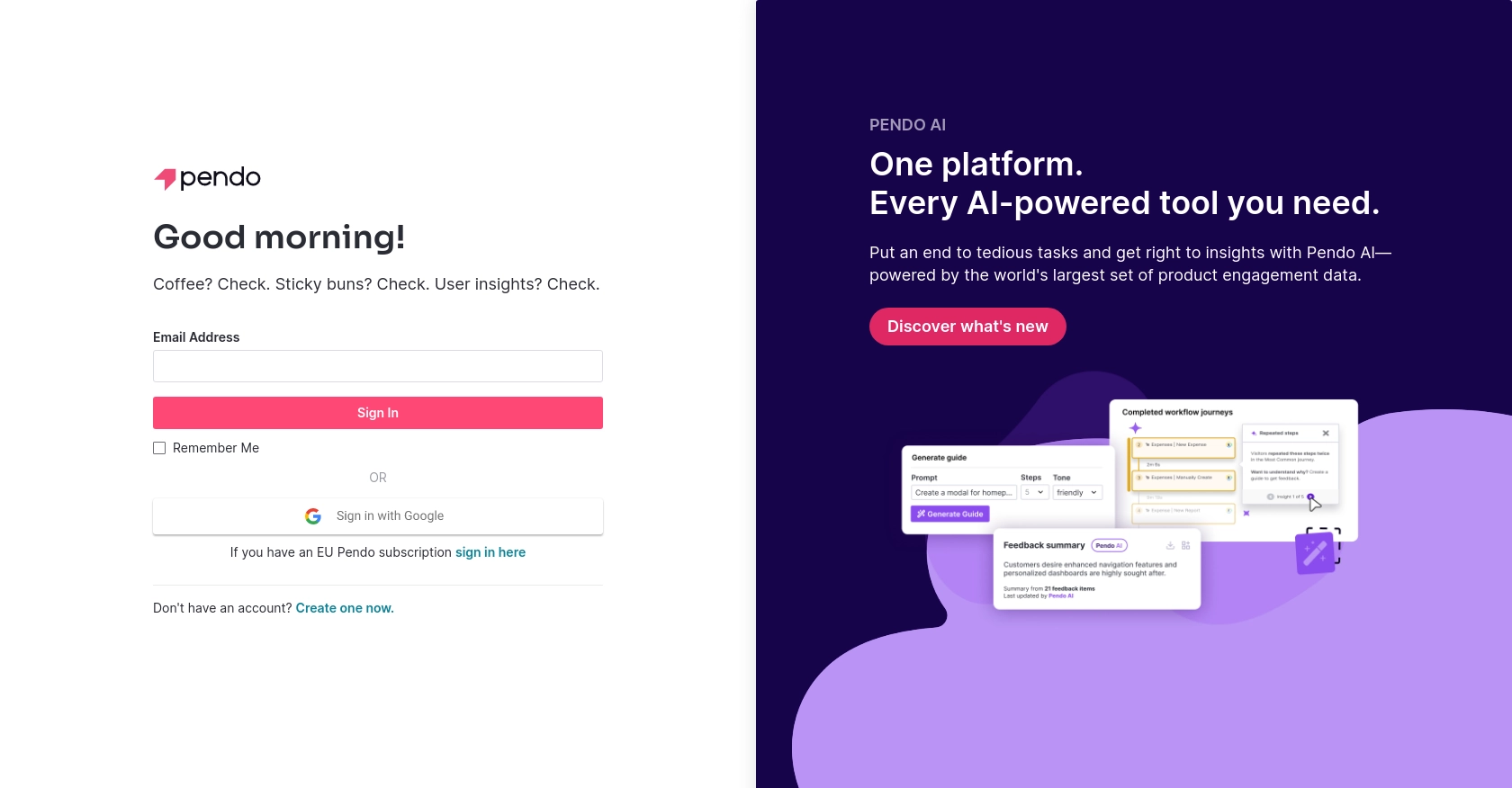
Introduction to Pendo API
Pendo is a powerful platform that provides product teams with insights into user behavior, helping them to create more engaging and effective software experiences. With features such as in-app messaging, user feedback, and detailed analytics, Pendo empowers businesses to understand and improve their digital products.
Integrating with Pendo's API allows developers to access valuable data, such as page views, which can be used to analyze user interactions and optimize product performance. For example, a developer might want to retrieve page view data to identify the most frequently visited pages in an application, enabling targeted enhancements and improved user engagement.
Setting Up Your Pendo Test Account for API Access
Before you can start using the Pendo API to retrieve page view data, you need to set up a Pendo account. This will allow you to generate the necessary API key for authentication. Follow these steps to get started:
Step 1: Sign Up for a Pendo Account
If you don't already have a Pendo account, visit the Pendo website and sign up for a free trial or a demo account. This will give you access to the platform's features and allow you to explore its capabilities.
Step 2: Accessing the API Key
Once your account is set up, log in to the Pendo dashboard. Navigate to the settings section where you can manage your account details. Look for the API key management area to generate a new API key. This key will be used to authenticate your API requests.
Step 3: Generate and Secure Your API Key
- Click on the option to create a new API key.
- Provide a name for your API key to help you identify its purpose later.
- Once generated, copy the API key and store it securely. You will need this key to authenticate your API requests in the Python code.
With your API key ready, you can now proceed to make API calls to Pendo and retrieve page view data. Ensure that your API key is kept confidential and not exposed in your code or shared publicly.
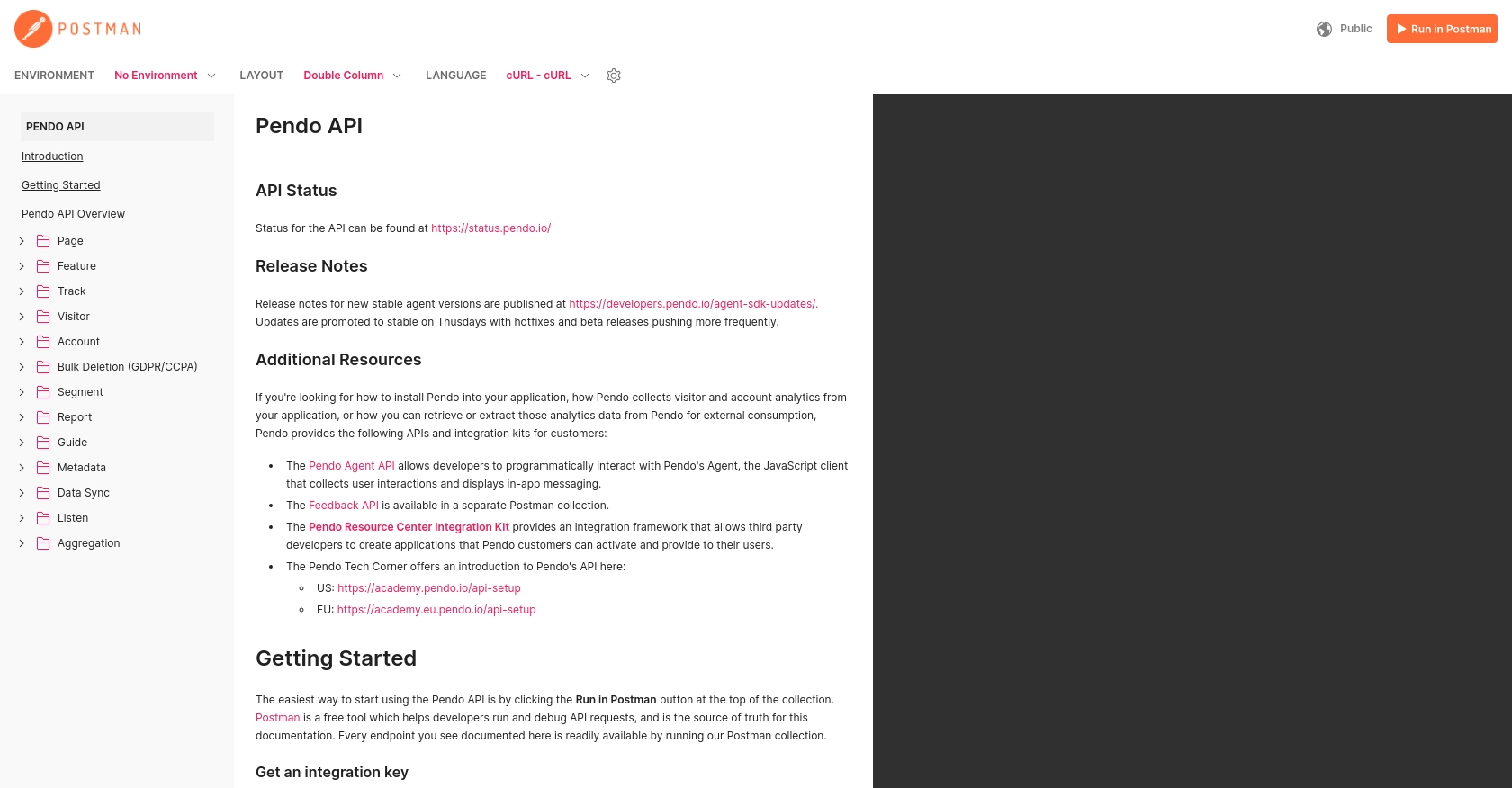
sbb-itb-96038d7
Making API Calls to Retrieve Page Views with Pendo API in Python
To effectively interact with the Pendo API and retrieve page view data, you'll need to use Python, a versatile programming language known for its simplicity and readability. This section will guide you through the process of setting up your Python environment, making the API call, and handling potential errors.
Setting Up Your Python Environment for Pendo API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1
- The Python package installer, pip
Once these are installed, open your terminal or command prompt and install the requests
library, which will help you make HTTP requests:
pip install requests
Writing Python Code to Retrieve Page Views from Pendo API
With your environment set up, you can now write the Python code to interact with the Pendo API. Create a file named get_pendo_page_views.py
and add the following code:
import requests
# Set the API endpoint and headers
endpoint = "https://engageapi.pendo.io/api/v1/pageViews"
headers = {
"Content-Type": "application/json",
"x-pendo-integration-key": "Your_API_Key"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
data = response.json()
# Process and print the page view data
for page_view in data.get("views", []):
print(f"Page ID: {page_view['id']}, Views: {page_view['count']}")
else:
print(f"Failed to retrieve data: {response.status_code} - {response.text}")
Replace Your_API_Key
with the API key you generated earlier. This script sends a GET request to the Pendo API endpoint for page views, processes the JSON response, and prints out the page view data.
Verifying Successful API Requests and Handling Errors
After running the script, you should see the page view data printed in your terminal. If the request fails, the script will output the error code and message. Common error codes include:
- 401 Unauthorized: Check if your API key is correct and has the necessary permissions.
- 404 Not Found: Ensure the endpoint URL is correct.
- 500 Internal Server Error: This indicates a problem with the Pendo server; try again later.
For more detailed information on error codes, refer to the Pendo API documentation.
Conclusion and Best Practices for Using Pendo API in Python
Integrating with the Pendo API to retrieve page view data can significantly enhance your ability to analyze user interactions and optimize your digital products. By following the steps outlined in this guide, you can efficiently set up your Python environment, authenticate your API requests, and handle potential errors.
Best Practices for Secure and Efficient API Integration with Pendo
- Secure API Key Storage: Always store your API keys securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of Pendo's rate limits to avoid throttling. Implement retry logic with exponential backoff to manage rate limit errors gracefully.
- Data Transformation: Consider transforming and standardizing the retrieved data to fit your application's needs, ensuring consistency across different data sources.
Streamline Your Integration Process with Endgrate
While integrating with Pendo is a valuable endeavor, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process across various platforms, including Pendo. By leveraging Endgrate, you can focus on your core product development while ensuring a seamless integration experience for your customers.
Visit Endgrate to learn more about how you can save time and resources by outsourcing your integration needs and building once for each use case instead of multiple times for different platforms.
Read More
Ready to get started?