Role-Based Access Control for APIs: Implementation Guide
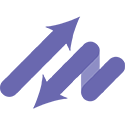
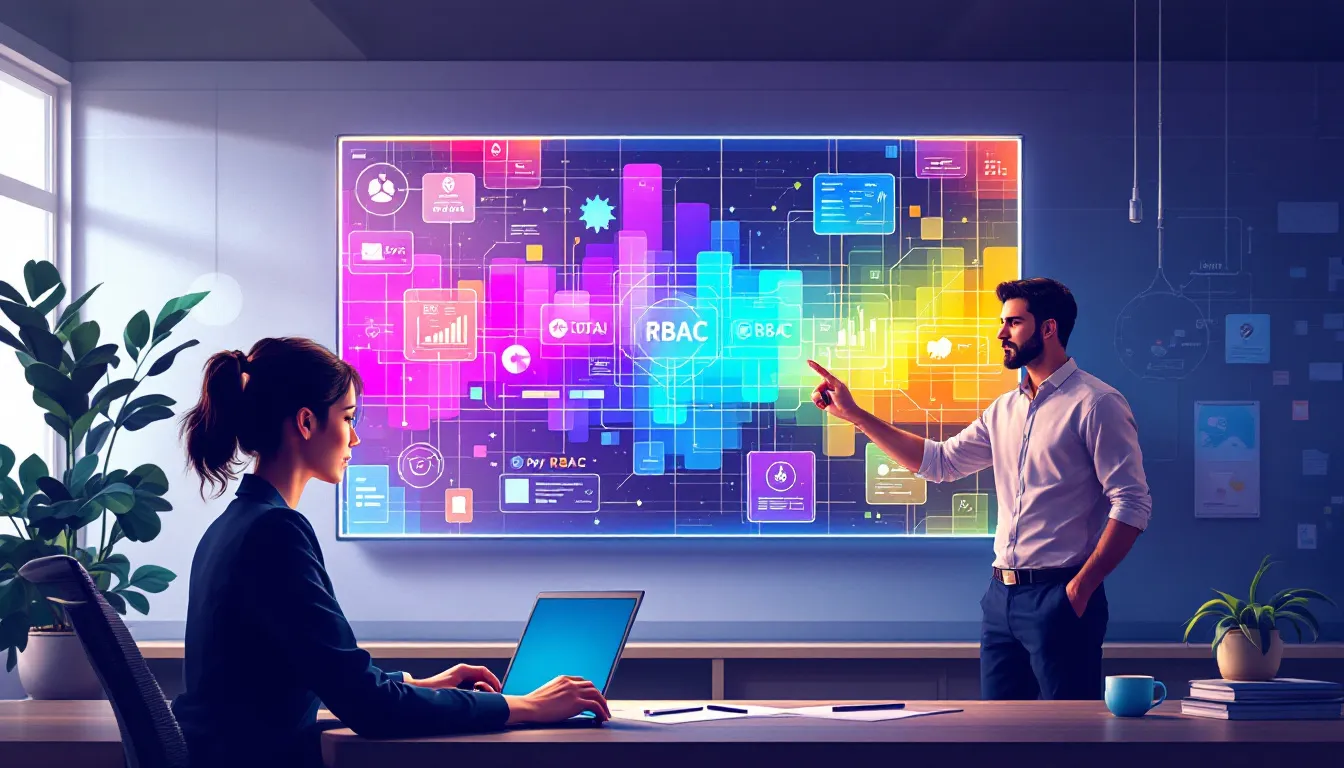
Want to secure your API with role-based access control (RBAC)? Here's a quick guide:
- Plan your RBAC structure
- Set up your environment
- Implement RBAC in your API
- Secure API endpoints
- Test and refine
Key benefits of RBAC for APIs:
- Simplifies access management
- Enhances security
- Scales for organizations of all sizes
Here's how to implement RBAC in your API:
- Go to Dashboard > Applications > APIs
- Select your API
- Find RBAC Settings
- Enable RBAC
- Turn on "Add Permissions in the Access Token"
- Save changes
This adds user permissions to access tokens, enabling role-based control in your API.
Remember: RBAC needs ongoing maintenance. Audit regularly, follow least privilege, update roles as needed, and keep your team informed about security practices.
Role | Example Permissions |
---|---|
Admin | Manage users, Delete products |
Manager | View reports, Edit product info |
Editor | Create/edit content, Moderate comments |
Viewer | View products, Read reviews |
By implementing RBAC, you'll have a solid foundation for managing API access based on user roles.
Related video from YouTube
Key parts of RBAC
RBAC for APIs has four main parts:
Users and identities
Users are people or systems needing API access. Each has a unique identity:
- Username/password
- API key
- OAuth token
Keep user data secure. Remove old accounts fast.
Roles and permissions
Roles group permissions. Permissions are allowed actions on resources.
Example:
Role | Permissions |
---|---|
Reader | View products, Read reviews |
Writer | Create products, Edit descriptions |
Admin | Delete products, Manage users |
Assign roles based on job needs. Give only necessary access.
Resources and actions
Resources are API data or functions. Actions are operations on resources.
Common API actions:
- GET (read)
- POST (create)
- PUT (update)
- DELETE (remove)
Link permissions to resource-action pairs. "Edit-product" allows PUT to /products.
Access rules
Access rules connect everything. They define who can do what to which resources.
Example: "Writers can edit product descriptions, not delete products."
Implement these in API code or through an authorization service.
Planning RBAC for your API
Let's break down RBAC planning for your API into simple steps:
Check API security needs
First, assess your API's security requirements:
- What resources need protection?
- How much security does each resource need?
- Any compliance rules to follow?
List user roles
Define your system's user roles:
Role | Description | Example Permissions |
---|---|---|
Admin | Full access | Manage users, Delete products |
Manager | Operations oversight | View reports, Edit product info |
Editor | Content management | Create/edit content, Moderate comments |
Viewer | Read-only | View products, Read reviews |
Link permissions to roles
Connect specific permissions to each role:
1. List all possible actions on resources
2. Assign actions to roles based on job needs
3. Give only necessary access
Example:
Role: Editor
Permissions:
- Create new blog posts
- Edit existing blog posts
- Moderate comments
- View site analytics
Create a role structure
Organize your roles clearly:
- Need role hierarchies?
- Use role inheritance?
- Plan for role changes
Keep your RBAC system flexible. You might need to adjust as your API grows.
Set up your work environment
To implement RBAC for your API, you need the right tools and setup. Here's how:
Pick an API framework
Choose a framework with built-in RBAC support. Some popular options:
Framework | Language | RBAC Support |
---|---|---|
Express.js | JavaScript | Yes, with middleware |
Django | Python | Built-in |
ASP.NET Core | C# | Native support |
Laravel | PHP | Includes RBAC features |
For ASP.NET Core, create a new project:
dotnet new webapi --auth SingleOrg --client-id <YOUR-CLIENT-ID> --tenant-id <TENANT-ID>
This sets up a web API project ready for RBAC.
Install needed tools
You'll need:
- Version control (Git)
- Package manager
- API testing tool
- Database management system
For ASP.NET Core, add these packages:
dotnet add package Microsoft.Identity.Web
dotnet add package Microsoft.Identity.Web.UI
Set up your test server
- Pick a server (local or cloud)
- Install your framework
- Set up a database for users and roles
- Configure HTTPS
For a local ASP.NET Core setup:
- Install .NET SDK
- Run
dotnet run
- Access your API at
https://localhost:5001
Add RBAC to your API
Let's add RBAC to your API. We'll create a user model, set up roles and permissions, assign roles to users, and implement a login system.
User Model
For an ASP.NET Core app, use the IdentityUser
class:
public class ApplicationUser : IdentityUser
{
public string Role { get; set; }
}
Roles and Permissions
Define roles in a roles.json
file:
{
"roles": [
{
"name": "Admin",
"permissions": ["create_record", "read_record", "update_record", "delete_record"]
},
{
"name": "Manager",
"permissions": ["create_record", "read_record", "update_record"]
},
{
"name": "Employee",
"permissions": ["create_record", "read_record"]
}
]
}
Role Assignment
Here's how to assign roles during registration:
[HttpPost("register")]
public async Task<IActionResult> Register(RegisterModel model)
{
var user = new ApplicationUser { UserName = model.Email, Email = model.Email, Role = model.Role };
var result = await _userManager.CreateAsync(user, model.Password);
if (result.Succeeded)
{
await _userManager.AddToRoleAsync(user, model.Role);
return Ok(new { message = "User registered successfully" });
}
return BadRequest(result.Errors);
}
Login System
Implement a JWT-based login system:
[HttpPost("login")]
public async Task<IActionResult> Login(LoginModel model)
{
var user = await _userManager.FindByEmailAsync(model.Email);
if (user != null && await _userManager.CheckPasswordAsync(user, model.Password))
{
var tokenHandler = new JwtSecurityTokenHandler();
var key = Encoding.ASCII.GetBytes(_configuration["Jwt:Key"]);
var tokenDescriptor = new SecurityTokenDescriptor
{
Subject = new ClaimsIdentity(new Claim[]
{
new Claim(ClaimTypes.Name, user.Id.ToString()),
new Claim(ClaimTypes.Role, user.Role)
}),
Expires = DateTime.UtcNow.AddDays(7),
SigningCredentials = new SigningCredentials(new SymmetricSecurityKey(key), SecurityAlgorithms.HmacSha256Signature)
};
var token = tokenHandler.CreateToken(tokenDescriptor);
return Ok(new { token = tokenHandler.WriteToken(token) });
}
return Unauthorized();
}
Secure your endpoints using the [Authorize]
attribute:
[Authorize(Roles = "Admin")]
[HttpGet("users")]
public IActionResult GetAllUsers()
{
// Implementation
}
That's it! You've added basic RBAC to your API. This setup gives you a solid foundation for managing access control based on user roles.
Protect API endpoints
Here's how to secure your API endpoints with Role-Based Access Control (RBAC):
Use middleware for role checks
Middleware can automatically check user roles before allowing API access. Here's an Express example:
function checkPermissionJson(scope_required) {
return (req, res, next) => {
const user = req.user || {};
const scopes = ['view: public'].concat(user.permissions || user.scopes || []);
if (scopes.includes(scope_required)) return next();
res.status(400).json({error: `Need ${scope_required}; have ${scopes.join(',')}`});
}
}
app.get('/api/admin', checkPermissionJson('admin'), (req, res) => {
// Admin-only code here
});
Add RBAC to route handlers
For finer control, add RBAC checks in your route handlers:
app.post('/api/orders', (req, res) => {
if (!req.user.permissions.includes('create:order')) {
return res.status(403).json({error: "Can't create orders"});
}
// Create order code
});
Handle wrong access attempts
Gracefully handle unauthorized access:
app.use((err, req, res, next) => {
if (err.name === 'UnauthorizedError') {
return res.status(401).json({
error: "Access denied",
details: "Missing permissions"
});
}
next(err);
});
This catches unauthorized attempts and returns a clear error message.
sbb-itb-96038d7
Test your RBAC setup
Testing your RBAC setup is key. Here's how to do it right:
Test each role assignment
Check if roles work as they should. Write unit tests for your API methods and controllers. Here's a C# example:
[Test]
[TestCase("Put", new []{"Admin","TeamMember"})]
[TestCase("Post", new []{"Admin","TeamMember"})]
[TestCase("Get", new []{"TeamMember"})]
public void Ensure_Methods_have_Correct_Roles(string methodToTest, List<string> roles) {
var controller = new myController();
Assert.IsTrue(controller.HasRoles(methodToTest, roles));
}
This test checks if HTTP methods have the right roles.
Test protected endpoints
Make sure your API endpoints are locked down. Use Postman or write tests to hit protected endpoints with different roles. Look for 401 or 403 responses when access is denied.
Test an admin-only endpoint like this:
const response = await fetch('/api/admin', {
headers: { 'Authorization': 'Bearer USER_TOKEN' }
});
assert.equal(response.status, 403, "Non-admin user should be forbidden");
Test different user roles
Try out all user roles to cover your bases. Create a test matrix for all roles and endpoints. Here's how:
1. Create test users for each role
Set up test accounts for all roles (Admin, Student, Faculty, etc.).
2. Define expected access for each role
Make a table showing which endpoints each role can access:
Endpoint | Admin | Student | Faculty |
---|---|---|---|
/api/users | Yes | No | No |
/api/courses | Yes | Yes | Yes |
/api/grades | Yes | No | Yes |
3. Automate role-based tests
Write scripts to test each endpoint with every role. Compare results to your access table.
4. Check for edge cases
Test tricky scenarios like users with multiple roles, nested permissions, and time-based restrictions.
Tips for managing RBAC
Managing RBAC for APIs isn't a set-it-and-forget-it task. Here's how to keep your system tight and effective:
Check roles and permissions often
Don't let your RBAC setup gather dust. Set a regular schedule to review roles and permissions. Are they still in line with what your business needs? Atlassian suggests doing this every quarter.
Pro tip: Use tools that do some of the heavy lifting for you. Auth0's RBAC feature, for example, flags unused roles or permissions. It's like having a digital assistant point out where you might be over-permissioning.
Give least needed access
Think of permissions like seasoning - use just enough, not too much. Here's a simple way to do it:
- List out all job functions
- Figure out which API endpoints each function needs
- Create roles that match these needs
- Assign users to roles based on their job
For example, your customer support team might only need to read user data, while your billing folks need to read AND write payment info.
Update roles when needed
Your business changes, and your RBAC should too. Don't wait for problems to pop up - be proactive about updating roles.
Set up a clear process:
- How can someone request a role change?
- Who needs to approve it?
- Document the changes and why they're happening
- Test in a safe environment first
- Let people know what's changing
And here's a crucial point: when someone leaves or switches jobs, update their access ASAP. IBM found that 19% of data breaches come from old login info, often from ex-employees. Don't let that be you.
RBAC for bigger apps
As your API grows, managing access gets tricky. Here's how to scale RBAC for larger apps:
Use role levels
Set up a role hierarchy:
1. Create role levels
Use a tiered structure:
Level | Description | Example Roles |
---|---|---|
1 | Basic access | Viewer, Reader |
2 | Standard ops | Editor, Contributor |
3 | Advanced functions | Manager, Admin |
4 | System-wide control | Super Admin |
2. Inherit permissions
Higher-level roles get lower-level permissions automatically. Less redundancy, easier updates.
3. Assign roles smart
Give users the lowest level role they need. It's safer.
Mix in attribute-based access
Combine RBAC with Attribute-Based Access Control (ABAC):
- Define user and resource attributes.
- Create rules using these attributes.
- Use both roles and attributes for access decisions.
Example: A "Manager" might have different permissions based on their department.
Try policy as code
Keep access rules separate from your main code:
- Write policies in a special language (like Rego for OPA).
- Store policies in version control.
- Use a policy engine to enforce rules.
This makes it easier to check, update, and test your access control.
"Policy as code lets us handle complex authorization rules better. We can version, review, and test our policies just like other code", says Emily Roth, Security Architect at Acme Corp.
Fix common RBAC problems
RBAC can be a headache. Let's tackle some common issues:
Fix permission conflicts
When roles clash, it's a mess. Here's how to clean it up:
1. Audit your roles
Look for overlapping permissions. They're often the culprits.
2. Use a permission matrix
A simple table can work wonders:
Role | Create Order | Approve Order | View Reports |
---|---|---|---|
Sales Rep | Yes | No | Limited |
Manager | Yes | Yes | Full |
Accountant | No | No | Full |
3. Implement role hierarchy
Set it up so higher roles inherit from lower ones. It's cleaner that way.
4. Stick to least privilege
Only give users what they absolutely need. No extras.
Solve role loop issues
Role loops are a nightmare. Here's how to wake up from it:
1. Map out role relationships
Draw it out. You'll spot those pesky circles.
2. Break the loop
Restructure. Get rid of those circular dependencies.
3. Use role composition
Build roles from smaller permission sets. It's like LEGO for access control.
4. Try ACLs
For the complex stuff, ACLs give you fine-grained control.
Speed up slow RBAC systems
Is your RBAC crawling? Let's make it run:
1. Optimize database queries
Index. Cache. Your database will thank you.
2. Implement sharding
Split those policy rules. Here's how with Casbin:
e = casbin.Enforcer("model.conf", "policy.csv")
e.load_filtered_policy(filter={"p": ["alice", "data1", "read"]})
3. Use multi-threading
Make Casbin use all your cores:
from casbin import ThreadedEnforcer
e = ThreadedEnforcer("model.conf", "policy.csv")
4. Deploy to a cluster
Got tons of traffic? Spread the load across servers.
5. Grant permissions to roles, not users
Fewer rules = faster enforcement. Simple as that.
Wrap-up
Let's recap how to set up RBAC for your API:
1. Plan your RBAC structure
List user roles, define permissions, and create a clear hierarchy.
2. Set up your environment
Choose an API framework, install tools, and configure your test server.
3. Implement RBAC in your API
Create user models, set up roles and permissions, assign roles to users, and build a secure login system.
4. Secure API endpoints
Use middleware for role checks, add RBAC to route handlers, and handle unauthorized access attempts.
5. Test and refine
Check role assignments, test protected endpoints, and verify different user roles.
Keep RBAC Sharp
RBAC isn't a one-and-done deal. It needs ongoing attention:
1. Audit regularly: Check roles and permissions often. Your API's growth can create new security risks.
2. Stick to least privilege: Give users only what they need. It's easier to add permissions than to clean up after a breach.
3. Stay nimble: Update roles as your API evolves. Outdated roles can become security weak spots.
4. Watch and record: Keep tabs on user actions. Good logs can help you catch issues early.
5. Team up on security: Make sure everyone gets RBAC. Your system is only as strong as its weakest link.
Remember: RBAC is a living system. Keep it current, keep it tight, and you'll keep your API secure.
FAQs
How to implement RBAC in API?
Setting up RBAC for your API is straightforward:
- Navigate to Dashboard > Applications > APIs
- Select your API
- Find RBAC Settings
- Turn on "Enable RBAC"
- Switch on "Add Permissions in the Access Token"
- Hit Save
This adds user permissions to the access token, enabling role-based access control in your API.
What is role-based access control for API?
RBAC for APIs manages user access to API resources. Here's how it works:
- Assign roles to users or groups
- Define permissions for each role
- Control API resource access based on these roles
RBAC brings several perks:
- Simplifies access management
- Boosts security
- Works for organizations of all sizes
Take a healthcare API, for example:
Doctors might access patient medical histories, nurses could see current patient status, and billing staff might view patient financial info
Related posts
Ready to get started?