Using the Gmail API to Send Messages (with PHP examples)
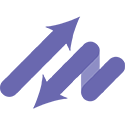
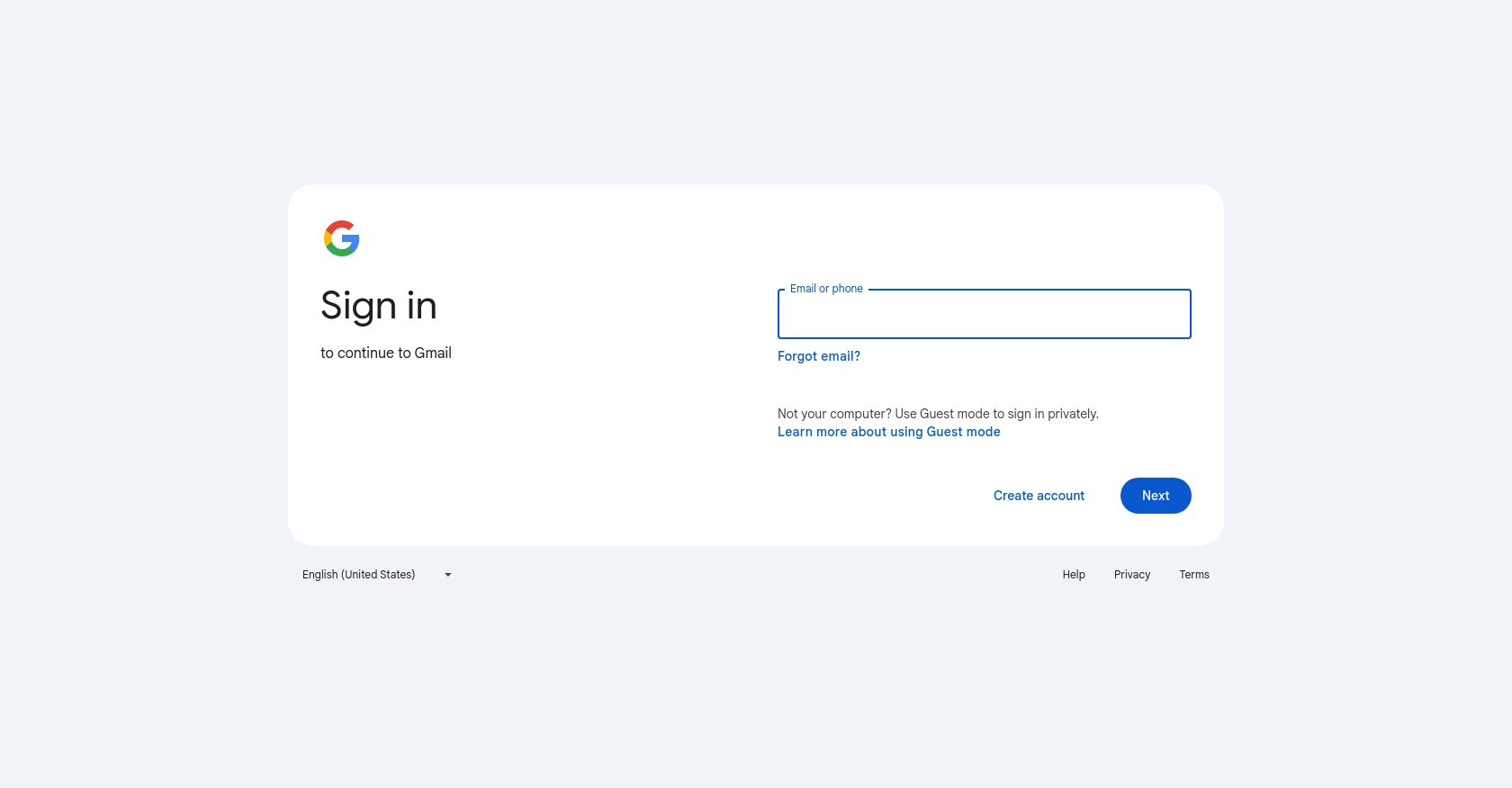
Introduction to Gmail API for Sending Emails
The Gmail API is a powerful tool that allows developers to access and interact with Gmail mailboxes programmatically. It provides a RESTful interface for various operations, including sending emails, managing drafts, and organizing messages. This makes it an ideal choice for developers looking to automate email-related tasks within their applications.
Integrating with the Gmail API can significantly enhance the functionality of your application by enabling automated email sending. For example, a developer might use the Gmail API to send transactional emails, such as order confirmations or password resets, directly from their application. This integration can streamline communication processes and improve user engagement.
In this article, we will explore how to use the Gmail API to send messages using PHP. We will cover the necessary steps to authenticate with the API, create email messages, and send them programmatically. By the end of this guide, you will have a solid understanding of how to leverage the Gmail API for your email-sending needs.
Setting Up Your Gmail API Test Environment
Before you can start sending emails using the Gmail API, you need to set up a Google Cloud project and configure OAuth 2.0 authentication. This will allow your application to securely access the Gmail API on behalf of users.
Create a Google Cloud Project
To begin, you need a Google Cloud project. Follow these steps to create one:
- Visit the Google Cloud Console.
- Navigate to Menu > IAM & Admin > Create a Project.
- Enter a project name and select your organization and location.
- Click Create to set up your project.
Enable the Gmail API
Once your project is created, enable the Gmail API:
- In the Google Cloud Console, go to Menu > APIs & Services > Library.
- Search for "Gmail API" and click on it.
- Click Enable to activate the API for your project.
Configure OAuth Consent Screen
Next, configure the OAuth consent screen to define what information is displayed to users:
- Go to Menu > APIs & Services > OAuth consent screen.
- Select the user type and fill out the required fields, such as application name and support email.
- Click Save and Continue.
Create OAuth 2.0 Credentials
Now, create the credentials needed for OAuth 2.0 authentication:
- Navigate to Menu > APIs & Services > Credentials.
- Click Create Credentials > OAuth client ID.
- Select the application type (e.g., Web application) and configure the authorized redirect URIs.
- Click Create to generate your client ID and client secret.
Make sure to securely store your client ID and client secret, as you will need them to authenticate your application with the Gmail API.
For more detailed instructions, refer to the official Google documentation: Create a Google Cloud project, Enable Google Workspace APIs, Configure OAuth consent, and Create access credentials.
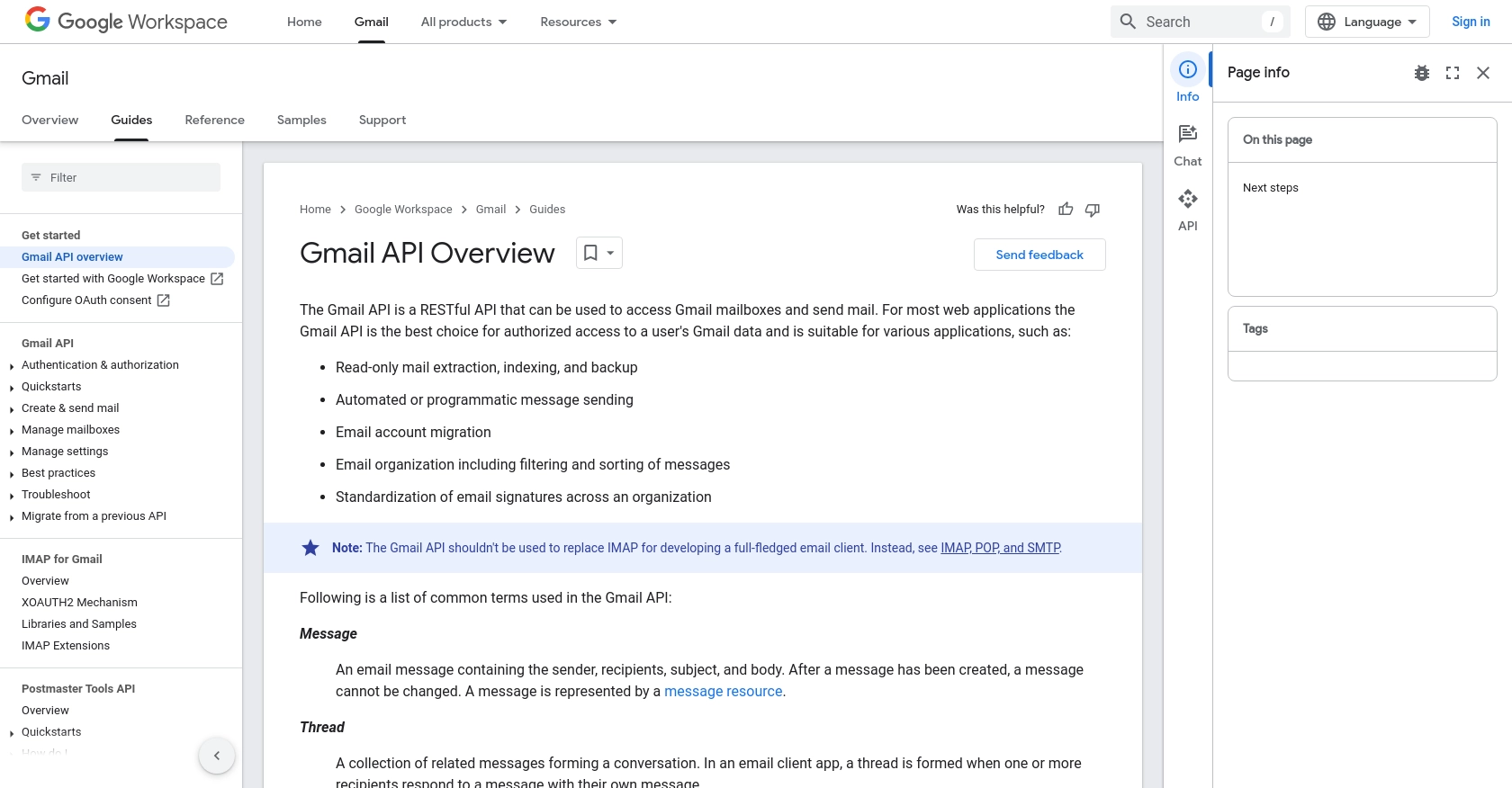
sbb-itb-96038d7
Making API Calls to Send Emails Using Gmail API with PHP
To send emails using the Gmail API with PHP, you need to set up your development environment and write the necessary code to interact with the API. This section will guide you through the process of making API calls, including setting up PHP, installing dependencies, and writing the code to send an email.
Setting Up PHP Environment for Gmail API
Before diving into the code, ensure you have the following prerequisites installed on your machine:
- PHP 7.4 or higher
- Composer, the PHP package manager
Once you have these installed, use Composer to install the Google Client Library for PHP by running the following command in your terminal:
composer require google/apiclient:^2.0
Writing PHP Code to Send Emails via Gmail API
Now that your environment is set up, you can write the PHP code to send an email using the Gmail API. Create a file named send_email.php
and add the following code:
require 'vendor/autoload.php';
use Google\Client;
use Google\Service\Gmail;
// Initialize the Google Client
$client = new Client();
$client->setApplicationName('Gmail API PHP Quickstart');
$client->setScopes(Gmail::GMAIL_SEND);
$client->setAuthConfig('credentials.json');
$client->setAccessType('offline');
// Get the Gmail service
$service = new Gmail($client);
// Create the email content
$email = new Google_Service_Gmail_Message();
$rawMessageString = "From: your-email@gmail.com\r\n";
$rawMessageString .= "To: recipient-email@gmail.com\r\n";
$rawMessageString .= "Subject: Test Email\r\n\r\n";
$rawMessageString .= "This is a test email sent from the Gmail API using PHP.";
// Encode the message
$rawMessage = base64_encode($rawMessageString);
$rawMessage = str_replace(['+', '/', '='], ['-', '_', ''], $rawMessage);
$email->setRaw($rawMessage);
// Send the email
try {
$message = $service->users_messages->send('me', $email);
echo 'Message sent successfully. Message ID: ' . $message->getId();
} catch (Exception $e) {
echo 'An error occurred: ' . $e->getMessage();
}
Verifying Successful Email Sending
After running the script, check the recipient's inbox to verify that the email was sent successfully. If the email appears, your integration is working correctly. If you encounter any errors, ensure that your OAuth credentials are correct and that you have the necessary permissions.
Handling Errors and Common Issues
While making API calls, you might encounter errors. Common issues include:
- Invalid credentials: Ensure your
credentials.json
file is correctly configured. - Insufficient permissions: Verify that your OAuth scopes include
Gmail::GMAIL_SEND
. - Network issues: Check your internet connection and retry the request.
For detailed error handling, refer to the Gmail API documentation.
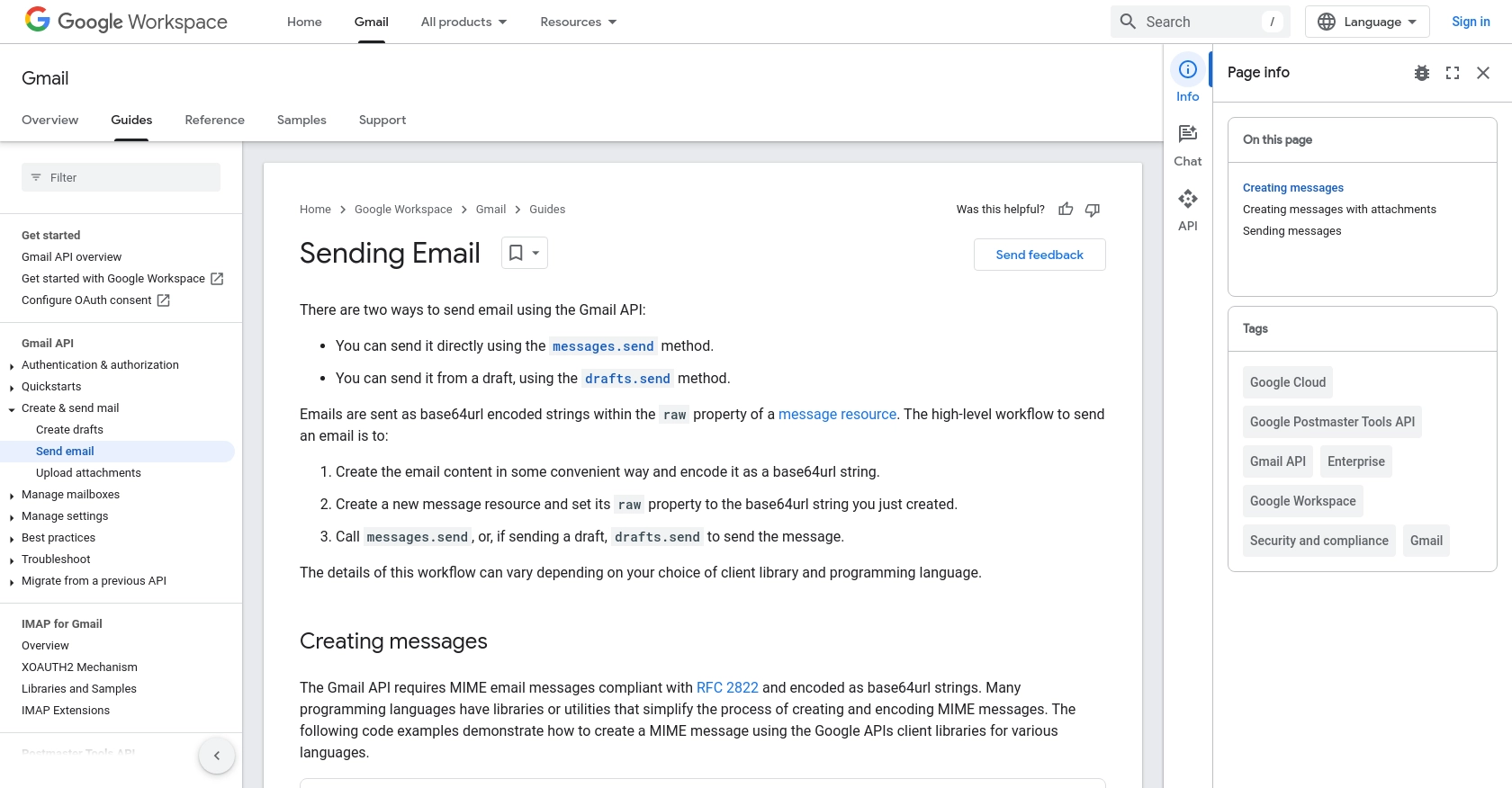
Best Practices for Using Gmail API in PHP Applications
When integrating the Gmail API into your PHP applications, it's essential to follow best practices to ensure security, efficiency, and maintainability. Here are some recommendations:
- Securely Store Credentials: Always keep your OAuth credentials, such as client ID and client secret, secure. Avoid hardcoding them in your source code. Instead, use environment variables or secure storage solutions.
- Handle Rate Limiting: The Gmail API enforces rate limits to ensure fair usage. Monitor your application's API usage and implement exponential backoff strategies to handle rate limit errors gracefully.
- Use Appropriate Scopes: Request only the necessary OAuth scopes for your application. This minimizes security risks and improves user trust by limiting access to sensitive data.
- Implement Error Handling: Ensure robust error handling in your application to manage API errors effectively. Log errors for debugging and provide user-friendly messages when issues occur.
- Optimize API Calls: Batch requests when possible to reduce the number of API calls and improve performance. This is especially useful for applications sending multiple emails in a short period.
Conclusion: Streamline Email Integrations with Endgrate
Integrating the Gmail API into your PHP applications can significantly enhance your email automation capabilities. By following the steps outlined in this guide, you can efficiently send emails programmatically, improving communication workflows and user engagement.
However, managing multiple integrations can be complex and time-consuming. That's where Endgrate comes in. With Endgrate, you can streamline your integration processes, allowing you to focus on your core product. Endgrate offers a unified API endpoint that connects to multiple platforms, including Gmail, providing an intuitive integration experience for your customers.
Save time and resources by leveraging Endgrate's powerful integration solutions. Visit Endgrate to learn more about how you can simplify your integration strategy and enhance your application's capabilities.
Read More
- https://endgrate.com/provider/gmail
- https://developers.google.com/gmail/api/guides
- https://developers.google.com/gmail/api/auth/scopes
- https://developers.google.com/workspace/guides/create-project
- https://developers.google.com/workspace/guides/enable-apis
- https://developers.google.com/workspace/guides/configure-oauth-consent
- https://developers.google.com/workspace/guides/create-credentials
- https://developers.google.com/gmail/api/guides/sending
Ready to get started?