Using the Nutshell API to Create or Update Contacts (with PHP examples)
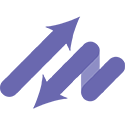
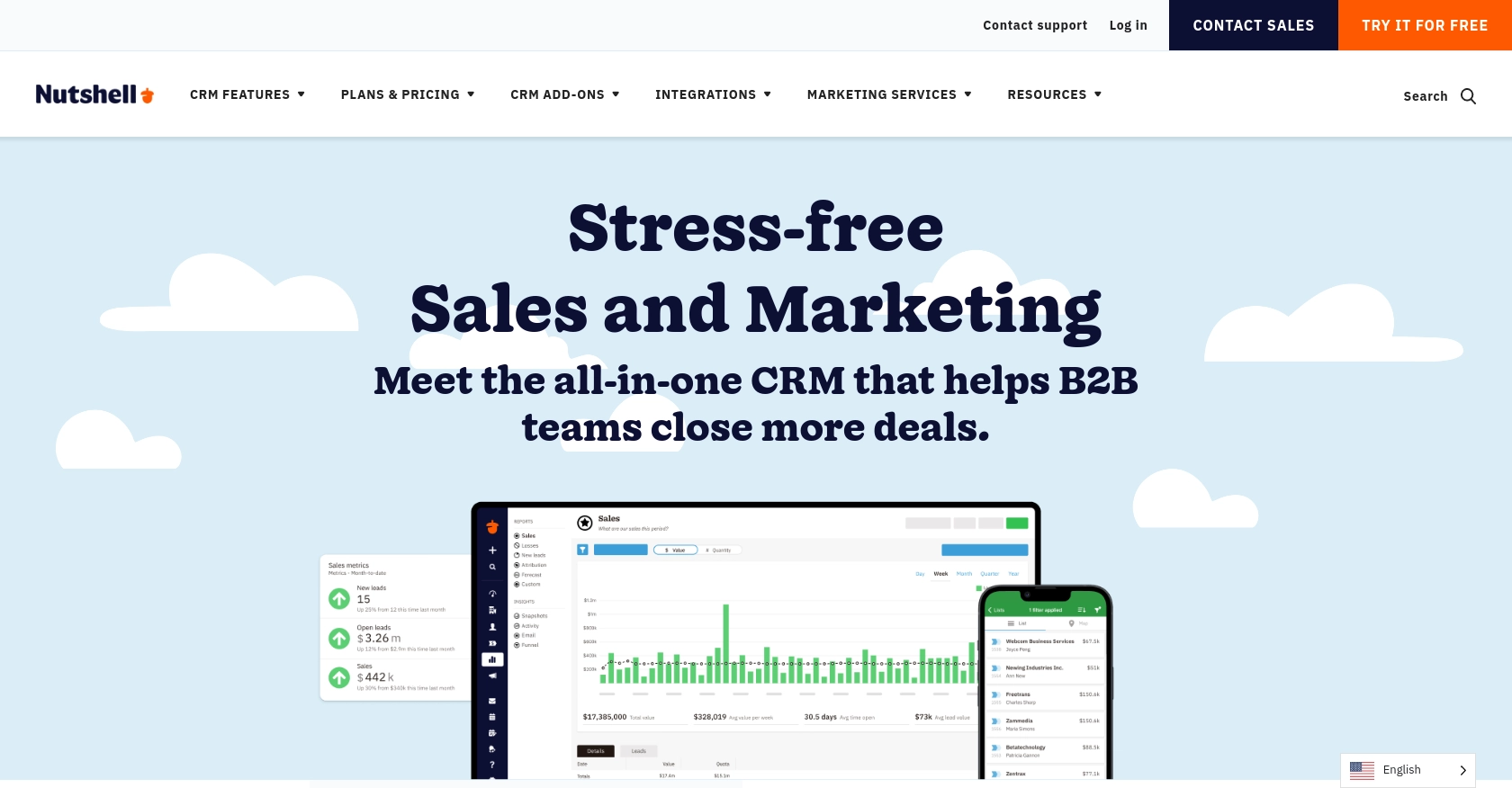
Introduction to Nutshell CRM API
Nutshell is a powerful CRM platform designed to help businesses manage their sales processes efficiently. With features like contact management, lead tracking, and sales automation, Nutshell provides a comprehensive solution for businesses looking to streamline their customer relationship management.
Developers may want to integrate with Nutshell's API to automate and enhance their CRM workflows. For example, using the Nutshell API, a developer can create or update contact information directly from a web application, ensuring that the CRM data remains up-to-date and accurate.
This article will guide you through using PHP to interact with the Nutshell API, focusing on creating and updating contacts. By the end of this tutorial, you'll be able to seamlessly integrate Nutshell's CRM capabilities into your applications, enhancing your business processes.
Setting Up Your Nutshell API Test Account
Before diving into the integration process with the Nutshell API, it's essential to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data. Here's how you can get started:
Creating a Nutshell Account
If you don't already have a Nutshell account, you can sign up for a free trial on the Nutshell website. Follow the on-screen instructions to complete the registration process. Once your account is created, log in to access the dashboard.
Generating an API Key for Authentication
To interact with the Nutshell API, you'll need an API key. Follow these steps to generate one:
- Navigate to the "Setup" tab in your Nutshell dashboard.
- Click on "API" to access the API settings.
- Create a new API key by selecting the appropriate permissions, such as "Form submissions + Wufoo."
- Save the API key securely, as you'll need it for authentication in your PHP scripts.
Understanding Nutshell API Authentication
The Nutshell API uses HTTP Basic authentication. You'll need to include an Authentication header in your API requests. The username is either your company's domain or a specific user's email address, and the password is the API key you generated.
Here's an example of how to structure your authentication in a cURL command:
curl -u <domain_or_username>:<api_token> \
-d '{ "method": "getContact", "params": { "contactId": 123 } }' \
https://app.nutshell.com/api/v1/json
Ensure you replace <domain_or_username>
and <api_token>
with your actual domain or email and API key.
Testing Your API Setup
Once you've set up your API key and authentication, it's a good idea to test your setup by making a simple API call. You can use the example cURL command above to retrieve a contact and verify that your authentication is working correctly.
If you encounter any issues, double-check your API key and authentication details. For further assistance, refer to the Nutshell API documentation or contact Nutshell support.
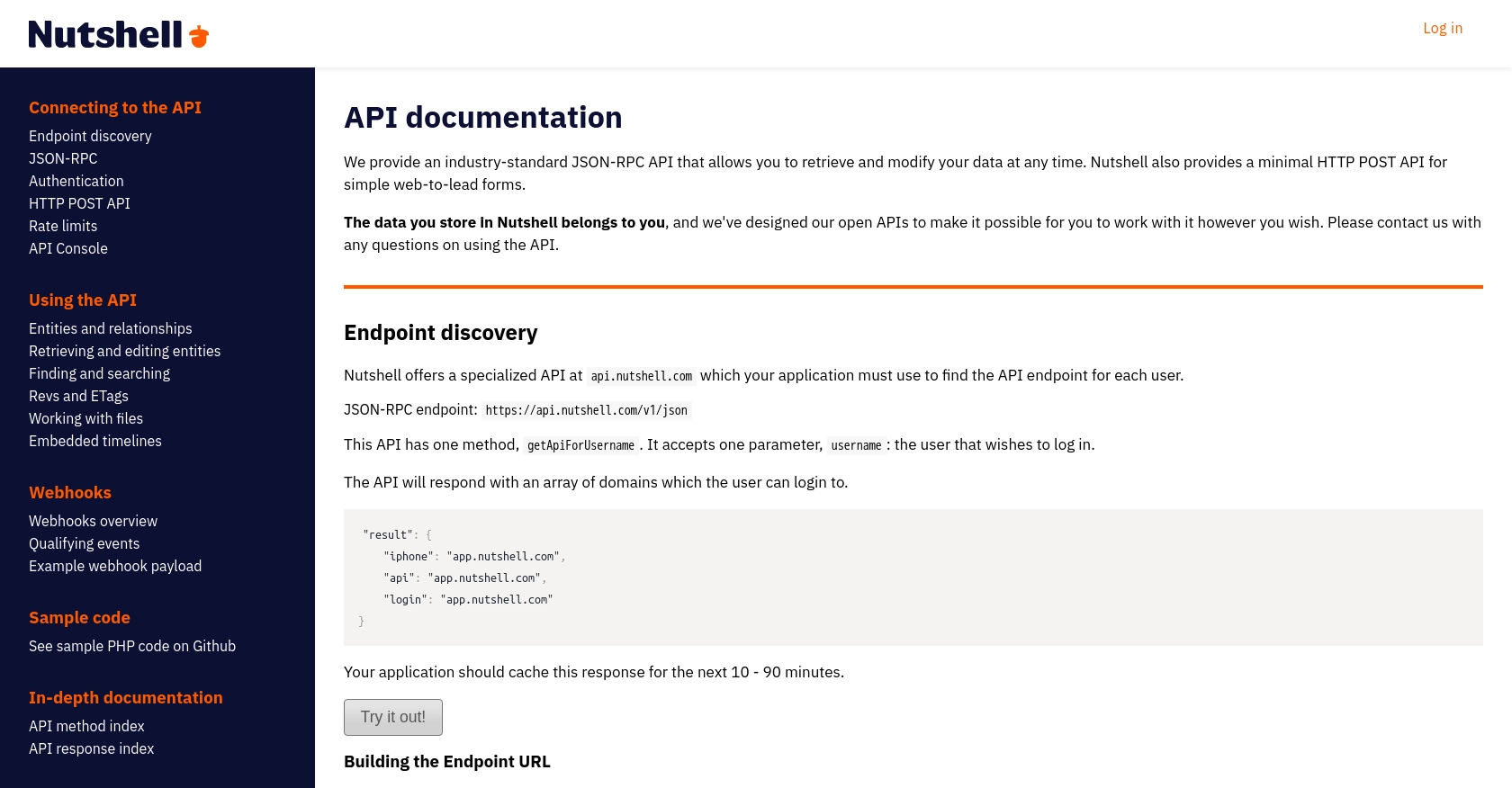
sbb-itb-96038d7
Making API Calls with PHP to Create or Update Contacts in Nutshell
To effectively interact with the Nutshell API using PHP, you'll need to understand how to structure your API calls for creating or updating contacts. This section will guide you through the necessary steps, including setting up your PHP environment, writing the code, and handling responses.
Setting Up Your PHP Environment for Nutshell API Integration
Before you begin coding, ensure that your PHP environment is properly configured. You'll need PHP 7.4 or later and the cURL extension enabled. You can verify your PHP version and extensions using the following command:
php -v
Ensure that cURL is installed by checking your PHP configuration:
php -m | grep curl
Writing PHP Code to Create a Contact in Nutshell
To create a contact in Nutshell, you'll need to send a JSON-RPC request to the API endpoint. Here's a sample PHP script to create a new contact:
<?php
$apiUrl = 'https://app.nutshell.com/api/v1/json';
$username = 'your_email_or_domain';
$apiKey = 'your_api_key';
$data = [
'method' => 'newContact',
'params' => [
'contact' => [
'name' => 'John Doe',
'email' => ['john.doe@example.com'],
'phone' => ['123-456-7890'],
'description' => 'New contact from PHP script'
]
]
];
$options = [
CURLOPT_URL => $apiUrl,
CURLOPT_RETURNTRANSFER => true,
CURLOPT_HTTPAUTH => CURLAUTH_BASIC,
CURLOPT_USERPWD => "$username:$apiKey",
CURLOPT_POST => true,
CURLOPT_POSTFIELDS => json_encode($data),
CURLOPT_HTTPHEADER => ['Content-Type: application/json']
];
$ch = curl_init();
curl_setopt_array($ch, $options);
$response = curl_exec($ch);
curl_close($ch);
echo $response;
?>
Replace your_email_or_domain
and your_api_key
with your actual Nutshell credentials. This script sends a request to create a new contact with the specified details.
Updating an Existing Contact in Nutshell Using PHP
To update an existing contact, you'll need the contact's ID and revision number. Here's how you can update a contact's information:
<?php
$contactId = 42; // Replace with the actual contact ID
$rev = '6'; // Replace with the actual revision number
$data = [
'method' => 'editContact',
'params' => [
'contactId' => $contactId,
'rev' => $rev,
'contact' => [
'description' => 'Updated contact description'
]
]
];
$options[CURLOPT_POSTFIELDS] = json_encode($data);
$ch = curl_init();
curl_setopt_array($ch, $options);
$response = curl_exec($ch);
curl_close($ch);
echo $response;
?>
This script updates the contact's description. Ensure you have the correct contact ID and revision number to avoid conflicts.
Handling API Responses and Errors
After making an API call, it's crucial to handle the response correctly. The Nutshell API will return a JSON response that you can decode and process:
$responseData = json_decode($response, true);
if (isset($responseData['error'])) {
echo 'Error: ' . $responseData['error']['message'];
} else {
echo 'Success: Contact updated/created successfully.';
}
Check for errors in the response and handle them appropriately. Refer to the Nutshell API documentation for detailed error codes and messages.
By following these steps, you can efficiently create or update contacts in Nutshell using PHP, enhancing your CRM integration capabilities.
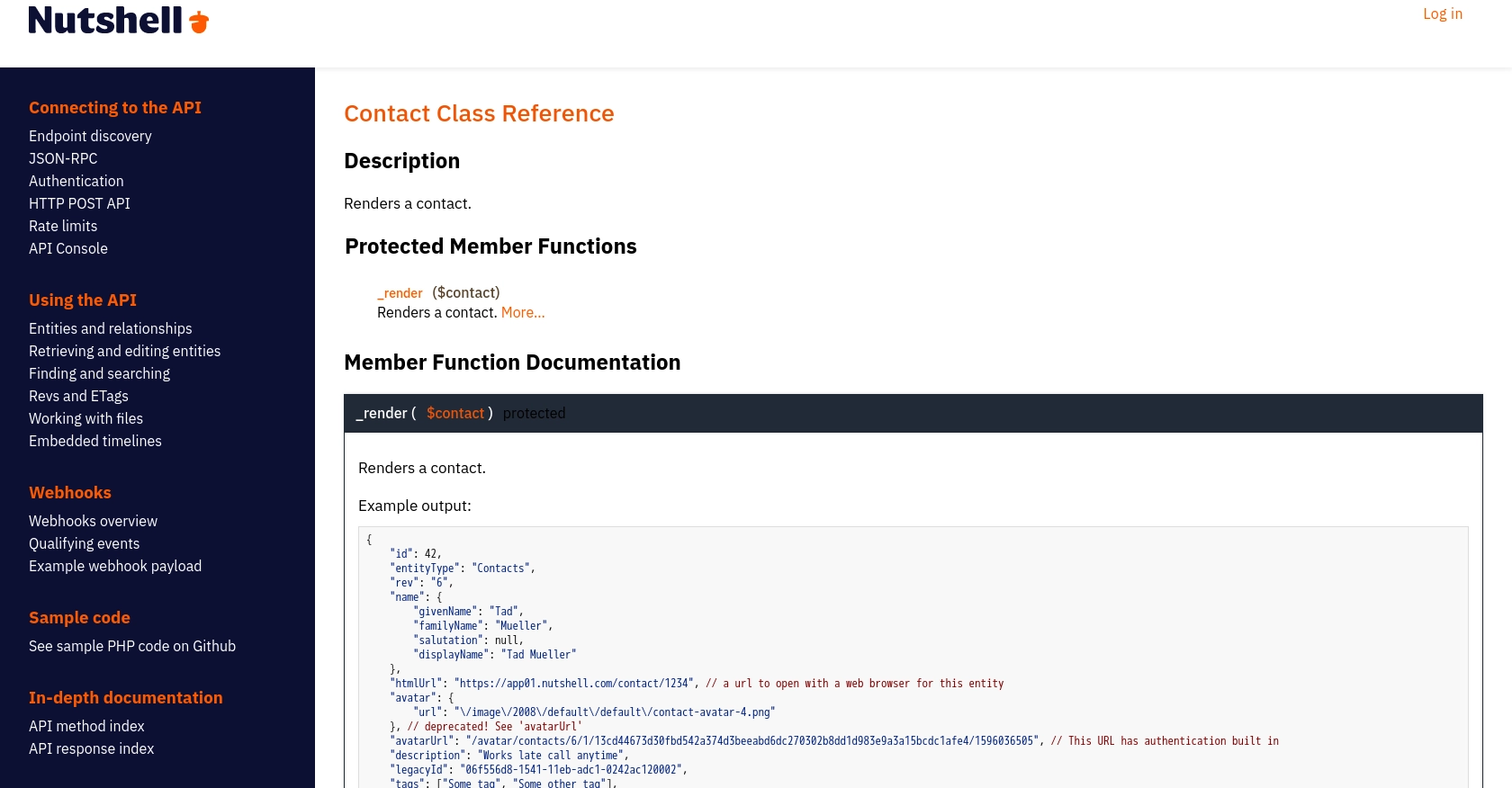
Conclusion: Best Practices for Nutshell API Integration with PHP
Integrating Nutshell's CRM capabilities into your applications using PHP can significantly enhance your business processes by automating contact management and ensuring data accuracy. Here are some best practices to consider when working with the Nutshell API:
Securely Storing API Credentials
Always store your API credentials securely. Avoid hardcoding them in your scripts. Instead, use environment variables or secure vaults to manage sensitive information.
Handling Nutshell API Rate Limits
Be mindful of the API rate limits to avoid service disruptions. Implement logic to handle rate limit responses gracefully, such as retrying requests after a delay. For more details, refer to the Nutshell API documentation.
Efficient Data Management and Transformation
Ensure that data fields are standardized and transformed as needed before sending them to Nutshell. This helps maintain consistency and accuracy across your CRM data.
Implementing Error Handling and Logging
Robust error handling is crucial for identifying and resolving issues quickly. Implement logging to capture API request and response details, which can aid in troubleshooting and performance monitoring.
Leveraging Endgrate for Simplified Integrations
If managing multiple integrations becomes overwhelming, consider using Endgrate. It offers a unified API endpoint that connects to various platforms, including Nutshell, allowing you to streamline your integration processes and focus on your core product development.
By following these best practices, you can optimize your integration with Nutshell's API, ensuring a seamless and efficient CRM experience for your business.
Read More
Ready to get started?