Using the Nutshell API to Create or Update Contacts in Javascript
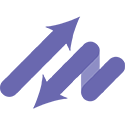
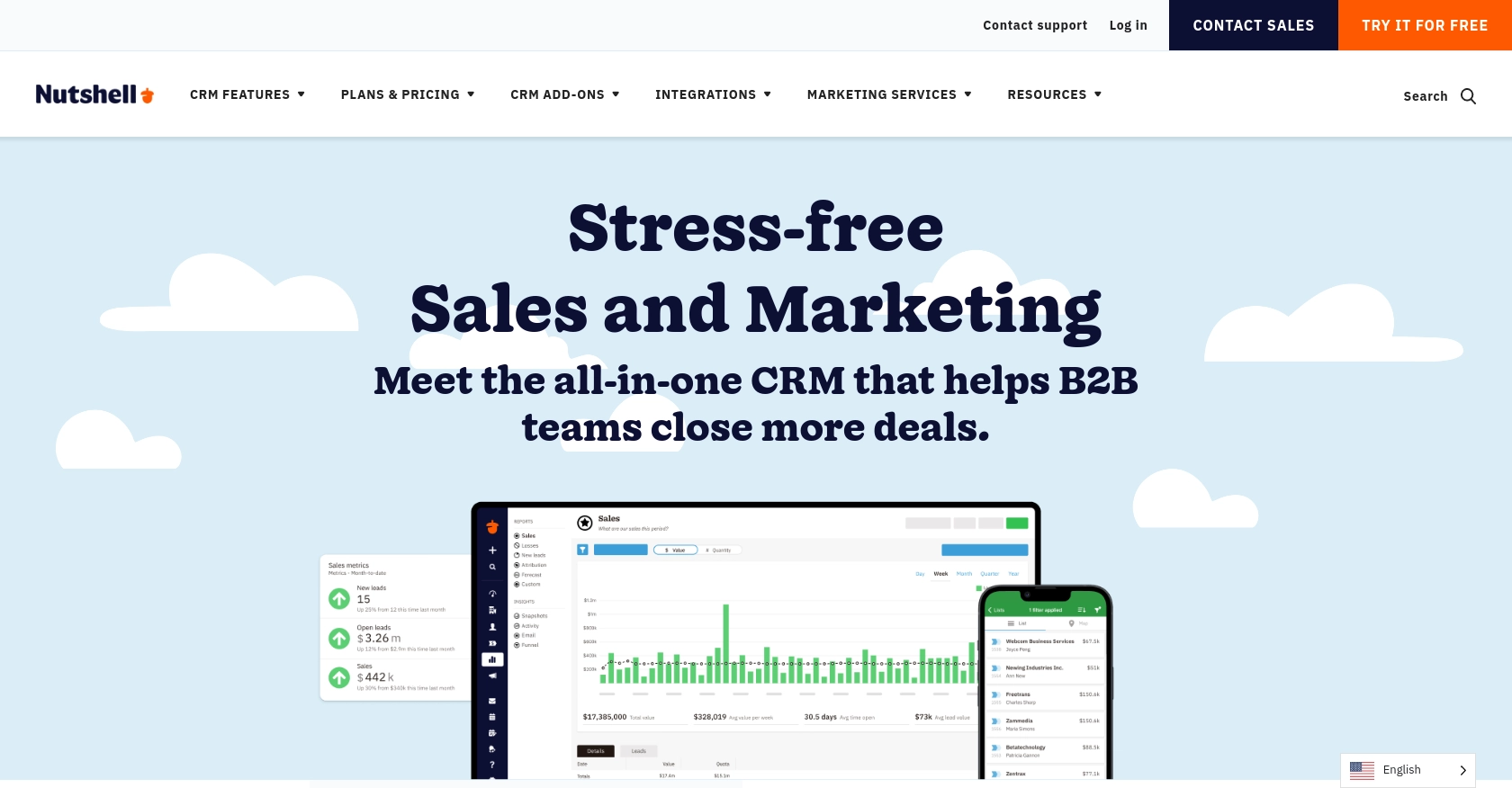
Introduction to Nutshell CRM
Nutshell is a powerful CRM platform designed to help businesses manage their sales processes efficiently. With its user-friendly interface and robust features, Nutshell enables teams to streamline communication, track leads, and close deals faster.
Integrating with Nutshell's API allows developers to automate and enhance CRM functionalities, such as managing contact information. For example, you can use the Nutshell API to create or update contacts directly from your application, ensuring that your sales team always has the most current data at their fingertips.
Setting Up Your Nutshell API Test Account
Before diving into integrating with the Nutshell API, it's essential to set up a test account to safely experiment with API calls without affecting live data. This section will guide you through creating a sandbox environment and obtaining the necessary credentials for authentication.
Creating a Nutshell Sandbox Account
To begin, you'll need to create a Nutshell account if you don't already have one. Visit the Nutshell website and sign up for a free trial or demo account. This will provide you with access to the platform's features and allow you to test API interactions.
Generating an API Key for Authentication
The Nutshell API uses HTTP Basic authentication, requiring a username and an API key. Follow these steps to generate your API key:
- Log in to your Nutshell account.
- Navigate to the "Setup" tab in the top navigation bar.
- Select "API" from the sidebar menu.
- Click on "Create New API Key" and provide a name for your key.
- Ensure the key has the necessary permissions for accessing and modifying contacts.
- Save the API key securely, as it will be used as the password in your API requests.
Configuring API Access with HTTP Basic Authentication
Once you have your API key, you can configure your application to authenticate API requests. Use your company's domain or a specific user's email address as the username, and the API key as the password. Here's an example of how to set up authentication in a JavaScript application using the fetch API:
const username = 'your-email@example.com';
const apiKey = 'your-api-key';
const authHeader = 'Basic ' + btoa(username + ':' + apiKey);
fetch('https://app.nutshell.com/api/v1/json', {
method: 'POST',
headers: {
'Authorization': authHeader,
'Content-Type': 'application/json'
},
body: JSON.stringify({
method: 'getApiForUsername',
params: { username: 'your-username' },
id: 1
})
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Replace your-email@example.com
and your-api-key
with your actual credentials. This setup will allow you to authenticate and interact with the Nutshell API securely.
For more detailed information on authentication, refer to the Nutshell API documentation.
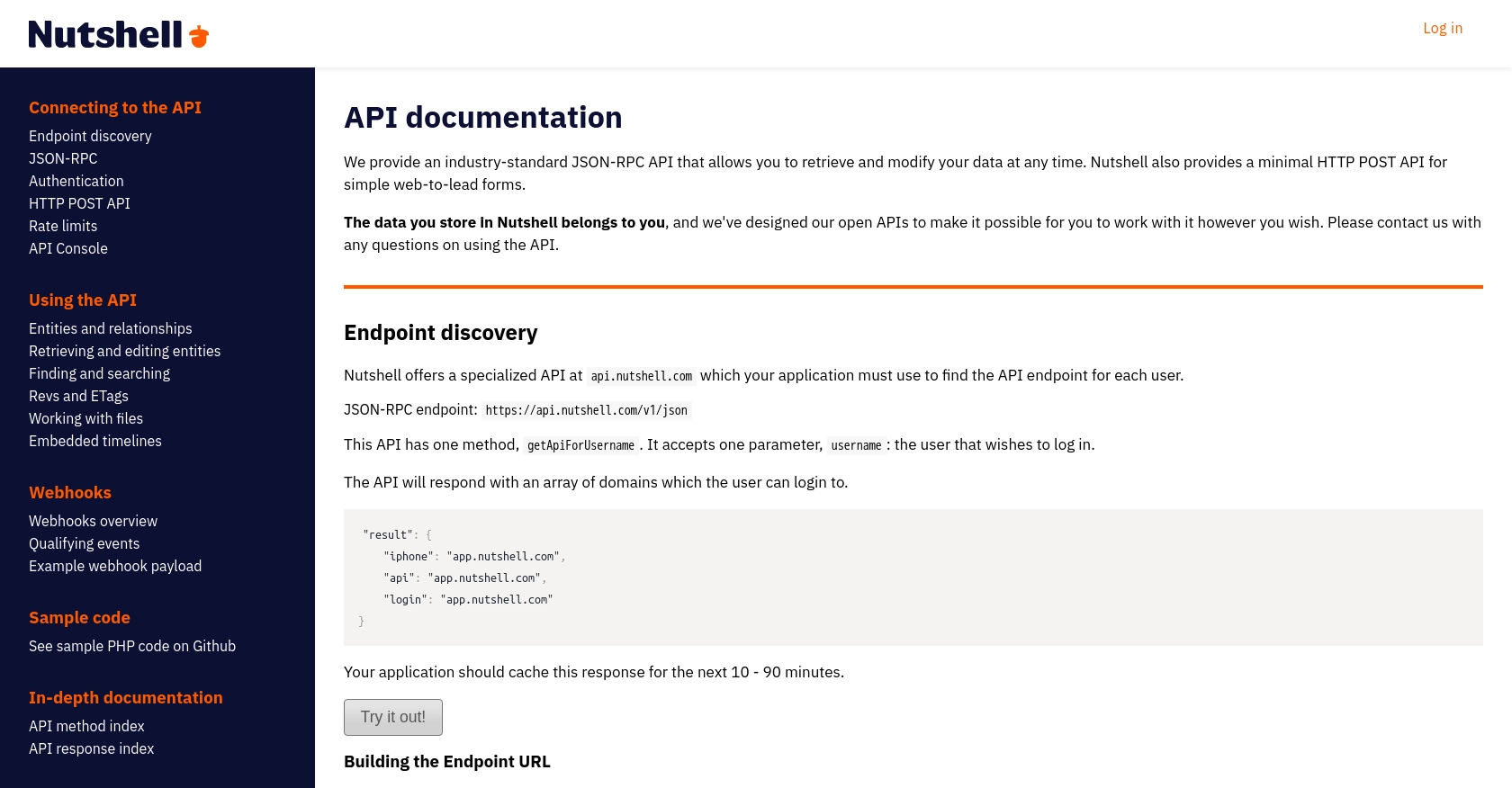
sbb-itb-96038d7
Making API Calls to Nutshell for Contact Management in JavaScript
Setting Up Your JavaScript Environment for Nutshell API Integration
To interact with the Nutshell API using JavaScript, ensure you have a modern JavaScript environment set up. This includes having Node.js installed if you're running scripts server-side, or a browser that supports the Fetch API for client-side operations.
You'll also need to install any necessary dependencies. For server-side applications, you can use Node.js with the node-fetch
package to make HTTP requests. Install it using:
npm install node-fetch
Creating or Updating Contacts Using Nutshell API
With your environment ready, you can now proceed to create or update contacts in Nutshell. The following example demonstrates how to make an API call to create a new contact using JavaScript:
const fetch = require('node-fetch');
const username = 'your-email@example.com';
const apiKey = 'your-api-key';
const authHeader = 'Basic ' + Buffer.from(username + ':' + apiKey).toString('base64');
const createContact = async () => {
const contactData = {
method: 'newContact',
params: {
contact: {
name: 'John Doe',
email: ['john.doe@example.com'],
phone: ['123-456-7890']
}
},
id: 1
};
try {
const response = await fetch('https://app.nutshell.com/api/v1/json', {
method: 'POST',
headers: {
'Authorization': authHeader,
'Content-Type': 'application/json'
},
body: JSON.stringify(contactData)
});
const data = await response.json();
console.log('Contact Created:', data);
} catch (error) {
console.error('Error creating contact:', error);
}
};
createContact();
Replace your-email@example.com
and your-api-key
with your actual credentials. This code snippet demonstrates how to authenticate and send a request to create a contact in Nutshell.
Handling API Responses and Errors
After making an API call, it's crucial to handle the response appropriately. The Nutshell API will return a JSON response, which you can parse and use within your application. Always check for errors and handle them gracefully to ensure a smooth user experience.
For instance, if the API returns a 401 status code, it indicates an authentication error. Ensure your credentials are correct and retry the request. Refer to the Nutshell API documentation for more details on error codes and handling.
Verifying API Call Success in Nutshell
To verify that your API call succeeded, log in to your Nutshell account and check the contacts list. The new contact should appear there if the API call was successful. This step ensures that your integration is working as expected.
By following these steps, you can efficiently manage contacts in Nutshell using JavaScript, enhancing your CRM capabilities and ensuring your sales team has up-to-date information.
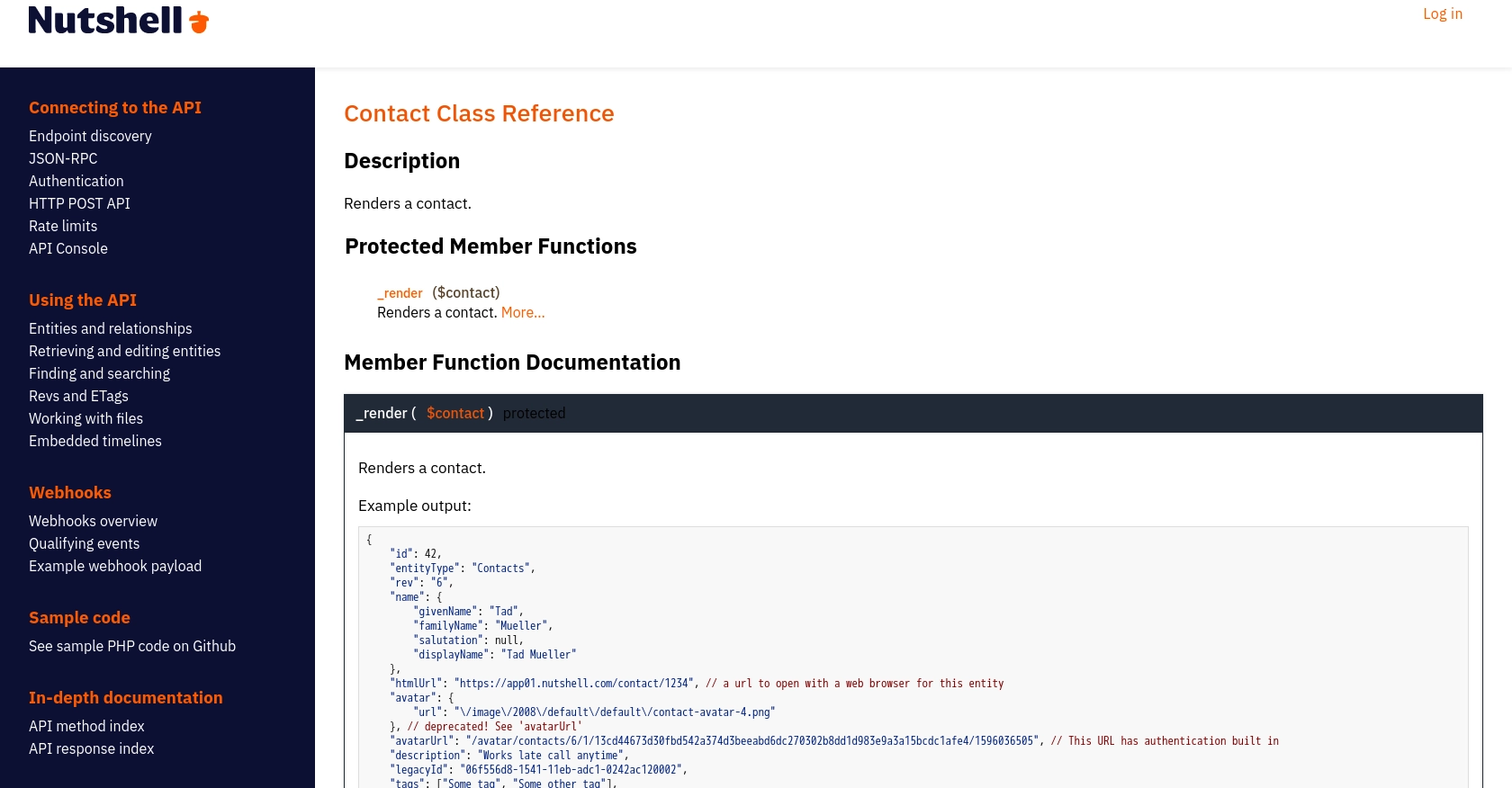
Conclusion and Best Practices for Using Nutshell API in JavaScript
Integrating with the Nutshell API using JavaScript offers a powerful way to enhance your CRM capabilities by automating contact management. By following the steps outlined in this guide, you can create or update contacts efficiently, ensuring your sales team has access to the most current data.
Best Practices for Nutshell API Integration
- Securely Store Credentials: Always store your API keys and credentials securely. Consider using environment variables or a secure vault to keep them safe.
- Handle Rate Limits: Be aware of any rate limits imposed by the Nutshell API to avoid service interruptions. Implement retry logic with exponential backoff if necessary.
- Data Standardization: Ensure that data fields are standardized before sending them to the API. This helps maintain consistency across your CRM.
- Error Handling: Implement robust error handling to manage API response errors gracefully. This ensures a seamless user experience.
By adhering to these best practices, you can optimize your integration with the Nutshell API, providing a seamless and efficient CRM experience for your team.
Streamline Your Integrations with Endgrate
If managing multiple integrations is becoming a challenge, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers.
Visit Endgrate to learn more about how you can simplify your integration processes and enhance your CRM capabilities with ease.
Read More
Ready to get started?