Using the Sage 100 API to Get Tax Schedules (with Python examples)
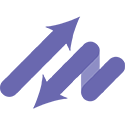
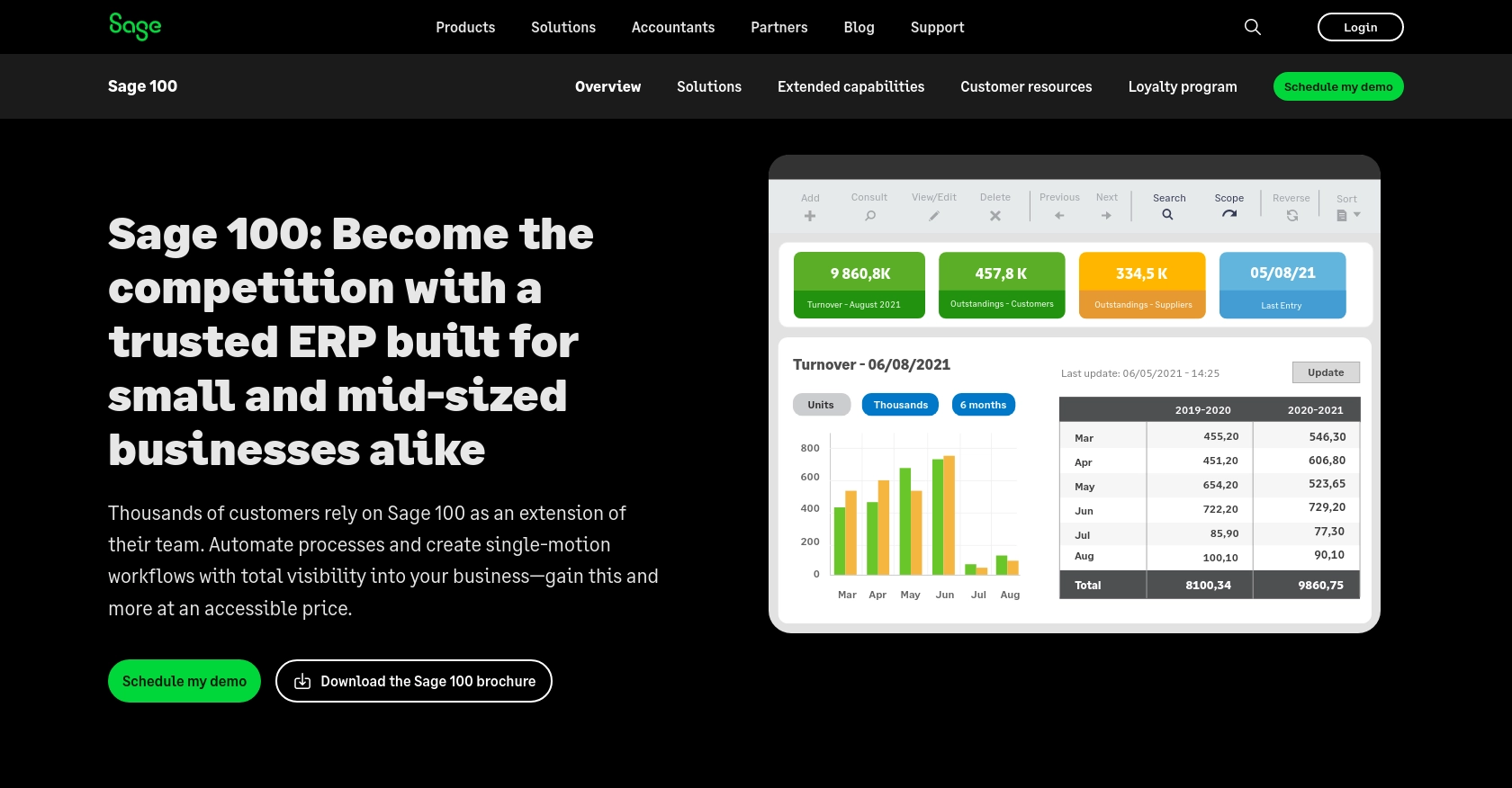
Introduction to Sage 100 API Integration
Sage 100 is a comprehensive ERP solution designed to streamline business operations, including accounting, inventory management, and customer relationship management. It is widely used by small to medium-sized businesses to enhance efficiency and productivity.
Integrating with the Sage 100 API allows developers to access and manipulate various data points within the system. For example, retrieving tax schedules using the Sage 100 API can help businesses automate tax calculations and ensure compliance with local tax regulations.
This article will guide you through using Python to interact with the Sage 100 API, specifically focusing on retrieving tax schedules. By following this tutorial, developers can efficiently access and manage tax-related data within Sage 100.
Setting Up a Test/Sandbox Account for Sage 100 API Integration
Before you can start interacting with the Sage 100 API to retrieve tax schedules, you'll need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data.
Creating a Sage 100 Sandbox Account
To begin, you'll need access to a Sage 100 environment. If you don't have one, contact Sage or your Sage 100 provider to inquire about a sandbox or test account. This will provide you with a safe space to test API interactions.
Configuring the Sage 100 ODBC Driver
Once you have access to a Sage 100 environment, the next step is to configure the Sage 100 ODBC driver. This is crucial for establishing a connection to the Sage 100 database. Follow these steps:
- Ensure the Sage 100 ODBC driver is installed on your system. You can download it from the Sage website or contact your provider for assistance.
- Access the ODBC Data Source Administrator on your system.
- Create a DSN (Data Source Name) that connects to the Sage 100 ERP system using the correct server, database, and authentication settings.
For detailed instructions, refer to the Sage 100 ODBC Driver Configuration Guide.
Setting Up Authentication for Sage 100 API
The Sage 100 API uses a custom authentication method. Follow these steps to set up authentication:
- Open the Sage 100 application and navigate to the Library Master module.
- Select Setup > System Configuration.
- On the ODBC Driver tab, enable the C/S ODBC Driver.
- Enter the server name or IP address where the ODBC application or service is running.
- Specify the server port, or leave it blank to use the default port, 20222.
Ensure that the ODBC connection is tested and working correctly by using the Test Connection feature in the ODBC Data Source Administrator.
Generating API Credentials
To interact with the Sage 100 API, you'll need to generate API credentials. This typically involves creating an application within the Sage 100 environment and obtaining the necessary keys or tokens.
Consult the Sage 100 documentation or your provider for specific instructions on generating these credentials, as the process may vary based on your setup.
With your test account and ODBC driver configured, you're now ready to start making API calls to retrieve tax schedules from Sage 100.
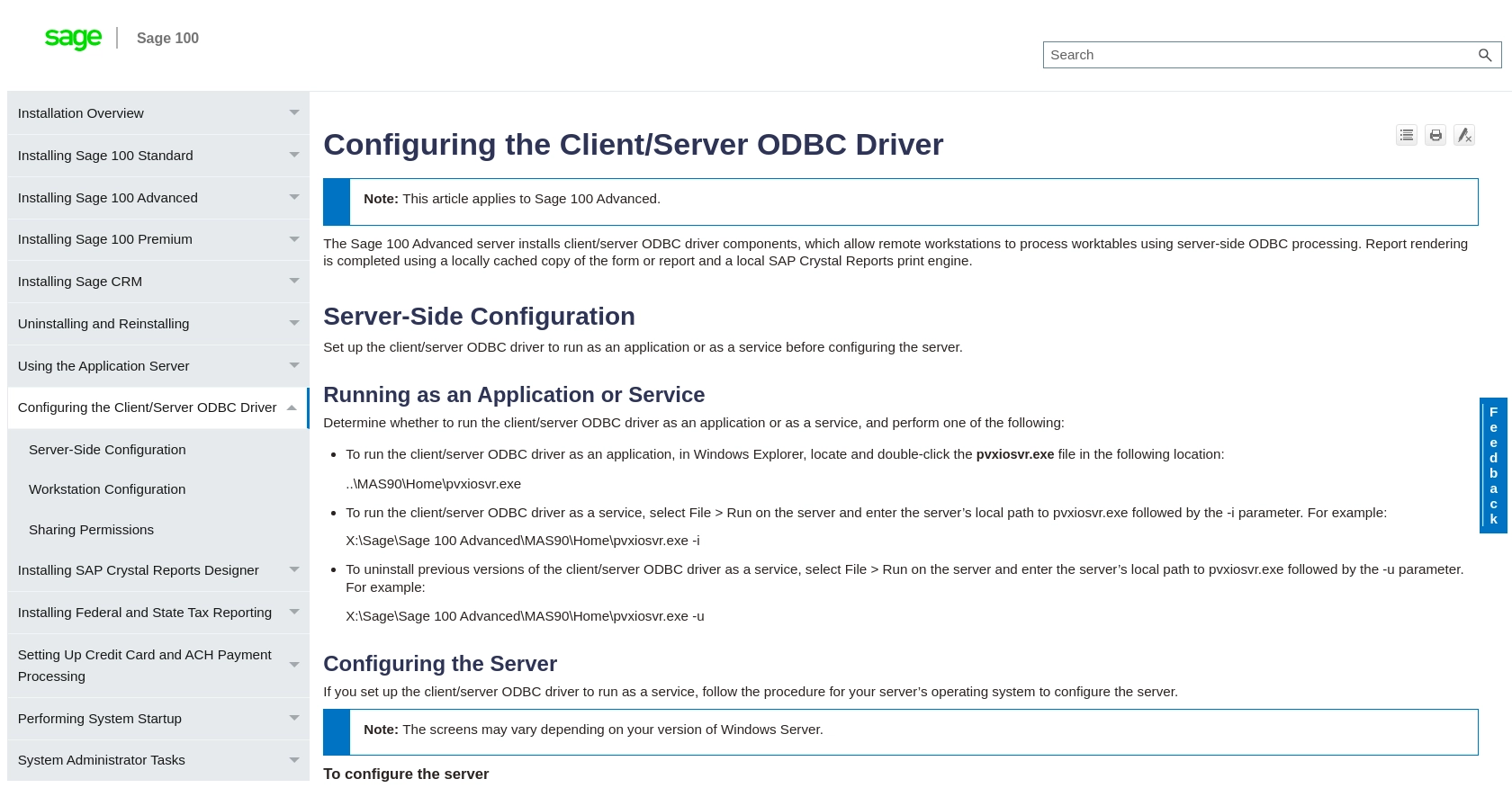
sbb-itb-96038d7
Making API Calls to Retrieve Tax Schedules from Sage 100 Using Python
To interact with the Sage 100 API and retrieve tax schedules, you'll need to use Python to establish a connection and perform the necessary API calls. This section will guide you through setting up your Python environment and executing the API call to access tax schedules.
Setting Up Your Python Environment for Sage 100 API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1
- The Python package installer, pip
Once these are installed, open your terminal or command prompt and install the necessary library to interact with the Sage 100 API:
pip install pyodbc
This library will allow you to connect to the Sage 100 database using ODBC.
Executing the API Call to Retrieve Tax Schedules
With your environment set up, you can now write the Python code to connect to the Sage 100 database and retrieve tax schedules. Create a file named get_tax_schedules.py
and add the following code:
import pyodbc
# Define the DSN and connection details
dsn = 'Your_DSN_Name'
user = 'Your_Username'
password = 'Your_Password'
# Establish a connection to the Sage 100 database
connection = pyodbc.connect(f'DSN={dsn};UID={user};PWD={password}')
# Create a cursor object to execute SQL queries
cursor = connection.cursor()
# Define the SQL query to retrieve tax schedules
query = "SELECT * FROM SY_SalesTaxSchedule"
# Execute the query
cursor.execute(query)
# Fetch and print the results
tax_schedules = cursor.fetchall()
for schedule in tax_schedules:
print(schedule)
# Close the connection
connection.close()
Replace Your_DSN_Name
, Your_Username
, and Your_Password
with your actual DSN, username, and password.
Verifying the API Call and Handling Errors
Run the script using the following command:
python get_tax_schedules.py
If successful, you should see the tax schedules printed in your terminal. Verify the results by cross-checking with the data in your Sage 100 sandbox environment.
To handle potential errors, wrap your connection and query execution in a try-except block to catch exceptions such as connection failures or query errors:
try:
# Connection and query code here
except pyodbc.Error as e:
print("Error: ", e)
finally:
connection.close()
For more detailed information on the Sage 100 API and its capabilities, refer to the Sage 100 File Layouts and Object Reference Documentation.
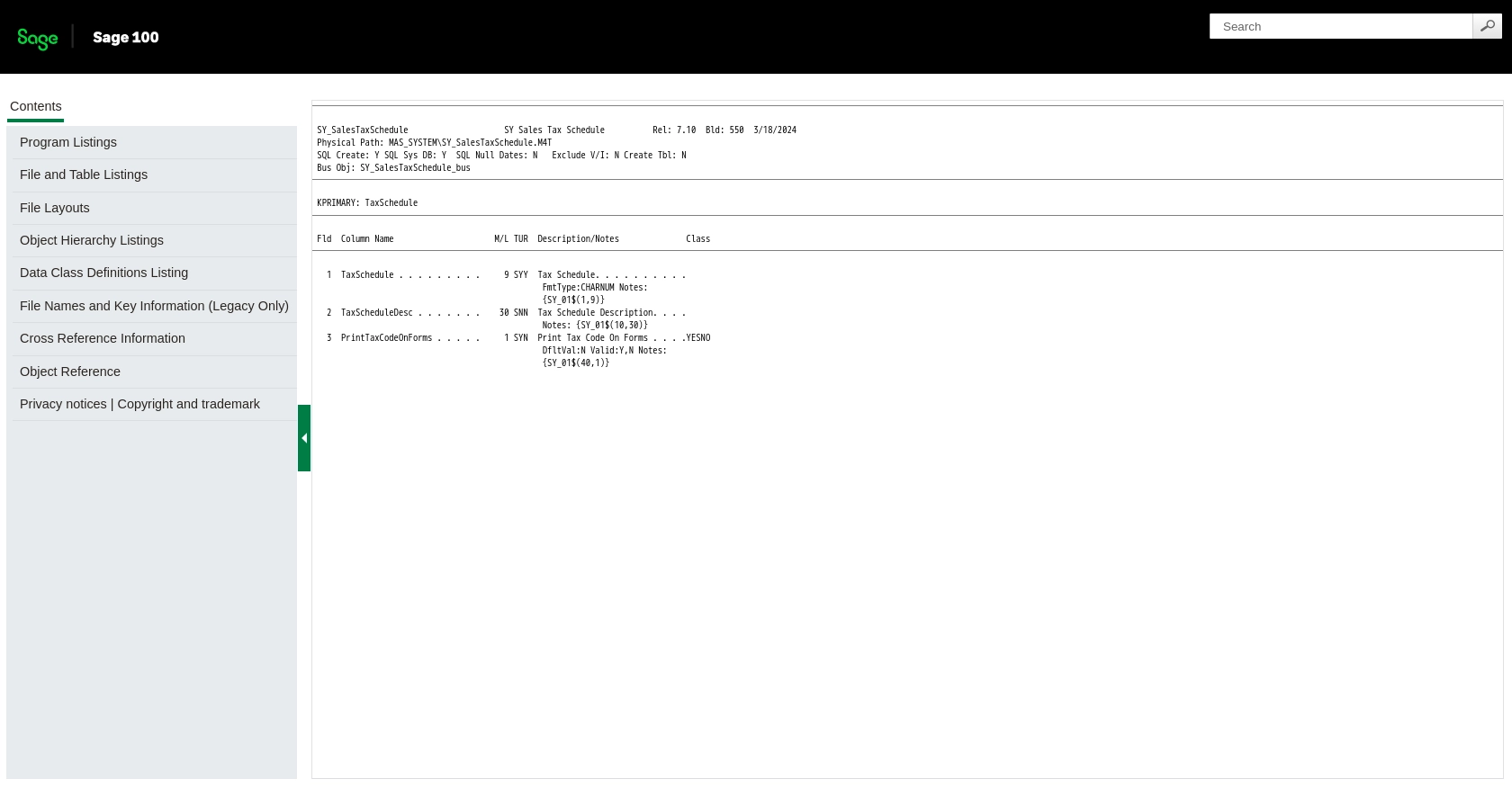
Conclusion and Best Practices for Sage 100 API Integration
Integrating with the Sage 100 API to retrieve tax schedules using Python can significantly enhance your business operations by automating tax calculations and ensuring compliance. By following the steps outlined in this guide, you can efficiently access and manage tax-related data within Sage 100.
Best Practices for Secure and Efficient Sage 100 API Usage
- Secure Storage of Credentials: Always store your DSN, username, and password securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the Sage 100 API. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that the data retrieved from Sage 100 is standardized and transformed as needed to fit your application's requirements.
- Error Handling: Implement robust error handling to manage connection issues and query failures. Use logging to track and debug errors effectively.
Enhance Your Integration Strategy with Endgrate
While integrating with Sage 100 API can be a powerful tool for your business, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Sage 100.
By using Endgrate, you can save time and resources, allowing you to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers. Explore how Endgrate can streamline your integration strategy by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/sage100
- https://help-sage100.na.sage.com/InstallGuide/2022/Content/InstallGuide/Config_ODBC_Driver.htm
- https://help-sage100.na.sage.com/2022/FLOR/index.htm#File_Layouts/Library_Master/SY_SalesTaxSchedule.htm?Highlight=SY_SalesTaxSchedule
- https://help-sage100.na.sage.com/2022/FLOR/index.htm#File_Layouts/Library_Master/SY_SalesTaxScheduleDetail.htm?Highlight=SY_SalesTaxScheduleDetail
- https://help-sage100.na.sage.com/2022/FLOR/index.htm#File_Layouts/Library_Master/SY_SalesTaxCodeDetail.htm?Highlight=SY_SalesTaxCodeDetail
- https://help-sage100.na.sage.com/2022/FLOR/index.htm#File_Layouts/Library_Master/SY_SalesTaxCode.htm?Highlight=SY_SalesTaxCode
Ready to get started?