10 Session Management Security Best Practices
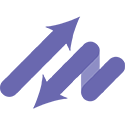

Want to keep your web app secure? Here's how to lock down your session management:
- Use strong, random session IDs
- Set secure cookie settings
- Implement proper session timeouts
- Encrypt all session data transfers
- Create effective logout features
- Prevent session fixation attacks
- Use multi-factor authentication
- Monitor for suspicious activity
- Secure server-side sessions
- Educate users on session safety
Quick Comparison:
Best Practice | Why It Matters | Difficulty |
---|---|---|
Strong session IDs | Prevents guessing/hijacking | Medium |
Secure cookies | Stops unauthorized access | Easy |
Session timeouts | Limits attack windows | Easy |
Data encryption | Protects sensitive info | Medium |
Proper logouts | Fully terminates sessions | Easy |
Anti-fixation | Blocks session takeovers | Medium |
Multi-factor auth | Adds extra security layer | Hard |
Activity monitoring | Catches threats early | Medium |
Server-side security | Protects backend data | Hard |
User education | Creates security-aware users | Medium |
These practices will help you build a fortress around your users' sessions. Don't skimp on security - one breach can cost you big time.
Related video from YouTube
Create Strong Session IDs
Strong session IDs are your first defense against session hijacking. Here's how to make them secure:
- Use a Cryptographically Secure Pseudorandom Number Generator (CSPRNG)
- Make IDs long and complex (at least 16 hex characters)
- Ensure each ID is unique
- Regenerate IDs after login, privilege changes, and password updates
- Keep IDs meaningless (no sensitive info or PII)
- Use generic names for session cookies
- Never put IDs in URLs
These practices make your session IDs tough to guess or exploit. It's a key part of your security strategy.
"Effective session management is vital for maintaining the security, performance, and scalability of web applications."
2. Set Safe Cookie Settings
Cookie settings can make or break your session security. Here's what you need to know:
1. HTTPOnly Flag
Stops scripts from touching your cookies. Use it for all cookies that don't need script access.
2. Secure Flag
Only sends cookies over HTTPS. It's like a bodyguard for your data.
3. SameSite Flag
Blocks cookies from cross-origin requests. It's your shield against CSRF attacks.
4. Expiration
Use session cookies when you can. If you need persistent cookies, give them a strict expiration date.
5. Domain
Leave it empty. This keeps subdomains from snagging your cookie.
Here's a cheat sheet for implementing these settings:
Setting | How to Do It |
---|---|
HTTPOnly | Set-Cookie: id=a3fWa; HttpOnly |
Secure | Set-Cookie: id=a3fWa; Secure |
SameSite | Set-Cookie: id=a3fWa; SameSite=Strict |
Expiration | Set-Cookie: id=a3fWa; Expires=Wed, 21 Oct 2023 07:28:00 GMT |
Domain | Set-Cookie: id=a3fWa; Domain= |
For Java EE apps, set the secure attribute in web.xml:
<session-config>
<cookie-config>
<secure>true</secure>
</cookie-config>
</session-config>
In ASP.NET, add this to Web.config:
<httpCookies requireSSL="true" />
For PHP, set the secure attribute in php.ini:
session.cookie_secure = True
3. Set Proper Session End Times
Setting the right session end times is crucial for app security. Here's how to do it:
For sensitive apps, keep sessions short - 5-15 minutes of inactivity before logout. Less sensitive apps can stretch to 15-30 minutes.
Use both idle and absolute timeouts:
App Type | Idle Timeout | Absolute Timeout |
---|---|---|
High-risk | 5-15 minutes | 1-2 hours |
Low-risk | 15-30 minutes | 4-8 hours |
Warn users before logout to prevent data loss. When a session ends, wipe all related data from client and server sides. Redirect to the login page with a clear message.
Let users choose their timeout length (within limits) and review settings regularly.
"Insufficient session expiration... increases the exposure of other session-based attacks, as for the attacker to be able to reuse a valid session ID and hijack the associated session, it must still be active."
The key? Balance security and user experience. Too short, users get annoyed. Too long, you risk unauthorized access.
4. Protect Session Data Transfer
Keeping session data safe is crucial. Here's how to do it:
Use HTTPS: Encrypt everything with HTTPS. It's like a secret code for your data.
Set up HSTS: This tells browsers to always use HTTPS. It's like a bouncer for your website.
Secure those cookies:
Attribute | What it does |
---|---|
Secure | HTTPS only |
HttpOnly | No JavaScript access |
SameSite | Controls where cookies go |
Encrypt session data: For ASP.NET, use AES encryption. It's like a vault for your data.
VPNs are your friend: On public Wi-Fi? Use a VPN. It's like a private tunnel for your data.
New session IDs: Change them after logins or privilege changes. It's like getting a new key each time.
Do these things, and you'll make life much harder for the bad guys.
"Insufficient session expiration... increases the exposure of other session-based attacks, as for the attacker to be able to reuse a valid session ID and hijack the associated session, it must still be active."
5. Create Effective Logout Features
Good logout features keep user sessions safe. Here's how to nail it:
Make logout obvious: Put a logout button on every page. Users shouldn't have to hunt for it.
Kill the session: When a user logs out, destroy the session everywhere. Here's how in Node.js:
app.get('/logout', (req, res) => {
req.session.destroy(err => {
if (err) {
console.log(err)
} else {
res.clearCookie('connect.sid');
res.redirect('/');
}
});
});
This code wipes out the session and clears the cookie.
Set up auto-logout: Don't count on users to log out. Use timeouts for inactive sessions:
App Type | Timeout |
---|---|
Banking | 5-10 mins |
15-30 mins | |
Social | 1-2 hours |
Handle SSO: With Single Sign-On, logging out of one app should log out of all.
Show feedback: Let users know they've logged out. Redirect them to a logout page or login screen.
Change session IDs: Generate a new ID after each login to block old session ID attacks.
sbb-itb-96038d7
6. Stop Session Fixation Attacks
Hackers can hijack user accounts through session fixation attacks. Here's how to prevent them:
1. Change session IDs after login
This is crucial. Generate a new session ID right after a user logs in. Here's a PHP example:
<?php
session_start();
if (login_successful()) {
session_regenerate_id(true);
$_SESSION['logged_in'] = true;
}
?>
2. Use HTTPS and set secure cookie flags
Encrypt all session data and use these cookie settings:
Flag | Purpose |
---|---|
Secure | HTTPS only |
HttpOnly | No JavaScript access |
SameSite | Limited cross-site requests |
3. Monitor and timeout
Watch for sudden IP or user-agent changes. Set a cutoff for inactive sessions (e.g., 30 minutes).
4. Avoid URL-based session IDs
NEVER put session IDs in URLs. It's like leaving your house key under the doormat.
In 2021, CVS learned this the hard way. A session fixation breach exposed over a billion search queries, including emails and prescriptions. Don't be the next CVS.
Keep your code fresh and test often. Session security isn't a "set it and forget it" deal.
7. Use Multi-Step Login
Want to stop 99.9% of account attacks? Use multi-factor authentication (MFA). It's like adding extra locks to your digital front door.
Here's how MFA works:
You prove who you are in two or more ways. It could be:
- Something you know (like a password)
- Something you have (like your phone)
- Something you are (like your fingerprint)
Setting up MFA isn't rocket science. Here's a quick guide:
1. Pick your factors
Choose which combo of "know", "have", and "are" works best for your team.
2. Choose your tool
There are tons of MFA options out there. Here are a few popular ones:
Tool | Who it's for | Starting cost |
---|---|---|
Google Authenticator | Solo users | Free |
Cisco Duo | Small teams | $3/user/month |
Auth0 | Developers | $35/month |
Okta | Big companies | $3/user/month |
3. Train your people
Show your team how to use MFA and why it matters. Make it clear: this stops bad guys from breaking in.
4. Start small, then grow
Test with a small group first. Iron out the kinks. Then roll it out to everyone.
5. Keep an eye on things
Watch for any hiccups or complaints. Tweak as needed.
Is MFA perfect? Nope. But it's WAY better than passwords alone. Remember: 81% of breaches happen because of weak passwords. MFA fixes that problem.
So, if you want rock-solid session security, MFA is a no-brainer.
8. Watch for Odd Session Activity
Spotting weird user behavior can help you catch security threats. Here's how:
1. Use User Behavior Analytics (UBA)
UBA tools track what users do in real-time. They help you find strange patterns that might mean trouble.
Splunk's UBA, for example, uses machine learning to spot things like:
- Logins from new places
- Sudden jumps in data access
- Activity at odd hours
2. Set up session recordings
These show you exactly how people use your app. Look for:
- Rage clicks (clicking like crazy)
- Weird ways of moving through the app
- Trying to get into off-limits areas
3. Keep an eye on logins
Watch for:
- Failed login attempts
- New devices showing up
- Use of unfamiliar IPs or VPNs
4. Use smart algorithms
Some good ones are:
Algorithm | What it does |
---|---|
K-Nearest Neighbors | Finds outliers based on how far they are from normal |
Isolation Forest | Uses decision trees to separate odd stuff |
One-Class SVM | Learns what's "normal" and flags what's not |
5. Act when something's off
If you see something weird:
- Lock the account
- Make them reset their password
- Add extra login steps
Here's a real example: Under Armour used UBA for their race training plans. They found people weren't using them much, so they made them better. After that, three times as many paid users started using the plans.
Just remember: Weird activity isn't always bad, but it's worth checking out. Stay alert, act fast, and keep your users safe.
9. Keep Server-Side Sessions Safe
Server-side sessions are crucial for user data security. Here's how to do it right:
Make session IDs unguessable
Use a CSPRNG to create IDs at least 128 bits long. Avoid patterns or meaningful info.
Encrypt the sensitive stuff
Don't store secrets in plain text. Encrypt user roles, account IDs, and payment info.
Lock it down with RBAC
Use role-based access control. Review permissions regularly.
Refresh session IDs
Generate new IDs at login, permission changes, and set intervals. This stops session fixation attacks.
Keep an eye out
Watch for red flags:
Flag | Why it matters |
---|---|
Login fails | Possible brute-force |
New devices | Potential account takeover |
Weird IPs | Might be a breach |
Ditch the default storage
Skip MemoryStore for production. Use databases, Redis, or ORM libraries instead.
Set timeouts
End inactive sessions after a set time. It limits damage from stolen session IDs.
OWASP says: "Session IDs should be at least 128 bits long to prevent brute force attacks."
10. Teach Users About Session Safety
User education is crucial for session security. Here's how to do it right:
Run phishing tests
Set up fake phishing emails to test employees. Meet with those who fall for it to prevent future slip-ups.
Keep it simple
Ditch the tech talk. Explain session safety in plain English.
Make it personal
Show how session security impacts work AND personal life. It'll stick better.
Mix up learning methods
Use short videos, quizzes, and real examples. Keeps things interesting.
Cover the essentials
Focus on:
Topic | Why it matters |
---|---|
Strong passwords | Stops easy account hacks |
Secure sharing | Prevents accidental data leaks |
Logging out | Protects shared device sessions |
Spotting phishing | Blocks a common attack method |
Keep it going
Security isn't a one-and-done deal. Schedule regular updates.
Track results
Monitor who finishes training and how they do. Use this to improve your program.
"Security awareness training should not be a compliance tick-box exercise but aim to influence long-term security behaviors."
Conclusion
Session management security isn't a set-it-and-forget-it deal. It's an ongoing process that needs constant attention.
Here's what you need to do:
Action | Why It Matters |
---|---|
Use strong, random session IDs | Stops hackers from guessing |
Always use HTTPS | Keeps data safe in transit |
Set proper timeouts | Limits how long sessions stay open |
Implement secure logouts | Fully ends sessions |
Teach users about session safety | Users become part of your defense |
Even small mistakes can cause big problems. Take T-Mobile Austria in 2018. Hackers got into customer data just by changing a URL digit. Yikes.
To stay safe:
- Do regular security checks
- Keep up with new best practices
- Test for common attacks like session fixation and XSS
Ruggero Contu from Gartner says:
"In 2024, we continue to anticipate the development of cloud security, the spreading use of the zero trust model, an increase in cybersecurity compliance requirements, and a rise in threat detection and response tools."
Bottom line: Stay on your toes. Use these best practices and keep watching for new threats. It's the best way to protect your users' data.
FAQs
What are the security issues with session management?
Session management faces two main threats:
- Session Fixation: Attackers use a known session ID to hijack sessions.
- Session Hijacking: Hackers steal valid session IDs to impersonate users.
These can lead to unauthorized access and data breaches. In 2018, British Airways exposed 380,000 customers' data due to poor session management.
Which session management can reduce security attacks?
To boost security:
- Regenerate Session IDs: Create new IDs after login.
- Use HTTPS: Encrypt all session data.
- Set Short Timeouts: Limit the attack window.
Technique | Purpose | Impact |
---|---|---|
ID Regeneration | Stops fixation | High |
HTTPS | Encrypts data | High |
Timeouts | Limits attacks | Medium |
Related posts
Ready to get started?