How to Create or Update Accounts with the Freshsales API in Python
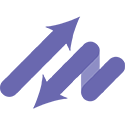
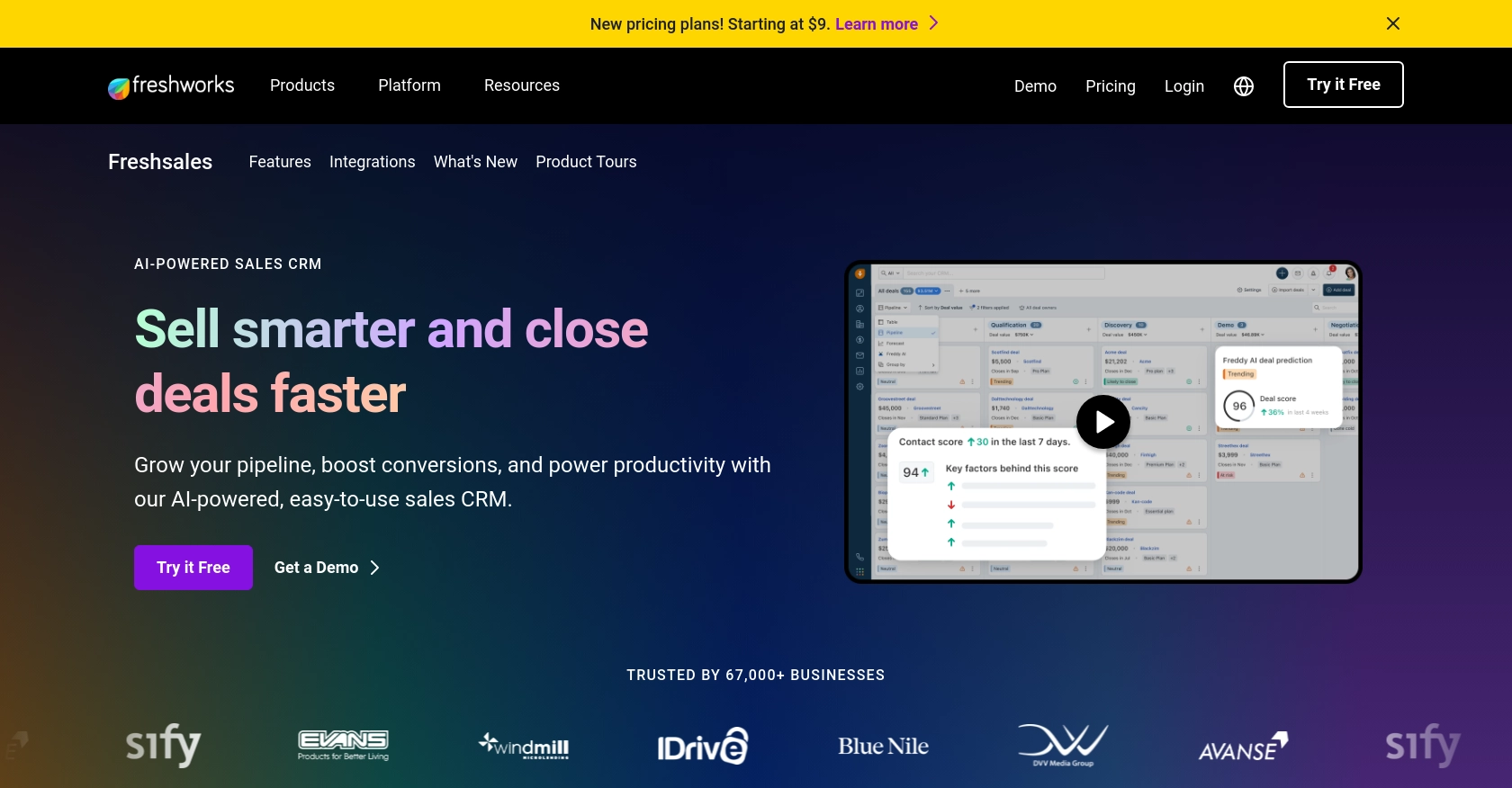
Introduction to Freshsales API Integration
Freshsales is a powerful customer relationship management (CRM) platform designed to help businesses streamline their sales processes. With features like lead scoring, email tracking, and built-in phone capabilities, Freshsales provides a comprehensive solution for managing customer interactions and driving sales growth.
Integrating with the Freshsales API allows developers to automate and enhance CRM functionalities, such as creating or updating account information. For example, a developer might use the Freshsales API to automatically update account details when a customer makes a purchase, ensuring that sales teams have the most up-to-date information at their fingertips.
Setting Up Your Freshsales Test or Sandbox Account
Before you can start integrating with the Freshsales API, you need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data.
Creating a Freshsales Account
If you don't already have a Freshsales account, you can sign up for a free trial on the Freshsales website. Follow these steps to create your account:
- Visit the Freshsales website.
- Click on the "Free Trial" button and fill in the required information, such as your name, email, and company details.
- Submit the form to create your account. You will receive a confirmation email with further instructions.
Accessing the Freshsales Sandbox Environment
Once your account is set up, you can access the sandbox environment to test API interactions. This environment mimics the live system, allowing you to test without impacting real data.
Generating API Key for Freshsales Authentication
Freshsales uses a custom authentication method. Follow these steps to generate your API key:
- Log in to your Freshsales account.
- Navigate to the "Settings" section from the main menu.
- Under "API Settings," find the option to generate an API key.
- Click "Generate" and copy the API key. Store it securely, as you will need it for authenticating your API requests.
With your Freshsales account and API key ready, you can now proceed to make API calls to create or update accounts using Python.
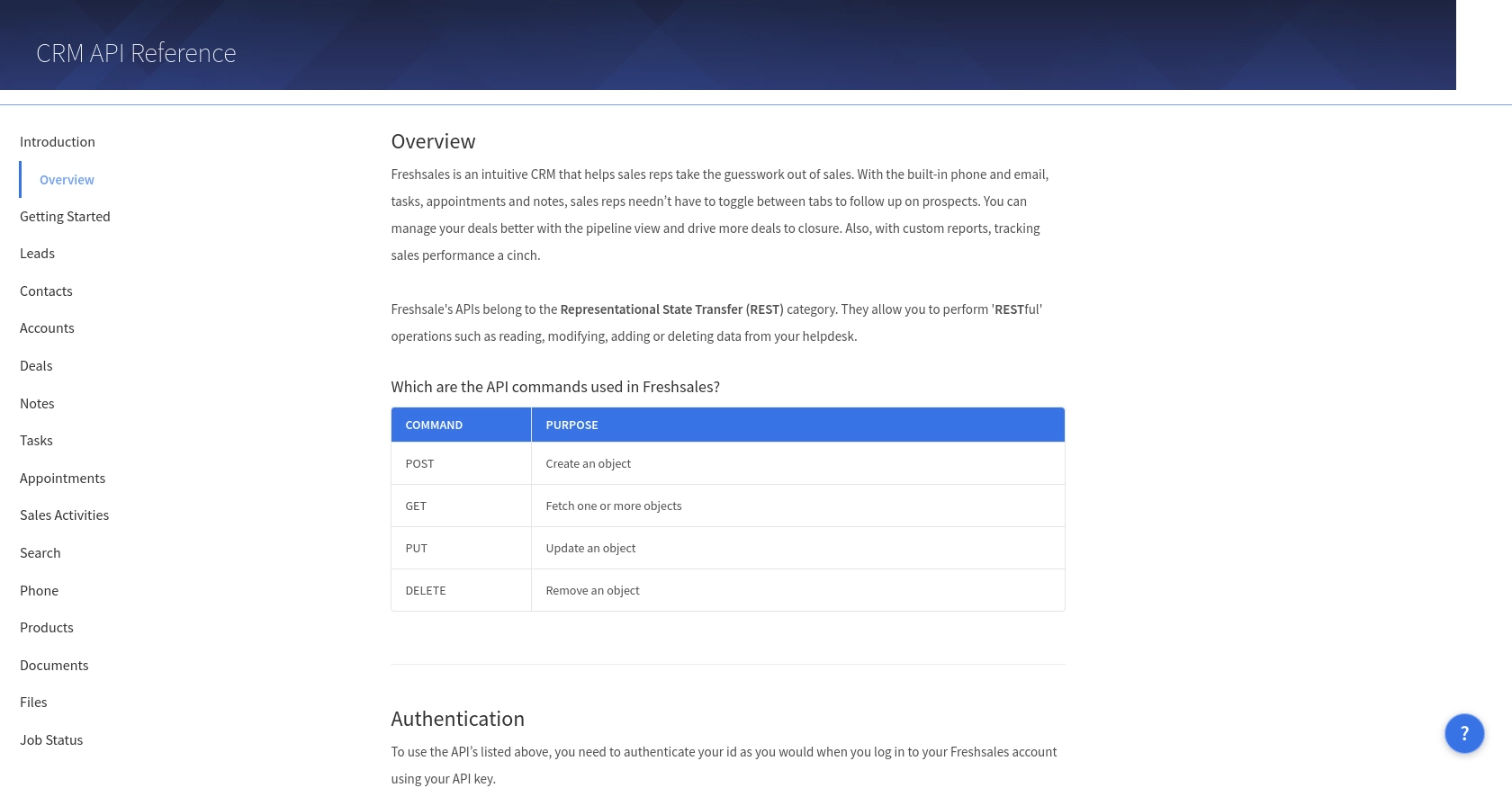
sbb-itb-96038d7
Making API Calls to Create or Update Accounts with Freshsales in Python
To interact with the Freshsales API using Python, you'll need to ensure you have the correct setup and dependencies. This section will guide you through the process of making API calls to create or update account information in Freshsales.
Prerequisites for Freshsales API Integration with Python
Before proceeding, make sure you have the following installed on your machine:
- Python 3.x
- The Python package installer, pip
You'll also need to install the requests
library, which is used to make HTTP requests. You can install it using the following command:
pip install requests
Creating or Updating Accounts with Freshsales API
Now that your environment is set up, you can proceed to create or update accounts using the Freshsales API. Below is a sample Python script to achieve this:
import requests
# Set the Freshsales API endpoint
url = "https://yourdomain.freshsales.io/api/accounts"
# Set the request headers
headers = {
"Content-Type": "application/json",
"Authorization": "Token token=YOUR_API_KEY"
}
# Define the account data
account_data = {
"account": {
"name": "New Account Name",
"industry": "Technology",
"website": "https://example.com"
}
}
# Make a POST request to create a new account
response = requests.post(url, json=account_data, headers=headers)
# Check if the request was successful
if response.status_code == 201:
print("Account created successfully.")
else:
print(f"Failed to create account. Status code: {response.status_code}")
print("Response:", response.json())
Replace YOUR_API_KEY
with the API key you generated earlier. The url
should be updated with your Freshsales domain.
Verifying API Call Success in Freshsales Sandbox
After running the script, you can verify the success of the API call by checking the Freshsales sandbox environment. If the account creation is successful, the new account should appear in your sandbox.
Handling Errors and Error Codes in Freshsales API
It's important to handle potential errors when making API calls. The Freshsales API will return various status codes to indicate the success or failure of your request. Common status codes include:
- 201: Account created successfully.
- 400: Bad request, often due to missing or incorrect parameters.
- 401: Unauthorized, indicating an issue with authentication.
- 404: Not found, indicating the endpoint is incorrect.
Always check the response and handle errors appropriately to ensure robust integration.
Best Practices for Freshsales API Integration
When integrating with the Freshsales API, it's crucial to follow best practices to ensure a secure and efficient implementation. Here are some key considerations:
Securely Storing Freshsales API Credentials
Always store your Freshsales API key securely. Avoid hardcoding it directly in your scripts. Instead, use environment variables or secure vaults to manage sensitive information.
Handling Freshsales API Rate Limits
Be mindful of Freshsales API rate limits to prevent your application from being throttled. Implement logic to handle rate limiting by checking the response headers for rate limit information and using exponential backoff strategies if necessary.
Transforming and Standardizing Data Fields
Ensure that data fields are transformed and standardized before sending them to Freshsales. This includes formatting dates, normalizing text fields, and validating data types to match Freshsales requirements.
Conclusion and Call to Action
Integrating with the Freshsales API using Python allows developers to automate CRM processes, enhancing the efficiency of sales operations. By following the steps outlined in this guide, you can create or update account information seamlessly.
For developers looking to streamline multiple integrations, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Endgrate provides a unified API endpoint that simplifies the integration experience, making it easier to manage connections across various platforms.
Visit Endgrate to learn more about how you can enhance your integration capabilities and provide a seamless experience for your customers.
Read More
Ready to get started?