How to Create or Update Contacts with the Microsoft Dynamics 365 Business Central API in PHP
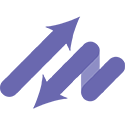
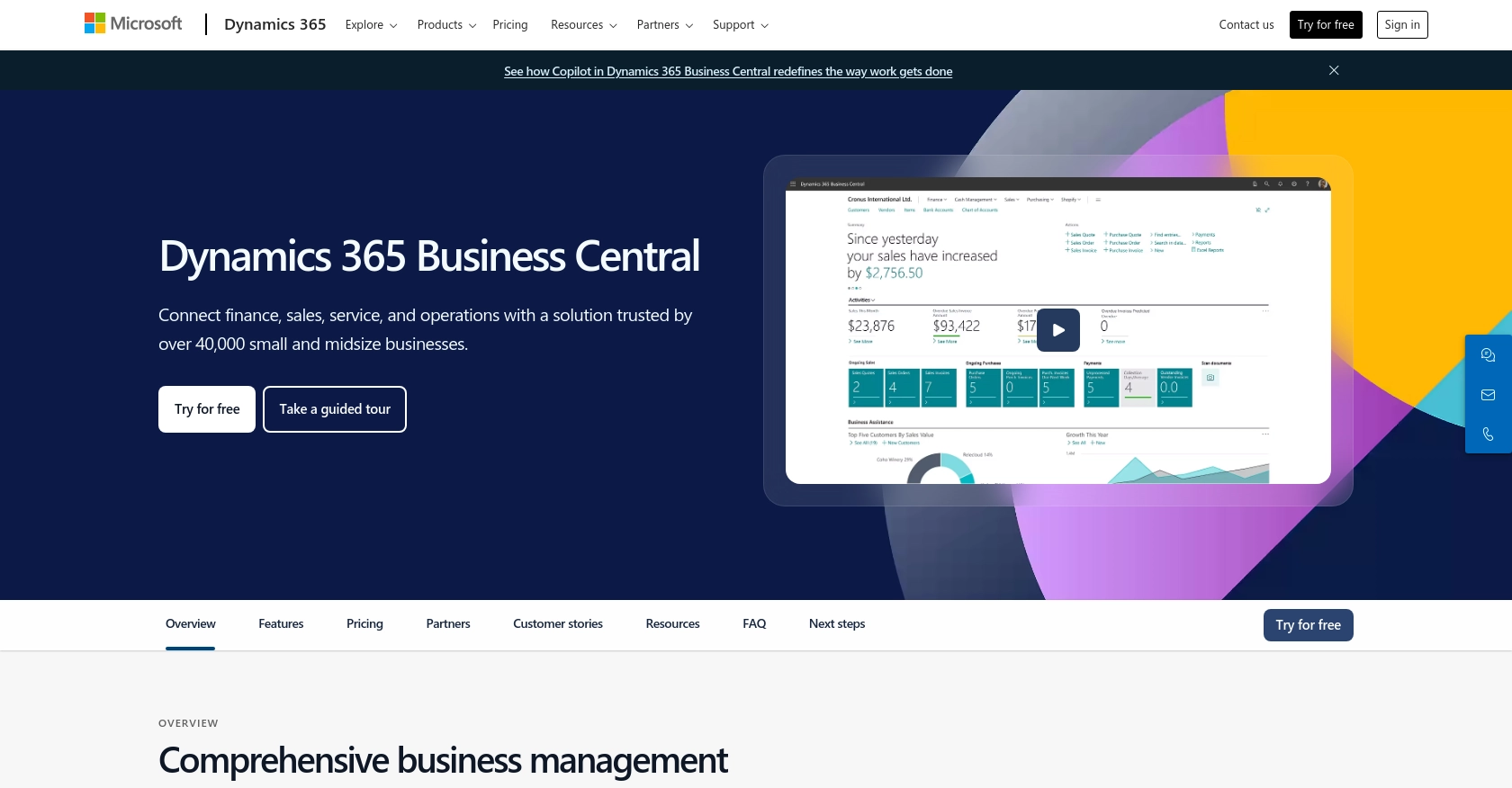
Introduction to Microsoft Dynamics 365 Business Central
Microsoft Dynamics 365 Business Central is a comprehensive business management solution designed for small to medium-sized enterprises. It integrates various business processes, including finance, sales, service, and operations, into a single platform, enabling companies to streamline their operations and make informed decisions.
Developers may want to connect with Microsoft Dynamics 365 Business Central to automate and enhance business workflows. For example, integrating with its API allows developers to create or update contact information directly from external systems, ensuring that customer data is always up-to-date and accessible across the organization.
Setting Up a Microsoft Dynamics 365 Business Central Sandbox Account
Before you can start integrating with the Microsoft Dynamics 365 Business Central API, you'll need to set up a sandbox account. This allows you to test your integration without affecting live data. Microsoft provides a free trial that you can use to explore the platform and its capabilities.
Step-by-Step Guide to Creating a Microsoft Dynamics 365 Business Central Sandbox Account
-
Sign Up for a Free Trial:
Visit the Microsoft Dynamics 365 Business Central website and sign up for a free trial. Follow the instructions to create your account.
-
Access the Admin Center:
Once your account is set up, log in to the Microsoft 365 Admin Center. Here, you can manage your Dynamics 365 environment.
-
Create a Sandbox Environment:
Navigate to the Dynamics 365 admin center and select 'Environments'. Click on 'New' to create a new environment. Choose 'Sandbox' as the type and provide a name for your environment.
-
Configure the Sandbox:
After creating the sandbox, you can configure it to suit your testing needs. This includes setting up sample data and customizing settings.
Registering an App for OAuth Authentication
To interact with the Microsoft Dynamics 365 Business Central API, you'll need to register an application in your Microsoft Entra ID tenant. This will allow you to use OAuth for authentication.
-
Register Your Application:
Go to the Azure portal and navigate to 'Azure Active Directory'. Select 'App registrations' and click 'New registration'.
-
Provide App Details:
Enter a name for your app and choose the supported account types. For redirect URI, you can use
https://localhost
during development. -
Configure API Permissions:
Once registered, go to 'API permissions' and add the necessary permissions for Dynamics 365. Ensure you have 'Access Dynamics 365 as organization users' permission.
-
Generate Client Secret:
Under 'Certificates & secrets', create a new client secret. Copy the secret value as it will be used in your PHP application for authentication.
For more detailed instructions on setting up OAuth authentication, refer to the official documentation: Use OAuth authentication with Microsoft Dataverse.
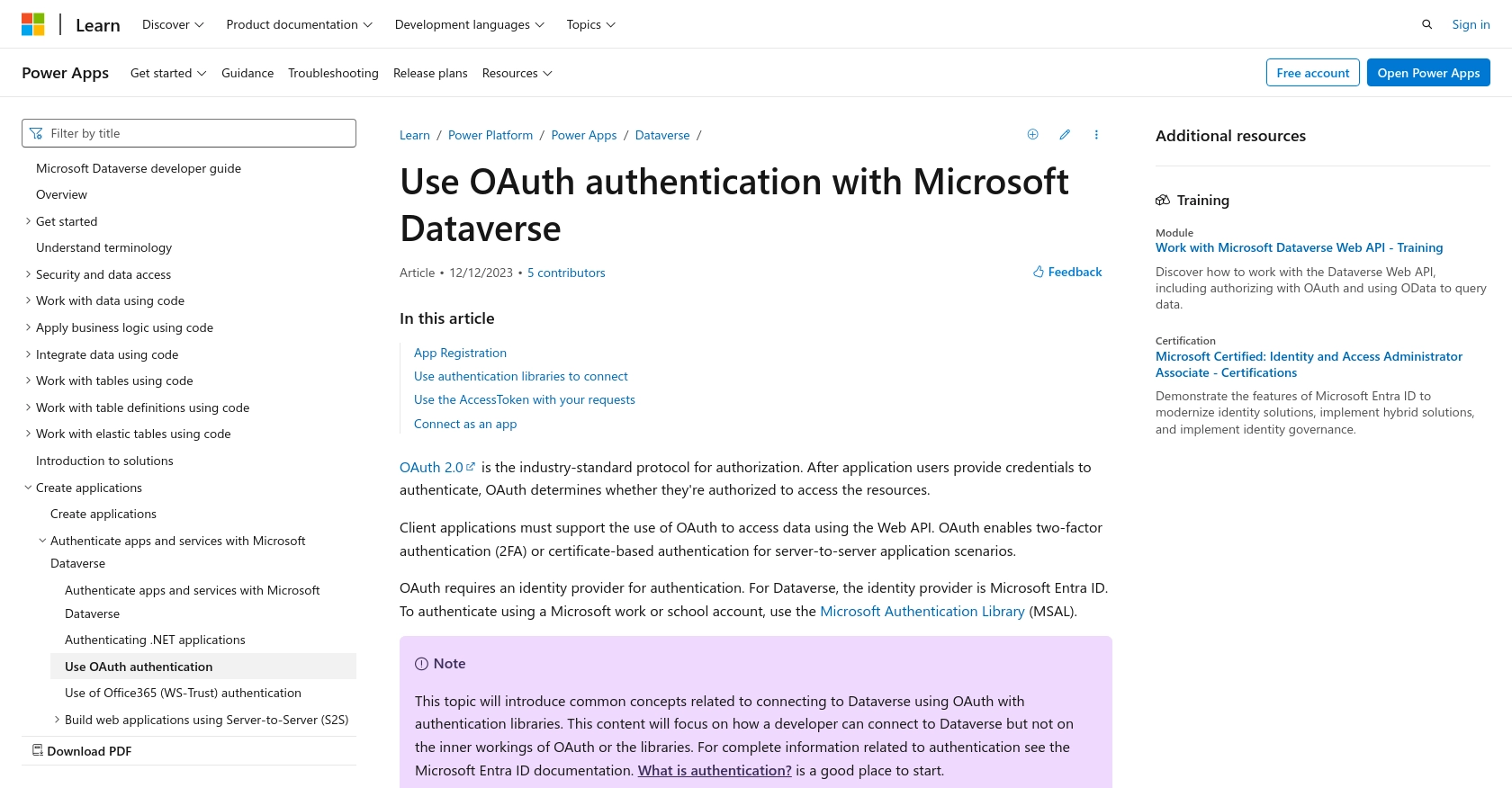
sbb-itb-96038d7
Making API Calls to Microsoft Dynamics 365 Business Central with PHP
To interact with the Microsoft Dynamics 365 Business Central API using PHP, you'll need to set up your environment and write code to perform API requests. This section will guide you through the process of creating or updating contacts using PHP.
Setting Up Your PHP Environment
Before making API calls, ensure you have the following prerequisites:
- PHP 7.4 or higher installed on your machine.
- Composer for managing dependencies.
- The
guzzlehttp/guzzle
library for making HTTP requests. Install it using Composer:
composer require guzzlehttp/guzzle
Creating or Updating Contacts in Microsoft Dynamics 365 Business Central
Follow these steps to create or update contacts using the API:
-
Set Up Authentication:
Use the OAuth token obtained during the app registration process. This token will be included in the request headers for authentication.
-
Define the API Endpoint:
The endpoint for creating or updating contacts is:
POST https://{businesscentralPrefix}/api/v2.0/companies({id})/contacts({id})
-
Write the PHP Code:
Create a PHP script to make the API call:
require 'vendor/autoload.php'; use GuzzleHttp\Client; $client = new Client(); $token = 'Your_OAuth_Token'; // Replace with your OAuth token $businessCentralPrefix = 'your_business_central_prefix'; $companyId = 'your_company_id'; $contactId = 'your_contact_id'; $url = "https://{$businessCentralPrefix}/api/v2.0/companies({$companyId})/contacts({$contactId})"; $contactData = [ 'id' => '5d115c9c-44e3-ea11-bb43-000d3a2feca1', 'number' => '108001', 'type' => 'Company', 'displayName' => 'CRONUS USA, Inc.', 'companyNumber' => '17806', 'companyName' => 'CRONUS US', 'businessRelation' => 'Vendor', 'addressLine1' => '7122 South Ashford Street', 'city' => 'Atlanta', 'state' => 'GA', 'country' => 'US', 'postalCode' => '31772', 'phoneNumber' => '+1 425 555 0100', 'email' => 'ah@contoso.com' ]; $response = $client->post($url, [ 'headers' => [ 'Authorization' => "Bearer {$token}", 'Content-Type' => 'application/json' ], 'json' => $contactData ]); if ($response->getStatusCode() == 201) { echo "Contact created or updated successfully."; } else { echo "Failed to create or update contact."; }
-
Run the Script:
Execute the PHP script from the command line:
php create_update_contact.php
Verifying the API Call
After running the script, verify that the contact has been created or updated in your Microsoft Dynamics 365 Business Central sandbox environment. Check the response code and message to ensure the operation was successful.
Handling Errors and Response Codes
It's crucial to handle potential errors when making API calls. Common HTTP status codes include:
- 201 Created: The contact was successfully created.
- 400 Bad Request: The request was malformed.
- 401 Unauthorized: Authentication failed. Check your OAuth token.
- 404 Not Found: The specified resource could not be found.
For more detailed error handling, refer to the official documentation: Create contacts - Business Central.
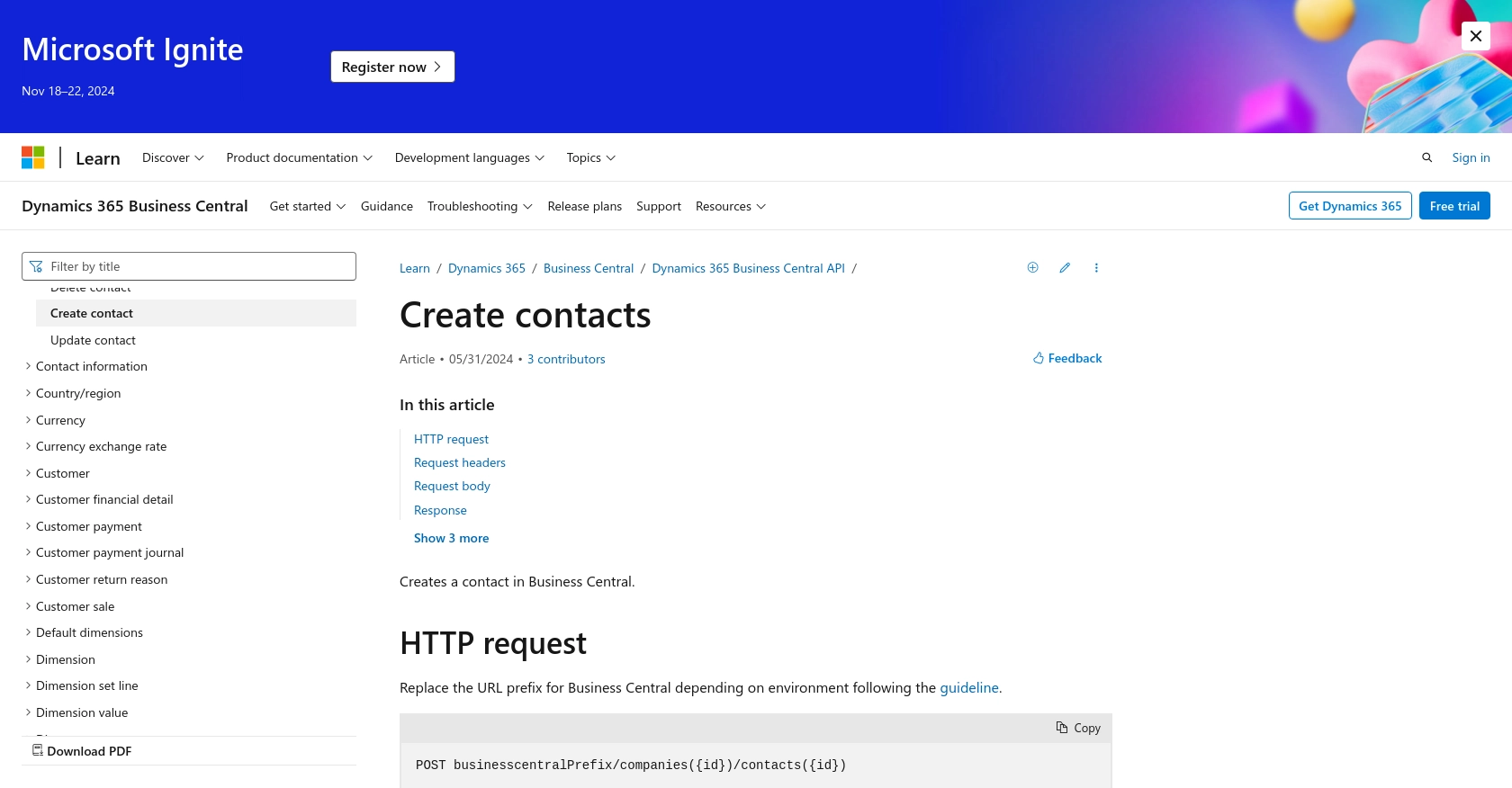
Conclusion and Best Practices for Integrating with Microsoft Dynamics 365 Business Central API
Integrating with the Microsoft Dynamics 365 Business Central API using PHP allows developers to automate and streamline business processes, ensuring that contact information is consistently up-to-date across platforms. By following the steps outlined in this guide, you can efficiently create or update contacts, enhancing your organization's workflow.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store OAuth tokens and client secrets securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limiting: Be aware of any rate limits imposed by the API and implement retry logic or backoff strategies to handle HTTP 429 Too Many Requests responses gracefully.
- Standardize Data Formats: Ensure that data fields are consistently formatted to avoid errors during API interactions and maintain data integrity across systems.
Leverage Endgrate for Streamlined Integration Solutions
While building integrations manually can be effective, it can also be time-consuming and complex. Consider using Endgrate to simplify your integration processes. Endgrate offers a unified API endpoint that connects to multiple platforms, including Microsoft Dynamics 365 Business Central, allowing you to focus on your core product while outsourcing integration tasks.
With Endgrate, you can build once for each use case and enjoy an intuitive integration experience for your customers. Save time and resources by leveraging Endgrate's robust integration capabilities.
Visit Endgrate to learn more about how you can enhance your integration strategy.
Read More
- https://endgrate.com/provider/microsoftdynamics365businesscentral
- https://learn.microsoft.com/en-us/power-apps/developer/data-platform/authenticate-oauth
- https://learn.microsoft.com/en-us/graph/use-the-api
- https://learn.microsoft.com/en-us/dynamics365/business-central/dev-itpro/api-reference/v2.0/api/dynamics_contact_create
Ready to get started?