How to Create or Update Items with the Sage 100 API in Python
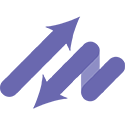
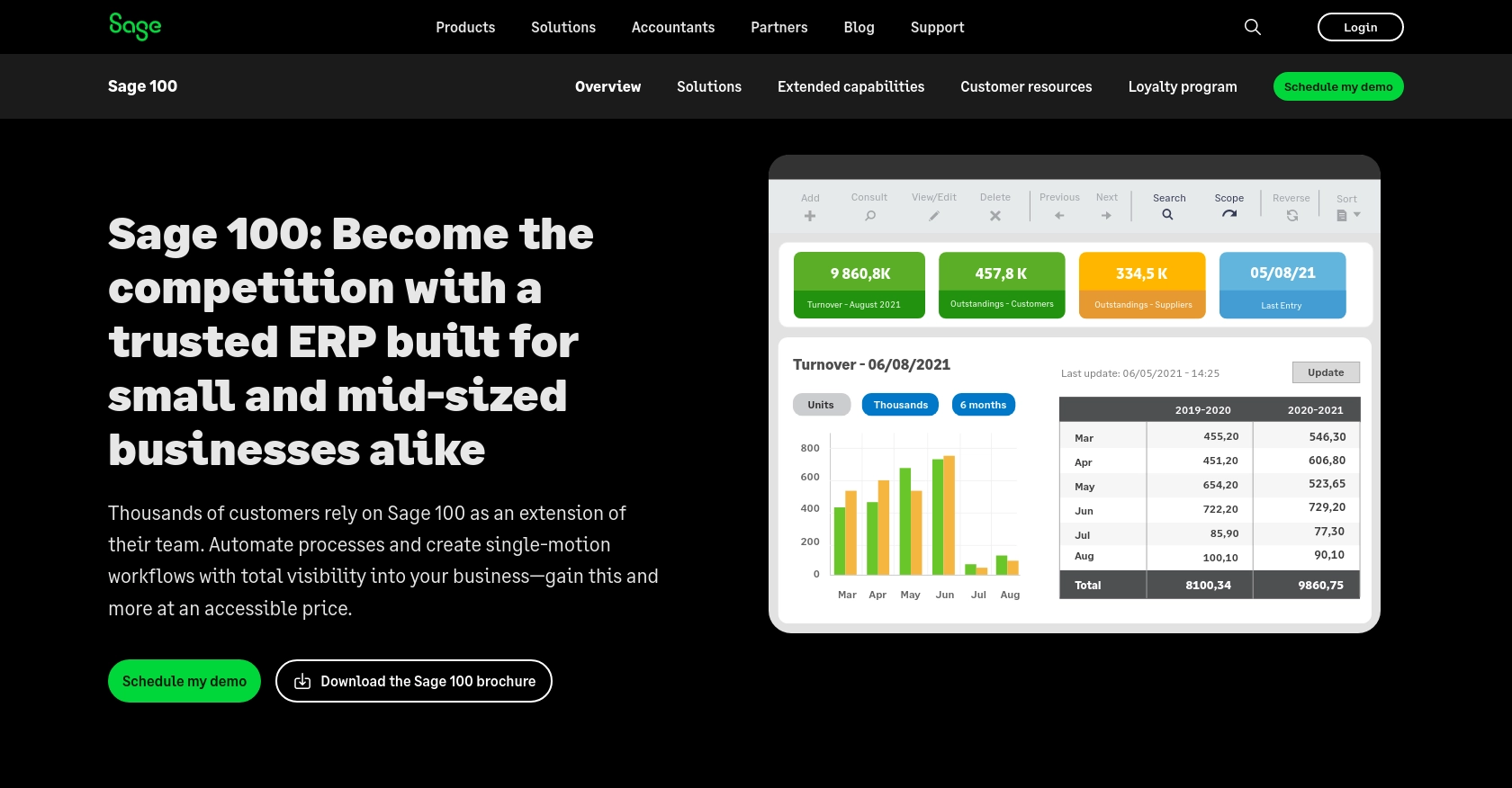
Introduction to Sage 100 API Integration
Sage 100 is a comprehensive enterprise resource planning (ERP) software solution designed to help businesses manage their accounting, inventory, and operations efficiently. With its robust features and customizable options, Sage 100 is a popular choice for small to medium-sized businesses looking to streamline their processes.
Integrating with the Sage 100 API allows developers to automate and enhance various business operations, such as inventory management. For example, you can create or update items in the Sage 100 system using Python, enabling seamless synchronization of product data across different platforms and improving inventory accuracy.
Setting Up Your Sage 100 Test Environment
Before you can start integrating with the Sage 100 API, it's essential to set up a test environment. This will allow you to safely experiment with API calls without affecting your live data. Sage 100 provides a way to configure a test environment using the ODBC driver, which is crucial for accessing the database and performing operations like creating or updating items.
Installing and Configuring the Sage 100 ODBC Driver
To interact with the Sage 100 API, you need to install and configure the Sage 100 ODBC driver. Follow these steps to set up the driver:
- Ensure the Sage 100 ODBC driver is installed on your system. You can download it from the Sage 100 website or contact your system administrator for assistance.
- Access the ODBC Data Source Administrator on your system. You can find this in the Control Panel under Administrative Tools.
- Create a new Data Source Name (DSN) that connects to the Sage 100 ERP system. Provide the correct server, database, and authentication settings.
- Test the connection to ensure it is successful. This will confirm that the ODBC driver is correctly configured.
For detailed instructions, refer to the Sage 100 ODBC Driver Configuration Guide.
Creating a Sage 100 Test Account
Once the ODBC driver is set up, you need to create a test account within Sage 100:
- Log in to your Sage 100 system with administrative privileges.
- Navigate to the Library Master module and select the User Maintenance option.
- Create a new user account specifically for testing purposes. Assign the necessary permissions to access and modify inventory data.
- Ensure that the test account is configured to use the client/server ODBC driver.
Configuring Sage 100 for API Access
To enable API access, configure the Sage 100 system to allow external connections:
- In Sage 100, go to Library Master > Setup > System Configuration.
- On the ODBC Driver tab, check the "Enable C/S ODBC Driver" option.
- Enter the server name or IP address where the ODBC application or service is running.
- Specify the server port, or leave it blank to use the default port, 20222.
- Save the configuration and restart the Sage 100 client to apply the changes.
With these steps completed, your Sage 100 test environment is ready for API integration using Python.
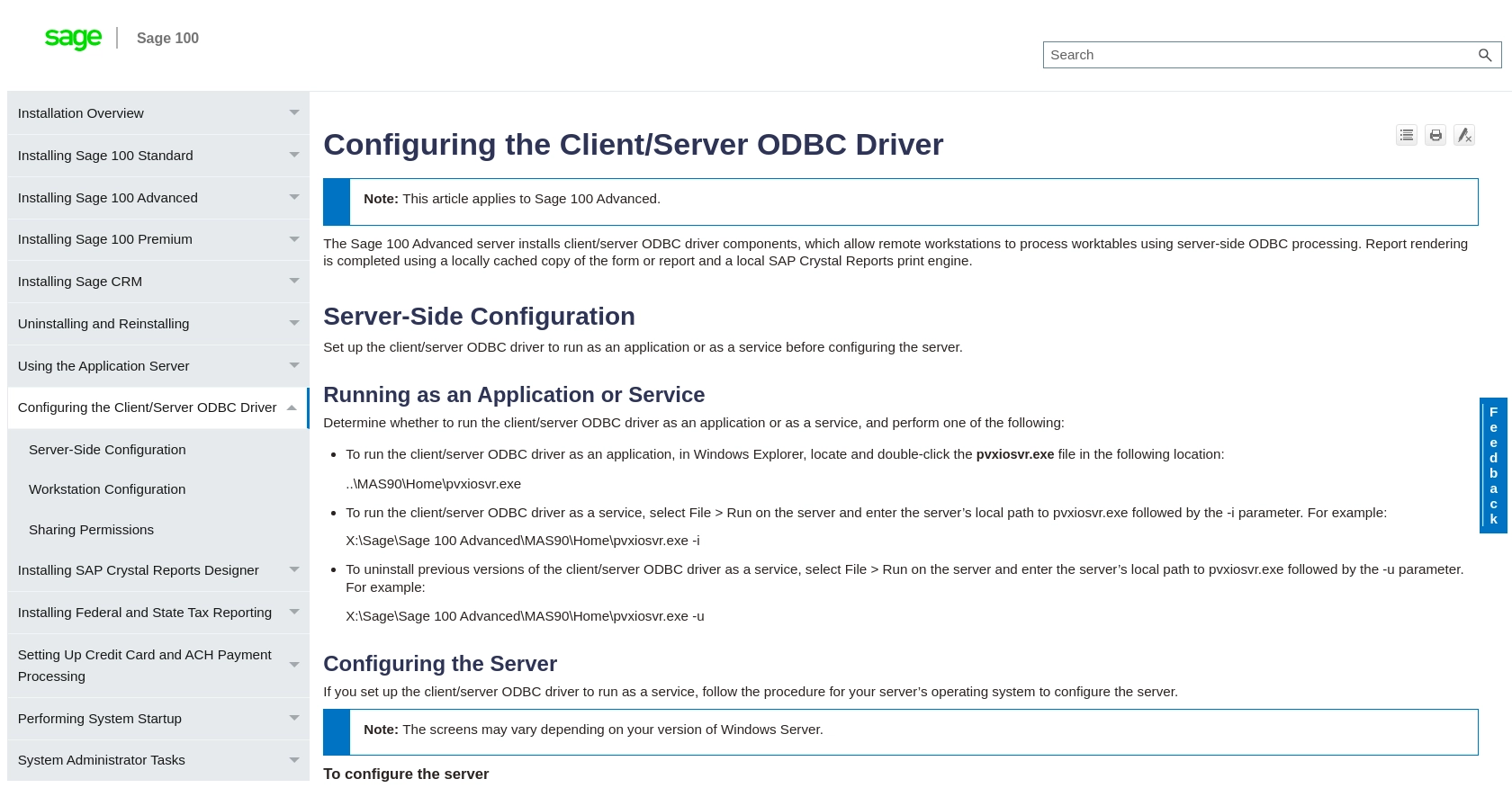
sbb-itb-96038d7
Making API Calls to Create or Update Items in Sage 100 Using Python
To interact with the Sage 100 API for creating or updating items, you'll need to use Python to establish a connection and execute the necessary operations. This section will guide you through the process, including setting up your Python environment and writing the code to perform API calls.
Setting Up Your Python Environment for Sage 100 API Integration
Before making API calls, ensure your Python environment is correctly set up. You'll need Python 3.x and the necessary libraries to interact with the Sage 100 API via ODBC.
- Install Python 3.x from the official Python website if it's not already installed on your system.
- Use pip to install the
pyodbc
library, which allows Python to connect to databases using ODBC:
pip install pyodbc
Writing Python Code to Create or Update Items in Sage 100
With your environment set up, you can now write the Python code to create or update items in Sage 100. Below is an example of how to connect to the Sage 100 database and perform these operations.
import pyodbc
# Define the connection string
dsn = 'Your_DSN_Name'
user = 'Your_Username'
password = 'Your_Password'
# Establish the connection
connection = pyodbc.connect(f'DSN={dsn};UID={user};PWD={password}')
# Create a cursor object
cursor = connection.cursor()
# Define the SQL query to create or update an item
item_code = 'ITEM001'
item_description = 'New Item Description'
query = f"""
UPDATE CI_Item
SET ItemDescription = '{item_description}'
WHERE ItemCode = '{item_code}';
IF @@ROWCOUNT = 0
BEGIN
INSERT INTO CI_Item (ItemCode, ItemDescription)
VALUES ('{item_code}', '{item_description}');
END
"""
# Execute the query
cursor.execute(query)
# Commit the transaction
connection.commit()
# Close the connection
cursor.close()
connection.close()
print("Item created or updated successfully.")
In this code, we first establish a connection to the Sage 100 database using the ODBC driver. We then create a cursor object to execute SQL queries. The example query checks if an item with a specific code exists and updates it; if not, it inserts a new item. Finally, we commit the transaction and close the connection.
Verifying API Call Success in Sage 100
After running the Python script, verify the success of the operation by checking the Sage 100 system:
- Log in to your Sage 100 test environment.
- Navigate to the inventory module and search for the item code used in the script.
- Confirm that the item description has been updated or the new item has been created.
Handling Errors and Exceptions in Sage 100 API Calls
It's crucial to handle potential errors when making API calls. Use try-except blocks in Python to catch exceptions and manage errors gracefully:
try:
# Execute the query
cursor.execute(query)
connection.commit()
except pyodbc.Error as e:
print(f"An error occurred: {e}")
finally:
cursor.close()
connection.close()
By incorporating error handling, you can ensure that your application remains robust and provides meaningful feedback in case of issues.
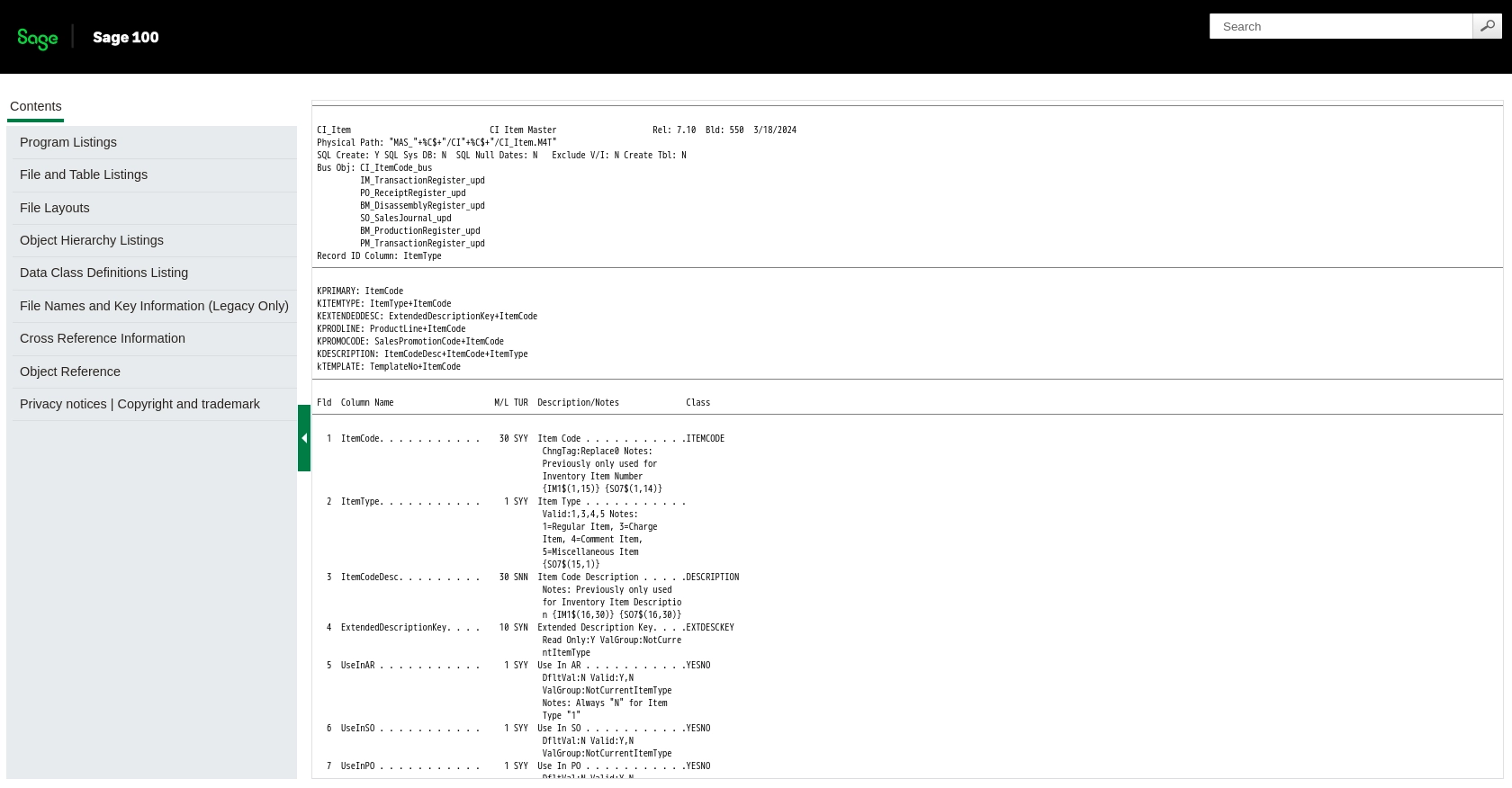
Best Practices for Sage 100 API Integration
When integrating with the Sage 100 API, it's essential to follow best practices to ensure a secure and efficient implementation. Here are some key considerations:
- Securely Store Credentials: Always store user credentials securely, using encryption and environment variables to protect sensitive information.
- Handle Rate Limiting: Be aware of any rate limits imposed by the Sage 100 API and implement retry logic to handle requests gracefully. Refer to the API documentation for specific rate limit details.
- Data Standardization: Ensure that data fields are standardized and transformed as needed to maintain consistency across different systems.
- Error Handling: Implement comprehensive error handling to manage exceptions and provide meaningful feedback to users.
Streamline Your Integrations with Endgrate
Building and maintaining integrations can be time-consuming and complex. With Endgrate, you can simplify the process by leveraging a single API endpoint to connect with multiple platforms, including Sage 100.
Endgrate allows you to focus on your core product by outsourcing integrations, saving time and resources. Whether you're dealing with Sage 100 or other platforms, Endgrate provides an intuitive integration experience that enhances your product's capabilities.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?