How to Create or Update Leads with the Lemlist API in PHP
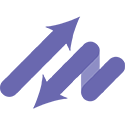
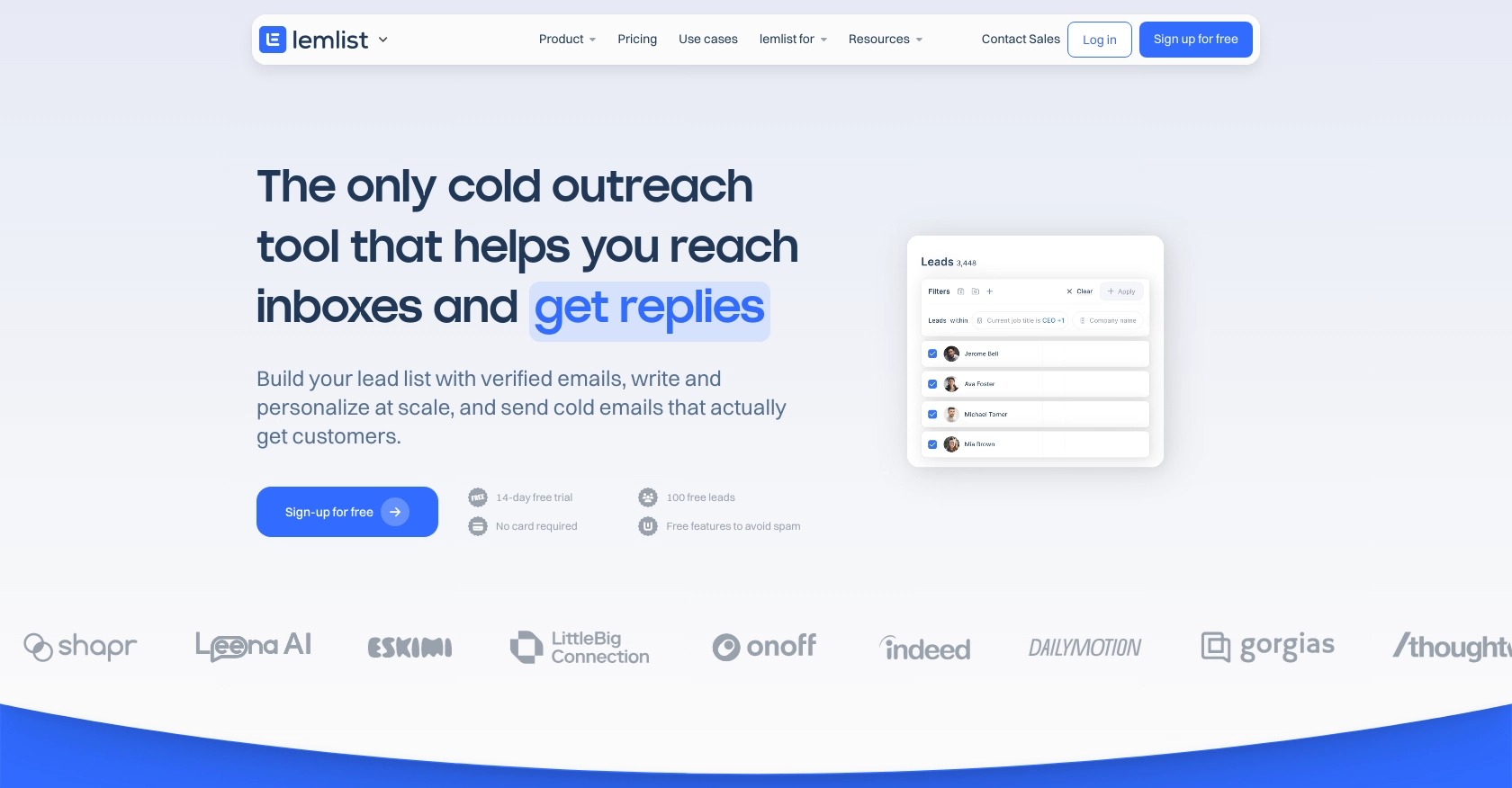
Introduction to Lemlist API for Lead Management
Lemlist is a powerful email outreach platform designed to help businesses personalize and automate their email campaigns. With its robust features, Lemlist enables users to engage with leads more effectively, track campaign performance, and optimize outreach strategies.
Integrating with Lemlist's API allows developers to streamline lead management processes by automating the creation and updating of leads. For example, a developer might use the Lemlist API to automatically add new leads from a CRM system or update existing lead information based on recent interactions, ensuring that the sales team always has the most current data.
Setting Up Your Lemlist Account for API Access
Before you can start creating or updating leads with the Lemlist API, you'll need to set up your Lemlist account and obtain the necessary API key for authentication. Lemlist uses an API key-based authentication method, which simplifies the process of connecting to their API.
Creating a Lemlist Account
If you don't already have a Lemlist account, you'll need to sign up for one. Visit the Lemlist website and follow the instructions to create a free account. Once your account is set up, you can log in to access the dashboard.
Generating Your Lemlist API Key
To interact with the Lemlist API, you'll need an API key. Follow these steps to generate your API key:
- Log in to your Lemlist account and navigate to the settings section.
- Look for the API settings or integrations tab.
- Click on the option to generate a new API key.
- Copy the generated API key and store it securely, as you'll need it to authenticate your API requests.
Testing Your Lemlist API Key
Once you have your API key, it's a good idea to test it to ensure it's working correctly. You can do this by making a simple API call to verify your credentials. Here's a basic example using PHP:
$apiKey = 'your_api_key_here';
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, 'https://api.lemlist.com/api_endpoint');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Authorization: Bearer ' . $apiKey,
]);
$response = curl_exec($ch);
curl_close($ch);
if ($response) {
echo 'API key is valid!';
} else {
echo 'Failed to authenticate with Lemlist API.';
}
Replace your_api_key_here
with the API key you generated. This code snippet makes a basic request to the Lemlist API to verify that your API key is valid.
With your Lemlist account set up and your API key ready, you're now prepared to start integrating with the Lemlist API to create or update leads using PHP.
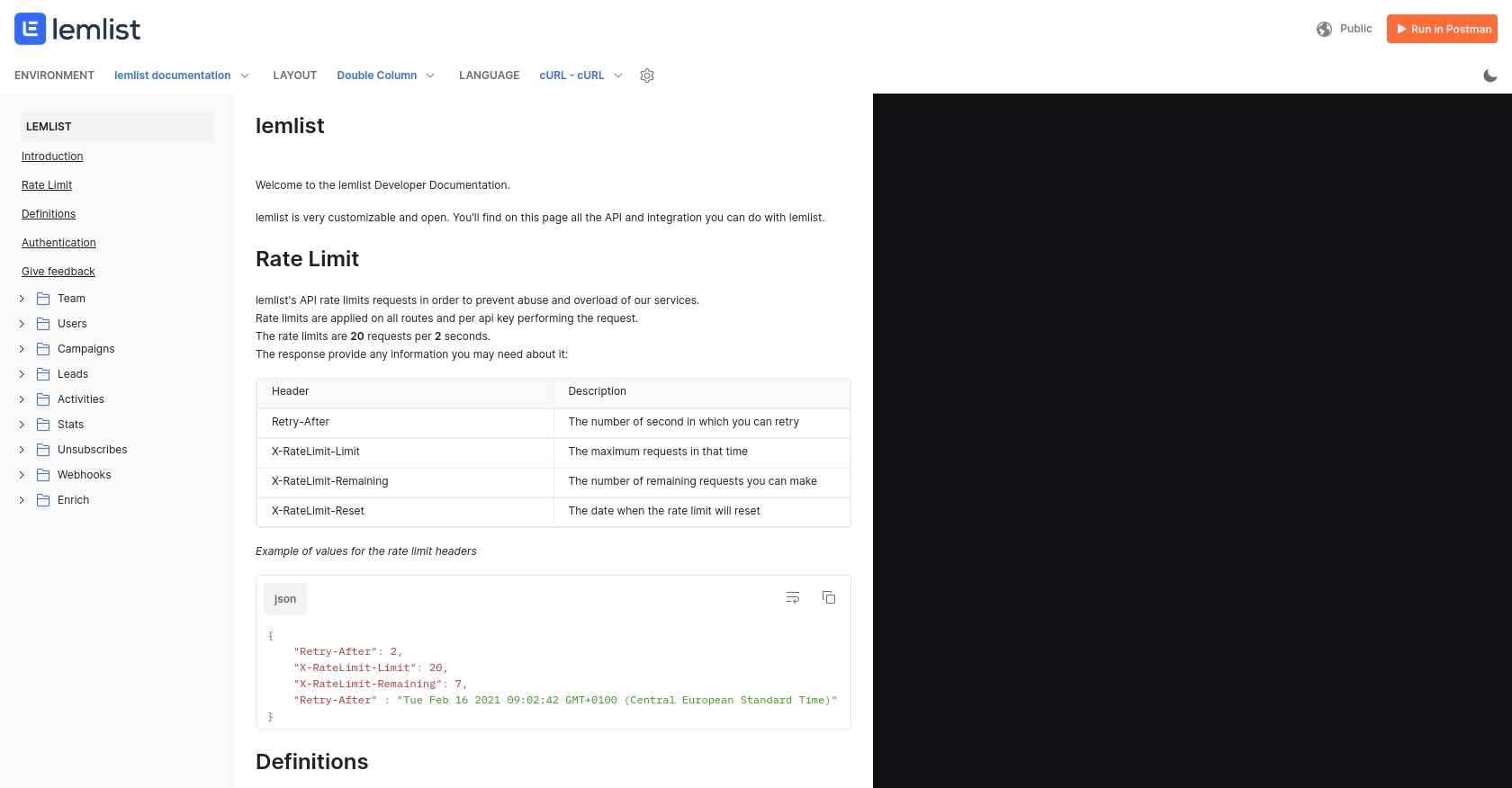
sbb-itb-96038d7
Making API Calls to Create or Update Leads with Lemlist in PHP
To effectively interact with the Lemlist API for creating or updating leads, you'll need to use PHP to make HTTP requests. This section will guide you through the process of setting up your PHP environment, making the necessary API calls, and handling responses.
Setting Up Your PHP Environment for Lemlist API Integration
Before you begin, ensure that you have PHP installed on your machine. You can verify this by running php -v
in your terminal. Additionally, you'll need the cURL extension enabled, which is typically included with PHP installations.
Creating or Updating Leads with Lemlist API Using PHP
To create or update leads, you'll need to make POST requests to the Lemlist API endpoint. Below is a sample PHP script demonstrating how to perform this action:
$apiKey = 'your_api_key_here';
$leadData = [
'email' => 'lead@example.com',
'firstName' => 'John',
'lastName' => 'Doe',
// Add other lead properties as needed
];
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, 'https://api.lemlist.com/api_endpoint');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Authorization: Bearer ' . $apiKey,
'Content-Type: application/json'
]);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($leadData));
$response = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
curl_close($ch);
if ($httpCode == 200) {
echo 'Lead created or updated successfully!';
} else {
echo 'Failed to create or update lead. HTTP Status Code: ' . $httpCode;
}
Replace your_api_key_here
with your actual API key. This script initializes a cURL session, sets the necessary options for a POST request, and sends lead data to the Lemlist API.
Handling API Responses and Errors
After making the API call, it's crucial to handle the response appropriately. The example above checks the HTTP status code to determine if the request was successful. A status code of 200 indicates success, while other codes may indicate errors.
For more detailed error handling, you can parse the response body to extract error messages or codes provided by the Lemlist API. This will help you diagnose issues and improve the robustness of your integration.
Verifying Successful API Requests in Lemlist
To ensure that your API calls are successful, you can log in to your Lemlist account and check the leads section. Newly created or updated leads should appear there, reflecting the changes made by your API requests.
By following these steps, you can efficiently manage leads in Lemlist using PHP, streamlining your email outreach and lead management processes.
Conclusion: Best Practices for Lemlist API Integration in PHP
Integrating with the Lemlist API using PHP can significantly enhance your lead management processes by automating the creation and updating of leads. To ensure a smooth integration, consider the following best practices:
Securely Storing Lemlist API Credentials
Always store your API key securely. Avoid hardcoding it directly into your scripts. Instead, use environment variables or secure vaults to manage sensitive information.
Handling Lemlist API Rate Limits
Be mindful of any rate limits imposed by the Lemlist API. Implement logic to handle rate limit responses gracefully, such as retrying requests after a delay. Check the Lemlist API documentation for specific rate limit details.
Standardizing Data Fields for Lemlist API
Ensure that the data you send to the Lemlist API is standardized and validated. This helps prevent errors and ensures consistency in your lead data.
Transforming Data for Lemlist API Compatibility
When integrating with multiple systems, you may need to transform data to match the Lemlist API's expected format. Use mapping functions or middleware to handle these transformations efficiently.
Enhancing Your Integration Strategy with Endgrate
Consider using Endgrate to simplify your integration efforts. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Endgrate provides a unified API endpoint that connects to multiple platforms, including Lemlist, offering an intuitive integration experience for your customers.
By following these best practices and leveraging tools like Endgrate, you can optimize your Lemlist API integration, ensuring efficient and effective lead management.
Read More
Ready to get started?