How to Create or Update Records with the Postgres API in Javascript
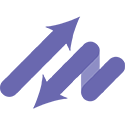
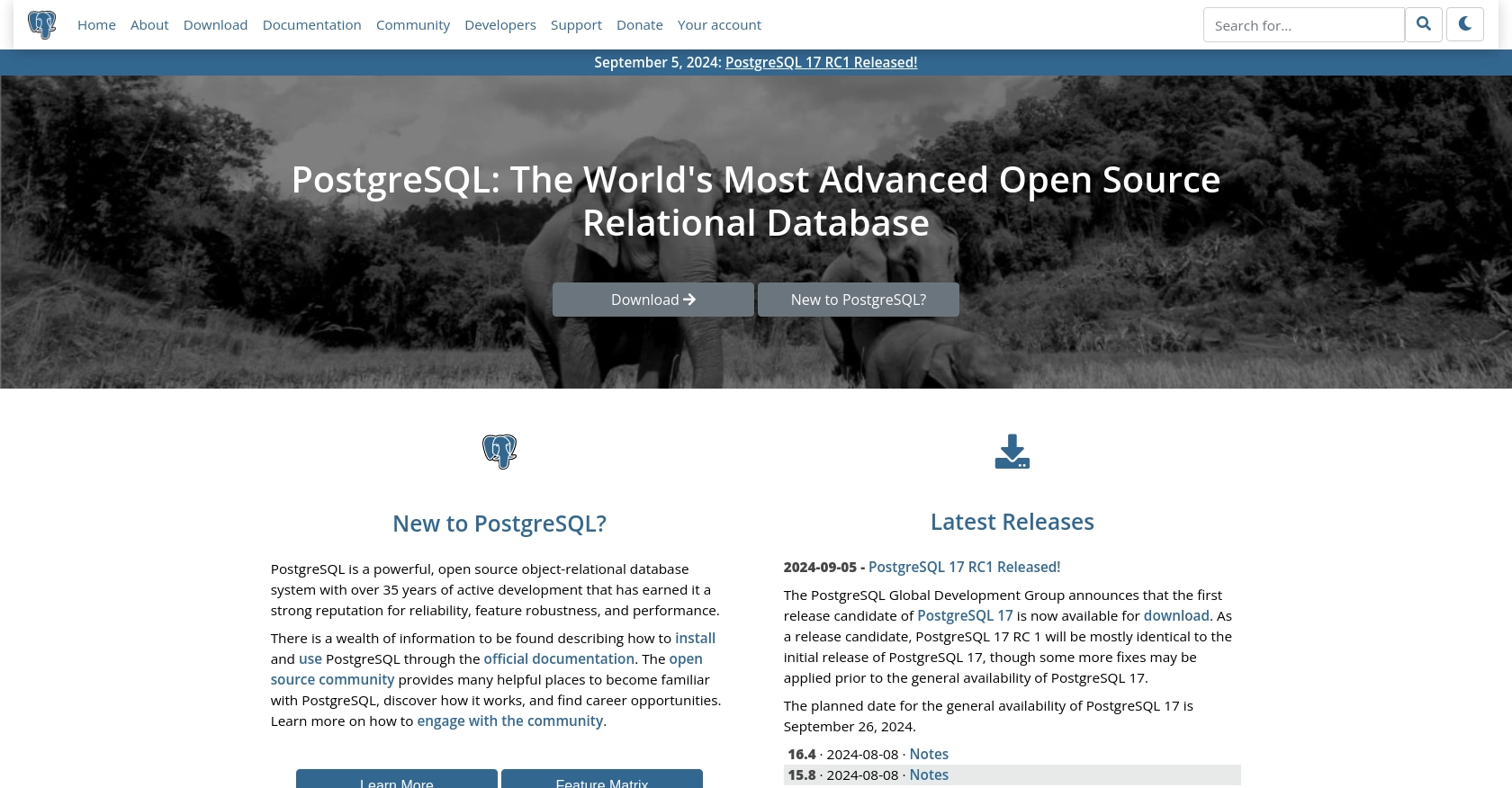
Introduction to PostgreSQL API Integration
PostgreSQL, often referred to as Postgres, is a powerful, open-source relational database management system known for its robustness, scalability, and compliance with SQL standards. It is widely used by developers and organizations to manage and store data efficiently.
Integrating with the PostgreSQL API allows developers to perform various database operations programmatically, such as creating or updating records. This can be particularly useful in scenarios where you need to automate data management tasks or integrate PostgreSQL with other applications. For example, a developer might want to update customer records in a PostgreSQL database based on real-time data from a web application, ensuring that the database remains current and accurate.
Setting Up a Test Environment for PostgreSQL API Integration
Before you can start creating or updating records with the PostgreSQL API in JavaScript, it's essential to set up a test environment. This allows you to safely experiment with API calls without affecting your production data. PostgreSQL provides several options for setting up a test or sandbox environment.
Installing PostgreSQL Locally
To begin, you can install PostgreSQL on your local machine. This setup is ideal for development and testing purposes. Follow these steps to install PostgreSQL:
- Visit the PostgreSQL download page and choose the appropriate version for your operating system.
- Follow the installation instructions provided for your platform.
- Once installed, use the PostgreSQL command-line tool,
psql
, to create a new database for testing purposes.
Creating a Test Database
After installing PostgreSQL, you need to create a test database. This database will be used to perform API operations:
CREATE DATABASE test_db;
Connect to the newly created database using psql
:
psql -U your_username -d test_db
Setting Up Authentication
PostgreSQL uses a robust authentication system to secure access to databases. For testing purposes, you can configure simple password authentication:
- Edit the
pg_hba.conf
file, typically located in the PostgreSQL data directory. - Add an entry to allow password authentication for your test database:
# TYPE DATABASE USER ADDRESS METHOD
host test_db all 127.0.0.1/32 md5
Restart the PostgreSQL server to apply the changes:
sudo service postgresql restart
Generating API Credentials
For API access, you'll need to generate credentials. PostgreSQL doesn't have a built-in API key system, so you'll typically use database roles and passwords:
- Create a new role with login privileges:
CREATE ROLE api_user WITH LOGIN PASSWORD 'your_password';
- Grant the necessary permissions to this role for your test database:
GRANT ALL PRIVILEGES ON DATABASE test_db TO api_user;
With your test environment set up, you are now ready to start integrating with the PostgreSQL API using JavaScript. This setup ensures that you can develop and test your API interactions without impacting your production data.
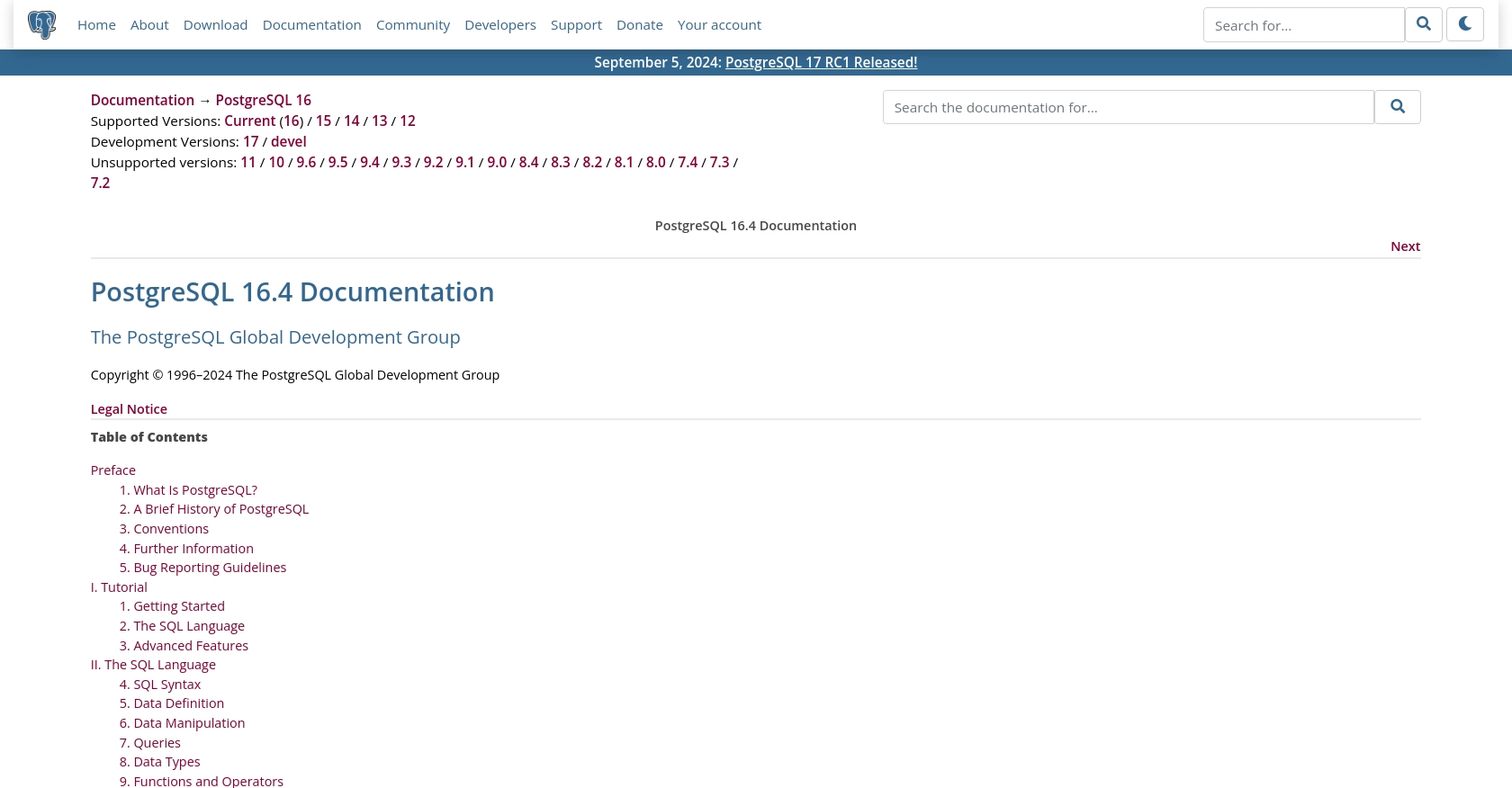
sbb-itb-96038d7
Making API Calls to Create or Update Records in PostgreSQL Using JavaScript
To interact with PostgreSQL from a JavaScript environment, you can use libraries such as pg
, which is a popular client for PostgreSQL in Node.js. This section will guide you through the process of setting up your environment and making API calls to create or update records in your PostgreSQL database.
Setting Up Your JavaScript Environment for PostgreSQL Integration
Before making API calls, ensure you have Node.js installed on your machine. You can download it from the official Node.js website. Once Node.js is installed, you can set up your project and install the necessary dependencies.
- Create a new directory for your project and navigate into it:
mkdir postgres-api-integration
cd postgres-api-integration
- Initialize a new Node.js project:
npm init -y
- Install the
pg
library:
npm install pg
Creating a Connection to PostgreSQL
With the pg
library installed, you can now create a connection to your PostgreSQL database. Use the following code to establish a connection:
const { Client } = require('pg');
const client = new Client({
user: 'api_user',
host: 'localhost',
database: 'test_db',
password: 'your_password',
port: 5432,
});
client.connect()
.then(() => console.log('Connected to PostgreSQL'))
.catch(err => console.error('Connection error', err.stack));
Replace api_user
, your_password
, and other connection details with your actual database credentials.
Creating Records in PostgreSQL Using JavaScript
To create a new record in your PostgreSQL database, you can use the following code snippet. This example demonstrates how to insert a new customer record:
const createRecord = async () => {
const query = `
INSERT INTO customers (name, email)
VALUES ($1, $2)
RETURNING *;
`;
const values = ['John Doe', 'john.doe@example.com'];
try {
const res = await client.query(query, values);
console.log('Record created:', res.rows[0]);
} catch (err) {
console.error('Error creating record', err.stack);
}
};
createRecord();
This code defines a function createRecord
that inserts a new customer into the customers
table. The RETURNING
clause allows you to retrieve the newly created record.
Updating Records in PostgreSQL Using JavaScript
To update an existing record, you can use a similar approach. Here's an example of updating a customer's email address:
const updateRecord = async () => {
const query = `
UPDATE customers
SET email = $1
WHERE name = $2
RETURNING *;
`;
const values = ['new.email@example.com', 'John Doe'];
try {
const res = await client.query(query, values);
console.log('Record updated:', res.rows[0]);
} catch (err) {
console.error('Error updating record', err.stack);
}
};
updateRecord();
This function updateRecord
updates the email of a customer identified by their name. The RETURNING
clause is used to fetch the updated record.
Handling Errors and Verifying API Calls
When making API calls, it's crucial to handle potential errors gracefully. The catch
block in the above examples logs any errors encountered during the database operations. Additionally, you can verify the success of your API calls by checking the returned data or querying the database directly to ensure the changes were applied.
By following these steps, you can effectively create and update records in a PostgreSQL database using JavaScript, enabling seamless integration and data management in your applications.
Best Practices for PostgreSQL API Integration in JavaScript
Successfully integrating with the PostgreSQL API using JavaScript requires adherence to best practices to ensure security, efficiency, and maintainability. Here are some key recommendations:
- Securely Store Credentials: Always store your database credentials securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Although PostgreSQL itself doesn't impose rate limits, be mindful of the server's capacity and implement throttling mechanisms in your application to prevent overwhelming the database with requests.
- Implement Error Handling: Ensure robust error handling in your API calls. Use try-catch blocks to manage exceptions and log errors for troubleshooting.
- Optimize Queries: Write efficient SQL queries to minimize load on the database. Use indexing and query optimization techniques to enhance performance.
- Data Transformation and Standardization: Standardize data formats and types before inserting or updating records to maintain consistency across your database.
Enhance Your Integration Strategy with Endgrate
Integrating with PostgreSQL is just one aspect of a broader integration strategy. If your team is managing multiple integrations, consider leveraging Endgrate to streamline the process. Endgrate offers a unified API endpoint that simplifies connecting to various platforms, including PostgreSQL.
By using Endgrate, you can:
- Save time and resources by outsourcing complex integrations and focusing on your core product development.
- Build once for each use case instead of multiple times for different integrations, reducing redundancy.
- Provide an intuitive integration experience for your customers, enhancing user satisfaction.
Explore how Endgrate can transform your integration approach by visiting Endgrate today.
Read More
Ready to get started?