How to Create Task with the Clickup API in Javascript
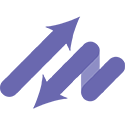
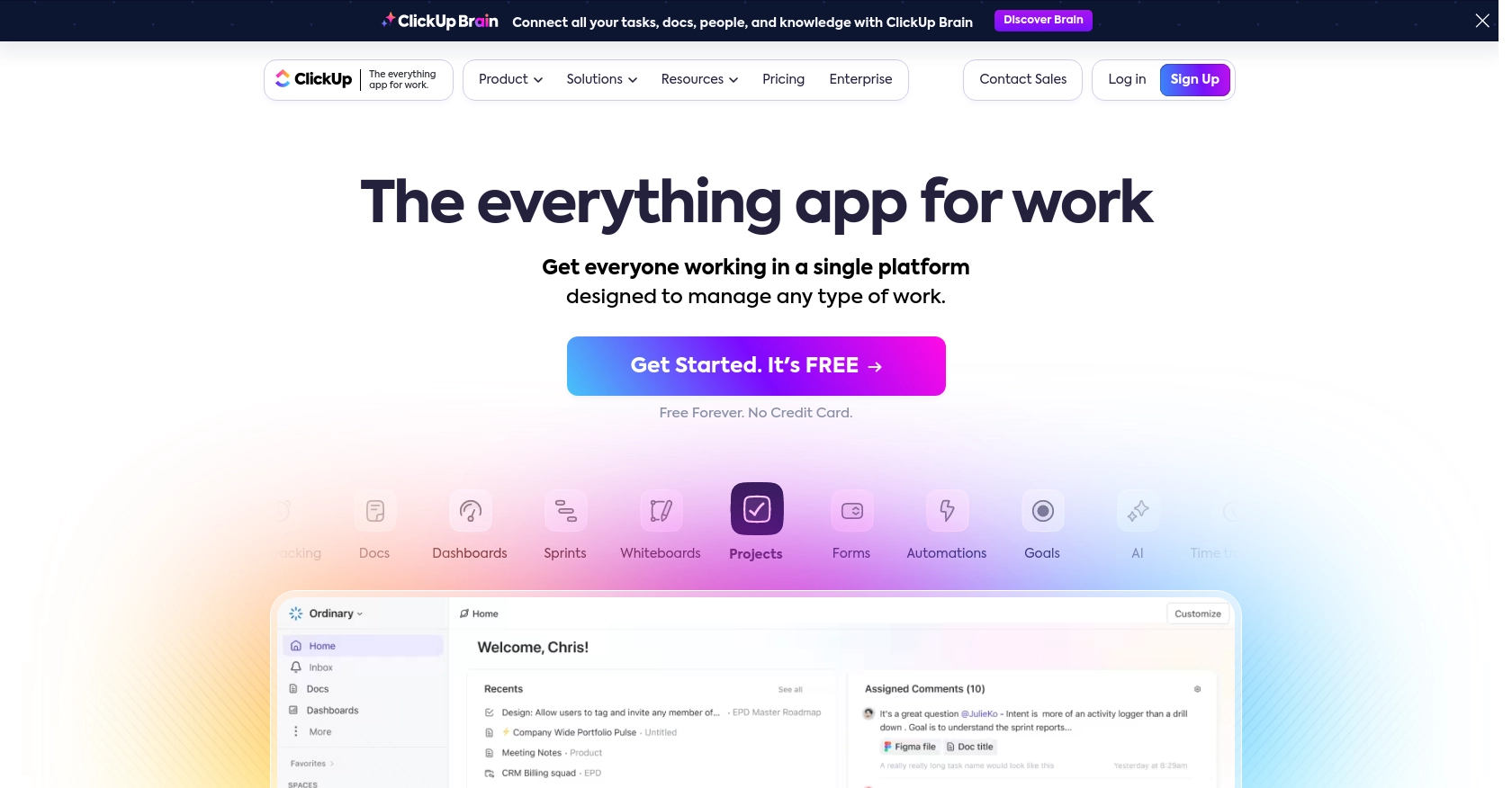
Introduction to ClickUp API Integration
ClickUp is a versatile project management tool that offers a wide range of features to help teams organize tasks, manage workflows, and enhance productivity. With its customizable interface and robust functionality, ClickUp is a popular choice for businesses looking to streamline their project management processes.
Integrating with ClickUp's API allows developers to automate and enhance task management within their applications. For example, by using the ClickUp API, a developer can create tasks programmatically, enabling seamless integration with other tools and systems. This can be particularly useful for automating task creation based on specific triggers or events within a business workflow.
Setting Up Your ClickUp Test or Sandbox Account
Before you can start creating tasks with the ClickUp API, you need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting your live data.
Creating a ClickUp Account
If you don't already have a ClickUp account, you can easily sign up for a free account on the ClickUp website. Follow the instructions to create your account and log in.
Generating a Personal API Token in ClickUp
To interact with the ClickUp API, you'll need to authenticate your requests using a personal API token. Here's how you can generate one:
- Log into your ClickUp account.
- In ClickUp 3.0, click your avatar in the upper-right corner and select Settings.
- Scroll down to the Apps section in the sidebar and click on it.
- Under API Token, click Generate.
- Copy and save your personal API token securely, as you'll need it for API requests.
For more details, you can refer to the ClickUp Authentication Documentation.
Setting Up OAuth for ClickUp API
If you're developing an application for others to use, you'll need to implement OAuth authentication. Follow these steps to set up OAuth:
- Log into ClickUp and click on your avatar in the lower-left corner.
- Select Integrations and then click on ClickUp API.
- Click Create an App and provide a name and redirect URL for your app.
- Once created, you'll receive a
client_id
andsecret
. Keep these credentials secure.
For more information on OAuth setup, visit the ClickUp OAuth Documentation.
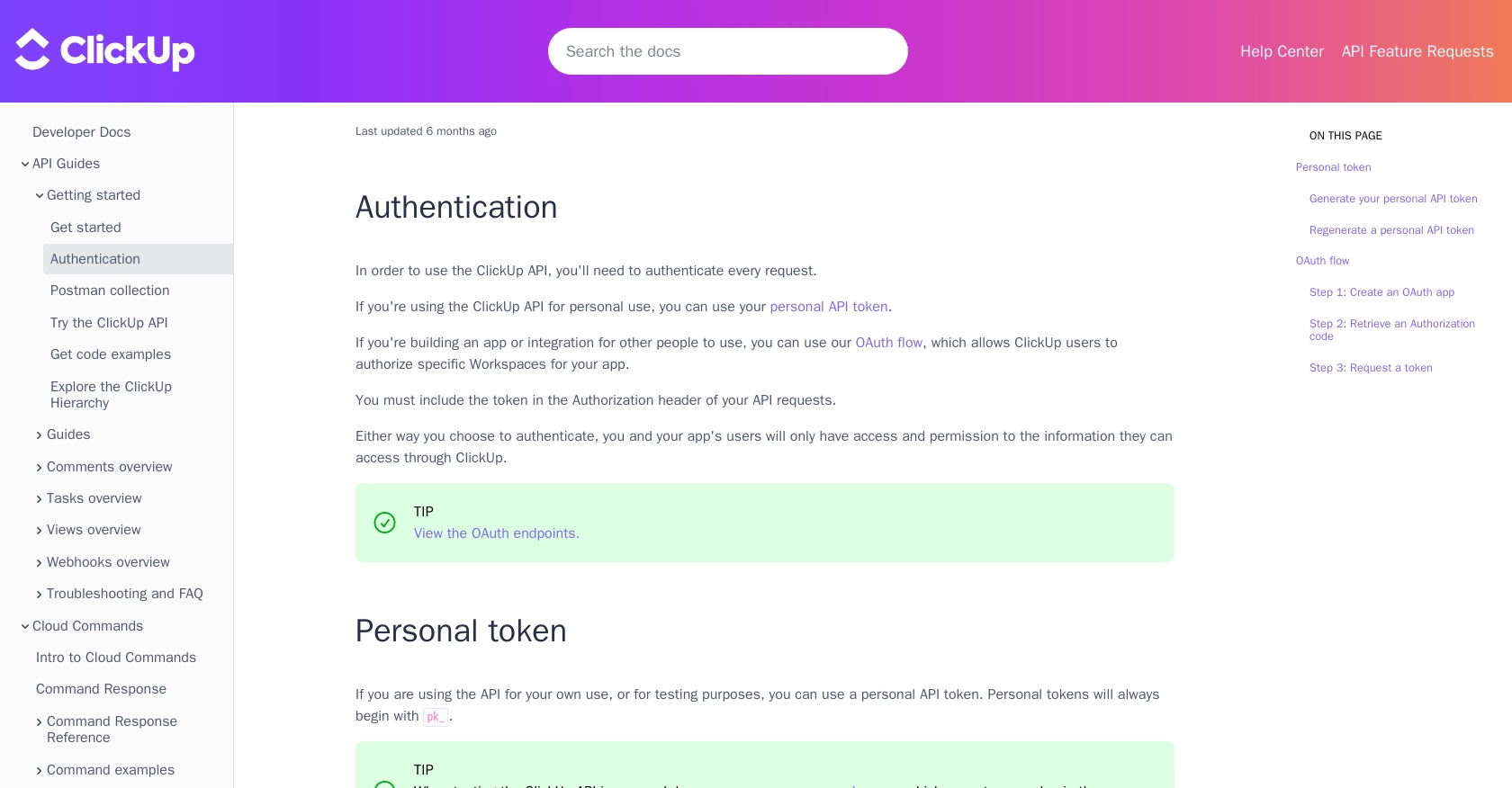
sbb-itb-96038d7
How to Make an API Call to Create a Task with ClickUp in JavaScript
To create a task using the ClickUp API in JavaScript, you'll need to set up your environment and write the necessary code to interact with the API. This section will guide you through the process, including setting up your JavaScript environment, writing the code, and handling responses and errors.
Setting Up Your JavaScript Environment for ClickUp API Integration
Before making API calls, ensure you have Node.js installed on your machine. You can download it from the official Node.js website. Once installed, you can use npm (Node Package Manager) to manage your dependencies.
To make HTTP requests, you'll need the axios
library. Install it using the following command:
npm install axios
Writing JavaScript Code to Create a Task with ClickUp API
Now that your environment is set up, you can write the code to create a task in ClickUp. Here's a step-by-step guide:
const axios = require('axios');
// Replace with your personal API token
const apiToken = 'pk_your_personal_token';
// Define the API endpoint and request headers
const endpoint = 'https://api.clickup.com/api/v2/list/{list_id}/task';
const headers = {
'Authorization': apiToken,
'Content-Type': 'application/json'
};
// Define the task data
const taskData = {
name: 'New Task Name',
description: 'New Task Description',
assignees: [183], // Replace with actual assignee IDs
status: 'Open',
priority: 3
};
// Make the POST request to create a task
axios.post(endpoint, taskData, { headers })
.then(response => {
console.log('Task Created Successfully:', response.data);
})
.catch(error => {
console.error('Error Creating Task:', error.response ? error.response.data : error.message);
});
In this code, replace {list_id}
with the actual list ID where you want to create the task. The apiToken
should be your personal API token generated from ClickUp. Customize the taskData
object with the desired task details.
Verifying Task Creation and Handling Errors
After running the code, you should see a success message with the task details if the request is successful. To verify, log into your ClickUp account and check the specified list for the newly created task.
If there's an error, the code will log the error message. Common errors include invalid tokens, incorrect list IDs, or missing required fields. Refer to the ClickUp API documentation for more details on error codes and troubleshooting.
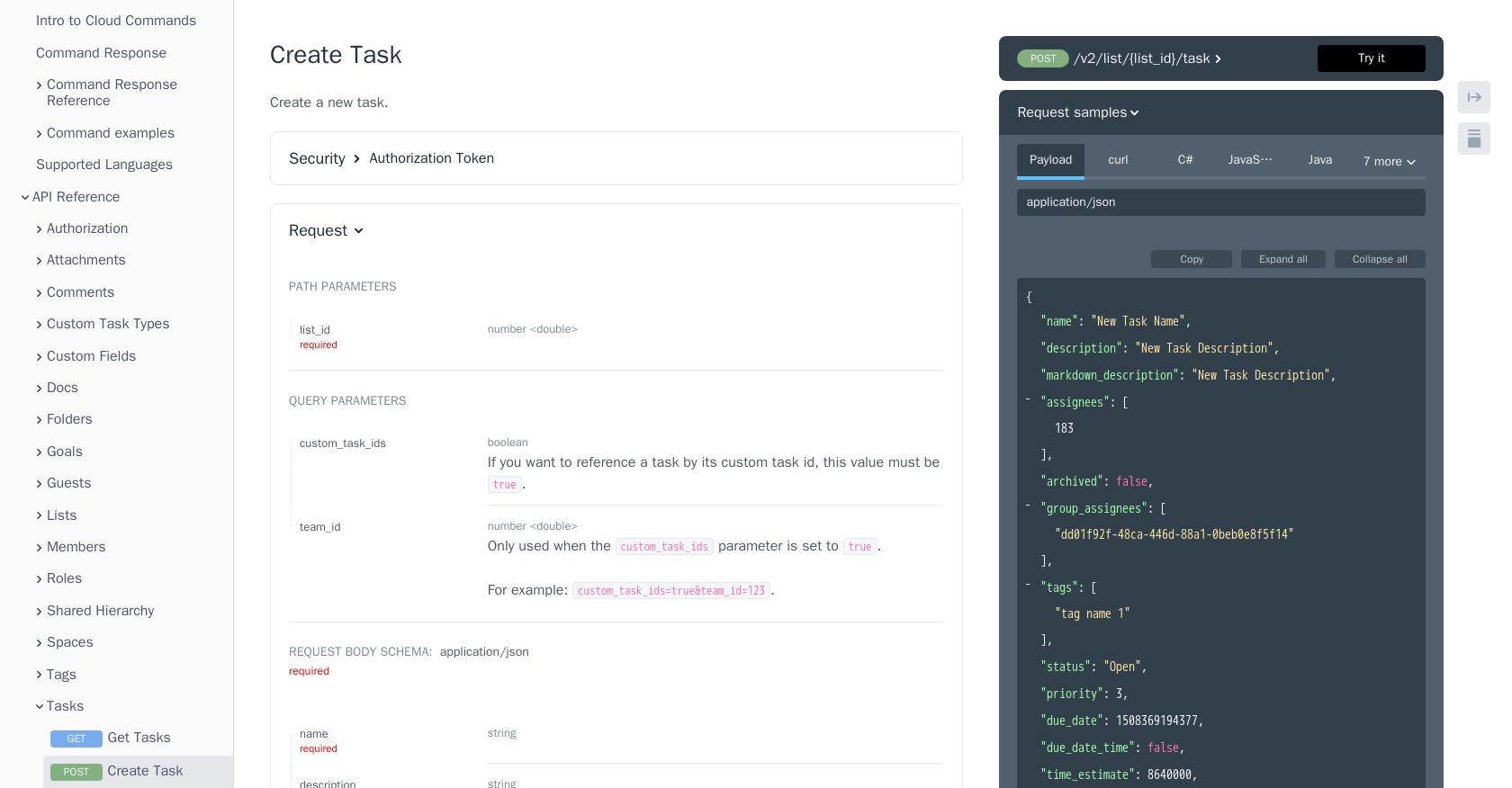
Conclusion and Best Practices for ClickUp API Integration in JavaScript
Integrating with the ClickUp API using JavaScript can significantly enhance your application's task management capabilities. By automating task creation, you can streamline workflows and improve productivity across your projects. However, it's crucial to follow best practices to ensure a secure and efficient integration.
Best Practices for Secure and Efficient ClickUp API Integration
- Secure Storage of API Tokens: Always store your API tokens securely. Avoid hardcoding them in your source code. Instead, use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be mindful of ClickUp's API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully. Check the ClickUp API documentation for specific rate limit details.
- Data Validation: Validate and sanitize all input data before making API requests. This helps prevent errors and ensures that the data sent to ClickUp is accurate and complete.
- Error Handling: Implement comprehensive error handling to manage API response errors effectively. Log errors for debugging and provide meaningful feedback to users when issues arise.
- Optimize API Calls: Minimize the number of API calls by batching requests or using webhooks to receive updates. This reduces latency and improves the overall performance of your integration.
Enhance Your Integration Experience with Endgrate
While integrating with ClickUp's API can be powerful, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process across various platforms, including ClickUp.
By leveraging Endgrate, you can:
- Save time and resources by outsourcing integration management.
- Build once for each use case and apply it across multiple integrations.
- Provide an intuitive integration experience for your users.
Explore how Endgrate can streamline your integration efforts and allow you to focus on your core product by visiting Endgrate's website.
Read More
Ready to get started?