Using the Google Forms API to Get Responses in Python
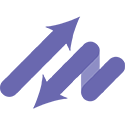
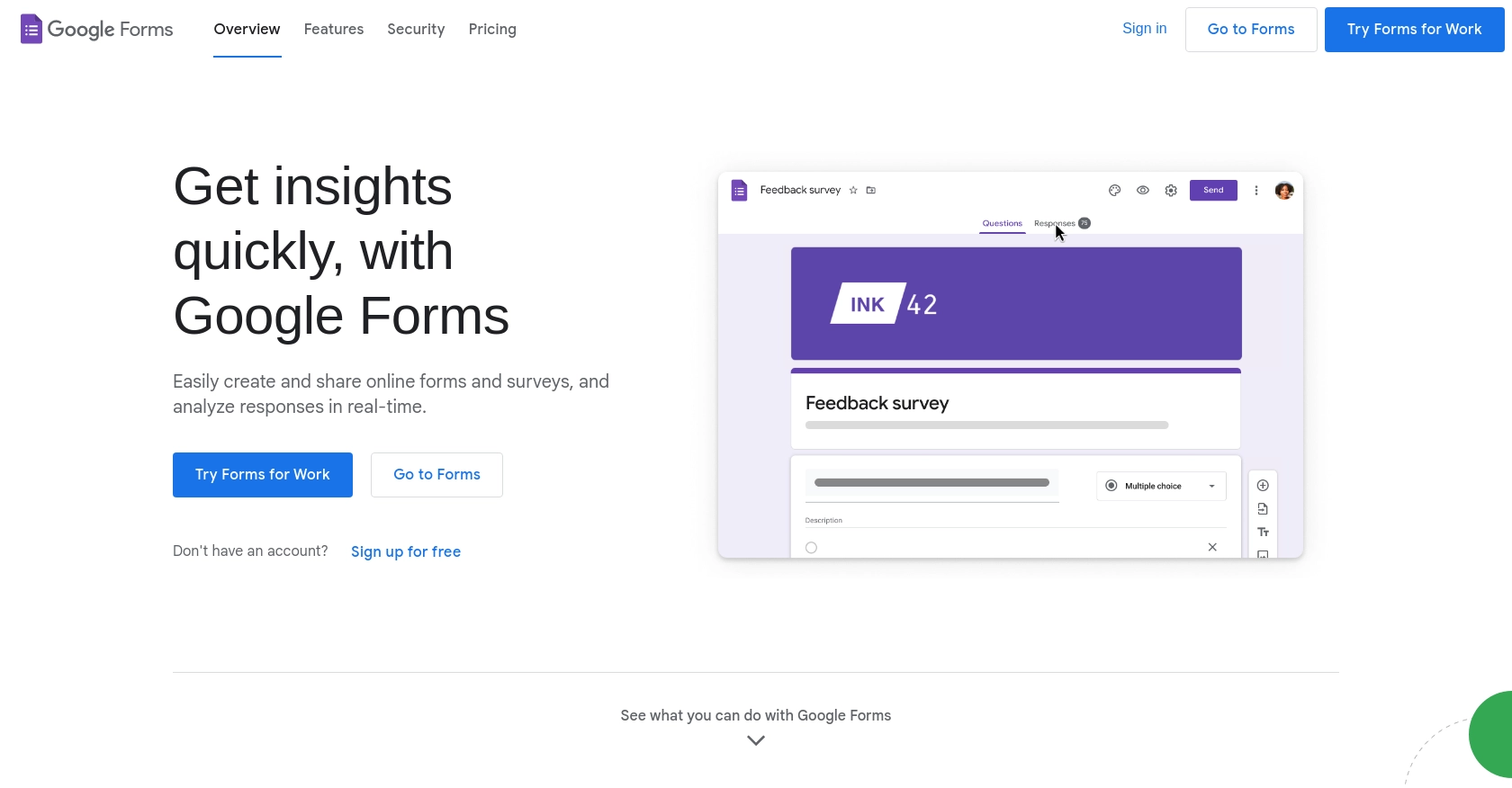
Introduction to Google Forms API
Google Forms is a versatile tool within the Google Workspace suite, allowing users to create surveys, quizzes, and forms with ease. Its integration capabilities make it a powerful choice for businesses and developers looking to streamline data collection and analysis.
By integrating with the Google Forms API, developers can automate the retrieval of form responses, enabling seamless data processing and analysis. For example, a developer might use the API to automatically fetch survey responses and analyze them in real-time, providing valuable insights for decision-making.
Setting Up Your Google Forms API Test Account
Before you can start interacting with the Google Forms API, you need to set up a Google Cloud project and configure OAuth 2.0 authentication. This setup will allow you to securely access and manage Google Forms data.
Create a Google Cloud Project for Google Forms API
To begin, you need a Google Cloud project. This project will serve as the foundation for enabling the Google Forms API and managing credentials.
- Go to the Google Cloud Console.
- Click on the Menu icon, then navigate to IAM & Admin > Create a Project.
- Enter a descriptive name for your project and click Create.
Enable Google Forms API in Your Project
Once your project is created, you need to enable the Google Forms API.
- In the Google Cloud Console, navigate to APIs & Services > Library.
- Search for Google Forms API and click on it.
- Click Enable to activate the API for your project.
Configure OAuth Consent Screen for Google Forms API
Next, configure the OAuth consent screen, which is required for user authorization.
- In the Google Cloud Console, go to APIs & Services > OAuth consent screen.
- Select the user type for your app and click Create.
- Fill out the required fields, such as app name and support email, then click Save and Continue.
Create OAuth Credentials for Google Forms API
Now, create OAuth credentials to authenticate your application.
- In the Google Cloud Console, navigate to APIs & Services > Credentials.
- Click Create Credentials and select OAuth client ID.
- Choose the application type that suits your needs (e.g., Web application) and configure the authorized redirect URIs.
- Click Create to generate your Client ID and Client Secret.
Ensure you securely store your Client ID and Client Secret, as these will be used to authenticate requests to the Google Forms API.
For more detailed guidance, refer to the official documentation: Create a Google Cloud Project, Enable Google Workspace APIs, Configure OAuth Consent, and Create Credentials.
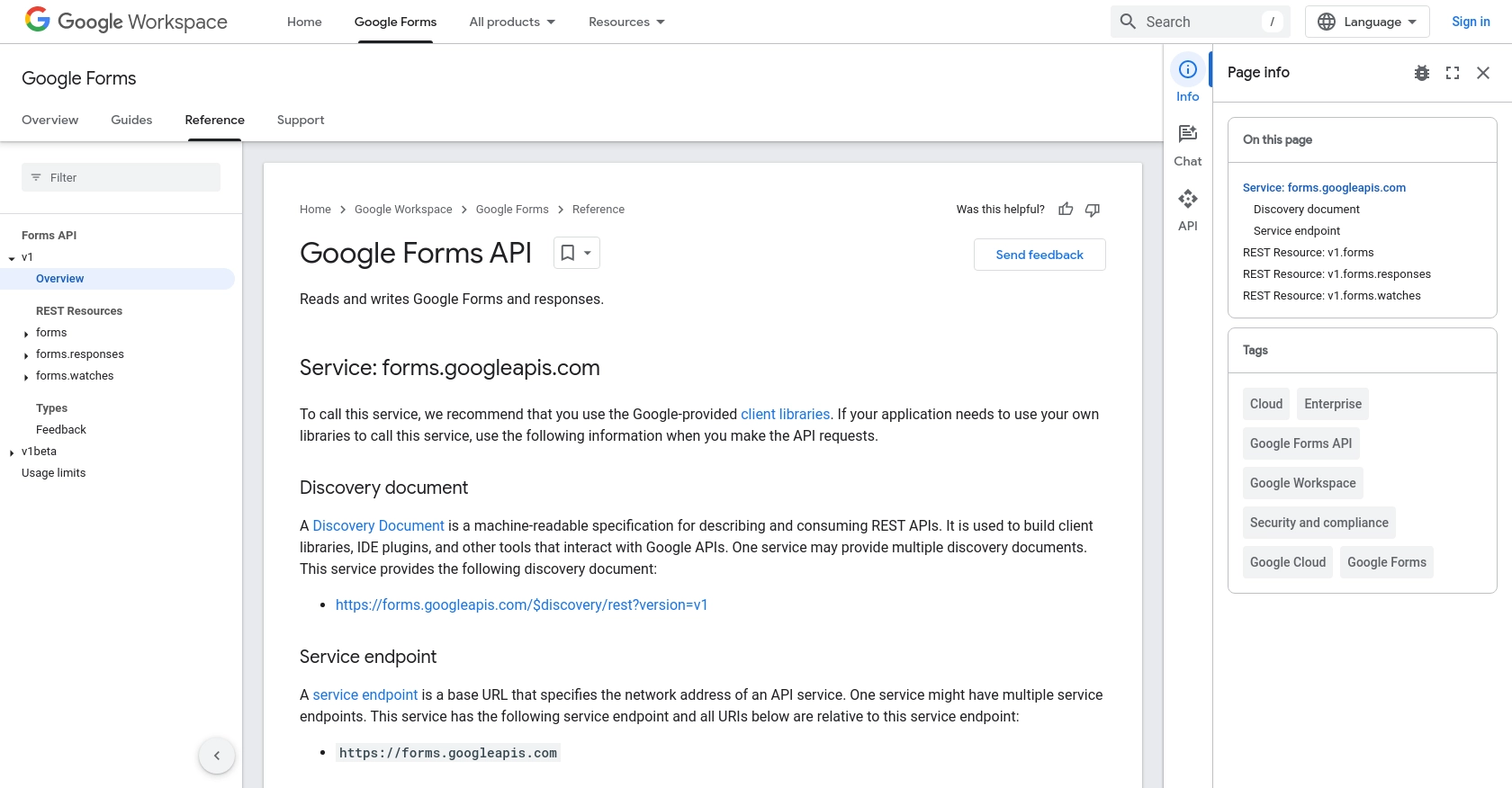
sbb-itb-96038d7
Making API Calls to Retrieve Google Forms Responses Using Python
To interact with the Google Forms API and retrieve form responses, you'll need to use Python. This section will guide you through setting up your environment, writing the necessary code, and handling potential errors.
Setting Up Your Python Environment for Google Forms API
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need to install the google-auth
and google-auth-oauthlib
libraries to handle OAuth 2.0 authentication.
- Install Python 3.11.1 from the official Python website if you haven't already.
- Open your terminal or command prompt and install the required libraries using pip:
pip install google-auth google-auth-oauthlib requests
Writing the Python Code to Access Google Forms Responses
With your environment set up, you can now write the Python script to fetch responses from a Google Form. The following code demonstrates how to authenticate and make a request to the Google Forms API.
import os
import requests
from google.oauth2.credentials import Credentials
from google_auth_oauthlib.flow import InstalledAppFlow
# Define the scopes and form ID
SCOPES = ['https://www.googleapis.com/auth/forms.responses.readonly']
FORM_ID = 'your_form_id_here'
def main():
# Authenticate and obtain credentials
creds = None
if os.path.exists('token.json'):
creds = Credentials.from_authorized_user_file('token.json', SCOPES)
if not creds or not creds.valid:
if creds and creds.expired and creds.refresh_token:
creds.refresh(Request())
else:
flow = InstalledAppFlow.from_client_secrets_file(
'credentials.json', SCOPES)
creds = flow.run_local_server(port=0)
with open('token.json', 'w') as token:
token.write(creds.to_json())
# Make a request to the Google Forms API
service = build('forms', 'v1', credentials=creds)
result = service.forms().responses().list(formId=FORM_ID).execute()
# Print the form responses
for response in result.get('responses', []):
print(response)
if __name__ == '__main__':
main()
Replace your_form_id_here
with the actual ID of your Google Form. The script first checks for existing credentials and refreshes them if necessary. It then makes a request to the Google Forms API to list the responses.
Verifying Successful API Calls and Handling Errors
After running the script, you should see the form responses printed in your terminal. To verify the success of your API call, check the output against the responses in your Google Forms dashboard.
If you encounter errors, refer to the following common error codes:
- 401 Unauthorized: Check your OAuth credentials and ensure they are correctly configured.
- 403 Forbidden: Verify that your API scopes are correctly set and that you have permission to access the form.
- 404 Not Found: Ensure the form ID is correct and that the form exists.
For more detailed error handling, consult the official Google Forms API documentation.
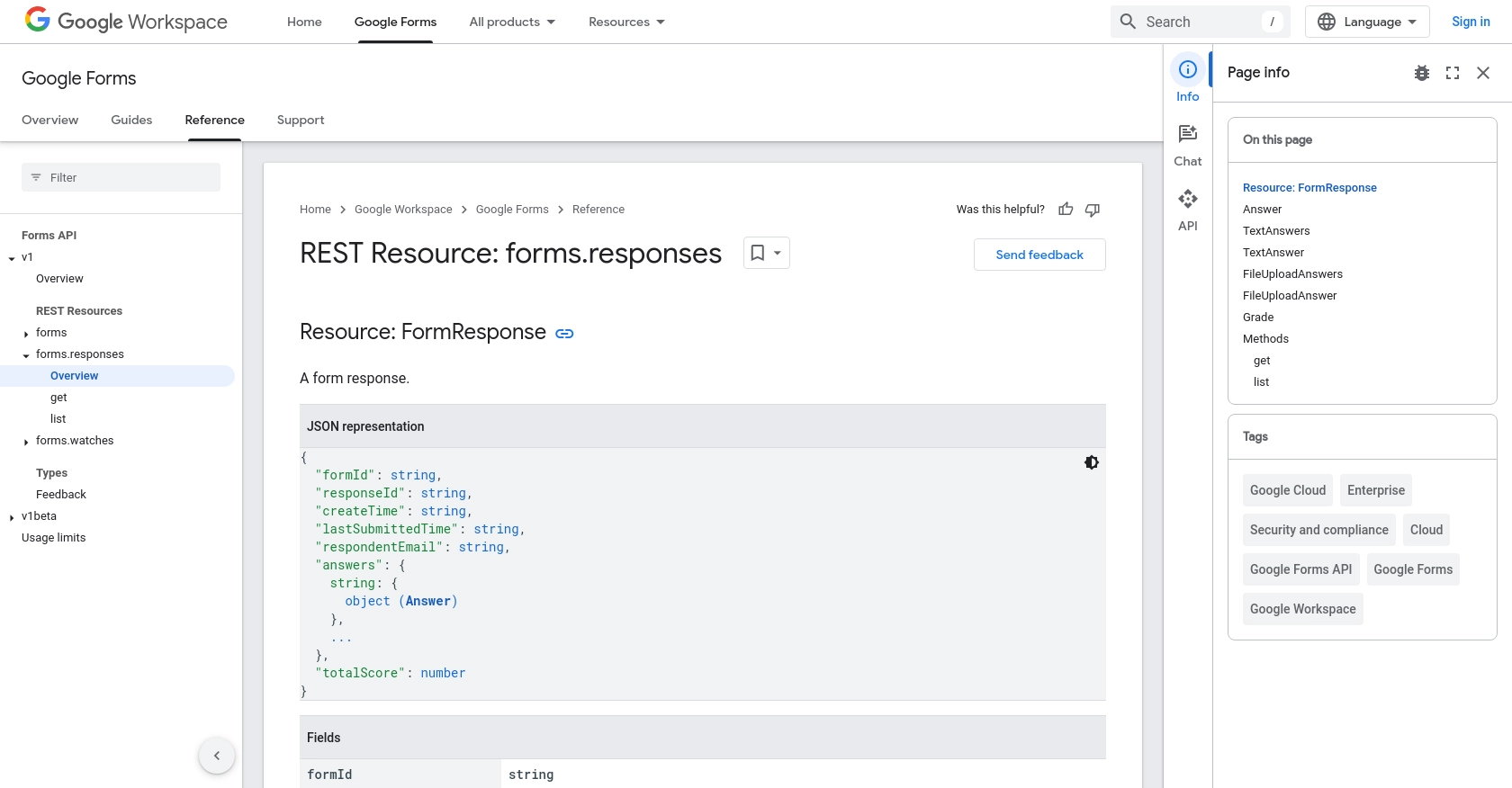
Conclusion and Best Practices for Using Google Forms API with Python
Integrating with the Google Forms API using Python allows developers to automate data retrieval and streamline data processing workflows. By following the steps outlined in this guide, you can efficiently access form responses and incorporate them into your applications for real-time analysis and decision-making.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your OAuth credentials securely. Consider using environment variables or secure vaults to protect sensitive information like Client IDs and Secrets.
- Handle Rate Limiting: Be mindful of the Google Forms API rate limits to avoid throttling. Implement exponential backoff strategies for retrying requests when encountering rate limit errors.
- Data Standardization: Ensure that the data retrieved from Google Forms is standardized and transformed as needed to fit your application's data model. This will facilitate seamless integration and data analysis.
Leverage Endgrate for Simplified Integration Management
While integrating with Google Forms API can be straightforward, managing multiple integrations across different platforms can become complex. Endgrate offers a unified API solution that simplifies integration management, allowing you to focus on your core product development.
With Endgrate, you can build once for each use case and leverage a single API endpoint to connect with multiple platforms, including Google Forms. This approach saves time and resources, providing an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate and discover the benefits of outsourcing integrations to streamline your development process.
Read More
- https://endgrate.com/provider/googleforms
- https://developers.google.com/forms/api/reference/rest
- https://developers.google.com/workspace/guides/create-project
- https://developers.google.com/workspace/guides/enable-apis
- https://developers.google.com/workspace/guides/configure-oauth-consent
- https://developers.google.com/workspace/guides/create-credentials
- https://developers.google.com/forms/api/reference/rest/v1/forms.responses
Ready to get started?