How to Get Accounts with the Quickbooks API in PHP
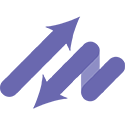
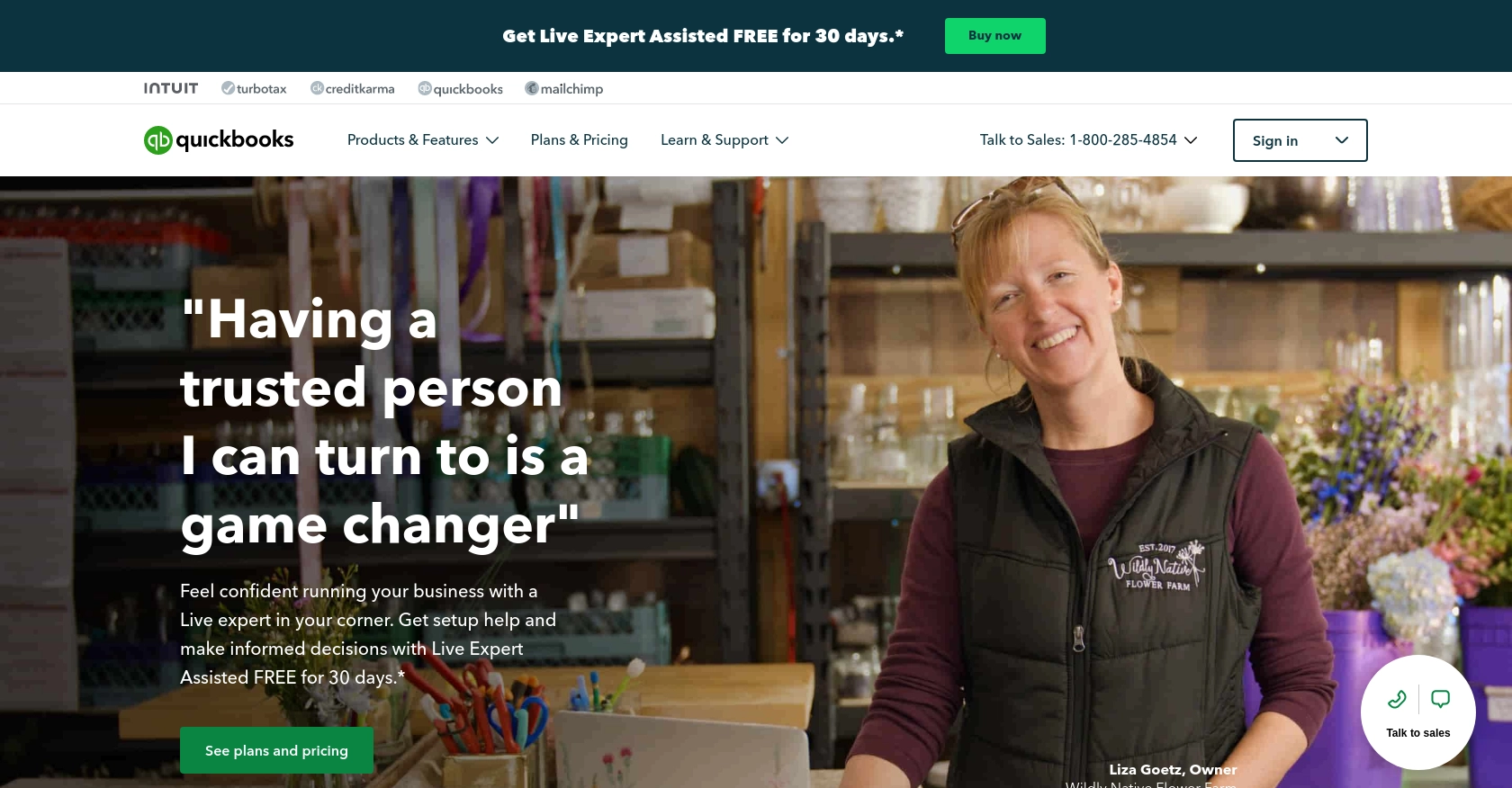
Introduction to QuickBooks API Integration
QuickBooks is a widely-used accounting software that helps businesses manage their financial operations with ease. It offers a comprehensive suite of tools for invoicing, expense tracking, payroll, and financial reporting, making it a popular choice for small to medium-sized enterprises.
Integrating with the QuickBooks API allows developers to automate and streamline accounting processes. For example, you can use the QuickBooks API to retrieve account information, enabling seamless synchronization of financial data between QuickBooks and other business applications. This integration can enhance efficiency by reducing manual data entry and ensuring data consistency across platforms.
Setting Up Your QuickBooks Sandbox Account for API Integration
Before you can start interacting with the QuickBooks API, you need to set up a sandbox account. This environment allows you to test your integration without affecting real data. Follow these steps to create your QuickBooks sandbox account and obtain the necessary credentials for OAuth authentication.
Step 1: Sign Up for a QuickBooks Developer Account
To begin, you need a QuickBooks Developer account. If you don't have one, visit the QuickBooks Developer Portal and sign up. This account will give you access to the sandbox environment and the tools needed for integration.
Step 2: Create a New App in QuickBooks
Once you have your developer account, log in and navigate to the "My Apps" section. Click on "Create an App" to start setting up your application. Choose "QuickBooks Online and Payments" as the platform for your app.
Step 3: Configure App Settings for OAuth Authentication
After creating your app, you'll need to configure its settings to enable OAuth authentication. Go to the "Keys & OAuth" section of your app settings. Here, you'll find your Client ID and Client Secret, which are essential for authenticating API requests.
For detailed instructions on obtaining these credentials, refer to the QuickBooks documentation.
Step 4: Set Up Redirect URIs
In the same "Keys & OAuth" section, you'll need to specify the redirect URIs for your application. These URIs are where users will be redirected after they authorize your app. Make sure to include the correct URIs that match your application's configuration.
Step 5: Access the QuickBooks Sandbox Environment
With your app configured, you can now access the QuickBooks sandbox environment. This environment simulates the live QuickBooks Online environment, allowing you to test API calls without affecting real data. You can find more details on setting up and using the sandbox in the QuickBooks documentation.
Once your sandbox account is set up and your app is configured, you're ready to start making API calls to retrieve account information from QuickBooks using PHP.
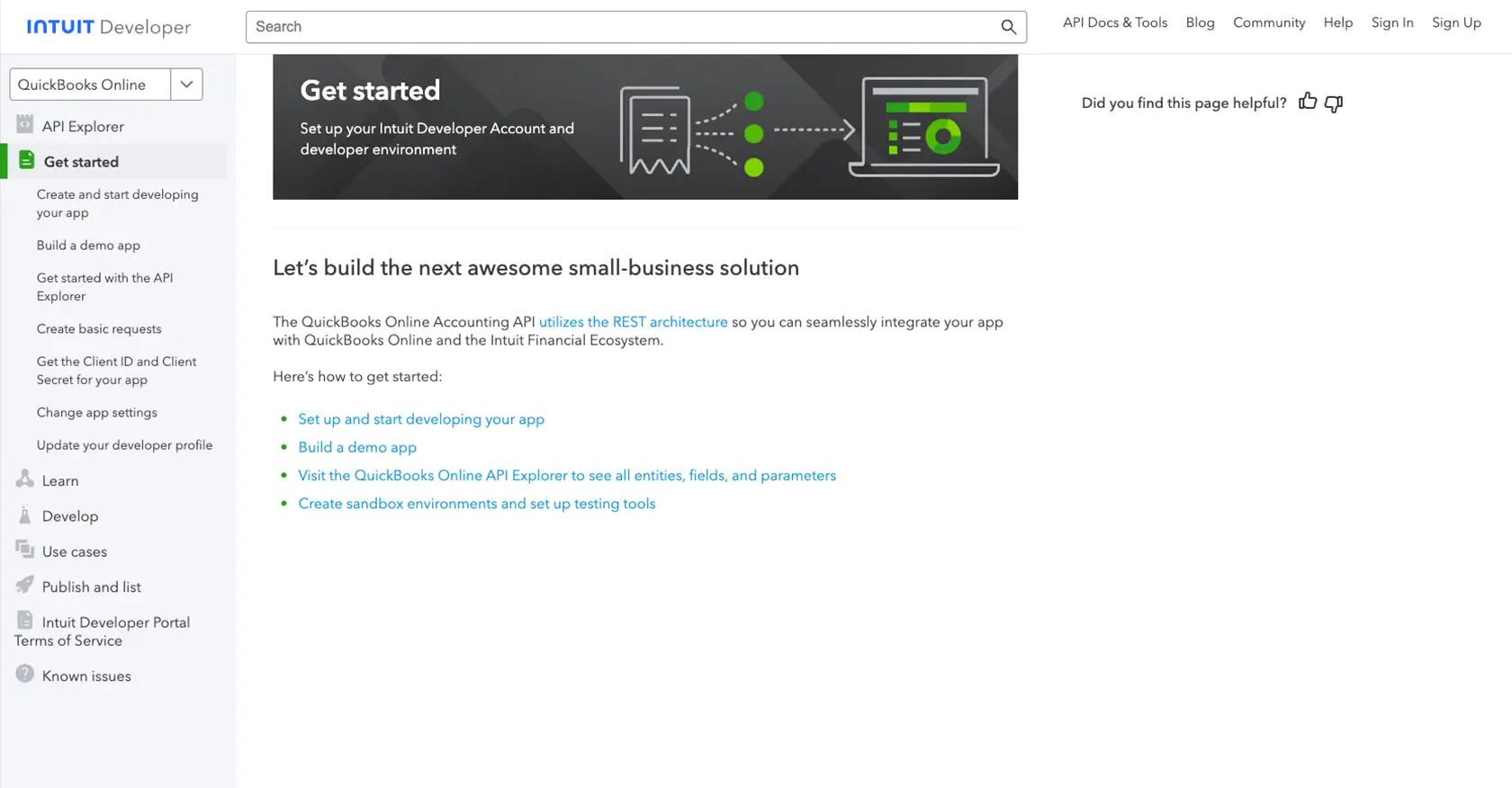
sbb-itb-96038d7
Making API Calls to Retrieve Accounts from QuickBooks Using PHP
To interact with the QuickBooks API and retrieve account information, you need to use PHP to make HTTP requests. This section will guide you through the process of setting up your PHP environment, installing necessary dependencies, and executing the API call to get account data from QuickBooks.
Setting Up Your PHP Environment for QuickBooks API Integration
Before making API calls, ensure that your PHP environment is properly configured. You will need PHP version 7.4 or higher and the Composer package manager to handle dependencies.
Installing Required PHP Packages for QuickBooks API
To interact with the QuickBooks API, you'll need the guzzlehttp/guzzle
package, which simplifies HTTP requests. Install it using Composer with the following command:
composer require guzzlehttp/guzzle
Writing PHP Code to Retrieve Accounts from QuickBooks
With your environment set up, you can now write the PHP code to make the API call. Create a file named get_quickbooks_accounts.php
and add the following code:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'Your_Access_Token'; // Replace with your OAuth access token
try {
$response = $client->request('GET', 'https://sandbox-quickbooks.api.intuit.com/v3/company/YOUR_COMPANY_ID/account', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Accept' => 'application/json',
]
]);
$data = json_decode($response->getBody(), true);
foreach ($data['Account'] as $account) {
echo 'Account Name: ' . $account['Name'] . '<br>';
echo 'Account Type: ' . $account['AccountType'] . '<br><br>';
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
?>
Replace Your_Access_Token
with the OAuth token obtained during the setup process and YOUR_COMPANY_ID
with your QuickBooks company ID.
Running the PHP Script and Verifying API Response
Execute the PHP script from the command line or a web server. You should see a list of accounts retrieved from your QuickBooks sandbox environment. If the request is successful, the account names and types will be displayed.
To verify the request, log in to your QuickBooks sandbox account and check the accounts section to ensure the data matches the API response.
Handling Errors and QuickBooks API Error Codes
When making API calls, it's crucial to handle potential errors. The QuickBooks API may return various error codes, such as 401 for unauthorized access or 404 if the resource is not found. Ensure your code gracefully handles these errors by catching exceptions and displaying appropriate messages.
For more information on error codes, refer to the QuickBooks API documentation.
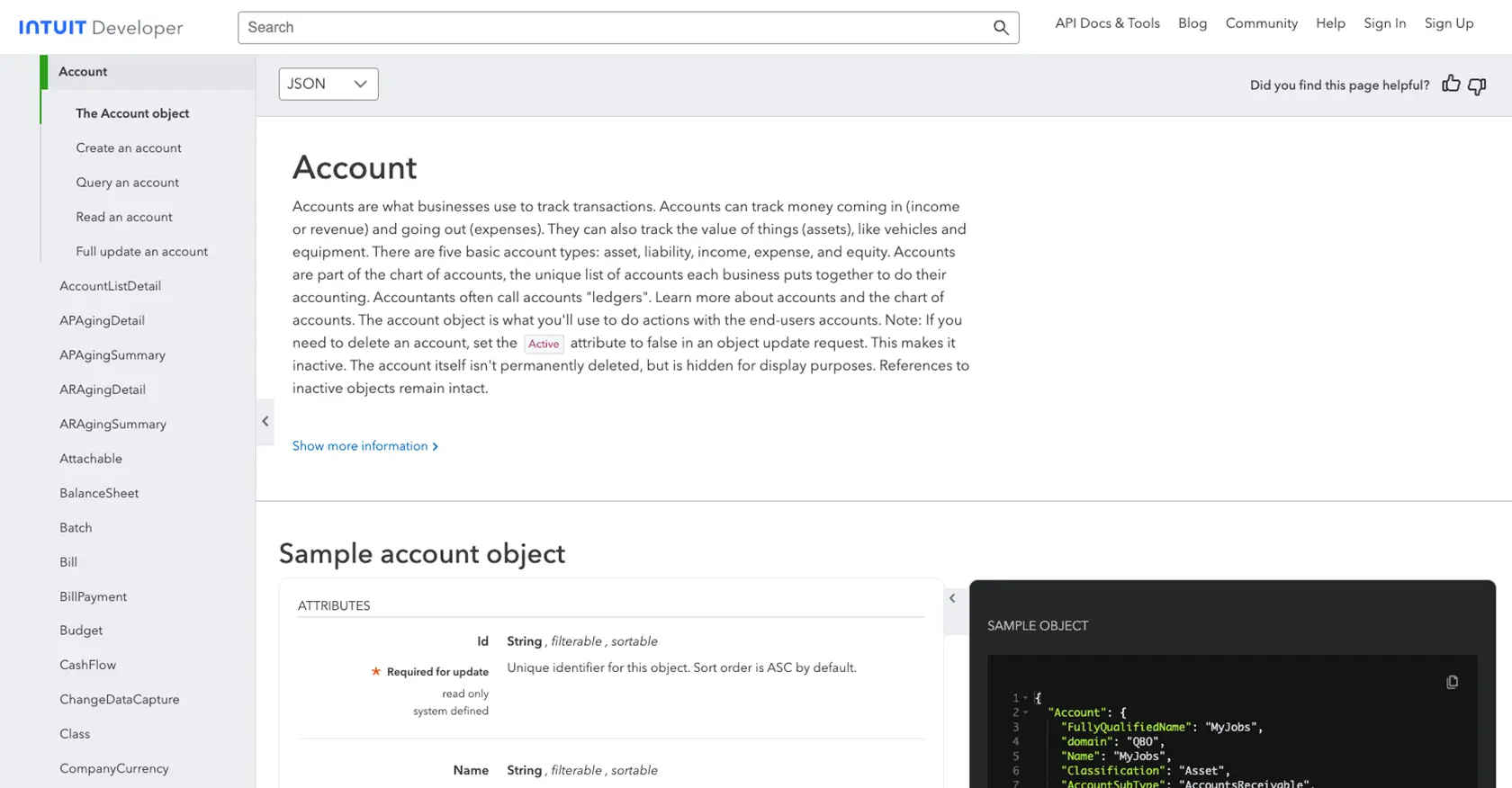
Conclusion and Best Practices for QuickBooks API Integration
Integrating with the QuickBooks API using PHP can significantly enhance your business's financial management capabilities by automating data synchronization and reducing manual entry. By following the steps outlined in this guide, you can efficiently retrieve account information from QuickBooks and ensure seamless integration with your existing systems.
Best Practices for Secure and Efficient QuickBooks API Usage
- Securely Store Credentials: Always store your OAuth credentials, such as the Client ID and Client Secret, securely. Consider using environment variables or a secure vault to prevent unauthorized access.
- Handle Rate Limiting: QuickBooks API has rate limits to prevent abuse. Ensure your application handles these limits gracefully by implementing retry logic or backoff strategies. For more details, refer to the QuickBooks API documentation.
- Standardize Data Fields: When integrating with multiple platforms, standardize data fields to maintain consistency across systems. This practice helps in data transformation and reduces discrepancies.
Streamline Your Integration Process with Endgrate
If you're looking to simplify your integration efforts and focus on your core product, consider using Endgrate. With Endgrate, you can build once for each use case and leverage a unified API endpoint to connect with multiple platforms, including QuickBooks. This approach saves time and resources, providing an intuitive integration experience for your customers.
Visit Endgrate to learn more about how you can streamline your integration process and enhance your product's capabilities.
Read More
- https://endgrate.com/provider/quickbooks
- https://developer.intuit.com/app/developer/qbo/docs/get-started/start-developing-your-app
- https://developer.intuit.com/app/developer/qbo/docs/get-started/get-client-id-and-client-secret
- https://developer.intuit.com/app/developer/qbo/docs/get-started/app-settings
- https://developer.intuit.com/app/developer/qbo/docs/api/accounting/all-entities/account
Ready to get started?