Using the ZoomInfo API to Get Companies (with Javascript examples)
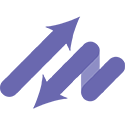
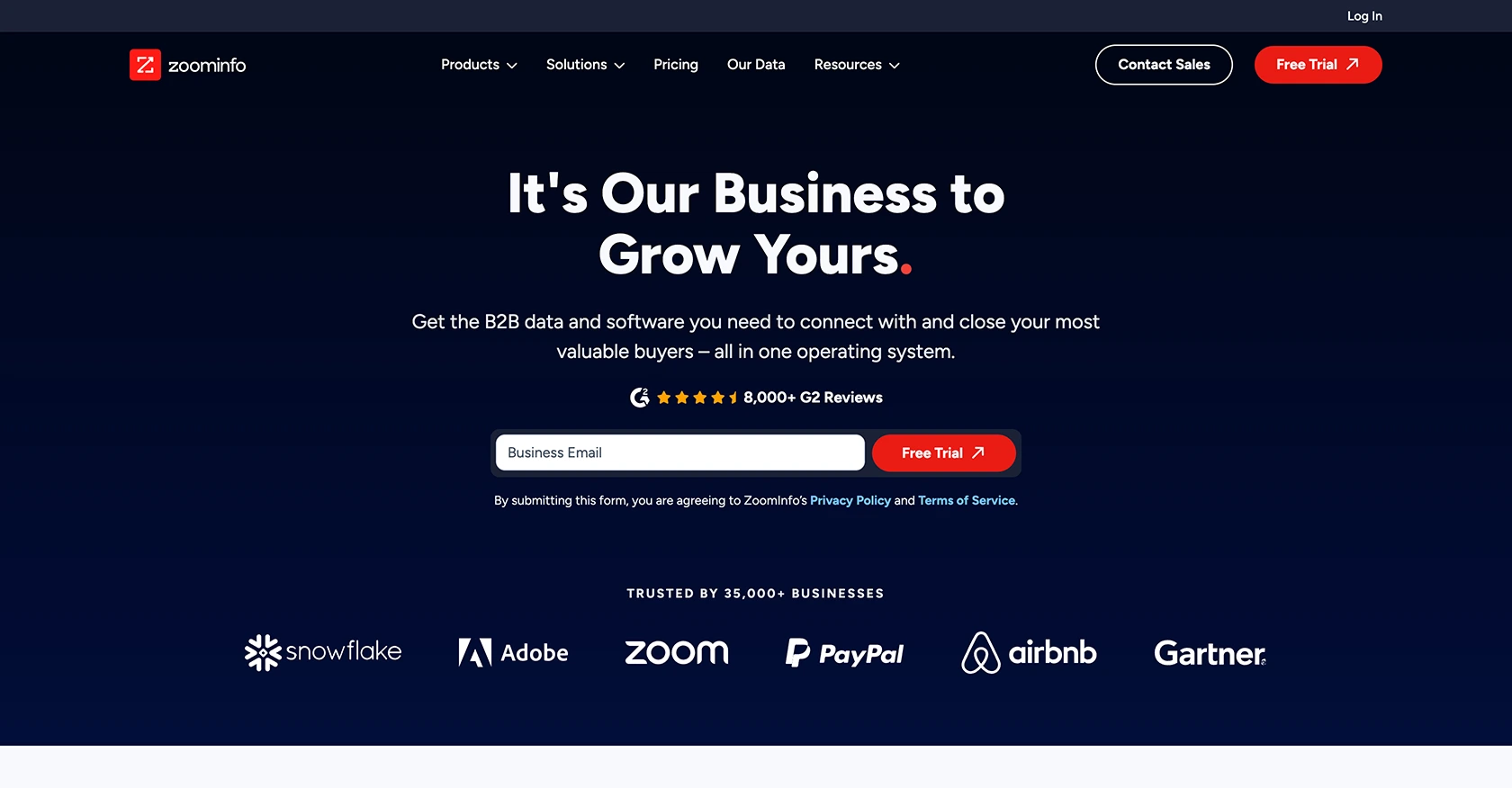
Introduction to ZoomInfo API
ZoomInfo is a powerful business intelligence platform that provides comprehensive data on companies and professionals. It is widely used by sales and marketing teams to enhance their lead generation and customer relationship strategies. With its extensive database, ZoomInfo offers valuable insights into company details, including industry, revenue, and employee count.
For developers, integrating with the ZoomInfo API can unlock a wealth of opportunities to access and manage company data programmatically. By using the API, developers can retrieve detailed information about companies, which can be used to enrich CRM systems, automate data entry, or enhance business analytics.
In this article, we will explore how to use the ZoomInfo API to get company information using JavaScript. This integration can be particularly useful for applications that require up-to-date business data for decision-making or customer engagement purposes.
Setting Up a ZoomInfo Test or Sandbox Account
Before you can start using the ZoomInfo API to retrieve company data, you need to set up a test or sandbox account. This will allow you to safely experiment with the API without affecting live data. ZoomInfo provides a custom authentication method, which you will need to configure to access the API.
Creating a ZoomInfo Developer Account
To begin, you need to create a ZoomInfo developer account. Follow these steps:
- Visit the ZoomInfo API documentation and sign up for a developer account.
- Once registered, log in to your account to access the developer dashboard.
Generating API Credentials for ZoomInfo
After setting up your developer account, you need to generate API credentials to authenticate your requests:
- Navigate to the API credentials section in your developer dashboard.
- Create a new application to obtain your client ID and client secret.
- Ensure you securely store these credentials, as they will be required for API authentication.
Configuring Custom Authentication for ZoomInfo API
ZoomInfo uses a custom authentication method for API access. Here's how to configure it:
- Use your client ID and client secret to obtain an access token. This token will be used to authenticate your API requests.
- Refer to the ZoomInfo authentication documentation for detailed instructions on generating and using the access token.
With your test account and API credentials set up, you're now ready to start making API calls to ZoomInfo to retrieve company data using JavaScript.
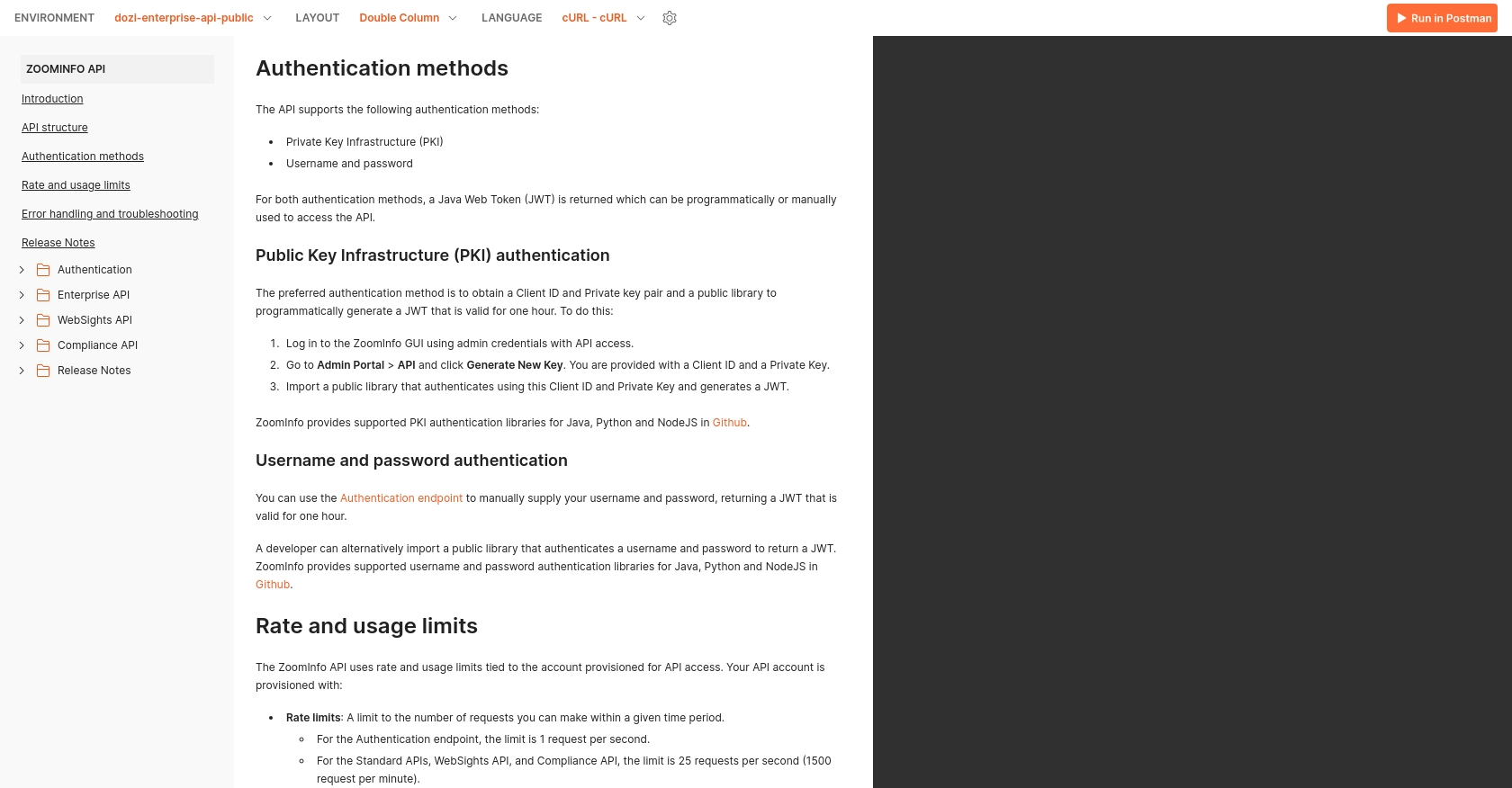
sbb-itb-96038d7
Making API Calls to ZoomInfo Using JavaScript
To interact with the ZoomInfo API and retrieve company data using JavaScript, you need to set up your development environment and write the necessary code to make API requests. This section will guide you through the process, including setting up JavaScript dependencies, writing the code to make API calls, and handling responses and errors.
Setting Up Your JavaScript Environment for ZoomInfo API Integration
Before you start coding, ensure you have Node.js installed on your machine. Node.js provides a runtime environment for executing JavaScript code outside a browser. You will also need the axios
library to handle HTTP requests. Install it using the following command:
npm install axios
Writing JavaScript Code to Retrieve Company Data from ZoomInfo API
With your environment ready, you can now write the JavaScript code to make API calls to ZoomInfo. Create a new file named getCompanies.js
and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://api.zoominfo.com/companies';
const headers = {
'Authorization': 'Bearer Your_Access_Token',
'Content-Type': 'application/json'
};
// Function to get company data
async function getCompanies() {
try {
const response = await axios.get(endpoint, { headers });
const companies = response.data;
console.log(companies);
} catch (error) {
console.error('Error fetching company data:', error.response ? error.response.data : error.message);
}
}
// Call the function
getCompanies();
Replace Your_Access_Token
with the access token you obtained during the authentication setup.
Understanding the JavaScript Code for ZoomInfo API Calls
In the code above, we use the axios
library to make a GET request to the ZoomInfo API endpoint for companies. The request includes headers for authorization and content type. The getCompanies
function handles the API call and logs the retrieved company data to the console. If an error occurs, it logs the error message.
Verifying Successful API Requests and Handling Errors
To verify that your API request was successful, check the console output for the company data. If the request fails, the error message will provide insights into what went wrong. Common issues might include invalid credentials or network errors.
For more detailed error handling, refer to the ZoomInfo API documentation for a list of error codes and their meanings.
Conclusion and Best Practices for ZoomInfo API Integration
Integrating with the ZoomInfo API using JavaScript provides developers with powerful tools to access and manage comprehensive company data. This capability can significantly enhance applications that rely on up-to-date business intelligence for decision-making and customer engagement.
To ensure a successful integration, consider the following best practices:
- Securely Store Credentials: Always store your API credentials, such as the client ID and client secret, securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limiting: Be mindful of ZoomInfo's rate limits to avoid service interruptions. Implement exponential backoff strategies to handle rate limit errors gracefully.
- Standardize Data Fields: When retrieving company data, ensure that the fields are standardized and transformed as needed to fit your application's data model.
- Implement Robust Error Handling: Use detailed error messages from the API to diagnose and resolve issues quickly. Refer to the ZoomInfo API documentation for error code references.
By following these best practices, you can create a reliable and efficient integration with the ZoomInfo API, unlocking valuable insights for your business applications.
For developers looking to streamline their integration processes across multiple platforms, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Endgrate's unified API offers an intuitive integration experience, enabling you to build once for each use case and connect seamlessly to various platforms, including ZoomInfo.
Read More
Ready to get started?