How to Get Accounts with the Sugar Market API in Python
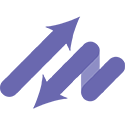
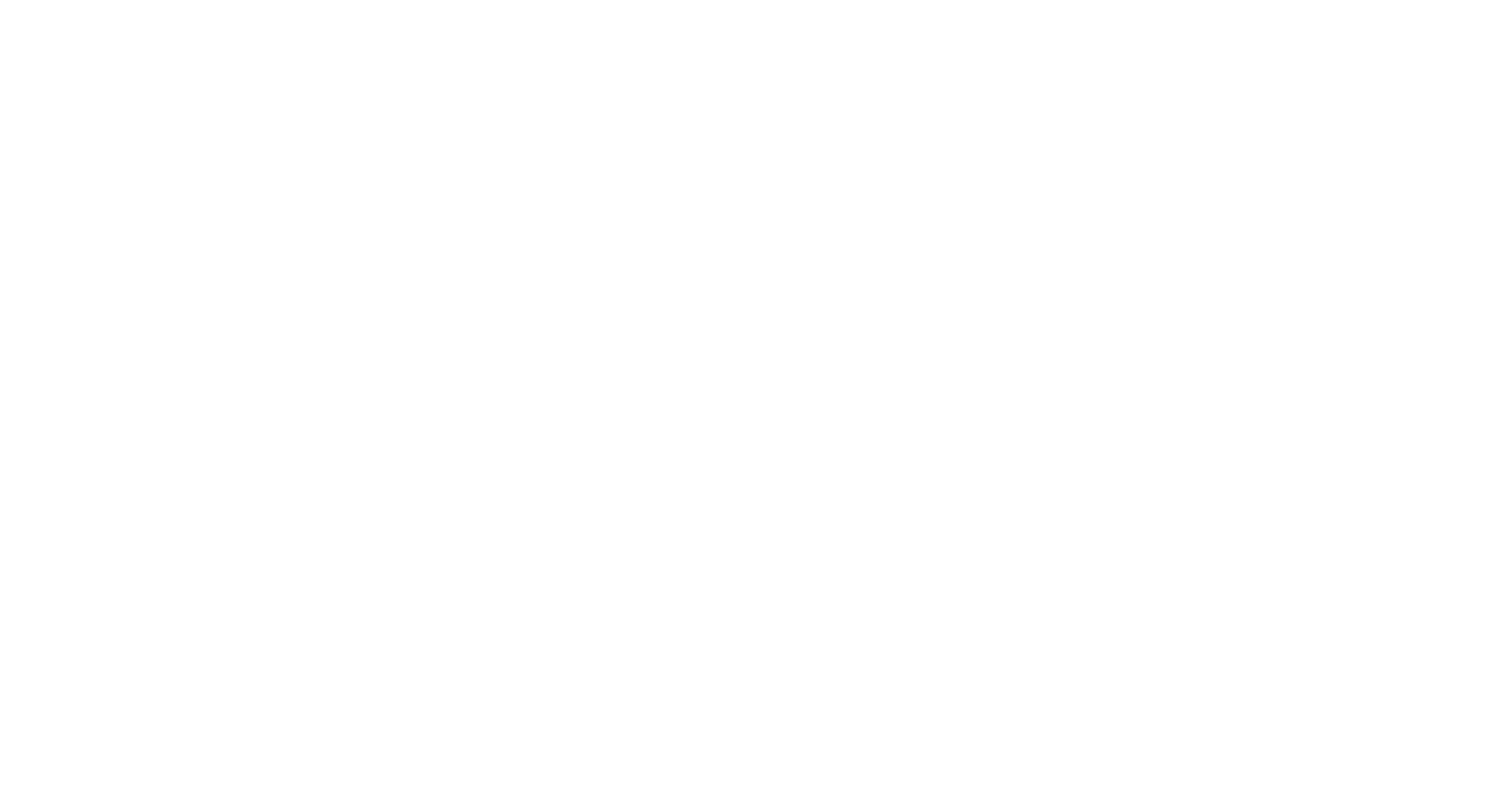
Introduction to Sugar Market API Integration
Sugar Market is a robust marketing automation platform that empowers businesses to enhance their marketing efforts through comprehensive tools and analytics. It offers features such as email marketing, lead nurturing, and campaign management, making it an essential tool for marketing teams aiming to optimize their strategies.
Integrating with the Sugar Market API allows developers to access and manage marketing data programmatically, enabling seamless automation and data synchronization. For example, a developer might want to retrieve account information from Sugar Market to analyze customer engagement and tailor marketing campaigns accordingly.
Setting Up Your Sugar Market Test/Sandbox Account
Before you can start interacting with the Sugar Market API, you'll need to set up a test or sandbox account. This environment allows you to safely test your API calls without affecting live data.
Creating a Sugar Market Account
If you don't already have a Sugar Market account, you can sign up for a free trial or request access to a sandbox account through the Sugar Market website. This will provide you with the necessary credentials to access the API.
Generating API Credentials for Sugar Market
Once your account is set up, you'll need to generate API credentials to authenticate your requests. Sugar Market uses a custom authentication method, so follow these steps to obtain your credentials:
- Log in to your Sugar Market account.
- Navigate to the API settings section, typically found under the account or developer settings.
- Create a new API application by providing the necessary details such as application name and description.
- Once the application is created, you will receive a client ID and client secret. Make sure to store these securely as they will be used for authentication.
Configuring OAuth for Sugar Market API
Although Sugar Market uses a custom authentication method, it may involve OAuth-like steps. Here's how you can configure it:
- Use the client ID and client secret obtained earlier to request an access token.
- Send a POST request to the token endpoint provided in the Sugar Market API documentation.
- Include your client ID and client secret in the request body to receive an access token.
- Store the access token securely, as it will be required for making API calls.
With your Sugar Market account and API credentials set up, you're now ready to start making API calls to retrieve account information. For more detailed information on authentication, refer to the Sugar Market API documentation.
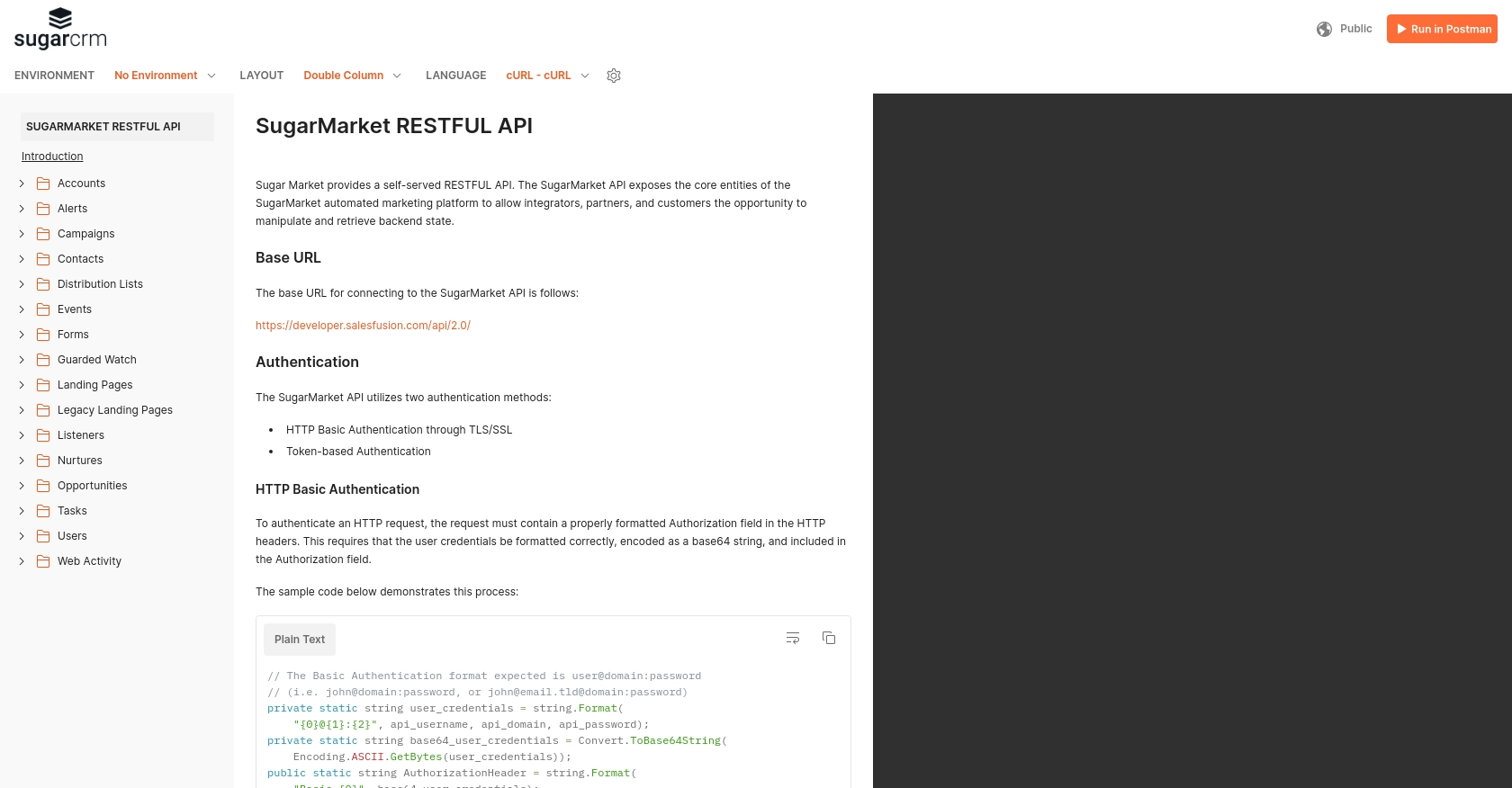
sbb-itb-96038d7
How to Make API Calls to Retrieve Accounts from Sugar Market Using Python
To interact with the Sugar Market API and retrieve account information, you'll need to use Python, a versatile programming language known for its simplicity and readability. This section will guide you through the process of setting up your Python environment, writing the necessary code, and executing API calls to fetch account data from Sugar Market.
Setting Up Your Python Environment for Sugar Market API Integration
Before you begin, ensure that you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests. You can install it using pip:
pip install requests
Writing Python Code to Retrieve Accounts from Sugar Market
With your environment ready, you can now write the Python script to make API calls to Sugar Market. Create a new file named get_sugar_market_accounts.py
and add the following code:
import requests
# Set the API endpoint and headers
endpoint = "https://api.sugarmarket.com/v1/accounts"
headers = {
"Authorization": "Bearer Your_Access_Token",
"Content-Type": "application/json"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
# Parse the JSON data from the response
accounts = response.json()
# Loop through the accounts and print their information
for account in accounts:
print(account)
else:
print(f"Failed to retrieve accounts: {response.status_code} - {response.text}")
Replace Your_Access_Token
with the access token you obtained during the authentication setup.
Executing the Python Script and Verifying API Call Success
Run the script from your terminal or command line using the following command:
python get_sugar_market_accounts.py
If successful, the script will output the account information retrieved from Sugar Market. You can verify the data by checking your Sugar Market sandbox account to ensure it matches the returned data.
Handling Errors and Troubleshooting API Calls to Sugar Market
While making API calls, you may encounter errors. It's crucial to handle these gracefully. The script above checks for a successful response with a status code of 200. If the request fails, it prints the status code and error message. Refer to the Sugar Market API documentation for detailed error code explanations and troubleshooting tips.
Conclusion and Best Practices for Sugar Market API Integration
Integrating with the Sugar Market API using Python provides a powerful way to automate and enhance your marketing efforts. By retrieving account information programmatically, you can gain valuable insights and tailor your marketing strategies to better engage with your customers.
Best Practices for Secure and Efficient Sugar Market API Usage
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Avoid hardcoding them in your scripts and consider using environment variables or secure vaults.
- Handle Rate Limiting: Be aware of any rate limits imposed by the Sugar Market API. Implement retry logic and exponential backoff to handle rate limit errors gracefully. Refer to the Sugar Market API documentation for specific rate limit details.
- Standardize Data Fields: When retrieving data, ensure that you standardize and transform fields as needed to maintain consistency across your application.
Enhance Your Integration Strategy with Endgrate
While building integrations with the Sugar Market API can be rewarding, it can also be time-consuming and complex, especially when dealing with multiple platforms. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Sugar Market.
By using Endgrate, you can save time and resources, allowing you to focus on your core product development. Build once for each use case and enjoy an intuitive integration experience for your customers. Explore how Endgrate can streamline your integration efforts by visiting Endgrate.
Read More
Ready to get started?