Using the OnePageCRM API to Get Contacts in Javascript
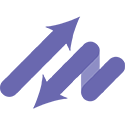
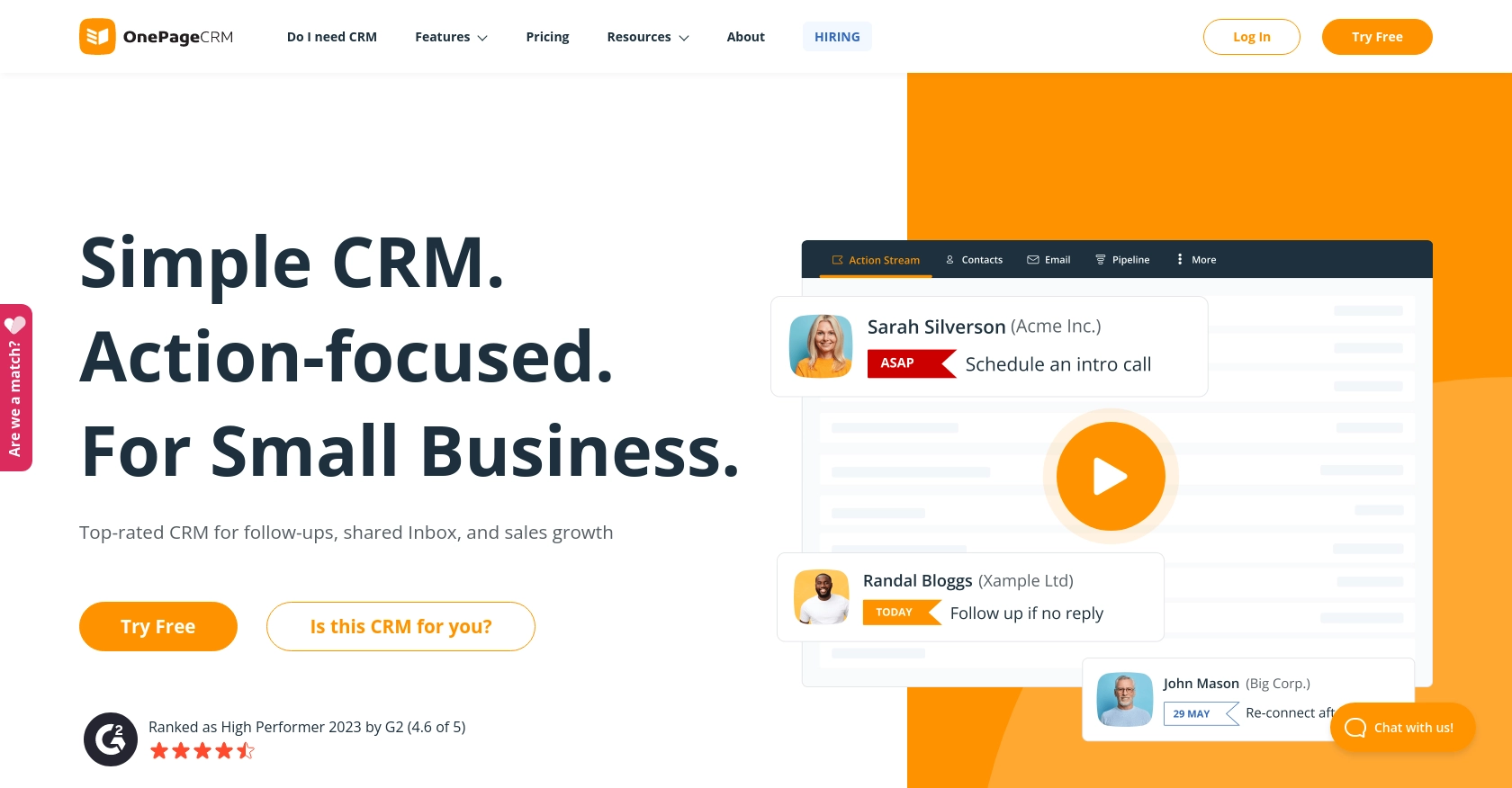
Introduction to OnePageCRM
OnePageCRM is a dynamic CRM platform designed to simplify sales processes and enhance productivity for businesses. It offers a streamlined approach to managing contacts, deals, and tasks, making it an ideal choice for sales teams looking to optimize their workflow.
Integrating with OnePageCRM's API allows developers to efficiently access and manage contact data, enabling seamless automation of sales activities. For example, a developer might use the OnePageCRM API to retrieve a list of contacts and integrate this data into a custom dashboard, providing sales teams with real-time insights and updates.
Setting Up Your OnePageCRM Test/Sandbox Account
Before you can start integrating with the OnePageCRM API, you'll need to set up a test or sandbox account. This will allow you to experiment with the API without affecting live data.
Creating a OnePageCRM Account
If you don't already have a OnePageCRM account, you can sign up for a free trial on their website. This trial will give you access to all the features you need to test the API.
- Visit the OnePageCRM website and click on the "Sign Up" button.
- Fill in the required information, such as your name, email, and password, to create your account.
- Once registered, you will receive a confirmation email. Follow the instructions in the email to verify your account.
Generating API Credentials for OnePageCRM
To interact with the OnePageCRM API, you'll need to generate API credentials. These credentials will include a client ID and client secret, which are necessary for authenticating your API requests.
- Log in to your OnePageCRM account.
- Navigate to the "Settings" section from the dashboard.
- Under "API & Integrations," select "API Keys."
- Click on "Generate New API Key" and provide a name for your key to easily identify it later.
- Once generated, make sure to copy the API key and store it securely, as you will need it for authentication in your code.
Understanding OnePageCRM Custom Authentication
OnePageCRM uses a custom authentication method for API access. This involves using the API key you generated to authenticate your requests. Ensure that you include this key in the headers of your API calls to successfully interact with the OnePageCRM API.
For more detailed information on authentication, refer to the OnePageCRM API documentation.
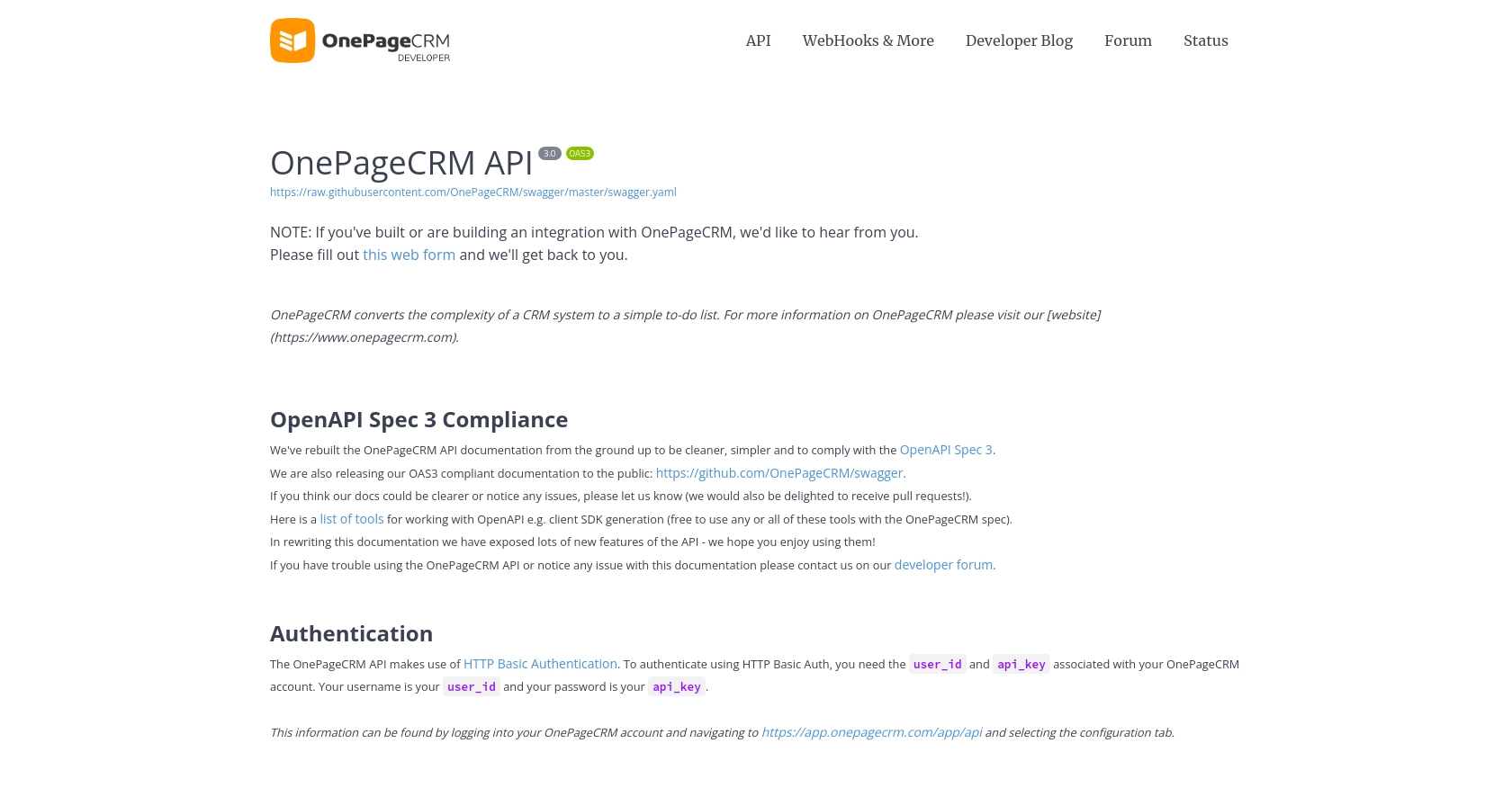
sbb-itb-96038d7
Making API Calls to Retrieve Contacts from OnePageCRM Using JavaScript
To interact with the OnePageCRM API and retrieve contacts, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment, writing the code, and handling responses and errors effectively.
Setting Up Your JavaScript Environment for OnePageCRM API
Before you begin coding, ensure you have a suitable environment for running JavaScript. You can use Node.js, which is a popular choice for executing JavaScript outside the browser.
- Download and install Node.js if you haven't already.
- Use npm (Node Package Manager) to install any necessary packages. For this tutorial, you'll need the
axios
library to make HTTP requests. Run the following command in your terminal:
npm install axios
Writing JavaScript Code to Fetch Contacts from OnePageCRM
Now that your environment is set up, you can write the JavaScript code to interact with the OnePageCRM API. Create a new file named get_contacts.js
and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://app.onepagecrm.com/api/v3/contacts';
const headers = {
'Authorization': 'Bearer YOUR_API_KEY'
};
// Function to get contacts
async function getContacts() {
try {
const response = await axios.get(endpoint, { headers });
const contacts = response.data.contacts;
console.log('Contacts retrieved successfully:', contacts);
} catch (error) {
console.error('Error fetching contacts:', error.response ? error.response.data : error.message);
}
}
// Call the function
getContacts();
Replace YOUR_API_KEY
with the API key you generated earlier. This code uses the axios
library to make a GET request to the OnePageCRM API endpoint for contacts. It handles the response by logging the contacts to the console and catches any errors that occur during the request.
Running the JavaScript Code and Verifying Results
To execute your JavaScript code, run the following command in your terminal:
node get_contacts.js
If successful, you should see a list of contacts printed in your terminal. This confirms that your API call was successful and that you have retrieved the contacts from OnePageCRM.
Handling Errors and Understanding Response Codes from OnePageCRM API
When making API calls, it's crucial to handle potential errors gracefully. The OnePageCRM API may return various HTTP status codes, such as:
- 200 OK: The request was successful.
- 400 Bad Request: The request was invalid or cannot be served.
- 401 Unauthorized: Authentication failed or user does not have permissions.
- 403 Forbidden: The request is understood, but it has been refused.
- 404 Not Found: The requested resource could not be found.
- 500 Internal Server Error: An error occurred on the server.
By checking the error.response
object in the catch block, you can log specific error messages and take appropriate actions based on the status code.
Best Practices for Using OnePageCRM API in JavaScript
When integrating with the OnePageCRM API, it's essential to follow best practices to ensure efficient and secure interactions. Here are some recommendations:
- Securely Store API Credentials: Always store your API keys securely and avoid hardcoding them in your source code. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Be mindful of the API's rate limits to prevent your application from being throttled. Implement retry logic with exponential backoff to handle rate limit responses gracefully.
- Standardize Data Fields: Ensure that the data retrieved from OnePageCRM is standardized and transformed as needed to fit your application's requirements. This will help maintain consistency across different integrations.
Enhance Your Integration Strategy with Endgrate
Building and maintaining integrations can be time-consuming and complex, especially when dealing with multiple platforms. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including OnePageCRM.
With Endgrate, you can:
- Save Time and Resources: Focus on your core product while Endgrate handles the integration complexities.
- Build Once, Deploy Everywhere: Create a single integration for each use case and apply it across multiple platforms effortlessly.
- Offer a Seamless Experience: Provide your customers with an intuitive and consistent integration experience.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate today.
Read More
Ready to get started?