How to Get Customers with the Commerce7 API in Javascript
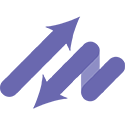
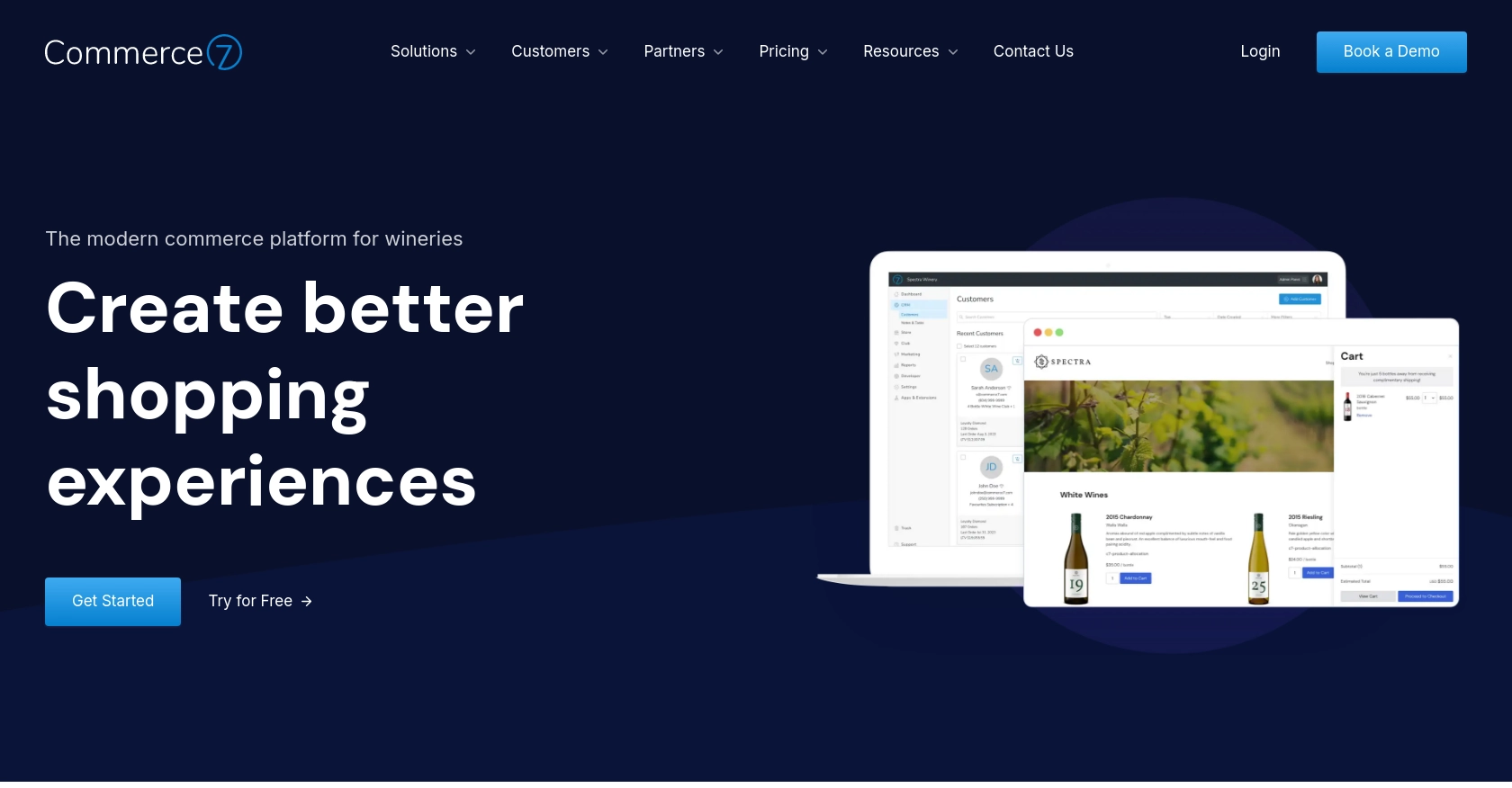
Introduction to Commerce7 API Integration
Commerce7 is a modern commerce platform designed specifically for the wine industry, offering a seamless experience for managing sales, customer relationships, and marketing efforts. Its robust API allows developers to build integrations that enhance the functionality of Commerce7, making it a powerful tool for wineries and retailers looking to streamline their operations.
Integrating with the Commerce7 API can enable developers to efficiently manage customer data, automate marketing campaigns, and personalize customer interactions. For example, a developer might use the Commerce7 API to retrieve customer information and tailor marketing strategies based on purchase history and preferences, thereby enhancing customer engagement and driving sales.
Setting Up Your Commerce7 Developer Account and App
Before you can start interacting with the Commerce7 API, you'll need to set up a developer account and create an app within the Commerce7 platform. This process will provide you with the necessary credentials to authenticate your API requests.
Creating a Commerce7 Developer Account
If you don't already have a Commerce7 account, follow these steps to create one:
- Visit the Commerce7 website and sign up for a developer account.
- Once registered, log in to your account to access the developer dashboard.
Creating an App in the Commerce7 App Dev Center
To interact with the Commerce7 API, you'll need to create an app. Here's how:
- Navigate to the Developers tab and select the App Dev Center, or go directly to https://dev-center.platform.commerce7.com.
- Click the Add App button to start the app creation process.
- Fill in the required details about your app, such as its name and description.
- Select the API endpoints you need access to for your integration.
- Once completed, save your app to generate the App ID and App Secret Key.
Obtaining Your App Credentials
After creating your app, you'll receive an App ID and an App Secret Key. These credentials are essential for authenticating your API requests:
- App ID: This is the unique identifier for your app.
- App Secret Key: This key is used for authenticating requests. Ensure you store it securely and do not expose it in your frontend code.
Authentication with Commerce7 API
Commerce7 uses Basic Auth for API authentication. You'll need to include your App ID and App Secret Key in your requests:
// Example of setting up Basic Auth headers in JavaScript
const headers = new Headers();
headers.append("Authorization", "Basic " + btoa("Your_App_ID:Your_App_Secret_Key"));
Replace Your_App_ID
and Your_App_Secret_Key
with your actual credentials.
For more detailed information on setting up your app, refer to the Commerce7 App Creation Guide and the Commerce7 API Documentation.
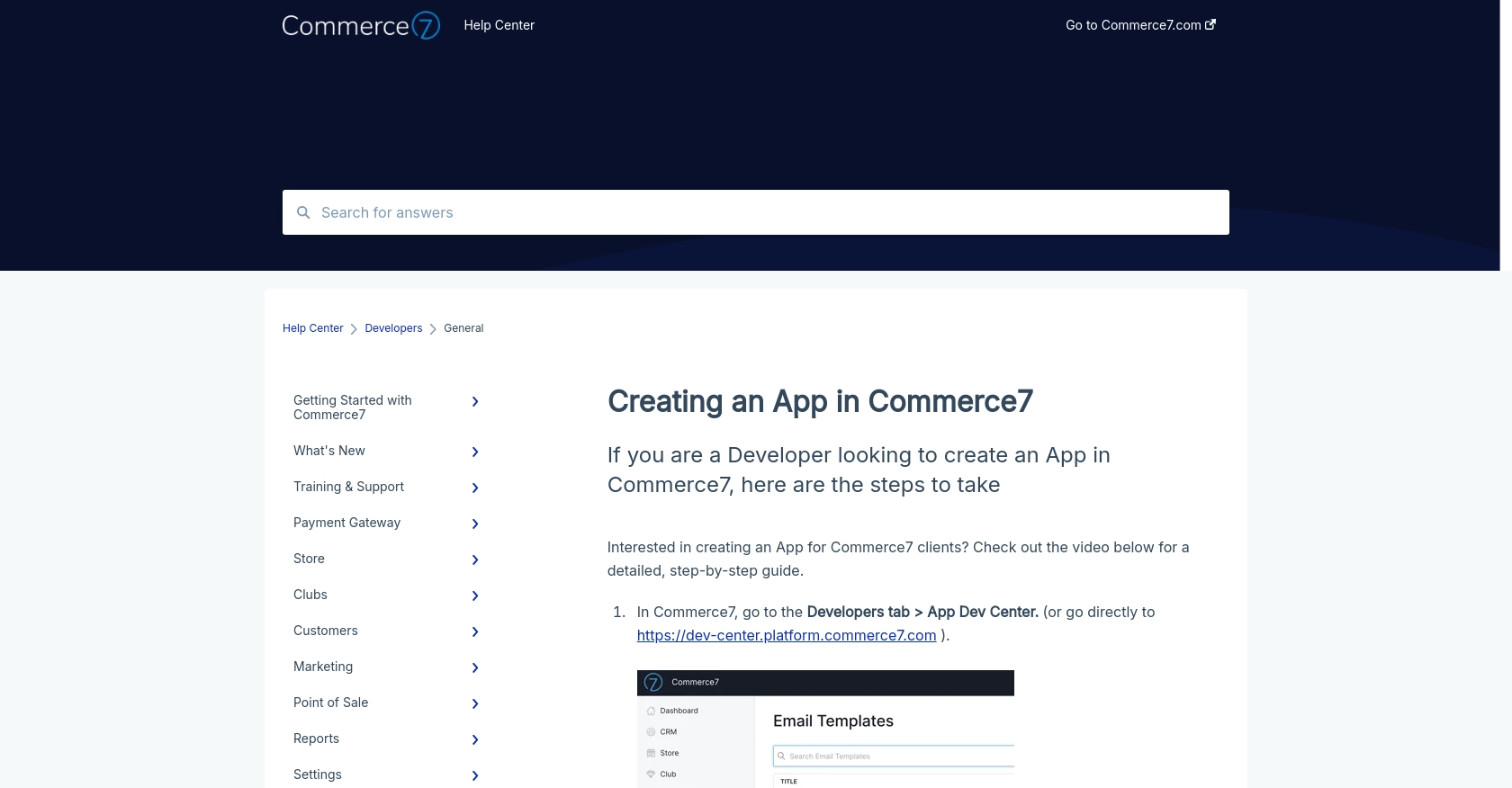
sbb-itb-96038d7
Making API Calls to Retrieve Customers with Commerce7 API in JavaScript
To interact with the Commerce7 API and retrieve customer data, you'll need to make HTTP requests using JavaScript. This section will guide you through the process of setting up your environment, writing the code, and handling responses and errors effectively.
Setting Up Your JavaScript Environment for Commerce7 API
Before making API calls, ensure you have a working JavaScript environment. You can use Node.js or a browser-based environment. For this tutorial, we'll use Node.js. Make sure you have Node.js installed on your machine.
Installing Required Dependencies
To make HTTP requests, we'll use the popular axios
library. Install it using npm:
npm install axios
Writing JavaScript Code to Retrieve Customers from Commerce7
Create a file named get_customers.js
and add the following code:
const axios = require('axios');
// Set up your credentials
const appId = 'Your_App_ID';
const appSecretKey = 'Your_App_Secret_Key';
const tenantId = 'Your_Tenant_ID';
// Configure the request headers
const headers = {
'Authorization': 'Basic ' + Buffer.from(appId + ':' + appSecretKey).toString('base64'),
'Content-Type': 'application/json'
};
// Define the API endpoint
const endpoint = `https://api.commerce7.com/v1/customer?tenant=${tenantId}`;
// Make the GET request to retrieve customers
axios.get(endpoint, { headers })
.then(response => {
console.log('Customers retrieved successfully:', response.data.customers);
})
.catch(error => {
console.error('Error retrieving customers:', error.response ? error.response.data : error.message);
});
Replace Your_App_ID
, Your_App_Secret_Key
, and Your_Tenant_ID
with your actual credentials.
Running the Code and Verifying the Output
Run the code using the following command:
node get_customers.js
If successful, you should see a list of customers printed in the console. Verify the data by checking the customer records in your Commerce7 sandbox account.
Handling Errors and Understanding Commerce7 API Error Codes
When making API calls, it's crucial to handle potential errors. The Commerce7 API may return various error codes, such as:
- 400 Bad Request: The request was invalid or cannot be served. Check your request parameters.
- 401 Unauthorized: Authentication failed. Verify your App ID and App Secret Key.
- 404 Not Found: The requested resource could not be found. Ensure the endpoint URL is correct.
- 500 Internal Server Error: An error occurred on the server. Try again later.
Refer to the Commerce7 API Documentation for more details on error handling.
Optimizing API Calls with Commerce7 Rate Limiting
Commerce7 imposes a rate limit of 100 requests per minute per tenant. To avoid hitting this limit, consider implementing rate limiting strategies in your application. For large datasets, use cursor-based pagination, which has no rate limits and provides better response times.
For more information on rate limiting, refer to the Commerce7 API Documentation.
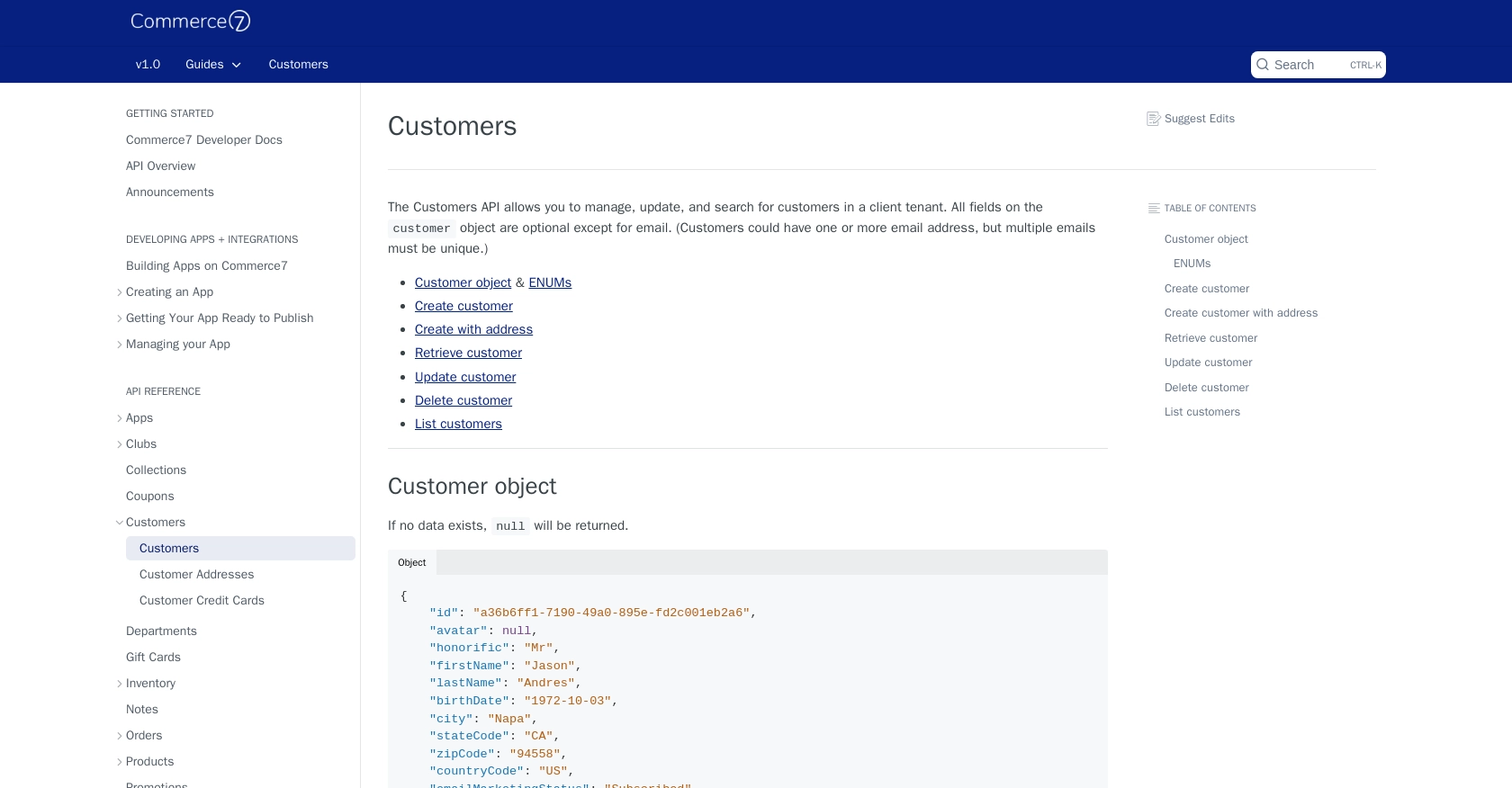
Conclusion and Best Practices for Using Commerce7 API in JavaScript
Integrating with the Commerce7 API using JavaScript provides a powerful way to manage customer data and enhance your business operations. By following the steps outlined in this guide, you can efficiently retrieve customer information and leverage it to personalize marketing strategies and improve customer engagement.
Best Practices for Secure and Efficient API Integration with Commerce7
- Secure Storage of Credentials: Always store your App ID and App Secret Key securely. Avoid exposing them in frontend code to prevent unauthorized access.
- Implement Rate Limiting: Be mindful of Commerce7's rate limit of 100 requests per minute per tenant. Implement strategies to handle rate limits effectively, such as using cursor-based pagination for large datasets.
- Handle Errors Gracefully: Ensure your application can handle API errors by checking response codes and implementing retry logic where appropriate.
- Data Transformation and Standardization: Standardize data fields to ensure consistency across different systems and improve data quality.
Streamlining Integration Development with Endgrate
Building and maintaining multiple integrations can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Commerce7. By using Endgrate, you can save time and resources, allowing you to focus on your core product development.
Explore how Endgrate can enhance your integration experience by visiting Endgrate and discover how it can streamline your integration processes.
Read More
Ready to get started?