How to Get Weekly Page Views with the Google Analytics API in Javascript
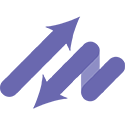
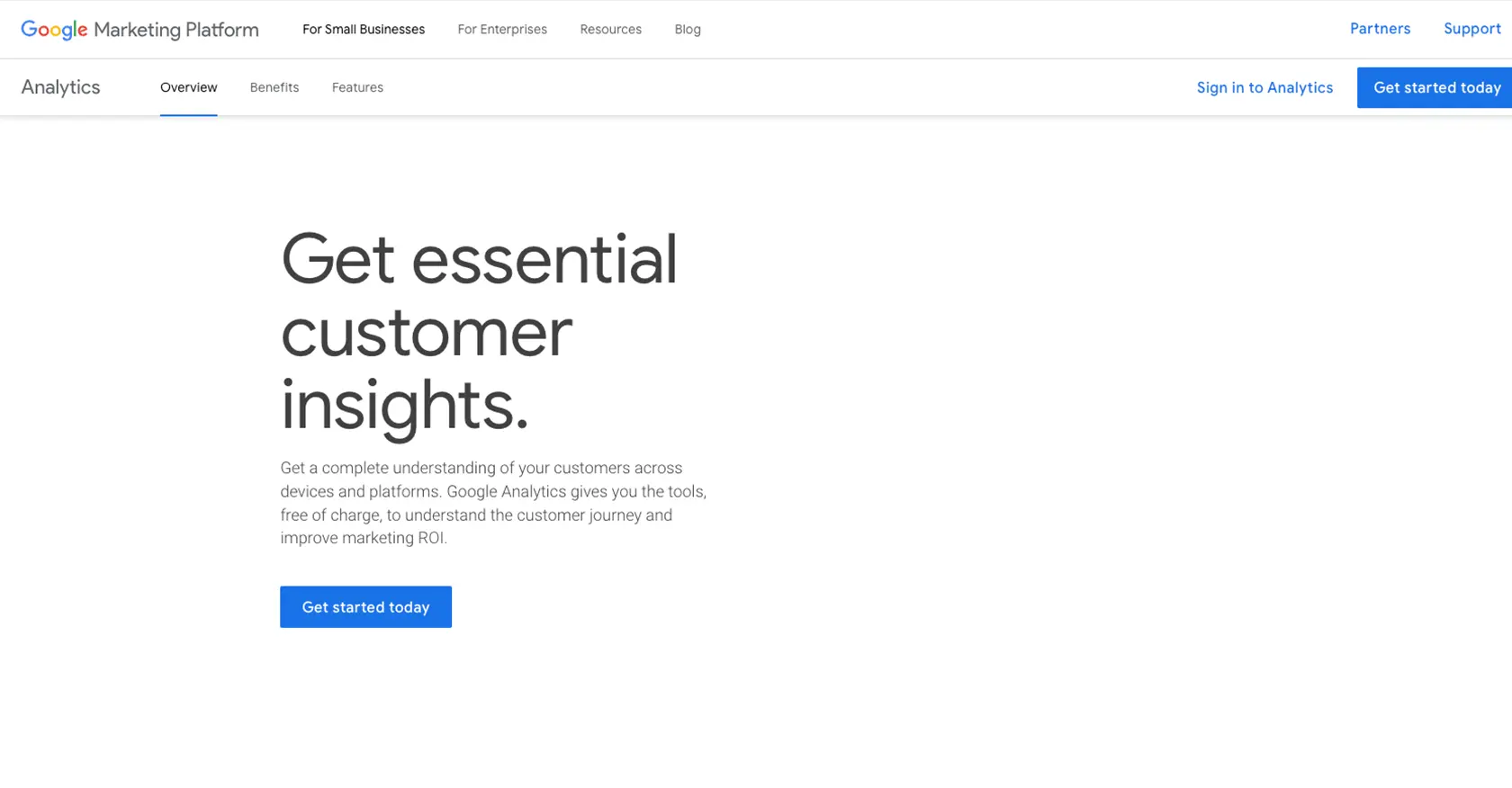
Introduction to Google Analytics API
Google Analytics is a powerful tool that provides insights into website traffic and user behavior. It helps businesses understand their audience, track marketing campaigns, and make data-driven decisions to improve their online presence.
Integrating with the Google Analytics API allows developers to access detailed analytics data programmatically. This can be particularly useful for automating reporting tasks or integrating analytics data with other business applications. For example, a developer might use the Google Analytics API to retrieve weekly page views and display them on a custom dashboard, providing real-time insights into website performance.
Setting Up Your Google Analytics API Test Account
Before you can start using the Google Analytics API to retrieve weekly page views, you need to set up a Google Cloud project and configure OAuth 2.0 authentication. This setup allows you to securely access Google Analytics data programmatically.
Create a Google Cloud Project for Google Analytics API
- Go to the Google Cloud Console.
- Click on the Menu icon, navigate to IAM & Admin, and select Create a Project.
- Enter a descriptive name for your project and click Create.
Enable Google Analytics API in Your Project
- In the Google Cloud Console, go to APIs & Services and click on Library.
- Search for Google Analytics API and click on it.
- Click Enable to activate the API for your project.
Configure OAuth Consent Screen for Google Analytics API
- In the Google Cloud Console, navigate to APIs & Services and select OAuth consent screen.
- Select the user type and fill out the required fields, such as application name and support email.
- Click Save and Continue.
Create OAuth 2.0 Credentials for Google Analytics API
- Go to APIs & Services and click on Credentials.
- Click Create Credentials and select OAuth client ID.
- Choose Web application as the application type.
- Enter a name for your OAuth client and add authorized redirect URIs.
- Click Create to generate your client ID and client secret.
- Note down the client ID and client secret as you will need them for authentication.
With your Google Cloud project and OAuth 2.0 credentials set up, you are now ready to make API calls to Google Analytics and retrieve data such as weekly page views.
For more detailed instructions, refer to the official documentation: Create a Google Cloud Project, Enable APIs, Configure OAuth Consent, and Create Credentials.
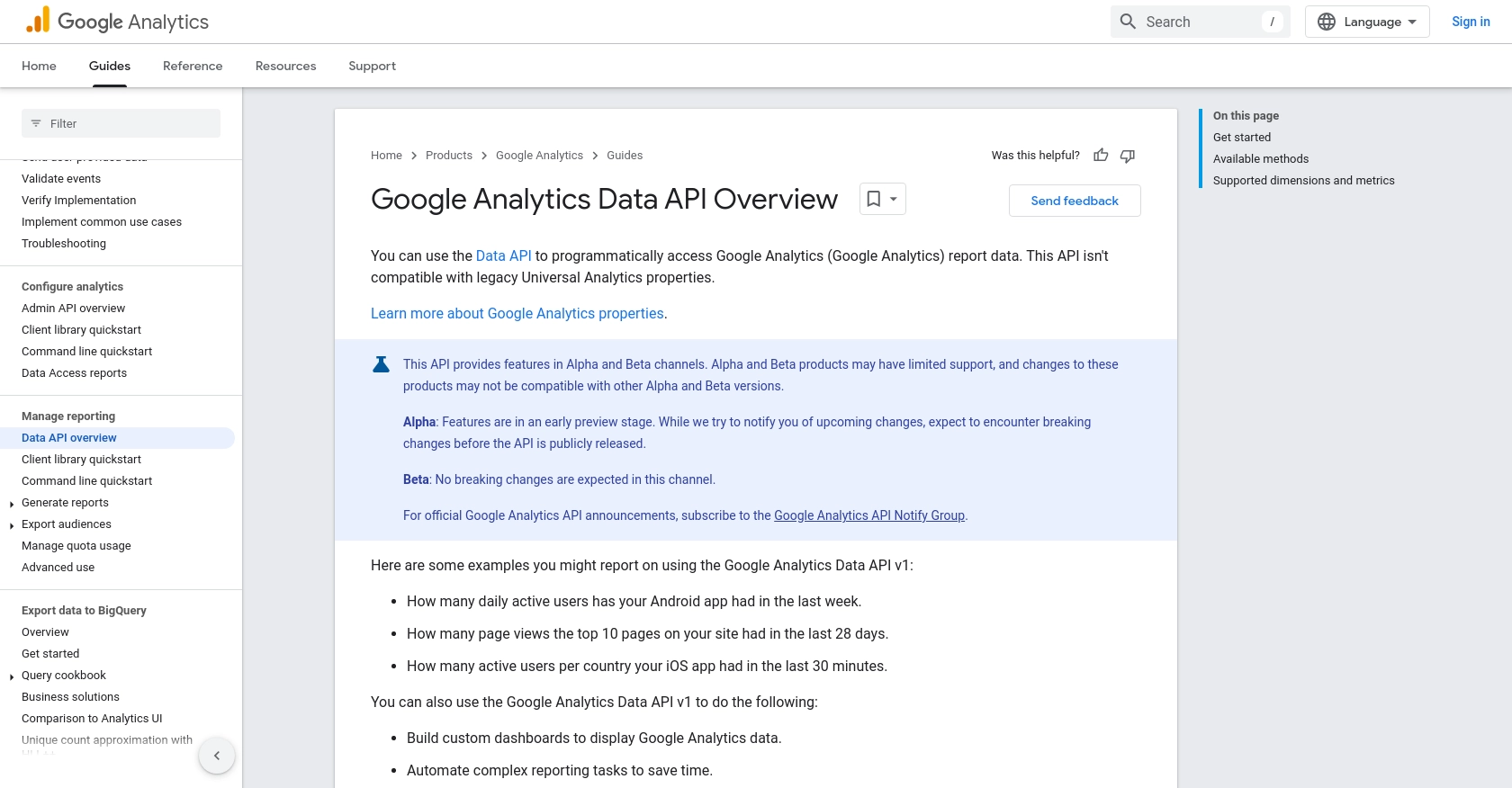
sbb-itb-96038d7
Making API Calls to Retrieve Weekly Page Views from Google Analytics Using JavaScript
To access weekly page views from Google Analytics using JavaScript, you'll need to interact with the Google Analytics Data API. This section will guide you through the necessary steps, including setting up your JavaScript environment, installing dependencies, and executing the API call.
Setting Up Your JavaScript Environment for Google Analytics API
Before making API calls, ensure you have Node.js installed on your machine. Node.js allows you to run JavaScript code outside of a browser, which is essential for server-side operations.
- Download and install Node.js from the official website.
- Verify the installation by running the following commands in your terminal:
node -v
npm -v
Installing Required Dependencies for Google Analytics API
You'll need the Google Analytics Data API client library for Node.js to interact with the API. Install it using npm:
npm install @google-analytics/data
Writing JavaScript Code to Fetch Weekly Page Views from Google Analytics
Create a JavaScript file named getWeeklyPageViews.js
and add the following code to it:
// Import the Google Analytics Data API client library
const { BetaAnalyticsDataClient } = require('@google-analytics/data');
// Initialize the client
const analyticsDataClient = new BetaAnalyticsDataClient();
// Function to fetch weekly page views
async function getWeeklyPageViews() {
const propertyId = 'YOUR-GA4-PROPERTY-ID'; // Replace with your Google Analytics 4 property ID
// Make the API call
const [response] = await analyticsDataClient.runReport({
property: `properties/${propertyId}`,
dateRanges: [
{
startDate: '7daysAgo',
endDate: 'yesterday',
},
],
dimensions: [
{
name: 'date',
},
],
metrics: [
{
name: 'pageviews',
},
],
});
// Print the results
console.log('Weekly Page Views:');
response.rows.forEach(row => {
console.log(`Date: ${row.dimensionValues[0].value}, Page Views: ${row.metricValues[0].value}`);
});
}
// Execute the function
getWeeklyPageViews();
Running Your JavaScript Code to Retrieve Data from Google Analytics
Execute the JavaScript file using Node.js to fetch the weekly page views:
node getWeeklyPageViews.js
Upon successful execution, you should see the weekly page views printed in your terminal, with each date and its corresponding page views.
Handling Errors and Verifying API Call Success
To ensure your API call is successful, check the response status and handle any errors that may occur. Modify your code to include error handling:
async function getWeeklyPageViews() {
try {
const [response] = await analyticsDataClient.runReport({
property: `properties/${propertyId}`,
dateRanges: [
{
startDate: '7daysAgo',
endDate: 'yesterday',
},
],
dimensions: [
{
name: 'date',
},
],
metrics: [
{
name: 'pageviews',
},
],
});
console.log('Weekly Page Views:');
response.rows.forEach(row => {
console.log(`Date: ${row.dimensionValues[0].value}, Page Views: ${row.metricValues[0].value}`);
});
} catch (error) {
console.error('Error fetching data:', error);
}
}
By implementing error handling, you can catch and log any issues that arise during the API call, ensuring a more robust integration.
For more detailed information on making API calls, refer to the official documentation: Google Analytics API Basics.
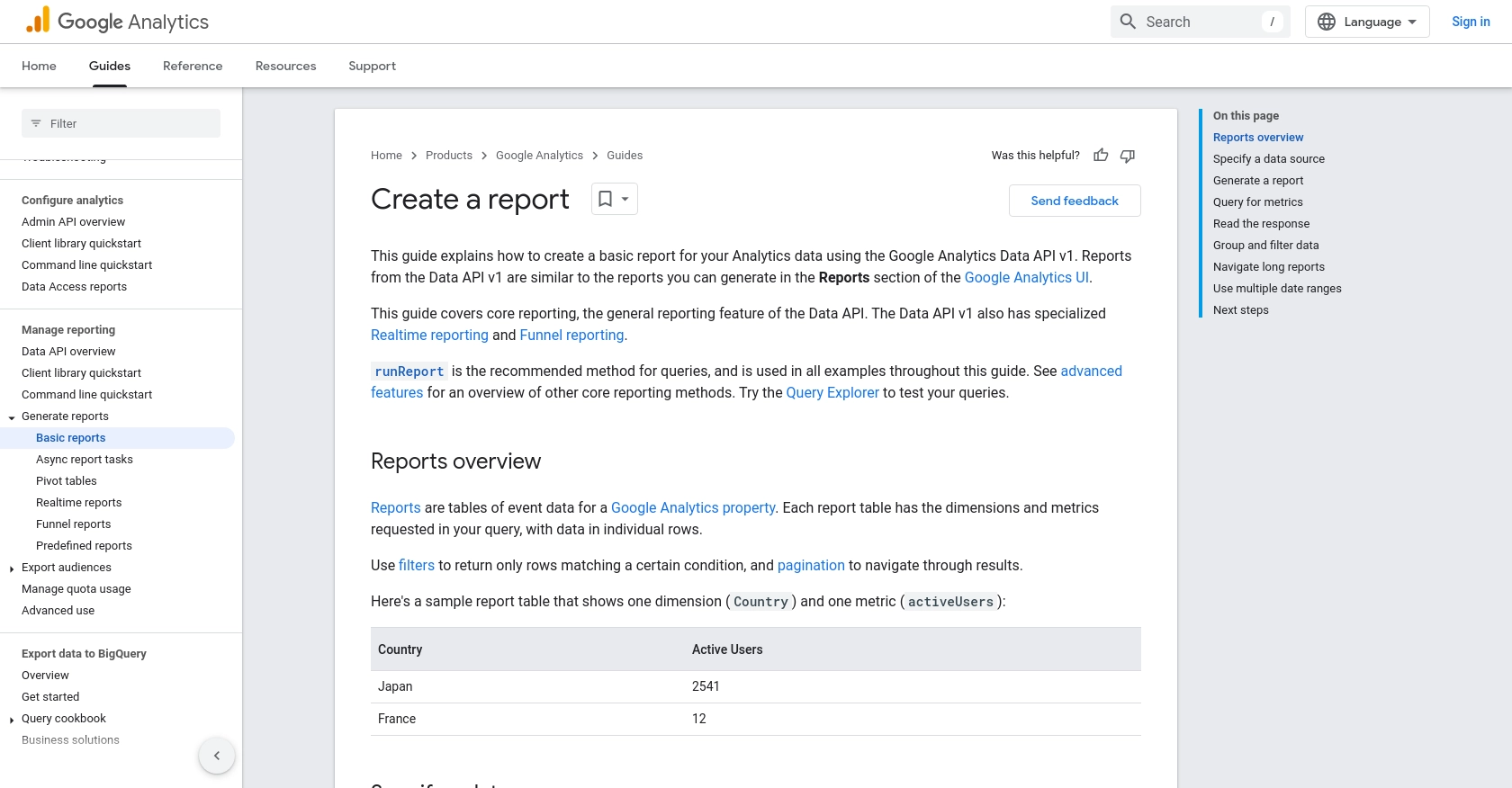
Conclusion and Best Practices for Using Google Analytics API with JavaScript
Integrating Google Analytics API with JavaScript provides a powerful way to access and analyze your website's data programmatically. By following the steps outlined in this guide, you can efficiently retrieve weekly page views and incorporate them into your custom dashboards or applications.
Best Practices for Managing Google Analytics API Integrations
- Securely Store Credentials: Ensure that your OAuth client ID and client secret are stored securely and not exposed in your codebase. Consider using environment variables or secure vaults for sensitive information.
- Handle Rate Limiting: Google Analytics API has rate limits in place. Implement retry logic and exponential backoff strategies to handle rate limit errors gracefully.
- Optimize API Calls: Minimize the number of API calls by batching requests and using efficient data queries to reduce latency and improve performance.
- Regularly Monitor and Update: Keep your integration up-to-date with the latest Google Analytics API changes and monitor its performance to ensure continued reliability.
Enhance Your Integration Strategy with Endgrate
While integrating with Google Analytics API can be highly beneficial, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies integration management across various platforms, including Google Analytics.
By leveraging Endgrate, you can streamline your integration processes, reduce development overhead, and focus on your core product. Visit Endgrate to learn more about how you can enhance your integration strategy and provide a seamless experience for your users.
Read More
- https://endgrate.com/provider/googleanalytics
- https://developers.google.com/analytics/devguides/reporting/data/v1
- https://developers.google.com/workspace/guides/create-project
- https://developers.google.com/workspace/guides/enable-apis
- https://developers.google.com/workspace/guides/configure-oauth-consent
- https://developers.google.com/workspace/guides/create-credentials
- https://developers.google.com/analytics/devguides/reporting/data/v1/basics
Ready to get started?