Using the Younium API to Create or Update Accounts in Python
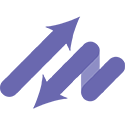
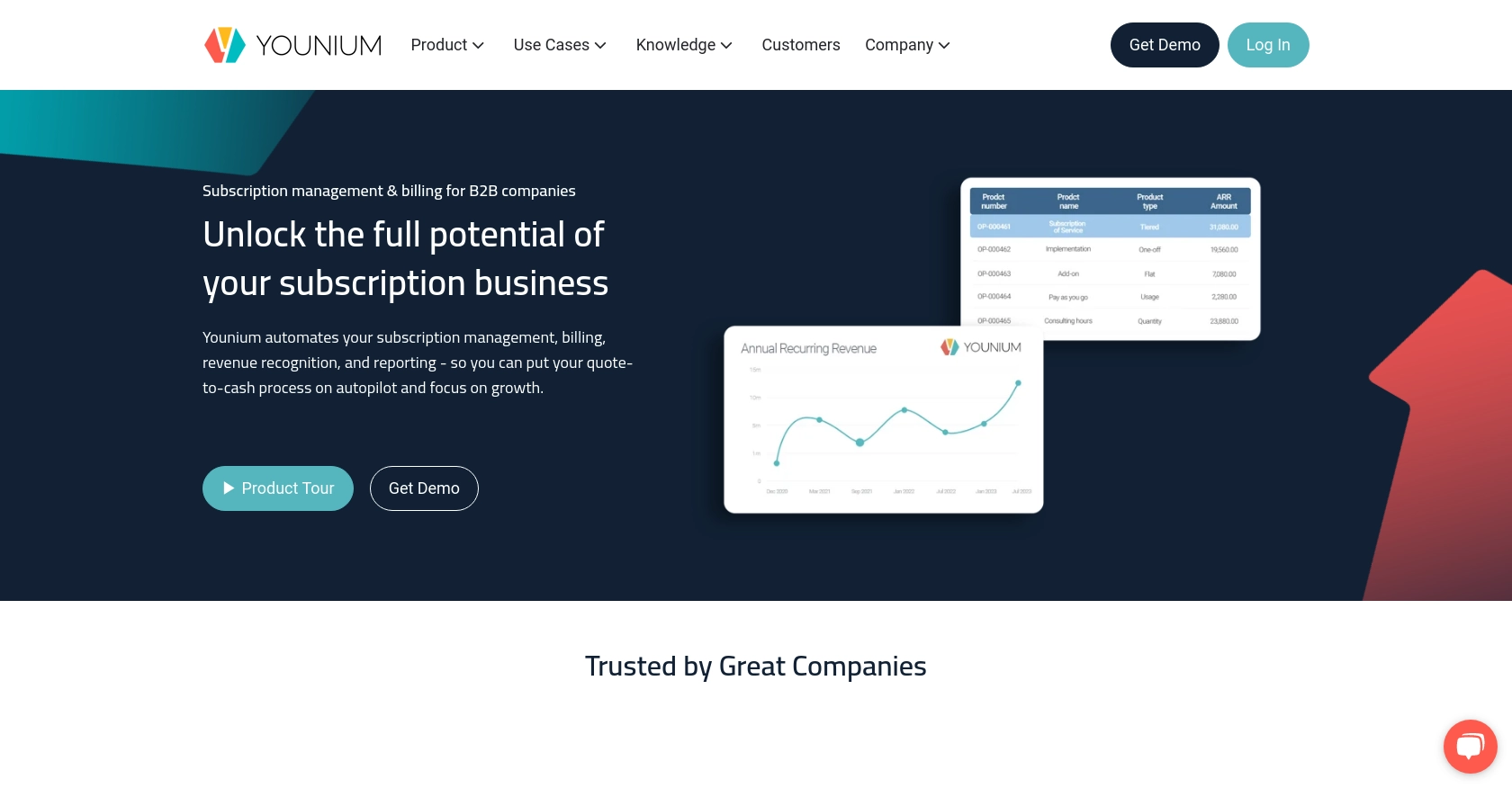
Introduction to Younium API Integration
Younium is a comprehensive subscription management platform designed to streamline billing, invoicing, and financial operations for B2B SaaS companies. It offers robust tools to manage complex subscription models, automate billing processes, and gain insights into financial performance.
Integrating with Younium's API allows developers to efficiently manage account data, automate updates, and ensure seamless financial operations. For example, a developer might use the Younium API to create or update customer accounts, ensuring that billing information is always current and accurate.
Setting Up Your Younium Sandbox Account for API Integration
Before you can start integrating with the Younium API, you'll need to set up a sandbox account. This environment allows you to test API interactions without affecting live data, ensuring a safe space for development and experimentation.
Creating a Younium Sandbox Account
If you don't already have a Younium account, you can sign up for a sandbox account on the Younium website. This will provide you with access to a test environment where you can safely develop and test your API integrations.
- Visit the Younium Developer Portal.
- Follow the instructions to create a new sandbox account.
- Once your account is created, log in to access the sandbox environment.
Generating API Tokens and Client Credentials
To authenticate your API requests, you'll need to generate API tokens and client credentials. Follow these steps to obtain the necessary credentials:
- Log in to your Younium account and open the user profile menu by clicking your name in the top right corner.
- Select "Privacy & Security" from the dropdown menu.
- Navigate to "Personal Tokens" and click "Generate Token".
- Provide a relevant description for the token and click "Create".
- Copy the generated Client ID and Secret Key. These credentials will be used to generate the JWT access token.
Acquiring a JWT Access Token
With your client credentials in hand, you can now generate a JWT access token. This token is necessary for authenticating your API requests:
import requests
# Define the endpoint and headers
url = "https://api.sandbox.younium.com/auth/token"
headers = {"Content-Type": "application/json"}
# Define the request body with client credentials
body = {
"clientId": "Your_Client_ID",
"secret": "Your_Secret_Key"
}
# Make the POST request to obtain the JWT token
response = requests.post(url, json=body, headers=headers)
# Extract the access token from the response
access_token = response.json().get("accessToken")
print("Access Token:", access_token)
Replace Your_Client_ID
and Your_Secret_Key
with the credentials you generated earlier. This script will return a JWT access token, valid for 24 hours, which you will use to authenticate subsequent API calls.
For more details on authentication, refer to the Younium Authentication Documentation.
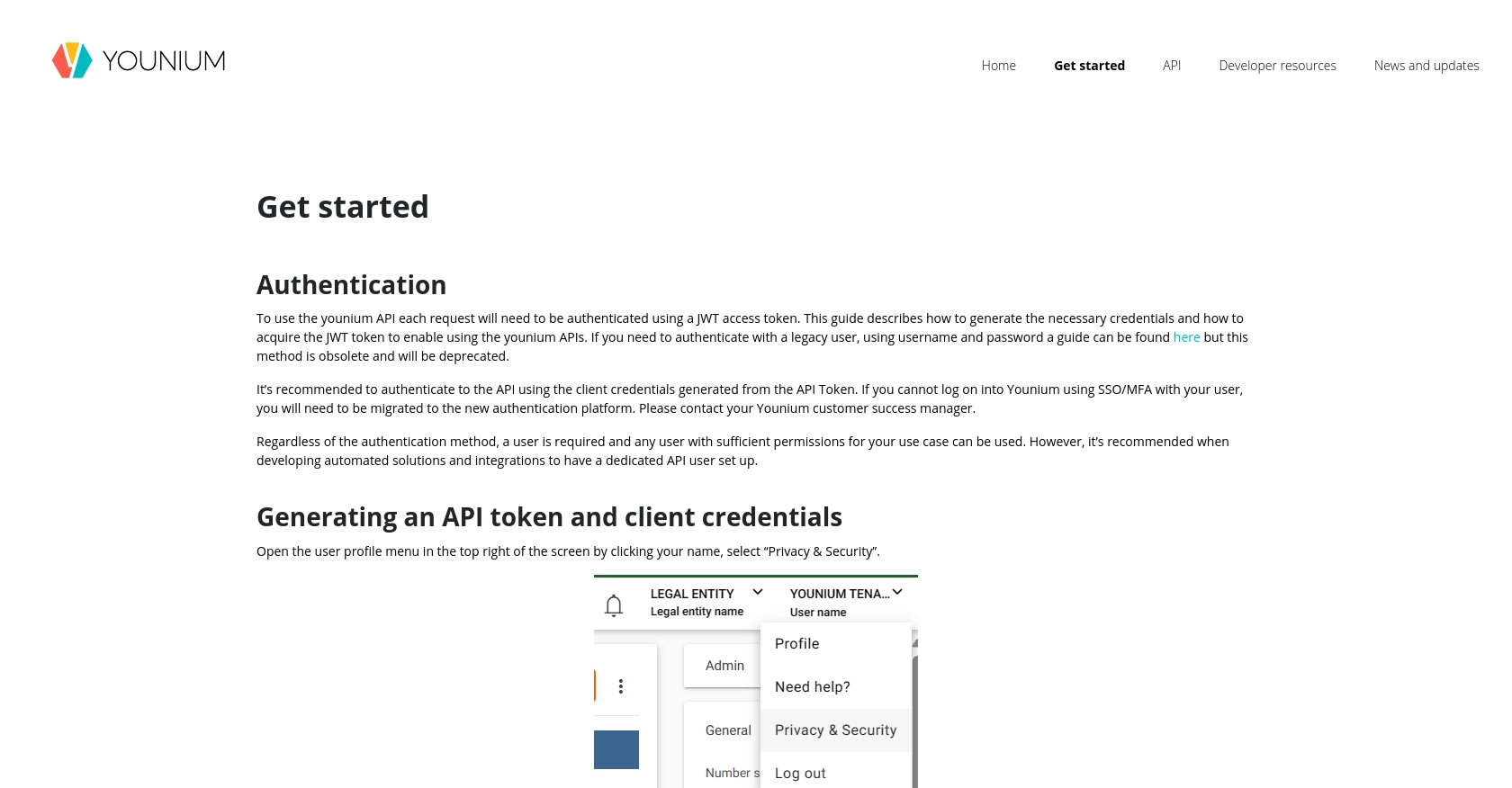
sbb-itb-96038d7
Making API Calls to Younium for Account Management Using Python
In this section, we will explore how to interact with the Younium API to create or update accounts using Python. This involves setting up the necessary environment, writing the code to make API requests, and handling potential errors effectively.
Setting Up Your Python Environment for Younium API Integration
Before making API calls, ensure you have Python 3.x installed on your machine. Additionally, you'll need the requests
library to handle HTTP requests. You can install it using pip:
pip install requests
Creating or Updating Accounts with Younium API
Once your environment is ready, you can proceed to create or update accounts in Younium. Below is a sample Python script to achieve this:
import requests
# Define the API endpoint for creating or updating accounts
url = "https://api.sandbox.younium.com/accounts"
# Set the headers, including the JWT access token
headers = {
"Authorization": "Bearer Your_Access_Token",
"Content-Type": "application/json",
"api-version": "2.1", # Optional but recommended
"legal-entity": "Your_Legal_Entity"
}
# Define the account data to be created or updated
account_data = {
"name": "Example Company",
"email": "contact@example.com",
"phone": "123-456-7890"
}
# Make the POST request to create or update the account
response = requests.post(url, json=account_data, headers=headers)
# Check the response status and handle errors
if response.status_code == 201:
print("Account created or updated successfully.")
else:
print(f"Failed to create or update account. Status Code: {response.status_code}")
print("Error:", response.json())
Replace Your_Access_Token
and Your_Legal_Entity
with the appropriate values. This script sends a POST request to the Younium API to create or update an account with the specified data.
Verifying API Call Success in Younium Sandbox
After running the script, you can verify the success of the API call by checking the Younium sandbox environment. The newly created or updated account should appear in your account list.
Handling Errors and Common Issues with Younium API
When interacting with the Younium API, you may encounter errors. Here are some common error codes and their meanings:
- 400 Bad Request: Indicates a malformed request. Check the request body and headers.
- 401 Unauthorized: The access token is missing, expired, or incorrect. Ensure your token is valid.
- 403 Forbidden: The request is authorized, but the action is forbidden. Verify the legal entity and permissions.
For more detailed error handling, refer to the Younium API Documentation.
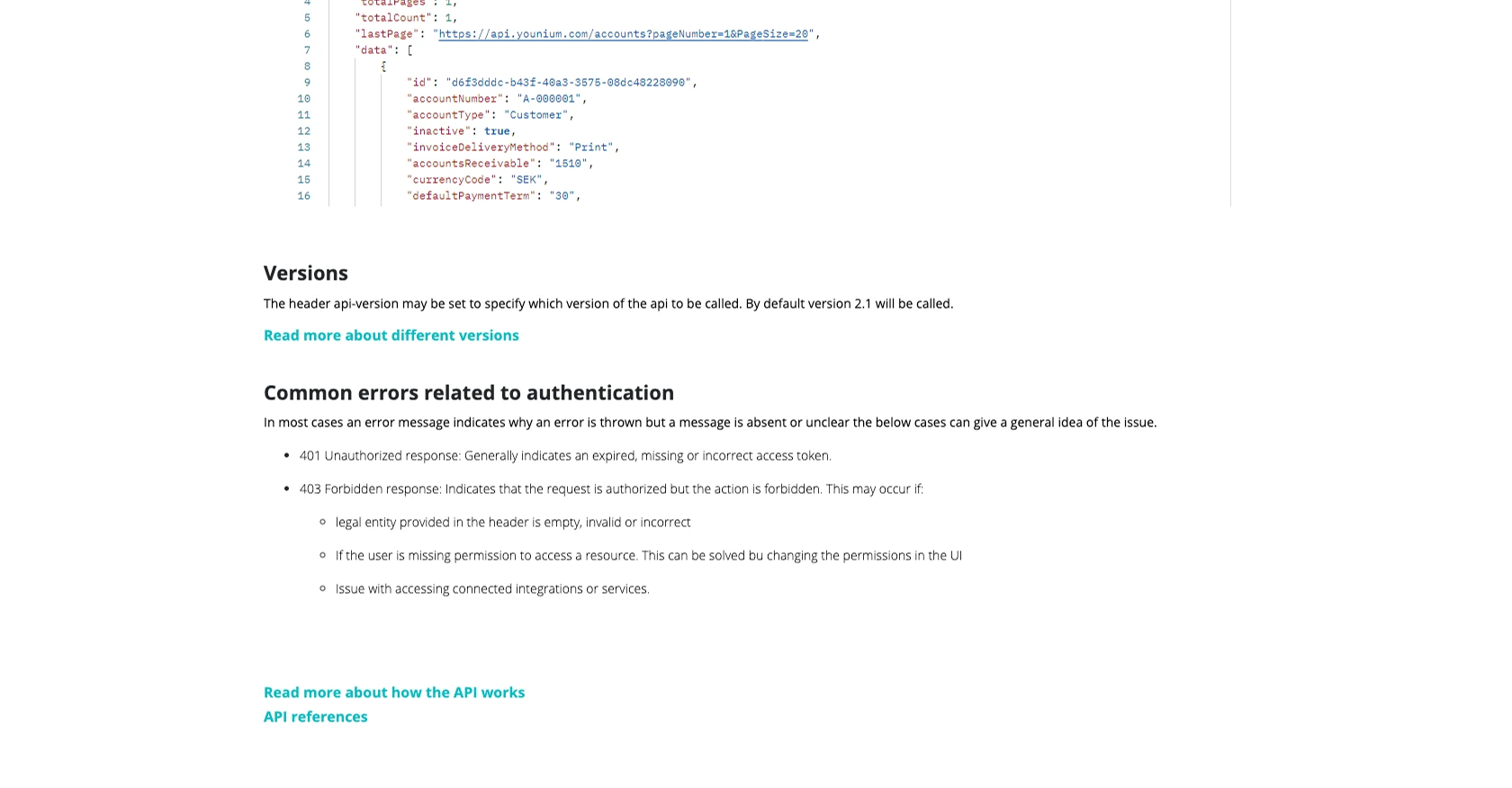
Conclusion and Best Practices for Younium API Integration
Integrating with the Younium API to manage accounts can significantly enhance your financial operations by automating account updates and ensuring data accuracy. By following the steps outlined in this guide, you can efficiently set up your environment, authenticate requests, and handle potential errors.
Best Practices for Secure and Efficient Younium API Usage
- Secure Storage of Credentials: Always store your client credentials and JWT tokens securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handling Rate Limits: Be mindful of any rate limits imposed by the API. Implement retry logic with exponential backoff to handle rate limiting gracefully.
- Data Standardization: Ensure that the data you send to Younium is standardized and validated to prevent errors and maintain data integrity.
- Regular Token Refresh: Since JWT tokens are valid for 24 hours, implement a mechanism to refresh tokens automatically to avoid authentication failures.
Streamlining Integrations with Endgrate
While integrating with individual APIs like Younium can be rewarding, it can also be time-consuming and complex, especially when managing multiple integrations. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Younium.
By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development. Whether you're building integrations for Younium or other platforms, Endgrate offers an intuitive and efficient solution to streamline your integration efforts.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?