Using the Hubspot API to Create or Update Contacts in PHP
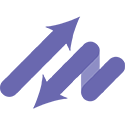
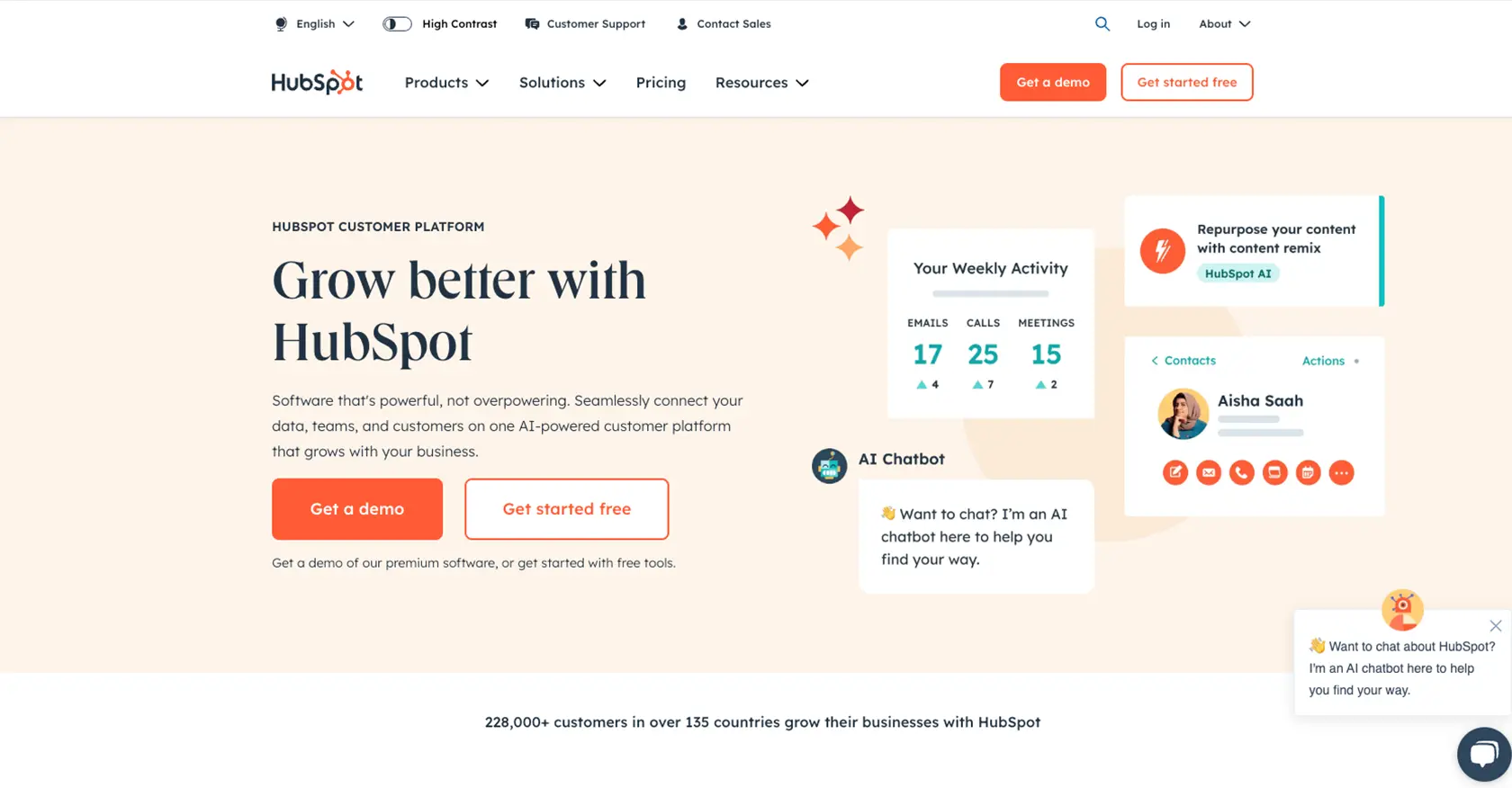
Introduction to HubSpot API for Contact Management
HubSpot is a comprehensive CRM platform that offers a suite of tools to help businesses manage their marketing, sales, and customer service efforts. Known for its user-friendly interface and robust features, HubSpot is a popular choice for businesses looking to streamline their customer relationship management processes.
Developers often integrate with HubSpot's API to automate and enhance customer data management. By connecting with HubSpot's API, developers can efficiently create or update contact information, ensuring that customer records are always up-to-date and accurate. This integration can be particularly useful for businesses that need to sync contact data across multiple platforms or automate marketing campaigns based on customer interactions.
In this article, we will explore how to use the HubSpot API to create or update contacts using PHP. This guide will provide step-by-step instructions and example code to help developers seamlessly integrate HubSpot's contact management capabilities into their applications.
Setting Up Your HubSpot Developer Account and Sandbox Environment
Before you can start integrating with the HubSpot API using PHP, you'll need to set up a HubSpot developer account and create a sandbox environment. This will allow you to test your API interactions without affecting live data.
Creating a HubSpot Developer Account
If you don't already have a HubSpot developer account, follow these steps to create one:
- Visit the HubSpot Developer Portal.
- Click on "Create a developer account" and fill out the necessary information.
- Once your account is created, log in to access the developer dashboard.
Setting Up a HubSpot Sandbox Account
HubSpot provides a sandbox environment for testing API integrations. Here's how to set it up:
- In your HubSpot developer account, navigate to the "Test Accounts" section.
- Click on "Create a test account" to generate a sandbox environment.
- Follow the prompts to configure your sandbox settings.
Configuring OAuth Authentication for HubSpot API
HubSpot uses OAuth for secure API authentication. To set this up, you'll need to create a private app:
- In your HubSpot account, go to "Settings" and select "Private Apps" under the "Integrations" section.
- Click on "Create a private app" and provide the necessary details.
- Under "Scopes," ensure you select the appropriate permissions for managing contacts, such as "crm.objects.contacts.read" and "crm.objects.contacts.write."
- Once the app is created, you'll receive a client ID and client secret. Keep these credentials secure as you'll need them to authenticate API requests.
For more detailed information on OAuth setup, refer to the HubSpot OAuth Documentation.
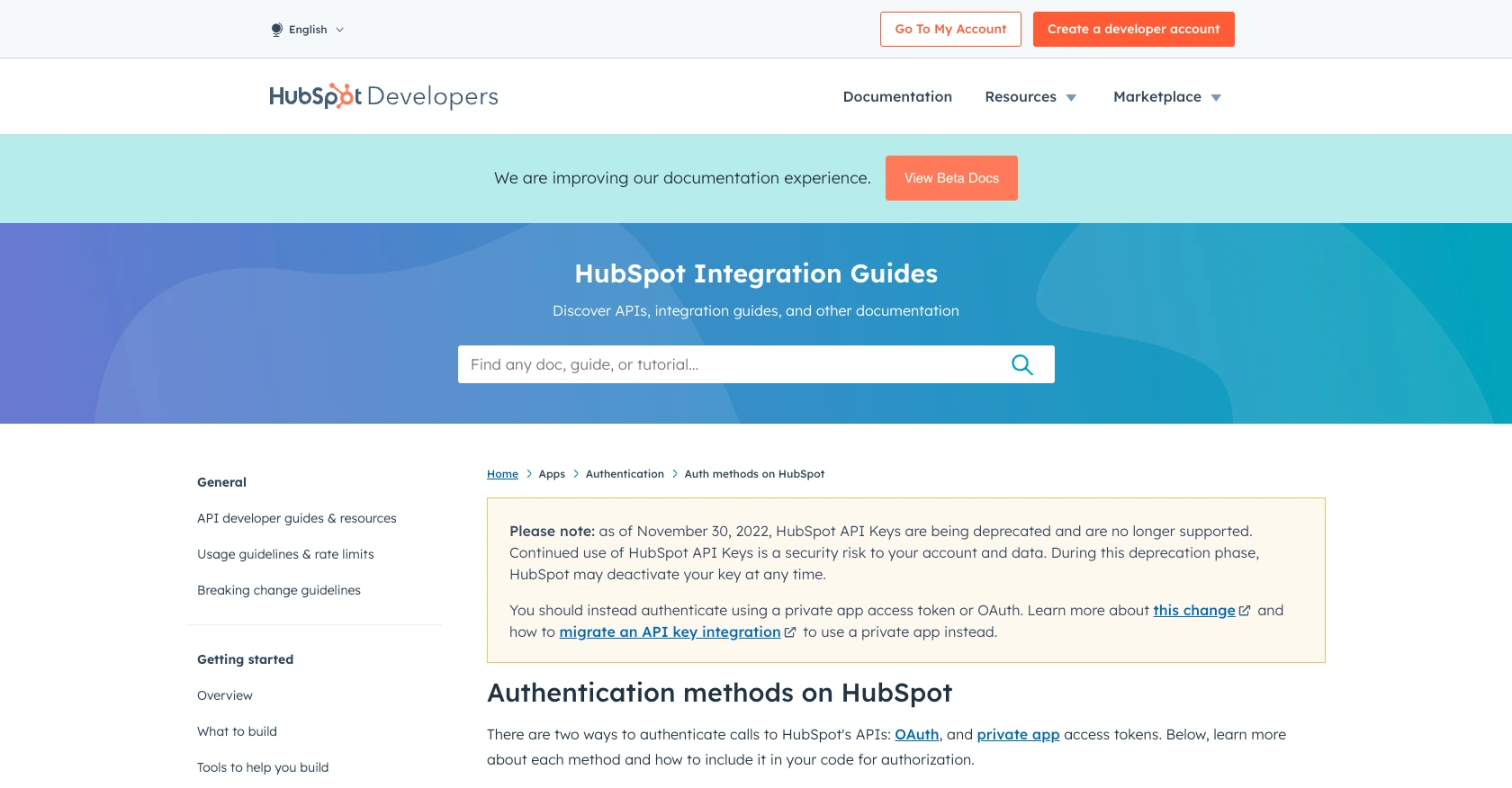
sbb-itb-96038d7
Making API Calls to HubSpot for Creating or Updating Contacts in PHP
To interact with the HubSpot API using PHP, you need to ensure you have the right environment set up. This section will guide you through the necessary steps to make API calls for creating or updating contacts in HubSpot using PHP.
Setting Up Your PHP Environment for HubSpot API Integration
Before making API calls, ensure your PHP environment is properly configured. You will need:
- PHP 7.4 or higher
- Composer for dependency management
Install the HubSpot API client for PHP using Composer:
composer require hubspot/api-client
Creating or Updating Contacts Using HubSpot API in PHP
With your environment ready, you can now create or update contacts in HubSpot. Below is a step-by-step guide with example code.
Example Code for Creating a Contact
To create a new contact, you will use the POST
method to send data to the HubSpot API. Here's how:
require 'vendor/autoload.php';
use HubSpot\Factory;
$client = Factory::createWithAccessToken('YOUR_ACCESS_TOKEN');
$contactData = [
'properties' => [
'email' => 'example@hubspot.com',
'firstname' => 'John',
'lastname' => 'Doe',
'phone' => '(555) 555-5555'
]
];
try {
$response = $client->crm()->contacts()->basicApi()->create($contactData);
echo "Contact created with ID: " . $response->getId();
} catch (Exception $e) {
echo "Error: " . $e->getMessage();
}
Replace YOUR_ACCESS_TOKEN
with your OAuth access token. This code creates a new contact with the specified properties.
Example Code for Updating a Contact
To update an existing contact, use the PATCH
method. You need the contact's ID or email:
$contactId = 'CONTACT_ID'; // Replace with actual contact ID
$updateData = [
'properties' => [
'phone' => '(555) 123-4567'
]
];
try {
$response = $client->crm()->contacts()->basicApi()->update($contactId, $updateData);
echo "Contact updated successfully.";
} catch (Exception $e) {
echo "Error: " . $e->getMessage();
}
Ensure you replace CONTACT_ID
with the actual ID of the contact you wish to update.
Verifying API Call Success and Handling Errors
After making an API call, verify its success by checking the response. If successful, the contact should appear in your HubSpot sandbox environment. Handle errors using try-catch blocks as shown in the examples. Common error codes include:
- 401 Unauthorized: Check your OAuth token.
- 404 Not Found: Verify the contact ID or email.
- 429 Too Many Requests: You have hit the rate limit. Refer to the HubSpot API Rate Limits for more information.
For more details on error handling, refer to the HubSpot Contacts API Documentation.
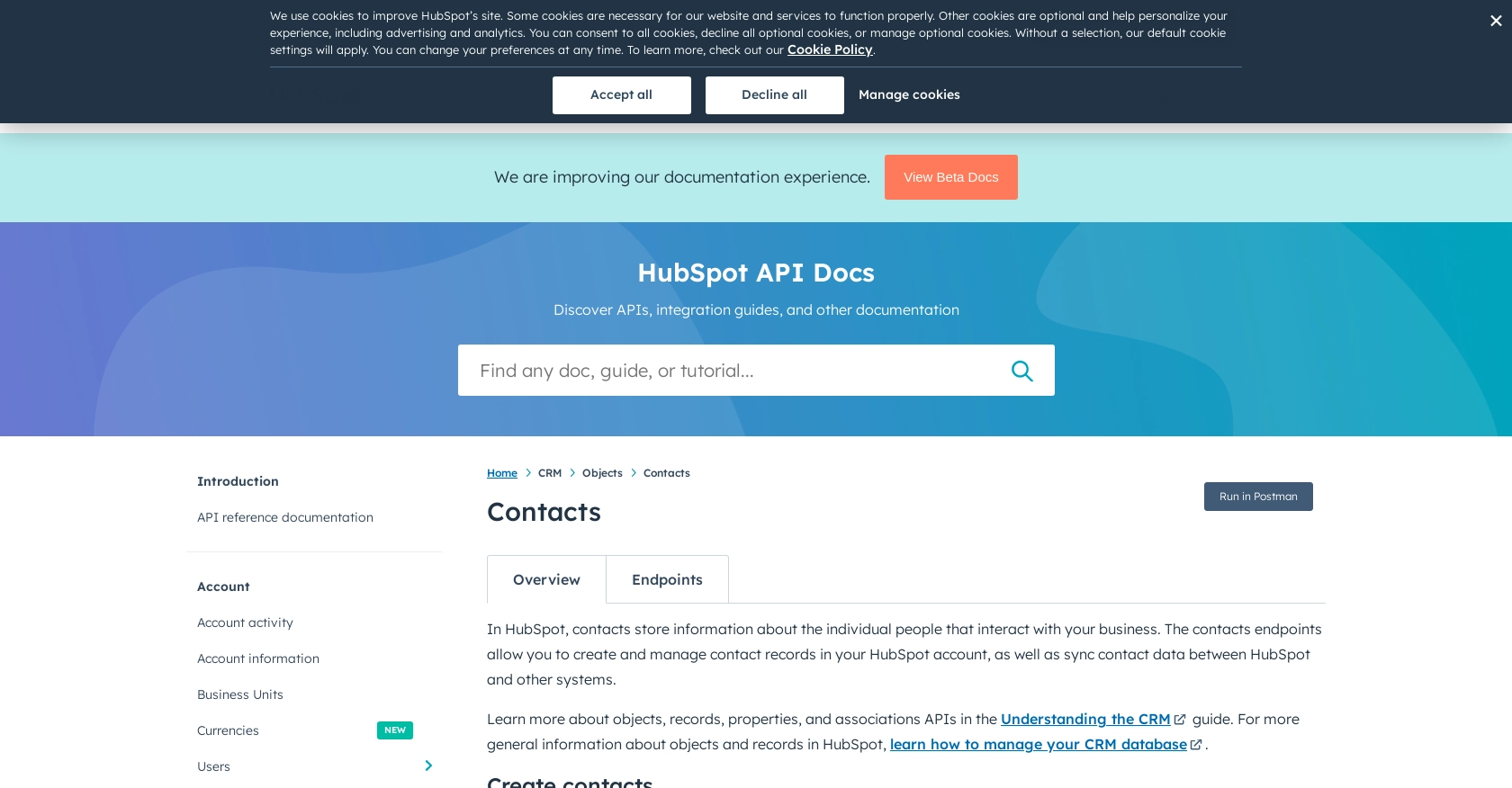
Conclusion and Best Practices for HubSpot API Integration in PHP
Integrating with the HubSpot API using PHP allows developers to efficiently manage contact data, ensuring that customer information is always current and accurate. By leveraging HubSpot's robust API, businesses can automate data synchronization across platforms, enhancing their marketing and customer relationship management efforts.
When working with the HubSpot API, it's crucial to follow best practices to ensure security and efficiency:
- Securely Store Credentials: Always store your OAuth access tokens securely and never hard-code them in your application. Consider using environment variables or a secure vault.
- Handle Rate Limits: Be mindful of HubSpot's rate limits, which allow 100 requests every 10 seconds for OAuth apps. Implement request throttling and error handling to manage 429 errors effectively. For more details, refer to the HubSpot API Rate Limits.
- Data Standardization: Ensure that data fields are standardized across your applications to maintain consistency and avoid errors during data synchronization.
- Error Handling: Implement robust error handling using try-catch blocks to manage exceptions and provide meaningful feedback to users.
By following these best practices, developers can create seamless integrations with HubSpot, enhancing their applications' functionality and user experience.
Streamline Your Integrations with Endgrate
If you're looking to simplify your integration processes, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers. Explore the possibilities with Endgrate by visiting Endgrate.
Read More
- https://endgrate.com/provider/hubspot
- https://developers.hubspot.com/docs/api/overview
- https://developers.hubspot.com/docs/api/intro-to-auth
- https://developers.hubspot.com/docs/api/usage-details
- https://developers.hubspot.com/docs/api/scopes
- https://developers.hubspot.com/docs/api/crm/contacts
- https://developers.hubspot.com/docs/api/crm/properties
Ready to get started?