Using the ZoomInfo API to Get Contacts in Python
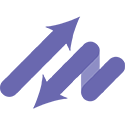
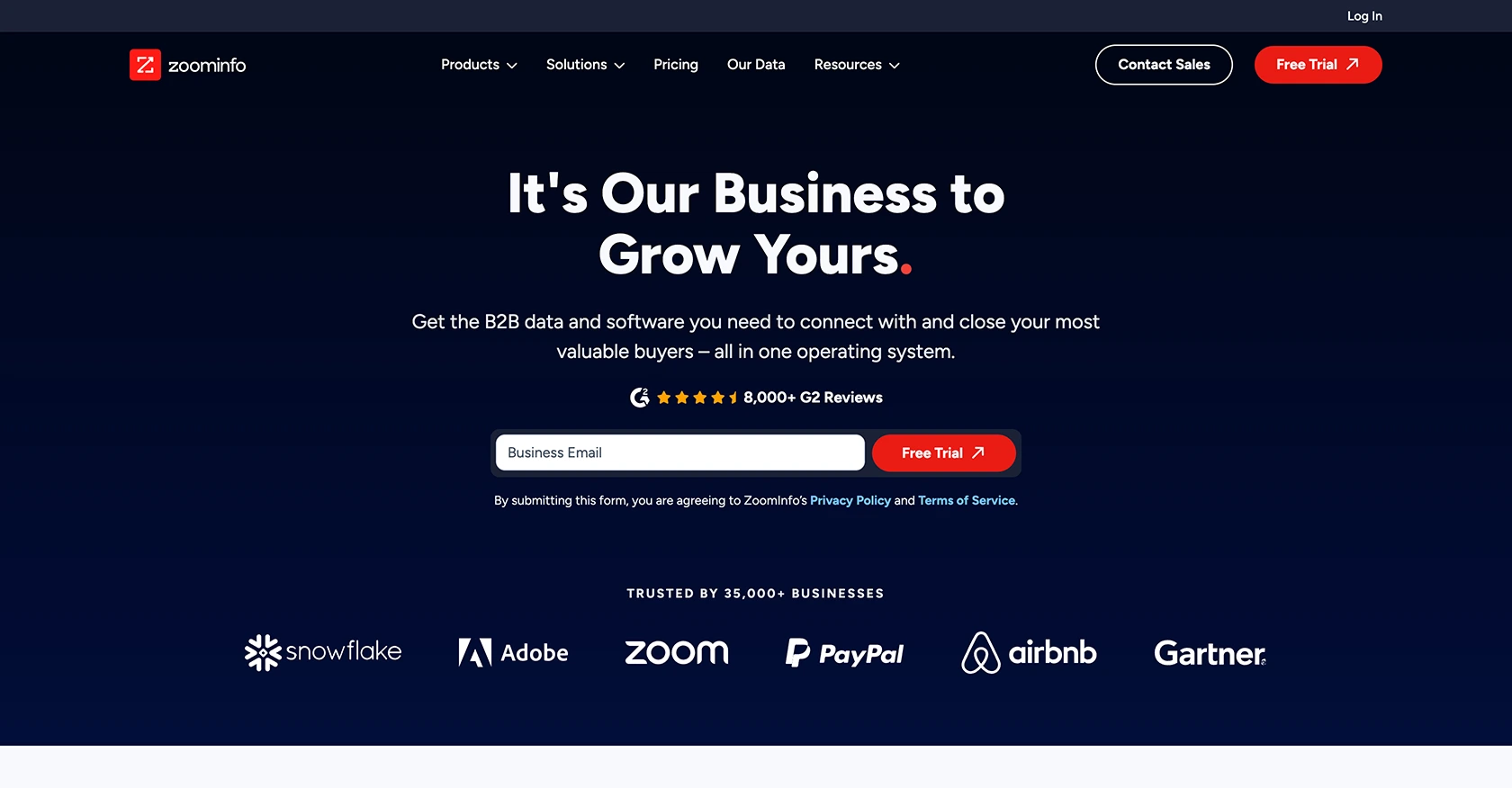
Introduction to ZoomInfo API
ZoomInfo is a powerful business intelligence platform that provides comprehensive data solutions to help companies identify, connect, and engage with their target audiences. With its vast database of business contacts and company information, ZoomInfo is an essential tool for sales and marketing teams looking to enhance their outreach strategies.
For developers, integrating with the ZoomInfo API offers the opportunity to access and manage detailed contact information programmatically. This can be particularly useful for automating lead generation processes or enriching CRM data with up-to-date contact details. For example, a developer might use the ZoomInfo API to retrieve a list of potential leads and automatically populate a sales dashboard, streamlining the workflow for sales representatives.
Setting Up Your ZoomInfo Test or Sandbox Account
Before you can start using the ZoomInfo API to retrieve contact information, you need to set up a test or sandbox account. This will allow you to experiment with the API without affecting live data. ZoomInfo provides developers with the necessary tools to create and manage sandbox environments, ensuring a smooth integration process.
Creating a ZoomInfo Developer Account
To begin, you'll need to sign up for a ZoomInfo developer account. This account will give you access to the API documentation and the ability to create sandbox environments for testing purposes.
- Visit the ZoomInfo API documentation page.
- Follow the instructions to register for a developer account.
- Once registered, log in to your account to access the developer dashboard.
Generating API Credentials for ZoomInfo
ZoomInfo uses a custom authentication method to secure API access. You'll need to generate API credentials to authenticate your requests.
- Navigate to the API credentials section in your developer dashboard.
- Create a new set of credentials by providing the necessary information, such as application name and description.
- Once created, you'll receive a client ID and client secret. Keep these credentials secure, as they are required for API authentication.
Configuring OAuth Authentication for ZoomInfo API
ZoomInfo's API requires OAuth-based authentication. Here's how to set it up:
- In your developer dashboard, locate the OAuth settings.
- Configure the redirect URI and scopes required for your application.
- Use the client ID and client secret to obtain an access token. This token will be used in the header of your API requests.
For detailed instructions, refer to the ZoomInfo authentication documentation.
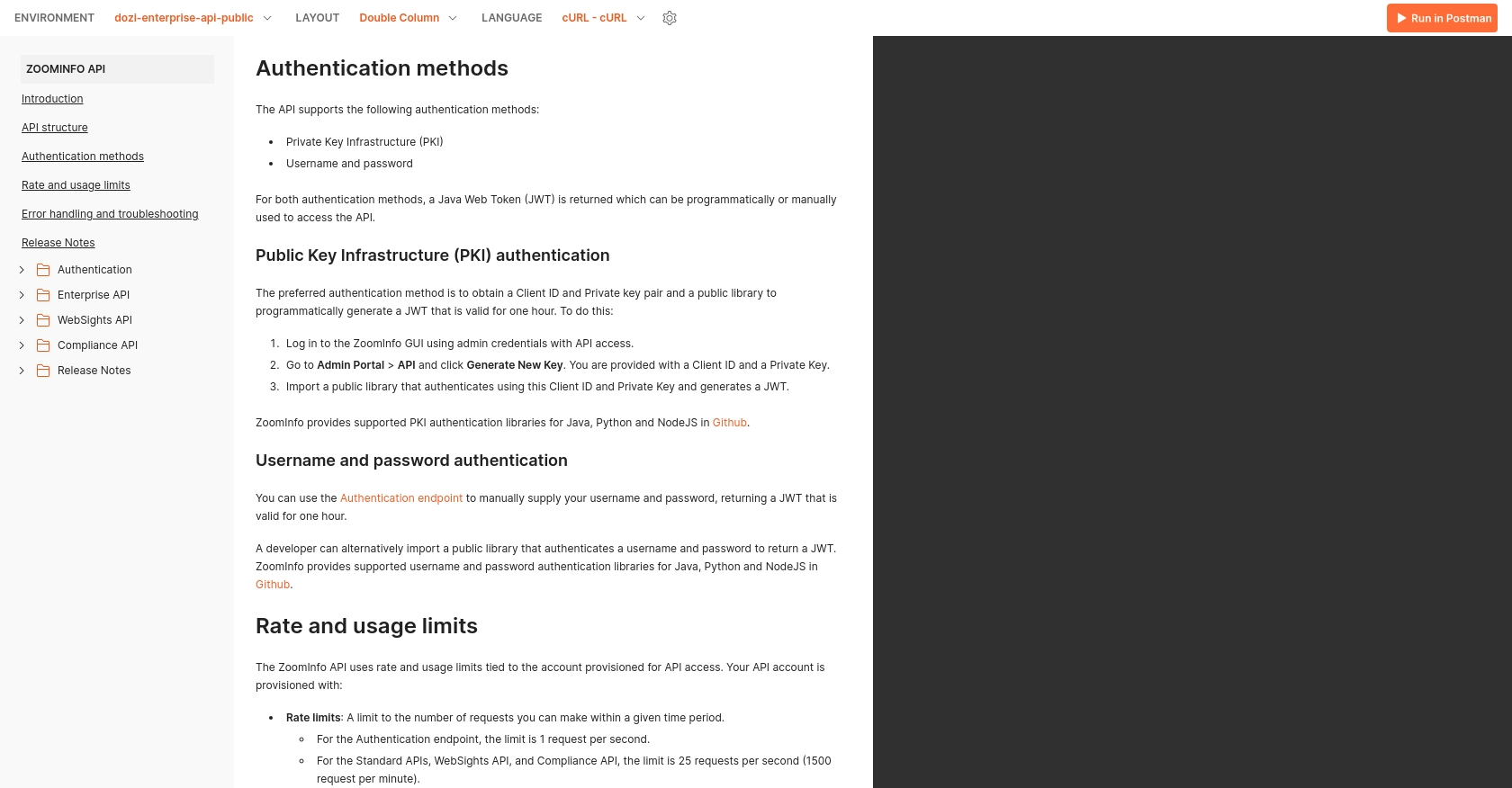
sbb-itb-96038d7
Making API Calls to ZoomInfo Using Python
To interact with the ZoomInfo API and retrieve contact information, you'll need to write a Python script that makes HTTP requests to the API endpoints. This section will guide you through the process of setting up your Python environment and making the necessary API calls.
Setting Up Your Python Environment for ZoomInfo API Integration
Before you begin, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need to install the requests
library, which simplifies making HTTP requests in Python.
- Install Python 3.11.1 from the official Python website if you haven't already.
- Use the following command to install the
requests
library:
pip install requests
Writing Python Code to Retrieve Contacts from ZoomInfo API
With your environment set up, you can now write a Python script to retrieve contacts from ZoomInfo. Follow these steps to create the script:
import requests
# Set the API endpoint and headers
endpoint = "https://api.zoominfo.com/contacts"
headers = {
"Authorization": "Bearer Your_Access_Token",
"Content-Type": "application/json"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
# Parse the JSON data from the response
data = response.json()
# Loop through the contacts and print their information
for contact in data["contacts"]:
print(contact)
else:
print(f"Failed to retrieve contacts: {response.status_code} - {response.text}")
Replace Your_Access_Token
with the access token obtained during the OAuth authentication setup. This token is required to authorize your API requests.
Verifying Successful API Calls and Handling Errors
After running your script, you should see the contact information printed in the console. This indicates that the API call was successful. If the request fails, the script will print an error message with the status code and response text, helping you diagnose any issues.
To verify the data, you can cross-check the returned contacts with those in your ZoomInfo sandbox account. This ensures that the API is correctly retrieving the expected data.
Handling ZoomInfo API Error Codes
When working with the ZoomInfo API, it's essential to handle potential errors gracefully. Common error codes include:
- 401 Unauthorized: Indicates that the access token is missing or invalid. Ensure your token is correct and hasn't expired.
- 403 Forbidden: Suggests that your account lacks the necessary permissions to access the resource.
- 404 Not Found: The requested resource could not be found. Verify the endpoint URL.
- 500 Internal Server Error: A server-side error occurred. Try the request again later.
For more detailed information on error handling, refer to the ZoomInfo API documentation.
Conclusion and Best Practices for ZoomInfo API Integration
Integrating with the ZoomInfo API allows developers to access a wealth of business contact information, streamlining processes like lead generation and CRM enrichment. By following the steps outlined in this guide, you can efficiently set up your environment, authenticate your requests, and retrieve valuable contact data using Python.
Best Practices for Secure and Efficient API Usage
- Secure Storage of Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Be aware of ZoomInfo's rate limits to avoid throttling. Implement exponential backoff strategies to handle rate limit errors gracefully. Refer to the ZoomInfo API documentation for specific rate limit details.
- Data Transformation and Standardization: Ensure that the data retrieved from ZoomInfo is transformed and standardized to fit your application's requirements. This can involve mapping fields and normalizing data formats.
Enhance Your Integration Strategy with Endgrate
While integrating with individual APIs like ZoomInfo can be powerful, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies integration management across various platforms, including ZoomInfo.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development. Build once for each use case and enjoy a seamless integration experience for your customers. Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website.
Read More
Ready to get started?